How to Retrieve Masked ID from the Cart ID in Magento 2
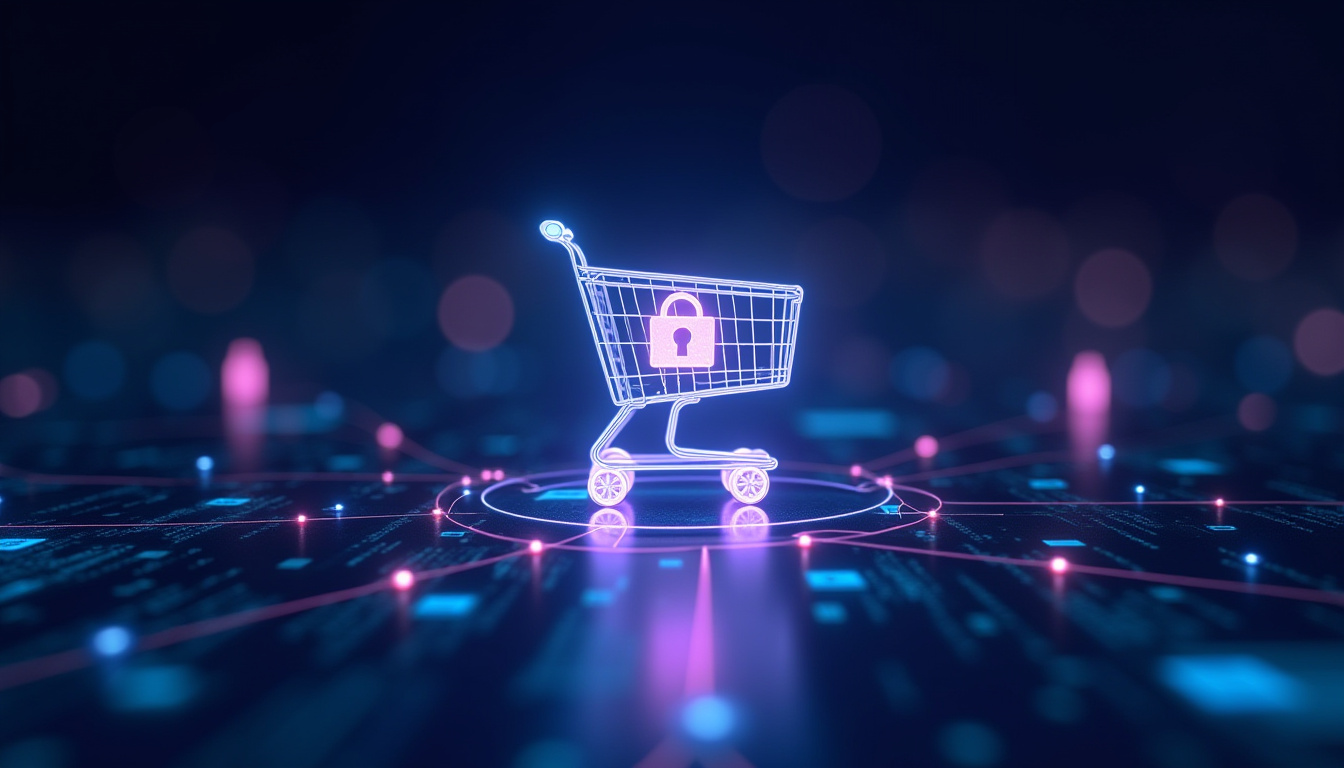
How to Retrieve Masked ID from the Cart ID in Magento 2
In Magento 2, a masked ID (guest cart hash) is a randomly generated string stored in the quote_id_mask
table. It is associated with guest customer carts, ensuring secure cart identification. Retrieving the masked ID from a cart ID is crucial when handling guest checkouts.
Table Of Content
Understanding Masked ID in Magento 2
Magento 2 introduces Masked IDs to enhance security and prevent the direct exposure of internal quote IDs for guest carts. These masked IDs ensure that guest users can continue their shopping sessions securely without revealing sensitive cart information.
What is a Masked ID?
A Masked ID is a unique identifier assigned to a guest user's shopping cart. Instead of using the quote_id directly, Magento generates a masked version that is used in API calls, checkout processes, and cart-related operations.
Why is it Important?
- Security – Prevents direct access to internal cart IDs.
- Data Privacy – Ensures guest users' carts remain protected.
