Converting Array Data to Serialized Format in Magento 2
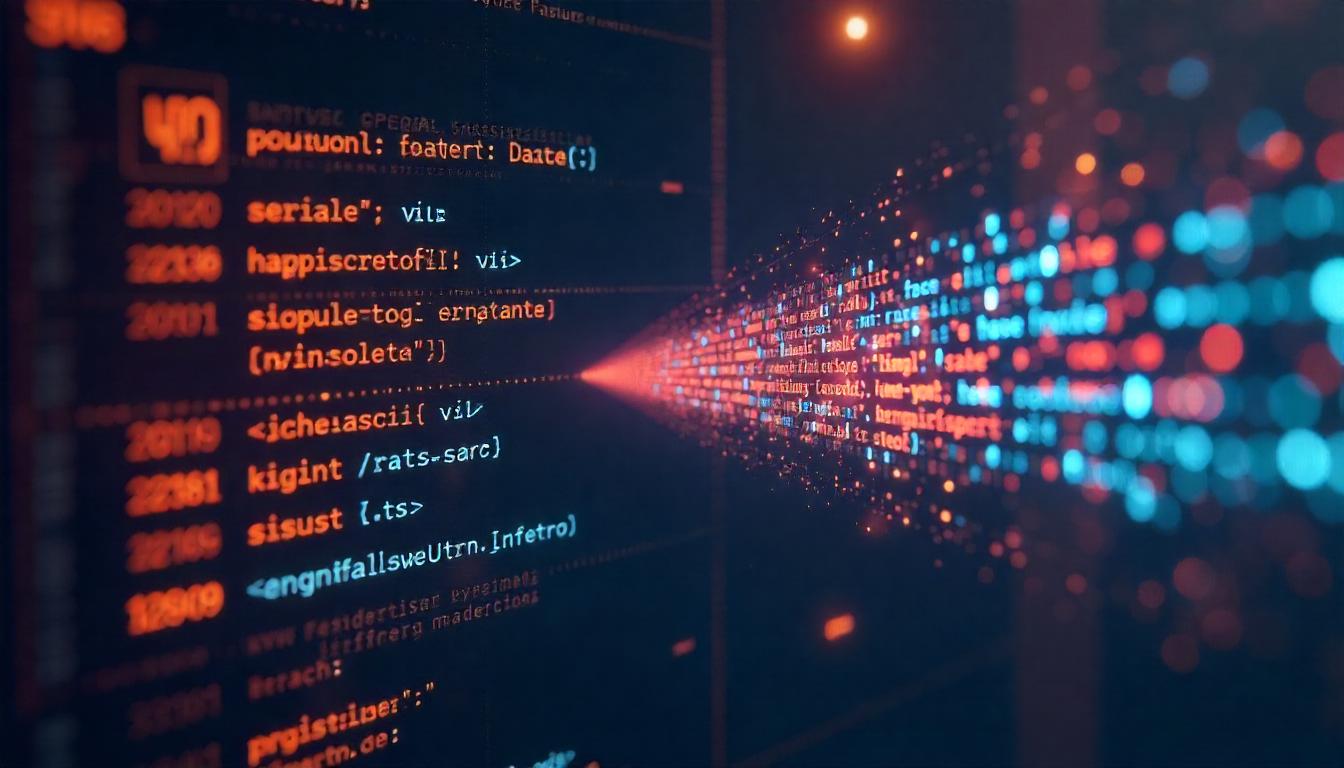
Converting Array Data to Serialized Format in Magento 2
Serialization in Magento 2 allows you to store and transmit array data without losing its structure or data types. Using SerializerInterface, you can efficiently convert arrays into a serialized format, making it easier to save complex data in databases or transfer it between system components.
Table Of Content
Converting Array Data to Serialized Format in Magento 2
In Magento 2, you can serialize array data using the built-in SerializerInterface
class. Serialization allows you to store or transmit PHP values without losing their type or structure.
Implementing Serialization in Magento 2
To serialize data,utilize theMagento\Framework\Serialize\SerializerInterface
interface. Here's how you can implement it:
<?php
namespace YourNamespace\YourModule\Model;
use Magento\Framework\Serialize\SerializerInterface;
class DataSerializer
{
/**
* @var SerializerInterface
*/
private $serializer;
/**
* Constructor
*
* @param SerializerInterface $serializer
*/
public function __construct(SerializerInterface $serializer)
{
$this->serializer = $serializer;
}
/**
* Serialize Data
*
* @return string
*/
public function serializeData()
{
$data = ['key' => 'value'];
return $this->serializer->serialize($data);
}
}
Usage Example
To serialize data, you can call the serializeData
method from a template or another class:
echo $this->serializeData();
Output
{"key":"value"}
Key Points
- Serialization Purpose: Serialization converts PHP data into a storable or transmittable format without losing its type or structure.
- SerializerInterface: Magento 2 provides the
SerializerInterface
for serialization tasks. - Data Types: You can serialize various data types, including strings, arrays, and integers.
Common Use Cases
Serialization is useful when you need to:
- Store complex data structures in a database.
- Transmit data between different system components.
- Maintain data integrity during storage or transmission.
Error Handling
While serializing data, ensure that the data is serializable and handle any exceptions that may arise during the process.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is Data Serialization in Magento 2?
Data serialization in Magento 2 converts PHP data structures into a storable or transmittable format while preserving their type and structure.
Which Class Handles Serialization in Magento 2?
Magento 2 uses the Magento\Framework\Serialize\SerializerInterface
class for data serialization.
How Do I Serialize an Array in Magento 2?
You can serialize an array using SerializerInterface
as follows:
$data = ['key' => 'value'];
$serializedData = $this->serializer->serialize($data);
Where Is Serialized Data Used in Magento 2?
Serialized data is commonly used in database storage, caching, API responses, and configuration settings.
What Are the Benefits of Using SerializerInterface
in Magento 2?
It ensures data consistency, improves performance, and provides a standardized way to handle serialization in Magento 2.
Can I Serialize Data Types Other Than Arrays?
Yes, you can serialize strings, integers, objects, and arrays using SerializerInterface
.
How Do I Deserialize Data in Magento 2?
You can deserialize data using:
$originalData = $this->serializer->unserialize($serializedData);
Where Can I Learn More About Magento 2 Serialization?
Check Magento’s official developer documentation for more details on handling serialization efficiently.