How to Get All Pending Reviews List in Magento 2.4.7
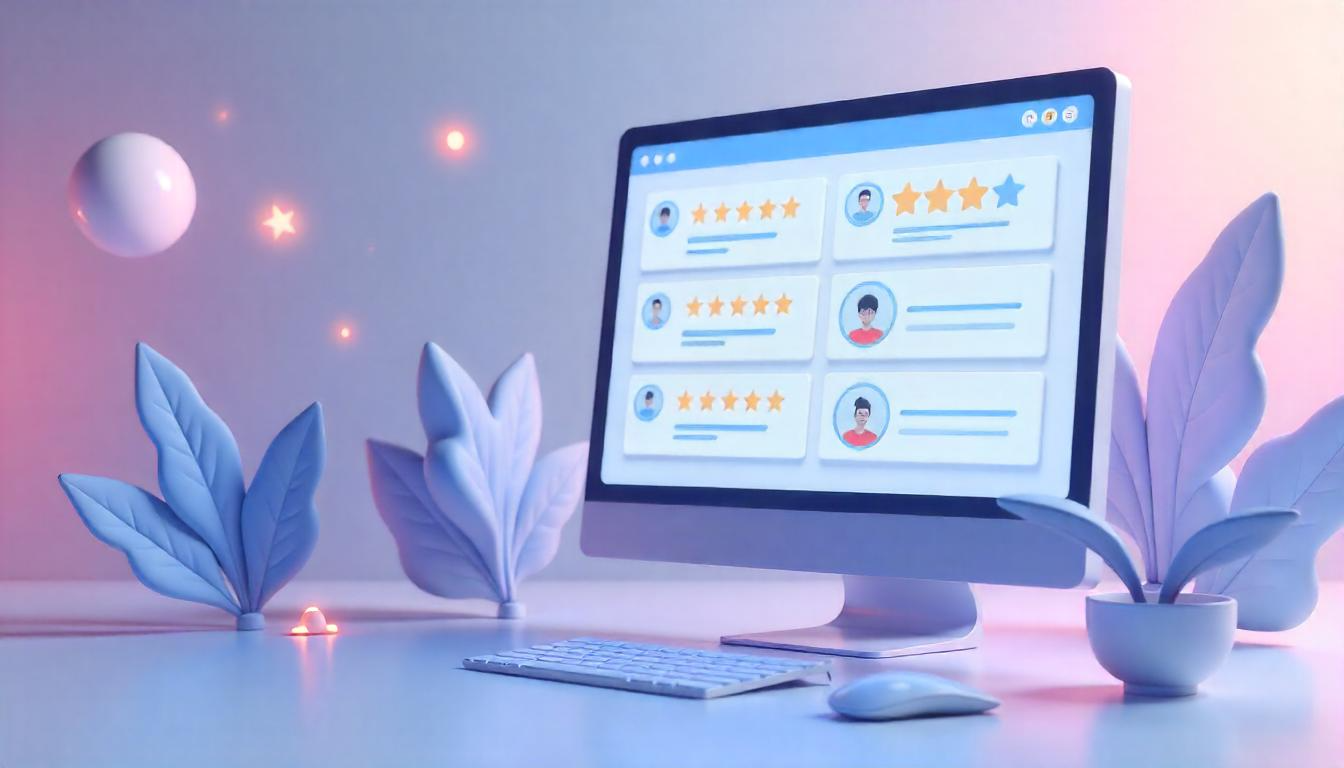
How to Get All Pending Reviews List in Magento 2.4.7
Managing customer reviews is a critical aspect of any eCommerce platform. Magento 2 provides a powerful review system where site administrators can view, approve, or modify customer reviews. In this guide, you will learn how to retrieve a list of all pending product reviews programmatically using Magento 2.4.7 (2025).
When a customer submits a review, it is stored with a status (e.g., pending, approved, or not approved). Pending reviews require administrator approval before they become publicly visible. This guide explains how to fetch all pending reviews so that the admin can review and process them accordingly.
Table Of Content
What Are Pending Reviews?
Pending reviews are customer reviews that have been submitted but have not yet been approved by the site administrator. These reviews are marked with a status of "pending" and remain hidden from the public until they are reviewed. Retrieving the list of pending reviews helps administrators manage and moderate customer feedback effectively, ensuring only high-quality content is displayed on the storefront.
Importance of Managing Pending Reviews
- Improves customer trust and brand reputation by ensuring only genuine feedback is published.
- Helps filter out spam and inappropriate content.
- Enables timely responses to customer feedback.
- Provides valuable insights into product performance and customer satisfaction.