- Session Continuity – Allows users to retrieve their carts across different sessions or devices.
Where is the Masked ID Stored?
Magento maintains the masked ID within the quote_id_mask table, which acts as a bridge between the internal quote_id and the externally exposed masked_id.
Database Tables Involved
Table Name | Description |
---|---|
quote_id_mask | Stores guest cart masked IDs and their associated quote_id. |
quote | Contains cart details, including items, totals, and customer session data. |
How is a Masked ID Generated?
Magento generates a masked ID when a guest user adds an item to the cart. The process follows these steps:
- A guest user adds a product to the cart.
- Magento creates a quote in the
quote
table. - A masked_id is generated and mapped to the
quote_id
in thequote_id_mask
table. - This masked ID is used in API requests, checkout, and guest cart retrieval.
Example Query to Retrieve a Masked ID
To fetch a masked ID for a given quote ID, run:
SELECT masked_id
FROM quote_id_mask
WHERE quote_id = <quote_id>;
To find the corresponding quote ID from a masked ID:
SELECT quote_id
FROM quote_id_mask
WHERE masked_id = '<masked_id>';
Masked ID in Magento API
Magento provides REST and GraphQL APIs that allow developers to retrieve and interact with masked IDs.
Retrieve Guest Cart Masked ID (REST API)
Endpoint:
POST /V1/guest-carts
Response:
{
"masked_id": "c1a2b3c4d5e6f7g8h9i0j"
}
Retrieve Guest Cart Masked ID (GraphQL API)
mutation {
createEmptyCart
}
Response:
{
"data": {
"createEmptyCart": "c1a2b3c4d5e6f7g8h9i0j"
}
}
Common Issues and Solutions
Issue | Cause | Solution |
---|---|---|
Guest Cart Not Retained | Masked ID not mapped correctly to quote_id. | Ensure quote_id_mask contains the correct mapping. |
Masked ID Expired | Session expired or quote cleared. | Extend guest cart lifetime in Magento settings. |
Cart Not Found via API | Masked ID does not exist. | Verify the masked ID in the quote_id_mask table. |
Pro Tips for Working with Masked IDs
- Always Use Masked IDs in API Calls – Never expose internal
quote_id
. - Monitor Expiry Time – Configure guest cart duration in
Stores → Configuration → Sales → Checkout
. - Test API Responses – Validate masked ID retrieval and mapping in your API logs.
By understanding and utilizing masked IDs effectively, Magento developers can ensure a secure and seamless guest checkout experience
How to Retrieve the Masked ID from Cart ID in Magento 2
Magento 2 uses masked IDs for guest carts to improve security and prevent exposing internal quote IDs. Retrieving a masked ID is crucial for handling guest carts in APIs, custom modules, and third-party integrations.
Using QuoteIdToMaskedQuoteIdInterface
Magento provides an interface to retrieve the masked ID for a given quote ID.
Implementation in a Custom Class
<?php
namespace Vendor\Module\Model;
use Magento\Framework\Exception\NoSuchEntityException;
use Magento\Framework\Exception\LocalizedException;
use Magento\Quote\Model\QuoteIdToMaskedQuoteIdInterface;
class MaskedHash
{
/**
* @var QuoteIdToMaskedQuoteIdInterface
*/
private $quoteIdToMaskedQuoteId;
public function __construct(
QuoteIdToMaskedQuoteIdInterface $quoteIdToMaskedQuoteId
) {
$this->quoteIdToMaskedQuoteId = $quoteIdToMaskedQuoteId;
}
/**
* Retrieve Masked ID by Quote ID
*
* @param int $quoteId
* @return string|null
* @throws LocalizedException
*/
public function getQuoteMaskId($quoteId)
{
try {
return $this->quoteIdToMaskedQuoteId->execute($quoteId);
} catch (NoSuchEntityException $exception) {
throw new LocalizedException(__('Current user does not have an active cart.'));
}
}
}
How to Call the Method
To fetch the masked ID for a specific quote ID, use the following:
$quoteId = 1;
echo $maskedId = $this->getQuoteMaskId($quoteId);
Expected Output
Scenario | Expected Output |
---|---|
If a masked ID exists for the cart | Returns a hashed masked ID (e.g., a1b2c3d4e5f6 ) |
If no masked ID exists for the cart | Error: Current user does not have an active cart. |
Troubleshooting Common Issues
Issue | Cause | Solution |
---|---|---|
Guest Cart Not Retained | Masked ID not mapped correctly to quote_id . |
Ensure quote_id_mask contains the correct mapping. |
Masked ID Expired | Guest session expired or cart was cleared. | Extend guest cart lifetime in Magento settings. |
Cart Not Found via API | Masked ID does not exist or is invalid. | Verify the masked ID in the quote_id_mask table. |
Masked ID Returns Empty Value | Quote ID is invalid or does not exist. | Check quote_id in quote and quote_id_mask tables. |
Guest Cart Items Lost | Cart session expired due to inactivity. | Increase session timeout under Stores → Configuration . |
How to Extend Guest Cart Lifetime
If guest carts expire too quickly, you can extend the session timeout:
Step 1: Update Magento Admin Settings
- Go to Stores → Configuration → Customers → Persistent Shopping Cart.
