Accessing Admin Quote Sessions in Magento 2
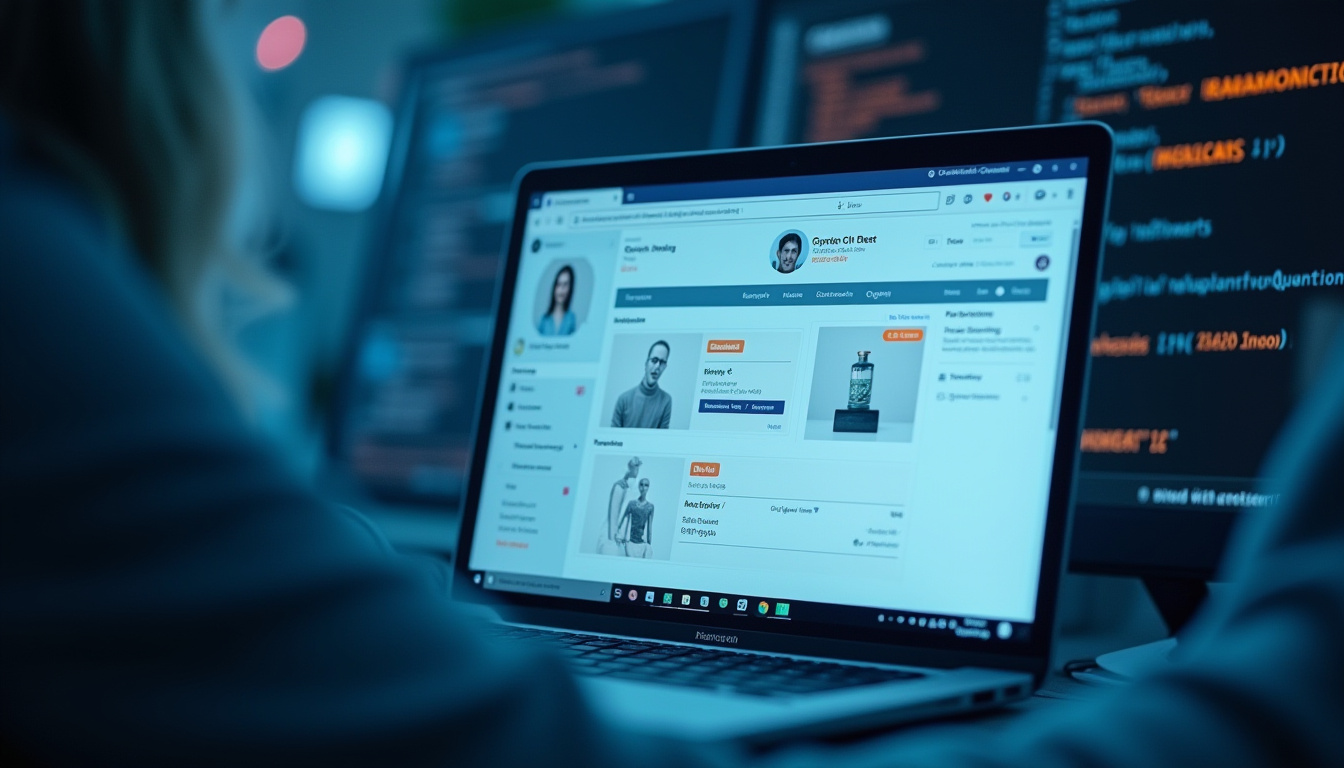
Accessing Admin Quote Sessions in Magento 2
Magento 2, the admin quote session is crucial for managing orders within the backend. The Magento\Backend\Model\Session\Quote class allows developers to retrieve and manipulate quote data when creating orders in the admin panel.
Table Of Content
Accessing Admin Quote Sessions in Magento 2
In Magento 2, managing backend quote sessions is essential for handling orders through the admin panel. To retrieve the current admin quote, utilize the Magento\Backend\Model\Session\Quote
class. This class provides methods to access and manipulate the quote associated with admin order creation.
Implementing the Admin Quote Session
Here's a concise example demonstrating how to set and get the admin session quote programmatically:
<?php
namespace Vendor\Module\Helper;
use Magento\Framework\App\Helper\AbstractHelper;
use Magento\Backend\Model\Session\Quote as AdminQuoteSession;
use Magento\Quote\Model\Quote;
class Data extends AbstractHelper
{
/**
* @var AdminQuoteSession
*/
private $adminQuoteSession;
public function __construct(AdminQuoteSession $adminQuoteSession)
{
$this->adminQuoteSession = $adminQuoteSession;
}
/**
* Set the admin quote session with customer and quote IDs.
*
* @param int $customerId
* @param int $quoteId
* @param int $storeId
* @return void
*/
public function setAdminQuoteSession(int $customerId, int $quoteId, int $storeId): void
{
$this->adminQuoteSession->setCustomerId($customerId);
$this->adminQuoteSession->setQuoteId($quoteId);
$this->adminQuoteSession->setStoreId($storeId);
}
/**
* Retrieve the current admin quote.
*
* @return Quote
*/
public function getAdminQuote(): Quote
{
return $this->adminQuoteSession->getQuote();
}
}
Key Methods for Admin Quote Sessions
The Magento\Backend\Model\Session\Quote
class offers several methods to manage the admin quote session effectively:
Method | Description |
---|---|
setCustomerId($id) |
Assigns a customer ID to the current quote session. |
setQuoteId($id) |
Sets the quote ID for the session. |
setStoreId($id) |
Defines the store ID associated with the quote. |
getQuote() |
Retrieves the current quote object. |
By leveraging these methods, developers can efficiently manage backend quote sessions, facilitating smoother order processing within the Magento 2 admin panel.
Additional Considerations
It's important to note that while the frontend utilizes Magento\Checkout\Model\Session
for quote management, the backend relies on Magento\Backend\Model\Session\Quote
. This distinction ensures that admin-specific order processes remain separate from the customer-facing frontend sessions.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is an Admin Quote Session in Magento 2?
An admin quote session in Magento 2 manages order data when creating or editing orders from the admin panel.
Which Class Handles Admin Quote Sessions in Magento 2?
Magento 2 uses the Magento\Backend\Model\Session\Quote
class to manage admin quote sessions.
How Do I Retrieve the Current Admin Quote?
You can get the current admin quote using:
$quote = $this->adminQuoteSession->getQuote();
What Information Can I Retrieve From an Admin Quote?
You can access quote items, quote ID, customer details, store ID, and extension attributes.
How Do I Set an Admin Quote Session in Magento 2?
You can set the quote session using:
$this->adminQuoteSession->setCustomerId($customerId);
$this->adminQuoteSession->setQuoteId($quoteId);
$this->adminQuoteSession->setStoreId($storeId);
What Is the Difference Between Frontend and Admin Quote Sessions?
The frontend uses Magento\Checkout\Model\Session
, while the admin panel uses Magento\Backend\Model\Session\Quote
to keep order processes separate.
Why Is Managing Admin Quote Sessions Important?
Proper management ensures smooth order processing, accurate customer data handling, and a better admin user experience.
Where Can I Learn More About Admin Quote Sessions?
Refer to Magento 2’s official documentation for in-depth details on managing admin quote sessions effectively.