Retrieving Store URLs in Magento 2 JavaScript Files
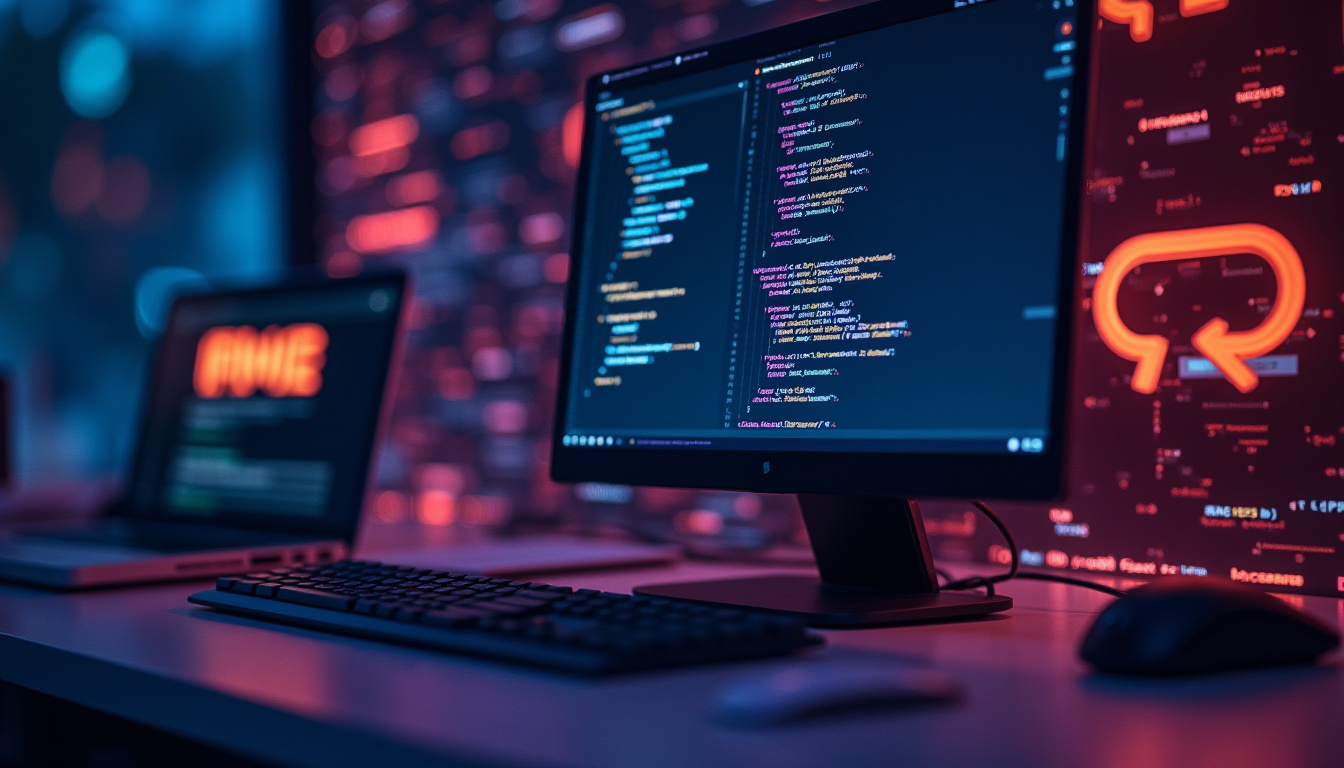
Retrieving Store URLs in Magento 2 JavaScript Files
Learn how to dynamically retrieve store URLs in Magento 2 JavaScript files using the 'mage/url' module. This guide covers fetching base and custom URLs for both frontend and backend areas, ensuring accurate and maintainable URL generation.
Table Of Content
Retrieving Store URLs in Magento 2 JavaScript Files
To access your store's base or custom URLs within Magento 2 JavaScript files, utilize the 'mage/url
' module. This approach ensures dynamic URL generation, accommodating any future changes to your store's structure.
Fetching URLs in Frontend JavaScript
In your JavaScript file, include 'mage/url
' as a dependency. Then, use the build method to construct the desired URL. Here's how:
define([
'mage/url'
], function (urlBuilder) {
// Generate the login page URL
var loginUrl = urlBuilder.build('customer/account/login');
console.log(loginUrl); // Outputs: http://yourstore.com/customer/account/login
});
Replace 'customer/account/login
' with any path relevant to your needs. The build method appends this path to your store's base URL, ensuring accuracy even if the base URL changes.
Accessing URLs in Backend (Admin) JavaScript
For admin-side JavaScript, the process differs slightly since 'mage/url' isn't configured for backend use. Instead, define the base URL in a template file and make it accessible to your JavaScript:
Create a Layout XML File
Define a block that renders a template containing your base URL.
<!-- File: app/code/YourVendor/YourModule/view/adminhtml/layout/default.xml -->
<?xml version="1.0"?>
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<body>
<referenceContainer name="js">
<block class="Magento\Backend\Block\Template" template="YourVendor_YourModule::js/base_url.phtml" name="base_url_block"/>
</referenceContainer>
</body>
</page>
Create the Template File
<!-- File: app/code/YourVendor/YourModule/view/adminhtml/templates/js/base_url.phtml -->
<script>
require(['mage/url'], function (urlBuilder) {
urlBuilder.setBaseUrl('= $block->getUrl('') ?>');
});
</script>
Here, = $block->getUrl('') ?>
outputs the admin base URL, which is then set using urlBuilder.setBaseUrl().
Utilize the Base URL in Your JavaScript
In your custom JavaScript file, you can now build URLs relative to the admin base URL:
define([
'mage/url'
], function (urlBuilder) {
// Generate a custom admin URL
var customAdminUrl = urlBuilder.build('yourmodule/controller/action');
console.log(customAdminUrl); // Outputs: http://yourstore.com/admin/yourmodule/controller/action
});
By integrating the 'mage/url' module in your JavaScript files, you can dynamically generate both frontend and backend URLs in Magento 2. This method ensures your URLs remain accurate and maintainable, adapting seamlessly to any changes in your store's configuration.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Do I Retrieve Store URLs in Magento 2 JavaScript Files?
Use the 'mage/url'
module to generate dynamic URLs in JavaScript files.
What Is the Purpose of the 'mage/url' Module?
The 'mage/url'
module helps build store URLs dynamically, ensuring accuracy even if the base URL changes.
How Can I Fetch a Custom URL in the Frontend?
Include 'mage/url'
as a dependency and use the build()
method:
define([
'mage/url'
], function (urlBuilder) {
var loginUrl = urlBuilder.build('customer/account/login');
console.log(loginUrl);
});
How Do I Retrieve URLs in the Magento 2 Admin Panel?
Magento 2 does not provide 'mage/url'
directly in the admin panel. You need to define the base URL in a template file and use JavaScript to retrieve it.
Can I Build Custom URLs in the Magento 2 Admin Panel?
Yes, once the base URL is set, you can use urlBuilder.build()
to generate admin URLs dynamically.
Why Should I Use 'mage/url' Instead of Hardcoding URLs?
Hardcoded URLs can break if the store’s base URL changes. The 'mage/url'
module ensures URLs are always accurate.
Where Can I Learn More About URL Handling in Magento 2?
Visit Magento 2’s official documentation for more details on managing URLs dynamically in JavaScript files.