Common Review Statuses
Status | Description |
---|---|
Pending | Reviews submitted by customers that are awaiting administrative approval. |
Approved | Reviews that have been approved by an administrator and are publicly visible. |
Rejected | Reviews that have been rejected due to spam or inappropriate content. |
Best Practices for Managing Pending Reviews:
>Administrators should regularly review pending reviews to ensure that customer feedback is moderated promptly. Implementing review notifications, automated spam filters, and moderation workflows can help streamline the process and maintain the quality of user-generated content.
Tips
Integrate automated moderation tools and set up email notifications to alert administrators when new reviews are submitted. This proactive approach minimizes manual workload and ensures that high-quality reviews are quickly approved for public display.
Magento 2 Review System Architecture
Magento 2 uses a structured approach to handling product and customer reviews. The system is built using a combination of models, factories, and resource collections to ensure scalability and flexibility.
Key Components
- Review Model (
Magento\Review\Model\Review
)- Manages individual review data such as ratings, customer details, and status.
- ReviewFactory (
Magento\Review\Model\ReviewFactory
)- Creates instances of the review model dynamically.
- Review Collection (
Magento\Review\Model\ResourceModel\Review\Collection
)- Provides a collection of review data with built-in filtering, sorting, and retrieval methods.
- Review Repository (
Magento\Review\Model\ReviewRepository
)- Enables fetching, updating, and deleting reviews using Magento's service contracts.
Database Tables Involved
Table Name | Description |
---|---|
review | Contains review records submitted by customers, including rating IDs and review titles. |
review_detail | Stores detailed review text, reviewer name, and customer-specific metadata. |
review_status | Defines various statuses such as pending, approved, and rejected. |
review_entity | Specifies the entity type being reviewed (e.g., product, customer). |
review_store | Maps reviews to specific store views in multi-store setups. |
review_entity_summary | Stores aggregate rating data for faster performance when retrieving average ratings. |
Review Statuses in Magento 2
>Magento categorizes reviews into multiple statuses, ensuring better control over what is displayed publicly.
Status | Description |
---|---|
Pending | New reviews waiting for moderation. |
Approved | Reviews that have been verified and published. |
Rejected | Reviews that did not pass moderation due to spam, irrelevance, or other violations. |
Best Practices for Managing Magento 2 Reviews
- Automate Moderation: Use third-party review moderation tools to flag spam or inappropriate content.
- Enable Email Notifications: Configure Magento to notify admins when a new review is submitted.
- Encourage Reviews: Send follow-up emails to customers requesting feedback after their purchase.
- Use Structured Data: Implement rich snippets to display review ratings in Google search results for better SEO.
Tip
To enhance user engagement, enable Magento’s built-in review reminder emails. This feature automatically reminds customers to leave a review after a purchase, improving the number of genuine reviews on your store.
Retrieving Pending Reviews in Magento 2
Magento 2's review system allows customers to submit feedback on products, which then undergoes moderation before appearing on the storefront. Pending reviews require admin approval before they become publicly visible. This guide explains how to retrieve pending reviews using a custom model and provides insights into Magento's review architecture.
Magento Review Workflow
Customer Submits a Review
- The review is stored in the database with a "Pending" status.
Admin Moderation
- Admins approve, reject, or edit the review via the Magento admin panel.
Review Publication
- Approved reviews are displayed on the storefront under the corresponding product.
Retrieving Pending Reviews Using a Custom Model
The following PHP code fetches pending reviews using ReviewFactory
and Magento’s review system.
Custom Model to Fetch Pending Reviews
<?php
namespace Vendor\Module\Model;
use Magento\Review\Model\Review;
use Magento\Review\Model\ReviewFactory;
use Magento\Review\Model\ResourceModel\Review\CollectionFactory;
class PendingReviews
{
private $reviewFactory;
private $reviewCollectionFactory;
public function __construct(
ReviewFactory $reviewFactory,
CollectionFactory $reviewCollectionFactory
) {
$this->reviewFactory = $reviewFactory;
$this->reviewCollectionFactory = $reviewCollectionFactory;
}
public function getPendingReviews()
{
$reviewCollection = $this->reviewCollectionFactory->create();
$reviewCollection->addFieldToFilter('status_id', Review::STATUS_PENDING)
->setOrder('created_at', 'DESC');
return $reviewCollection;
}
}
How to Fetch Pending Reviews in a Template
Call the getPendingReviews()
method to retrieve and display reviews dynamically.
$pendingReviews = $this->getPendingReviews();
if ($pendingReviews->getSize()) {
foreach ($pendingReviews as $review) {
echo "<pre>";
print_r($review->getData());
echo "</pre>";
}
} else {
echo "No pending reviews found.";
}
Magento 2 Review System Database Tables
Magento 2 stores review data across multiple tables for efficient organization and retrieval.
