How to Retrieve the Current Date and Time Programmatically in Magento 2
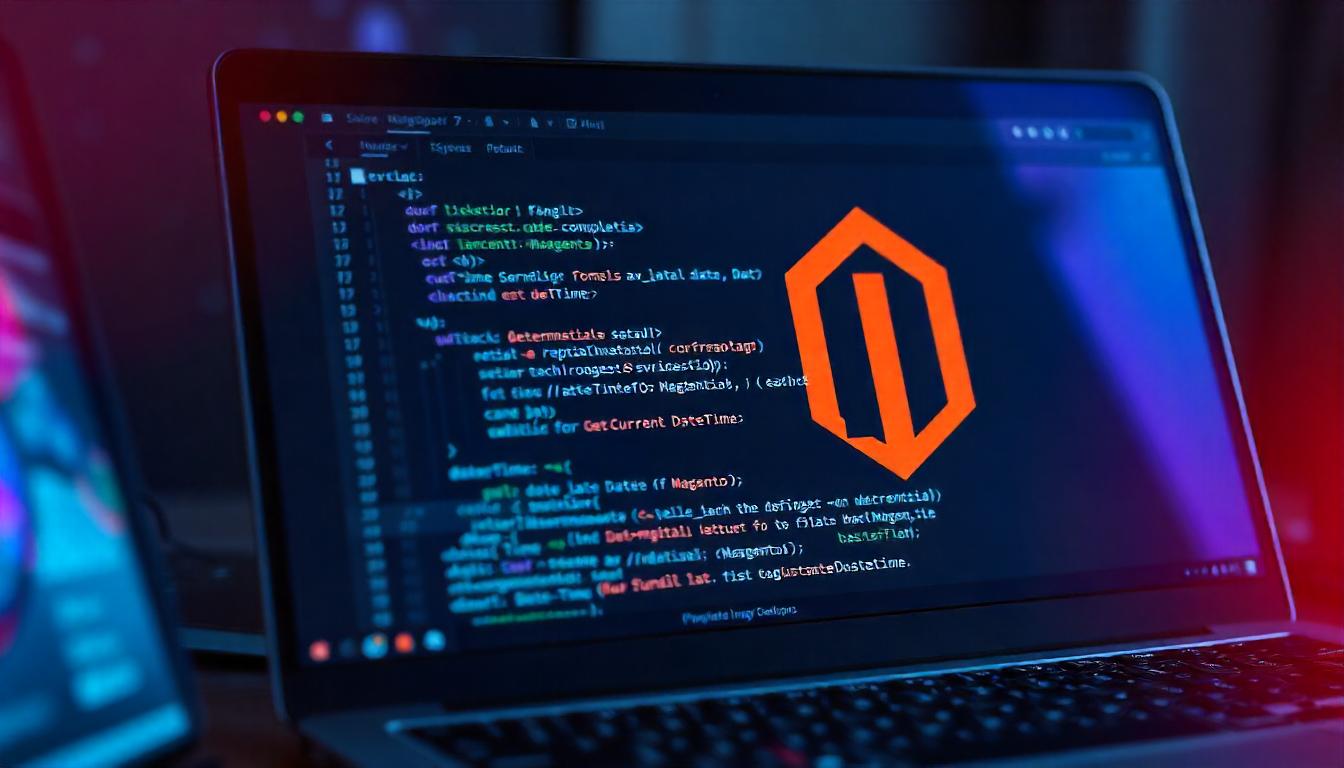
How to Retrieve the Current Date and Time Programmatically in Magento 2
Retrieving the current date and time programmatically in Magento 2 is a common requirement for various tasks, such as logging, scheduling, or adding timestamps to data. Magento 2 provides robust support for working with dates and times using its framework components. Here's a step-by-step guide on how to do it efficiently.
How to Retrieve the Current Date and Time Programmatically in Magento 2
To retrieve the current date and time in Magento 2, you can use the DateTime class. Here's a straightforward method:
public function getCurrentDateTime(): string
{
return (new \DateTime())->format('Y-m-d H:i:s');
}
Magento 2 offers several predefined date and time formats in the \Magento\Framework\Stdlib\DateTime
class:
Constant | Format | Example |
---|---|---|
DATETIME_PHP_FORMAT |
'Y-m-d H:i:s' | 2023-04-05 11:24:58 |
DATE_PHP_FORMAT |
'Y-m-d' | 2023-04-05 |
DATETIME_INTERNAL_FORMAT |
'yyyy-MM-dd HH:mm:ss' | 2023-04-05 11:24:58 |
DATE_INTERNAL_FORMAT |
'yyyy-MM-dd' | 2023-04-05 |
You can select any of these formats to suit your needs.
