Leveraging the sales_quote_address_collect_totals_before Event in Magento 2
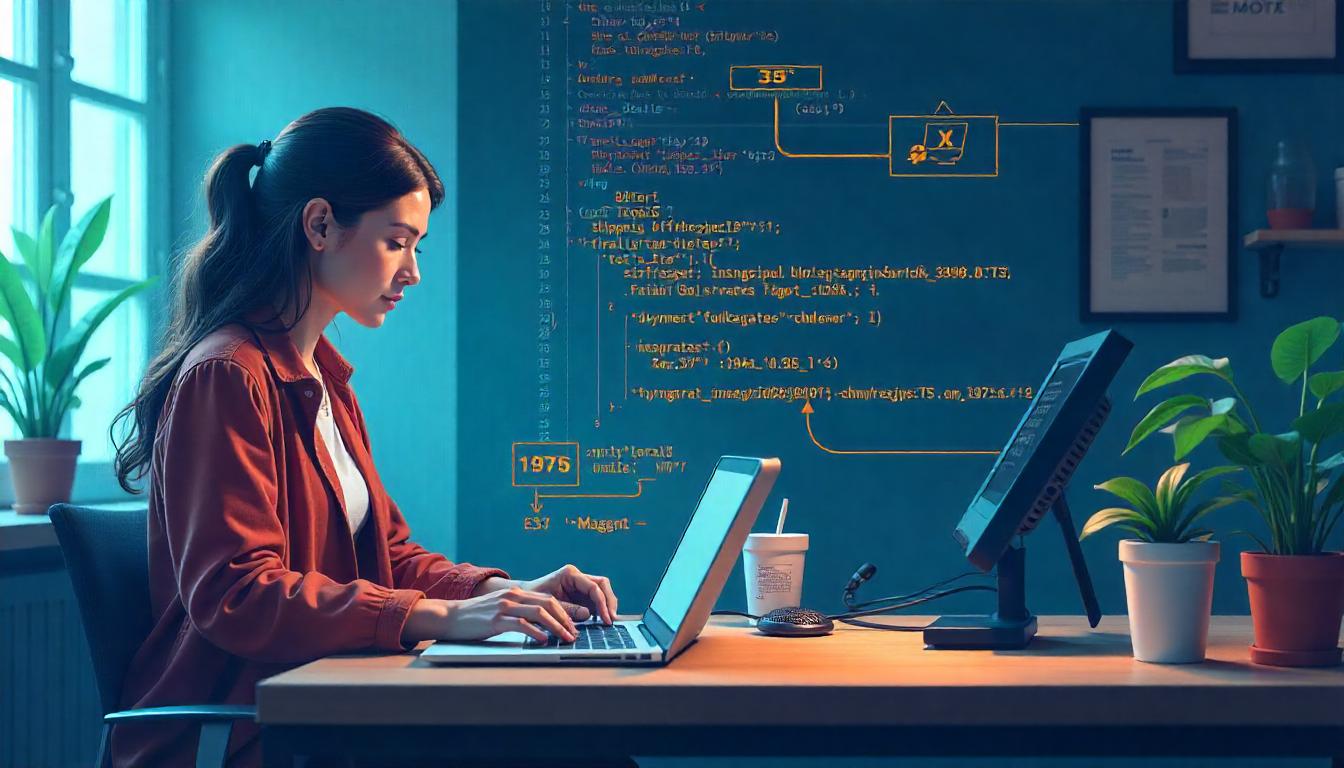
Leveraging the sales_quote_address_collect_totals_before Event in Magento 2
The sales_quote_address_collect_totals_before event in Magento 2 allows you to modify quote totals before Magento finalizes them. This event is useful for adding custom fees, discounts, or other adjustments based on shipping details or customer data.
