Implementing the sales_quote_address_collect_totals_after Event in Magento 2
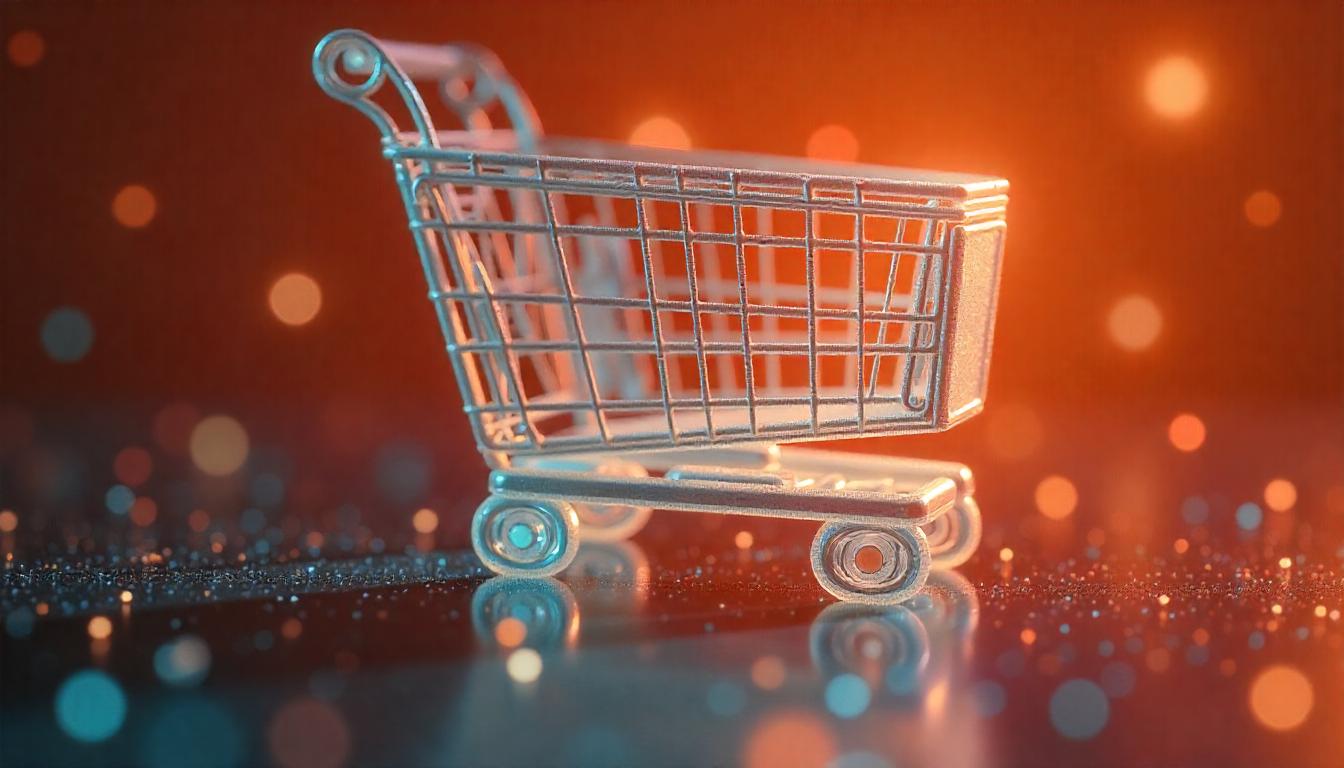
Implementing the sales_quote_address_collect_totals_after Event in Magento 2
The sales_quote_address_collect_totals_after event in Magento 2 allows you to modify quote data after the system calculates address totals. This is useful for customizing cart calculations, applying discounts, or adding custom logic post-total collection.
Implementing the sales_quote_address_collect_totals_after Event in Magento 2
In Magento 2, the sales_quote_address_collect_totals_after
event allows you to access and modify quote data after the system calculates totals for a quote address. This is useful for customizing cart calculations or adding custom logic post-total collection.
Setting Up the Event Observer
To use this event, you'll need to create an observer in your custom module. Here's how:
Define the Event in events.xml
:
Create or update the events.xml file in your module's etc directory to listen for the sales_quote_address_collect_totals_after event.
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Event/etc/events.xsd">
<event name="sales_quote_address_collect_totals_after">
<observer name="custom_totals_observer" instance="Vendor\Module\Observer\CustomTotalsObserver" />
</event>
</config>
Create the Observer Class:
Implement the logic to handle the event in the CustomTotalsObserver
class.
<?php
namespace Vendor\Module\Observer;
use Magento\Framework\Event\Observer;
use Magento\Framework\Event\ObserverInterface;
use Magento\Quote\Model\Quote;
class CustomTotalsObserver implements ObserverInterface
{
public function execute(Observer $observer)
{
// Retrieve the quote from the event observer
/** @var Quote $quote */
$quote = $observer->getEvent()->getQuote();
// Access the shipping assignment
$shippingAssignment = $observer->getEvent()->getShippingAssignment();
$address = $shippingAssignment->getShipping()->getAddress();
// Access the total object
$total = $observer->getEvent()->getTotal();
// Implement your custom logic here
// For example, adjust the total based on custom criteria
}
}
Key Components Available in the Observer:
- Quote Object (
$quote
): Represents the current quote, allowing access to items, customer data, and other quote-related information. - Shipping Assignment (
$shippingAssignment
): Contains shipping-related data, including the shipping address. - Total Object (
$total
): Holds the calculated totals for the quote address, which you can adjust as needed.
By leveraging the sales_quote_address_collect_totals_after
event, you can effectively customize the checkout process in Magento 2 to meet specific business requirements.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is the Purpose of the sales_quote_address_collect_totals_after Event in Magento 2?
The sales_quote_address_collect_totals_after
event allows developers to modify quote data after Magento calculates address totals, making it useful for custom pricing rules and additional calculations.
How Can I Use sales_quote_address_collect_totals_after in Magento 2?
You can listen to this event in an observer by defining it in the events.xml
file and implementing custom logic in an observer class.
What Is the Syntax for Defining This Event?
This code should be placed inside your module’s events.xml
file.
Where Should I Place the Observer Class?
The observer class should be placed inside Vendor\Module\Observer
and must implement ObserverInterface
.
How Do I Retrieve Quote Data Inside the Observer?
public function execute(Observer $observer)
{
$quote = $observer->getEvent()->getQuote();
}
You can use this method to access quote details for further modifications.
How Can I Fetch Address and Total Data Using This Event?
$shippingAssignment = $observer->getEvent()->getShippingAssignment();
$address = $shippingAssignment->getShipping()->getAddress();
$total = $observer->getEvent()->getTotal();
This lets you access the shipping address and total values within your custom logic.
What Are Some Use Cases for This Event?
Common use cases include modifying shipping costs, adding custom discounts, or implementing surcharge rules before finalizing totals.
Where Can I Learn More About Events in Magento 2?
Magento’s official documentation provides a detailed guide on events and observers for customizing checkout and quote calculations securely.