- Set Enable Persistence to Yes.
- Increase the Persistence Lifetime (Seconds) value (e.g.,
86400
for 24 hours). - Save the changes and clear the cache:
php bin/magento cache:flush
Step 2: Modify session.gc_maxlifetime
in PHP
Edit php.ini
to extend session lifetime:
session.gc_maxlifetime = 86400
Restart the server for changes to take effect.
Best Practices for Handling Masked IDs
1. Always Verify API Credentials
Ensure that you pass the correct masked ID when making API calls to retrieve guest carts.
2. Check Database Entries
Manually verify the quote_id_mask
table to confirm if a masked ID exists for the given quote ID.
3. Use Logging for Debugging
If masked IDs are not being retrieved correctly, implement logging:
$logger->info('Masked ID for Quote: ' . $maskedId);
4. Handle Exceptions Gracefully
Use try-catch blocks to handle errors and prevent crashes:
try {
$maskedId = $this->getQuoteMaskId($quoteId);
} catch (LocalizedException $e) {
$logger->error($e->getMessage());
}
5. Extend Guest Cart Expiry
Modify Magento settings and server configurations to extend the guest cart lifetime.
Key Takeaways
- Masked IDs protect guest cart information.
- Stored in
quote_id_mask
table, mapped toquote_id
. - Use
QuoteIdToMaskedQuoteIdInterface
to retrieve masked IDs securely. - Verify data in the database if guest carts are not working as expected.
- Extend session lifetime to prevent guest cart expiration.
Alternative Ways to Retrieve Masked ID in Magento 2
In addition to using QuoteIdToMaskedQuoteIdInterface
, you can retrieve the masked ID using database queries, REST APIs, and GraphQL.
1. Using Database Query (Direct SQL)
If you need to manually fetch the masked ID, execute the following SQL query in your Magento database:
SELECT masked_id FROM quote_id_mask WHERE quote_id = 1;
Replace 1
with the actual quote_id
of the cart you need to retrieve.
Additional Considerations:
- Ensure that the
quote_id
exists in thequote
table before querying thequote_id_mask
table. - If no result is returned, the guest cart may have expired or was never assigned a masked ID.
- Direct database queries should be used cautiously in production environments, as they bypass Magento’s built-in caching and security mechanisms.
2. Using REST API
Magento 2 provides a REST API to retrieve the masked cart ID, allowing seamless integration with third-party applications.
API Endpoint:
GET /V1/guest-carts/
Example Response:
{
"masked_id": "abcd1234efgh5678"
}
Steps to Retrieve Masked ID via REST API:
- Ensure your Magento store has API access enabled under Stores → Configuration → Services → Magento Web API.
- Send an authenticated GET request to
/V1/guest-carts/
. - The response will contain the guest cart’s
masked_id
.
Use Cases:
- When integrating Magento 2 with an external frontend, such as React or Vue.js, to retrieve guest cart information.
- When debugging API-based checkout flows.
3. Using GraphQL API
For Magento 2 stores with GraphQL support, you can retrieve the guest cart masked ID using GraphQL.
GraphQL Query:
query {
createEmptyCart
}
Example Response:
{
"data": {
"createEmptyCart": "abcd1234efgh5678"
}
}
Why Use GraphQL?
- Reduces the need for multiple API requests by retrieving only the required data.
- Ideal for headless Magento implementations.
4. Retrieving Masked ID via Magento CLI
Magento 2’s CLI provides powerful tools to manage carts and orders. You can retrieve the guest cart masked ID by running a custom command.
Create a Custom CLI Command
- Implement a CLI command in a custom module that fetches the masked_id from the database.