Table Name | Purpose |
---|---|
review | Stores the basic review details (e.g., rating, title, and customer ID). |
review_detail | Contains the full review text and metadata like reviewer name and store ID. |
review_status | Holds different review status values (Pending, Approved, Rejected). |
review_entity | Defines the type of entity being reviewed (product, customer, etc.). |
review_store | Associates reviews with store views in a multi-store Magento setup. |
review_aggregate | Stores pre-calculated rating summaries for performance optimization. |
customer_review | Maps reviews to registered customers for easy tracking. |
review_votes | Stores customer reactions to reviews (e.g., upvotes, downvotes). |
Magento 2 Review Status Codes
Magento assigns specific numeric values to each review status, which can be useful when filtering records in the database.
Status ID | Status Name | Description |
---|---|---|
1 | Pending | The review is awaiting admin approval. |
2 | Approved | The review has been published. |
3 | Rejected | The review has been rejected due to spam or other violations. |
To update a review’s status manually in MySQL:
UPDATE review SET status_id = 2 WHERE review_id = 10;
Best Practices for Managing Reviews Efficiently
Enable Email Notifications
- Configure Magento to notify admins when a new review is submitted.
Use Spam Filters
- Enable CAPTCHA for reviews to prevent spam.
Automate Review Moderation
- Use custom scripts to auto-approve reviews from verified buyers.
Sort and Filter Reviews
- Use the Magento admin panel to quickly moderate reviews by date, rating, or customer.
Pro Tip: Fetch Reviews Using SQL Queries
Retrieve all pending reviews directly from the database:
SELECT * FROM review WHERE status_id = 1 ORDER BY created_at DESC;
Find the total number of pending reviews:
SELECT COUNT(*) AS pending_count FROM review WHERE status_id = 1;
Filtering and Sorting Options in Magento Review Collection
Magento provides powerful methods to filter and sort review data efficiently. These methods help retrieve specific reviews based on various criteria.
Filtering Methods
addStatusFilter($status)
– Filters reviews based on their status (e.g., Pending, Approved, Rejected).addStoreFilter($storeId)
– Retrieves reviews for a specific store view, useful in multi-store setups.addCustomerFilter($customerId)
– Filters reviews by a specific customer ID.addEntityFilter($entityType, $entityId)
– Filters reviews by entity type (e.g., product, customer) and a specific entity ID.addVisibilityFilter()
– Retrieves reviews based on visibility settings, useful for restricting reviews to certain groups.
Sorting Methods
setDateOrder($direction = 'DESC')
– Sorts reviews based on submission date, defaulting to descending order.addRatingSummary()
– Retrieves reviews along with their aggregated rating summary for performance optimization.setPageSize($limit)
– Limits the number of reviews retrieved, improving query performance.
Customizing Filters for Specific Needs
Magento allows customization of review filters to suit different business requirements. Some common use cases include:
- Filtering reviews by customer group to target specific user segments.
- Displaying reviews only for specific product categories.
- Combining multiple filters to create a highly customized review collection for moderation or reporting.
Example: Retrieving Pending Reviews for a Specific Store
Below is an example of how to retrieve all pending reviews from a particular store:
$storeId = 1; // Example store ID
$status = Review::STATUS_PENDING;
$reviewCollection = $reviewFactory->create()->getProductCollection()
->addStatusFilter($status)
->addStoreFilter($storeId)
->setDateOrder();
Pro Tip:
Using setPageSize($limit)
improves performance by reducing the number of records retrieved at once, making database queries more efficient.
Best Practices and Tips for Managing Magento Reviews
Efficiently handling reviews in Magento requires following best practices to ensure performance, security, and reliability. Below are some expert recommendations:
1. Validation
- Ensure the collection is not empty before processing to avoid errors.
- Use
count($collection)
before looping through results to check if any reviews exist.
Example:
$reviewCollection = $reviewFactory->create()->getProductCollection()
->addStatusFilter(Review::STATUS_PENDING);
if ($reviewCollection->getSize() > 0) {
foreach ($reviewCollection as $review) {
echo $review->getTitle();
}
} else {
echo "No reviews found.";
}
2. Caching for Performance
- Cache frequently accessed review collections to reduce repeated database queries.
- Use Magento’s built-in caching mechanisms to store review lists for better efficiency.
Example: Store pending reviews in cache for 10 minutes
$cacheKey = 'pending_reviews_list';
$cache = $this->_cache; // Magento Cache Manager
if (!$reviews = $cache->load($cacheKey)) {
$reviews = $reviewFactory->create()->getProductCollection()
->addStatusFilter(Review::STATUS_PENDING)
->setPageSize(10);
$cache->save(serialize($reviews), $cacheKey, ['review_cache'], 600); // Store for 10 minutes
}
$reviews = unserialize($cache->load($cacheKey));
3. Error Handling
- Always wrap database queries in try-catch blocks to handle exceptions gracefully.