Leveraging the sales_quote_address_collect_totals_before Event in Magento 2
In Magento 2, the sales_quote_address_collect_totals_before
event lets you adjust custom totals before Magento calculates the final totals for a quote's address. This event is defined in the Magento_Quote/Model/Quote/TotalsCollector.php
file.
Implementing the sales_quote_address_collect_totals_before
Event
To use this event, you'll need to create an observer that listens for it and processes your custom logic. Here's how you can set it up:
Define the Event in events.xml
:
Create or update the events.xml file in your module's etc directory to include the sales_quote_address_collect_totals_before
event.
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:noNamespaceSchemaLocation="urn:magento:framework:Event/etc/events.xsd">
<event name="sales_quote_address_collect_totals_before">
<observer name="custom_totals_before_observer" instance="Vendor\Module\Observer\CustomTotalsBefore" />
</event>
</config>
Create the Observer Class:
Develop the observer class that contains the logic to modify the quote's totals before they're finalized.
<?php
namespace Vendor\Module\Observer;
use Magento\Framework\Event\Observer;
use Magento\Framework\Event\ObserverInterface;
use Magento\Quote\Model\Quote;
class CustomTotalsBefore implements ObserverInterface
{
public function execute(Observer $observer)
{
// Retrieve the quote from the event observer
/** @var Quote $quote */
$quote = $observer->getEvent()->getQuote();
// Access the shipping assignment
$shippingAssignment = $observer->getEvent()->getShippingAssignment();
$address = $shippingAssignment->getShipping()->getAddress();
// Access the totals
$total = $observer->getEvent()->getTotal();
// Implement your custom logic here
// For example, add a custom fee
$customFee = 10; // Define your custom fee amount
$total->setTotalAmount('custom_fee', $customFee);
$total->setBaseTotalAmount('custom_fee', $customFee);
// Add the custom fee to the grand total
$total->setGrandTotal($total->getGrandTotal() + $customFee);
$total->setBaseGrandTotal($total->getBaseGrandTotal() + $customFee);
}
}
Key Points to Consider:
- Event Timing: The
sales_quote_address_collect_totals_before
event is triggered before Magento calculates the final totals. This timing allows you to introduce or modify data that will influence the total calculation process. - Accessing Quote Data: Within the observer, you can access the quote, shipping assignment, and totals data. This access enables you to make precise adjustments based on the current state of the quote.
- Custom Logic Implementation: You can introduce custom fees, discounts, or other adjustments as needed. Ensure that any additions are appropriately reflected in the grand total to maintain consistency.
By leveraging the sales_quote_address_collect_totals_before
event, you can effectively customize the calculation of totals in Magento 2, tailoring it to meet specific business requirements.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is the sales_quote_address_collect_totals_before
Event in Magento 2?
The sales_quote_address_collect_totals_before
event in Magento 2 allows developers to modify quote totals before Magento finalizes them during the checkout process.
Where Is the sales_quote_address_collect_totals_before
Event Defined?
This event is defined in the Magento_Quote/Model/Quote/TotalsCollector.php
file, making it an essential part of the quote calculation process.
How Can I Implement the sales_quote_address_collect_totals_before
Event?
To implement this event, create an observer and register it in your module’s events.xml
file to process custom logic before the final quote total is calculated.
What Are the Key Elements of an Observer for This Event?
The observer should retrieve the quote, access shipping assignments and totals, and apply any custom logic to modify the total before finalization.
Can I Add Custom Fees Using This Event?
Yes. You can add custom fees by modifying the totals object within the observer and ensuring the fee is reflected in the grand total.
How Does This Event Affect Magento's Checkout Process?
Since this event runs before totals are finalized, any changes made will directly affect the checkout summary and final order calculation.
Where Are Quote Totals Stored in Magento 2?
Quote totals are stored in the sales_quote
and sales_quote_address
tables in the Magento database.
Where Can I Learn More About Customizing Quote Totals?
Refer to Magento’s official developer documentation for in-depth guides on modifying quote and order totals using events and observers.