Retrieve RMA Attribute Options by Code in Magento 2
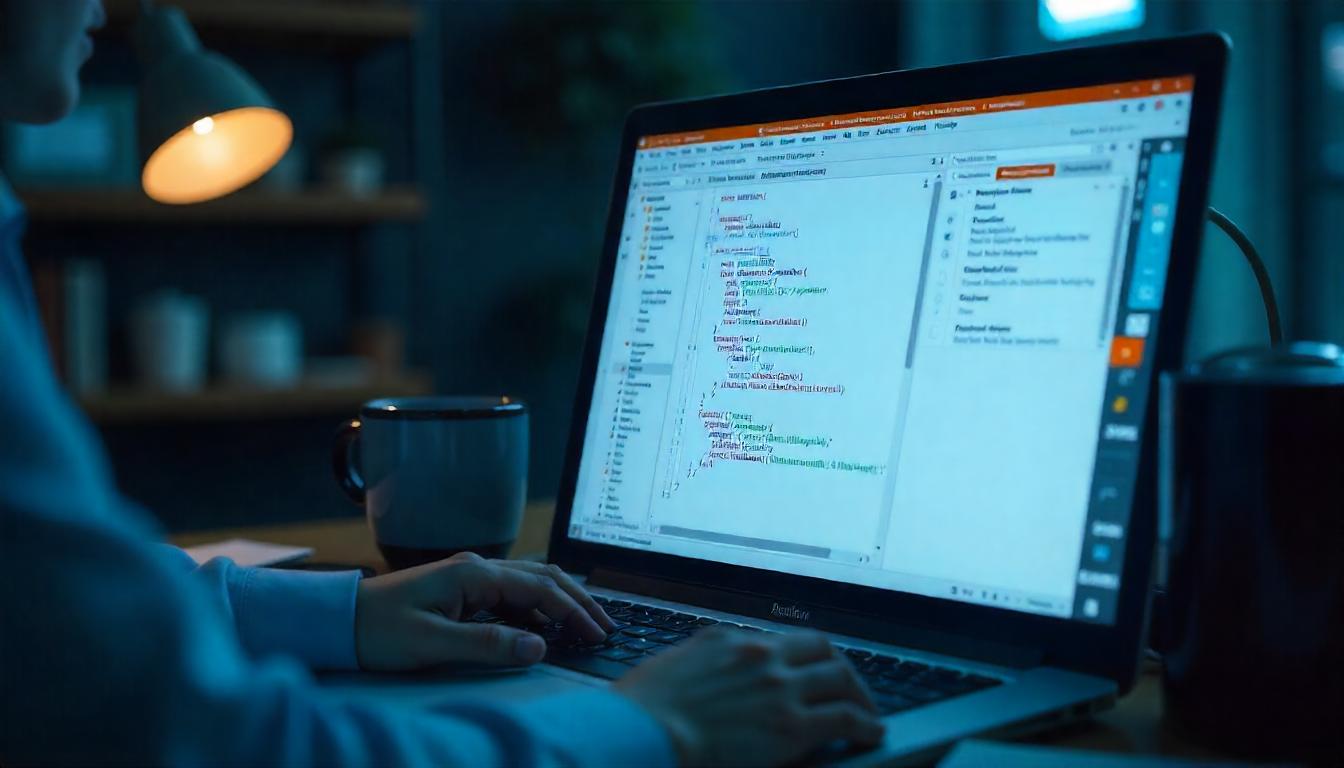
Retrieve RMA Attribute Options by Code in Magento 2
In Magento 2, you can fetch all available options for a specific Return Merchandise Authorization (RMA) attribute using the Magento\Eav\Api\AttributeOptionManagementInterface. This allows you to retrieve option IDs and their corresponding labels for attributes like condition, resolution, reason, and reason_other.
Table Of Content
Retrieve RMA Attribute Options by Code in Magento 2
To access all Return Merchandise Authorization (RMA) attribute options in Magento 2, utilize theMagento\Eav\Api\AttributeOptionManagementInterface
.Magento Commerce's native RMA includes four primary attribute codes: resolution
, condition
, reason
, and reason_other.
<?php
declare(strict_types=1);
namespace Vendor\Module\Model;
use Magento\Eav\Api\AttributeOptionManagementInterface;
use Magento\Rma\Api\RmaAttributesManagementInterface;
use Magento\Framework\Exception\InputException;
use Magento\Framework\Exception\StateException;
class RmaAttributeOption
{
private AttributeOptionManagementInterface $attributeOptionManagement;
public function __construct(
AttributeOptionManagementInterface $attributeOptionManagement
) {
$this->attributeOptionManagement = $attributeOptionManagement;
}
/**
* Retrieve all options for a given RMA attribute code.
*
* @param string $attributeCode
* @return array
* @throws InputException
* @throws StateException
*/
public function getRmaAttributeOptions(string $attributeCode): array
{
$options = $this->attributeOptionManagement->getItems(
RmaAttributesManagementInterface::ENTITY_TYPE,
$attributeCode
);
$rmaOptions = [];
foreach ($options as $option) {
$rmaOptions[$option->getValue()] = strtolower($option->getLabel());
}
return $rmaOptions;
}
}
Usage Example
To fetch options for the condition
attribute:
$rmaAttributeCode = 'condition';
$rmaOptions = $this->getRmaAttributeOptions($rmaAttributeCode);
Sample Output
Array
(
[7] => unopened
[9] => damaged
[8] => opened
)
In this array, keys represent option_id, and values are the corresponding option labels.
Additional Considerations
Note: Ensure the Magento_Rma module is enabled in your Magento 2 setup. If you've encountered issues with missing attributes, verify your module's installation and check for any errors during setup.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Are RMA Attribute Options in Magento 2?
RMA (Return Merchandise Authorization) attribute options define return reasons, conditions, and resolutions in Magento 2.
Which Attribute Codes Are Used in Magento 2 RMA?
Magento 2 RMA includes four main attribute codes:
resolution
condition
reason
reason_other
How Do I Retrieve RMA Attribute Options in Magento 2?
You can fetch RMA attribute options using the Magento\Eav\Api\AttributeOptionManagementInterface
interface.
What Code Snippet Retrieves RMA Attribute Options?
Use the following code snippet:
$options = $this->attributeOptionManagement->getItems(
RmaAttributesManagementInterface::ENTITY_TYPE,
$attributeCode
);
How Do I Fetch Options for a Specific RMA Attribute Code?
Call the function with the attribute code:
$rmaAttributeCode = 'condition';
$rmaOptions = $this->getRmaAttributeOptions($rmaAttributeCode);
What Is the Expected Output When Fetching RMA Options?
The output will be an array of option IDs and labels:
Array
(
[7] => unopened
[9] => damaged
[8] => opened
)
Why Are RMA Attribute Options Important?
They help define return conditions, making the RMA process smoother for admins and customers.
Where Can I Learn More About RMA Attribute Options?
Check Magento 2’s official documentation for more details on managing RMA attributes.