Ensuring SQL Query Security in Magento 2 with quoteInto()
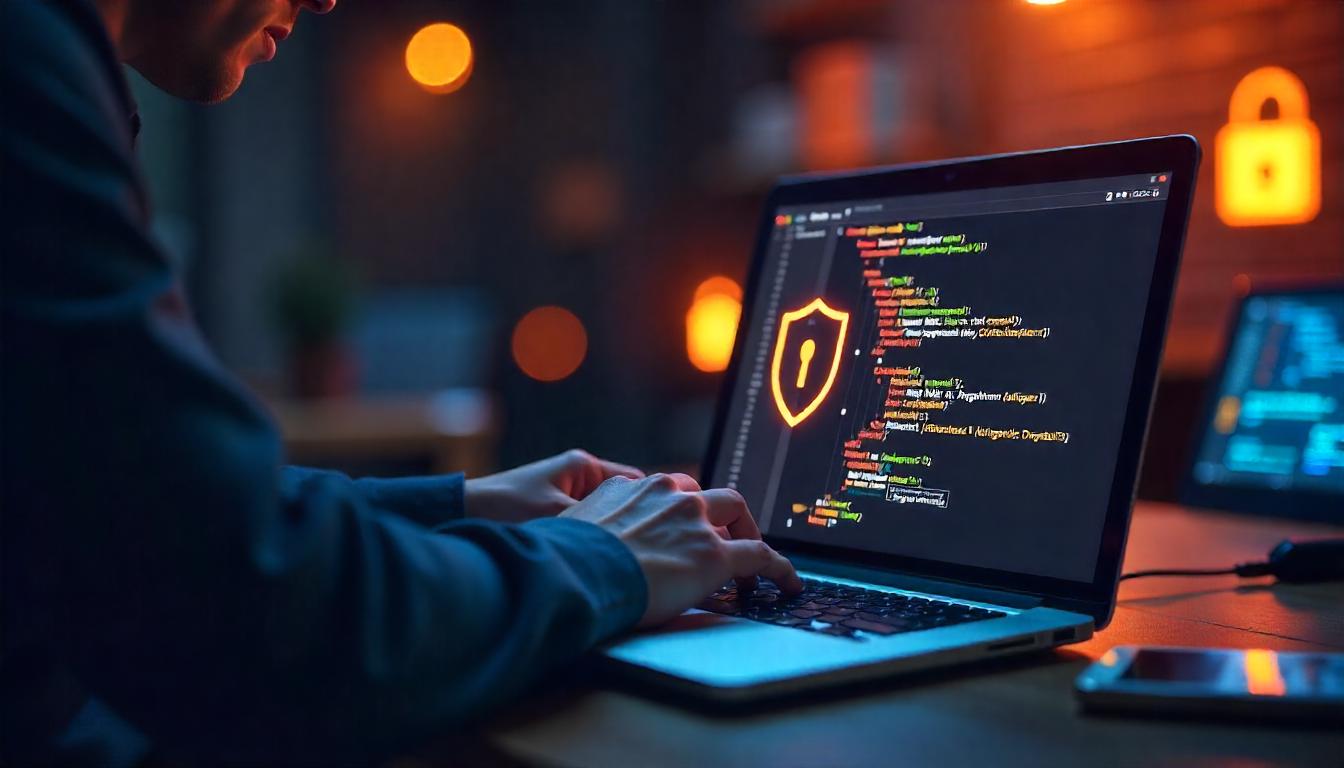
Ensuring SQL Query Security in Magento 2 with quoteInto()
SQL injection is a major security risk in any application. In Magento 2, the quoteInto() method helps prevent these attacks by safely quoting values in SQL queries. This method ensures user input is properly escaped before being inserted into database queries, reducing vulnerabilities.
Table Of Content
Ensuring SQL Query Security in Magento 2 with quoteInto()
To safeguard your Magento 2 application against SQL injection attacks, it's crucial to separate data values from SQL statements. Magento 2 offers the quoteInto()
method to help achieve this by safely quoting values within your queries.