- Prevent crashes when filtering by invalid store IDs or non-existent review statuses.
Example: Error handling when retrieving reviews
try {
$reviewCollection = $reviewFactory->create()->getProductCollection()
->addStatusFilter(Review::STATUS_PENDING)
->addStoreFilter(2);
} catch (\Exception $e) {
error_log("Error fetching reviews: " . $e->getMessage());
}
4. Security & Access Control
- Restrict review management access to authorized users using Magento ACL (Access Control Lists).
- Define permissions in
acl.xml
to ensure that only admin users with proper roles can access review functionality.
Example: ACL configuration in acl.xml
<acl>
<resources>
<resource id="Magento_Review::reviews">
<resource id="Magento_Review::manage_reviews" title="Manage Reviews" />
</resource>
</resources>
</acl>
- Apply ACL checks in your controller using:
$this->authorization->isAllowed('Magento_Review::manage_reviews');
5. Performance Optimization
- Paginate large review collections to improve loading times and reduce memory usage.
- Avoid loading thousands of reviews at once—fetch them in batches using
setCurPage()
andsetPageSize()
.
Example: Paginating reviews
$reviewCollection = $reviewFactory->create()->getProductCollection()
->addStatusFilter(Review::STATUS_APPROVED)
->setCurPage(1)
->setPageSize(20); // Fetch 20 reviews per page
Index Optimization: Regularly reindex the review_aggregate
table to ensure faster review retrieval.
Pro Tip:
- Use AJAX Lazy Loading to load reviews dynamically instead of fetching all at once.
- Optimize SQL Queries by selecting only necessary fields instead of SELECT *.
- Enable Review Moderation to prevent spam before it appears on the storefront.
- Send Notifications to admins when a new review is pending approval.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
In summary, managing pending reviews in Magento 2 is a critical component of maintaining a high-quality, engaging online storefront. By leveraging Magento 2’s built-in review models and collections, you can efficiently retrieve and manage customer feedback, ensuring that only quality, approved reviews are published. With the improvements introduced in Magento 2.4.7 (2025), this process is more secure, efficient, and customizable than ever.
The comprehensive guide provided above covers the complete process—from understanding the importance of pending reviews and the underlying database architecture to implementing a custom model for fetching pending reviews programmatically. It also outlines essential best practices, including error handling, performance optimizations like caching and pagination, and security measures such as access control and proper validation.
Adopting these strategies not only streamlines the review moderation process but also enhances the overall customer experience. By keeping your system updated and following the best practices outlined, you ensure a robust review management workflow that contributes to better customer engagement and trust in your eCommerce platform.
Implement these techniques to transform your review moderation into a seamless and effective process, paving the way for improved content quality and a more dynamic shopping experience.
FAQs
What are pending reviews in Magento 2?
Pending reviews are customer reviews that have been submitted but have not yet been approved by the site administrator. They remain hidden until moderated.
Why are pending reviews important for a Magento 2 store?
Pending reviews allow administrators to moderate content before it is publicly displayed, ensuring quality control and protecting the brand's reputation.
How can I retrieve pending reviews programmatically in Magento 2?
You can retrieve pending reviews by creating a custom model that uses ReviewFactory
to load a review collection and then applying the addStatusFilter()
method with the pending status.
Which Magento model is used for fetching review data?
The Magento\Review\Model\Review
model is used to manage and retrieve review data, while the ReviewFactory
helps create review instances.
What method is used to filter reviews by pending status?
The addStatusFilter()
method is used on the review collection to filter reviews that have a pending status.
Can I filter pending reviews by store ID?
Yes, you can add a store filter using the addStoreFilter()
method on the review collection to limit the results to a specific store.
How can I sort the pending review list?
You can sort the pending review collection by date using the setDateOrder()
method, which orders reviews based on their submission date.
What should I do if there are no pending reviews?
If no pending reviews are found, you may display a message indicating that there are no reviews awaiting moderation or check if the filters are correctly applied.
Which database tables are involved in the review process?
Key tables include review
(for basic review data), review_detail
(for detailed review content), and review_status
(for review status definitions).
How can I approve a pending review from the admin panel?
Site administrators can log in to the Magento admin panel, navigate to the Reviews section, review the pending items, and change their status to approved.
What does the addStatusFilter()
method do?
This method filters the review collection to include only reviews that match the specified status, such as pending, approved, or not approved.
Is it possible to paginate the pending review collection?
Yes, you can paginate the review collection to handle large volumes of data efficiently, improving load times and performance.
How should I implement error handling when retrieving pending reviews?
It is recommended to use try-catch blocks to capture exceptions and provide meaningful error messages if the review retrieval process fails.
What are some best practices for managing pending reviews in Magento 2?
Best practices include validating review collections, caching results when possible, paginating large datasets, and using proper ACL permissions to secure review management.