For more advanced date and time handling, such as working with time zones, Magento provides the \Magento\Framework\Stdlib\DateTime\TimezoneInterface.
This interface allows you to manage dates and times according to the store's configured time zone. Here's how you can use it:
use Magento\Framework\Stdlib\DateTime\TimezoneInterface;
class YourClass
{
protected $timezone;
public function __construct(TimezoneInterface $timezone)
{
$this->timezone = $timezone;
}
public function getStoreDateTime(): string
{
return $this->timezone->date()->format('Y-m-d H:i:s');
}
}
This method fetches the current store date and time in the 'Y-m-d H:i:s' format. Using TimezoneInterface ensures that the date and time are accurate according to the store's time zone settings.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Do You Retrieve the Current Date and Time Programmatically in Magento 2?
To retrieve the current date and time programmatically in Magento 2, use the DateTime()
method. This method fetches the date and time based on the server’s local time zone by default.
Which Method Should Be Used to Fetch the Current Date and Time?
Magento 2 uses the format()
method of the \DateTime
class to format the current date and time. A common format is 'Y-m-d H:i:s'
, which outputs something like '2023-04-05 11:24:58'.
Can You Retrieve GMT Date and Time in Magento 2?
Yes, you can retrieve GMT date and time in Magento 2. To do so, simply adjust the format and use Magento’s built-in timezone handling functionality to get the GMT time.
How Do You Format Date and Time in Magento 2?
In Magento 2, you can use predefined constants in the Magento\Framework\Stdlib\DateTime
class to format the date and time:
- DATETIME_PHP_FORMAT:
'Y-m-d H:i:s'
- DATE_PHP_FORMAT:
'Y-m-d'
- DATETIME_INTERNAL_FORMAT:
'yyyy-MM-dd HH:mm:ss'
- DATE_INTERNAL_FORMAT:
'yyyy-MM-dd'
Can You Share a Code Example for Fetching the Current Date and Time?
format('Y-m-d H:i:s');
}
}
What Additional Methods Can Be Used for Date and Time Management?
For more advanced date and time management, including working with time zones, you can use the \Magento\Framework\Stdlib\DateTime\TimezoneInterface
class. This interface allows you to retrieve the date and time based on the store's configured time zone.
Why Should You Consider Using TimezoneInterface for Date and Time?
Using TimezoneInterface
ensures that the date and time you retrieve are accurate according to the store's time zone, making it ideal for global stores with different time zone settings.
Is This Method Scalable for Large Projects?
Yes, using Magento’s built-in date and time functionality is efficient and scalable, ensuring consistency and accuracy in large projects that require time-sensitive operations.
What Best Practices Should You Follow for Date and Time Management in Magento 2?
Follow these best practices:
- Use Magento’s predefined constants for consistent formatting.
- Ensure that time zone handling is considered, especially for stores with global customers.
- Handle exceptions properly to avoid errors during time-based operations.
What Additional Methods Can Be Used for Date and Time Management?
For more advanced date and time management, including working with time zones, you can use the \Magento\Framework\Stdlib\DateTime\TimezoneInterface
class. This interface allows you to retrieve the date and time based on the store's configured time zone.
Why Should You Consider Using TimezoneInterface for Date and Time?
Using TimezoneInterface
ensures that the date and time you retrieve are accurate according to the store's time zone, making it ideal for global stores with different time zone settings.
Is This Method Scalable for Large Projects?
Yes, using Magento’s built-in date and time functionality is efficient and scalable, ensuring consistency and accuracy in large projects that require time-sensitive operations.
What Best Practices Should You Follow for Date and Time Management in Magento 2?
Follow these best practices:
- Use Magento’s predefined constants for consistent formatting.
- Ensure that time zone handling is considered, especially for stores with global customers.
- Handle exceptions properly to avoid errors during time-based operations.