Understanding the quoteInto() Method
The quoteInto()
method inserts a safely quoted value into a SQL statement at a placeholder. The placeholder is represented by a question mark (?), which gets replaced by the quoted value. This approach ensures that user inputs are properly escaped, mitigating the risk of SQL injection.
Method Definition
/**
* Inserts a quoted value into a SQL statement at the placeholder.
*
* @param string $text The SQL statement with a placeholder.
* @param mixed $value The value to quote and insert.
* @param string|null $type OPTIONAL SQL datatype
* @param int|null $count OPTIONAL number of placeholders to replace
* @return string The SQL statement with the quoted value inserted.
*/
public function quoteInto($text, $value, $type = null, $count = null);
Practical Example: Deleting Entries from core_config_data
Suppose you need to delete specific entries from the core_config_data
table. Using the quoteInto()
method ensures that the values in your SQL conditions are safely quoted.
<?php
namespace Your\Namespace\Model;
use Magento\Framework\App\ResourceConnection;
class ConfigDataManager
{
private const CORE_CONFIG_TABLE = 'core_config_data';
private ResourceConnection $resourceConnection;
public function __construct(ResourceConnection $resourceConnection)
{
$this->resourceConnection = $resourceConnection;
}
/**
* Deletes entries from the core_config_data table based on specified conditions.
*
* @param string $path The configuration path.
* @param string $scope The scope of the configuration (e.g., 'default', 'websites', 'stores').
* @param array $scopeIds An array of scope IDs to target.
* @return void
*/
public function deleteConfigEntries(string $path, string $scope, array $scopeIds): void
{
$connection = $this->resourceConnection->getConnection();
$tableName = $connection->getTableName(self::CORE_CONFIG_TABLE);
$conditions = [
$connection->quoteInto('path = ?', $path),
$connection->quoteInto('scope = ?', $scope),
$connection->quoteInto('scope_id IN (?)', $scopeIds)
];
$connection->delete($tableName, $conditions);
}
}
Key Points
- Secure Data Handling: By using
quoteInto()
, you ensure that data values are properly escaped, reducing the risk of SQL injection. - Placeholder Usage: The method replaces the question mark (?) in your SQL string with the safely quoted value.
- Versatility:
quoteInto()
can handle various data types and even multiple placeholders when specified.
Incorporating the quoteInto() method into your Magento 2 development practices is a straightforward way to enhance the security of your SQL queries. Always ensure that any user-supplied data is properly sanitized and quoted to maintain the integrity and security of your application.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is the Purpose of the quoteInto() Method in Magento 2?
The quoteInto()
method securely inserts quoted values into SQL statements, preventing SQL injection attacks.
How Does quoteInto() Prevent SQL Injection?
It replaces placeholders in SQL queries with properly escaped values, ensuring that user inputs do not alter query structure.
What Is the Syntax of quoteInto()?
public function quoteInto($text, $value, $type = null, $count = null);
It takes a SQL statement with a placeholder and safely inserts the quoted value.
Where Is quoteInto() Commonly Used in Magento 2?
It's commonly used in direct SQL queries, particularly in conditions within SELECT
, UPDATE
, and DELETE
statements.
How Can I Use quoteInto() in a Delete Query?
$connection->delete(
$tableName,
[
$connection->quoteInto('path = ?', $path),
$connection->quoteInto('scope = ?', $scope),
$connection->quoteInto('scope_id IN(?)', $scopeIds)
]
);
This ensures safe deletion by escaping values dynamically.
Can quoteInto() Handle Multiple Placeholders?
Yes, by passing an array as a value, you can replace multiple placeholders in a query safely.
Why Should I Use quoteInto() Instead of Concatenating Strings?
String concatenation is risky as it exposes queries to SQL injection. quoteInto()
ensures proper escaping and secure query execution.
Where Can I Learn More About SQL Security in Magento 2?
Check Magento’s official documentation for best practices on secure SQL queries and safe database interactions.