Example Implementation:
namespace Vendor\Module\Console\Command;
use Magento\Framework\App\State;
use Magento\Framework\Console\Cli;
use Magento\Quote\Model\QuoteIdToMaskedQuoteIdInterface;
use Symfony\Component\Console\Command\Command;
use Symfony\Component\Console\Input\InputArgument;
use Symfony\Component\Console\Input\InputInterface;
use Symfony\Component\Console\Output\OutputInterface;
class GetMaskedIdCommand extends Command {
private $quoteIdToMaskedQuoteId;
private $state;
public function __construct(
QuoteIdToMaskedQuoteIdInterface $quoteIdToMaskedQuoteId,
State $state
) {
$this->quoteIdToMaskedQuoteId = $quoteIdToMaskedQuoteId;
$this->state = $state;
parent::__construct();
}
protected function configure() {
$this->setName('cart:get-masked-id')
->setDescription('Retrieve the masked ID for a given quote ID')
->addArgument('quote_id', InputArgument::REQUIRED, 'Quote ID');
}
protected function execute(InputInterface $input, OutputInterface $output) {
try {
$this->state->setAreaCode('adminhtml');
$quoteId = $input->getArgument('quote_id');
$maskedId = $this->quoteIdToMaskedQuoteId->execute($quoteId);
$output->writeln('Masked ID: ' . $maskedId);
return Cli::RETURN_SUCCESS;
} catch (\Exception $e) {
$output->writeln("<error>" . $e->getMessage() . "</error>");
return Cli::RETURN_FAILURE;
}
}
}
Run the Command:
php bin/magento cart:get-masked-id 1
Expected Output:
Masked ID: abcd1234efgh5678
5. Using Magento Repository Method
Magento’s built-in repository pattern provides an alternative to fetching the masked ID via SQL queries.
Implementation:
use Magento\Quote\Model\QuoteIdToMaskedQuoteIdInterface;
use Magento\Framework\Exception\NoSuchEntityException;
class MaskedIdRetriever {
private $quoteIdToMaskedQuoteId;
public function __construct(QuoteIdToMaskedQuoteIdInterface $quoteIdToMaskedQuoteId) {
$this->quoteIdToMaskedQuoteId = $quoteIdToMaskedQuoteId;
}
public function getMaskedIdByQuoteId($quoteId) {
try {
return $this->quoteIdToMaskedQuoteId->execute($quoteId);
} catch (NoSuchEntityException $e) {
return null;
}
}
}
Usage:
$maskedId = $this->getMaskedIdByQuoteId(1);
echo $maskedId ?: 'Masked ID not found';
Best Practices for Handling Masked IDs in Magento 2
Security Considerations
- Do not expose raw
quote_id
values in public APIs; always use masked IDs. - Ensure guest cart data is cleared periodically to prevent clutter in the
quote_id_mask
table. - Use Magento’s built-in interfaces instead of raw SQL queries whenever possible.
Performance Optimization
- Regularly clean up expired guest cart entries to optimize database performance:
- Enable Magento caching to reduce API lookup times.
php bin/magento cron:run
Debugging Tips
- If the masked ID is missing, check whether the cart exists using:
- If API calls fail, ensure that the Magento Web API module is enabled and configured correctly.
SELECT * FROM quote WHERE entity_id = 1;
Common Issues & Solutions in Retrieving Masked ID in Magento 2
When working with guest carts and masked IDs in Magento 2, you may encounter various issues. Below is a detailed breakdown of common problems, their causes, and step-by-step solutions.
1. NoSuchEntityException
Cause:
- The requested cart ID does not exist in the
quote
table. - The provided cart ID belongs to a logged-in user, not a guest user.
- The quote has been deleted or expired due to session timeout or cleanup.
Solution:
- Verify if the cart ID exists using SQL:
- Check if the cart belongs to a guest user:
- If
customer_id
isNULL
, it’s a guest cart. - If
customer_id
has a value, it’s assigned to a logged-in user. - Recreate the guest cart if missing using the API:
- Check logs for additional errors:
SELECT * FROM quote WHERE entity_id = 1;
Replace 1 with the actual quote ID.
SELECT is_active, customer_id FROM quote WHERE entity_id = 1;
POST /V1/guest-carts/
Response:
{
"masked_id": "abcd1234efgh5678"
}
tail -f var/log/exception.log
2. Masked ID is Null
Cause:
- The user is logged in, and masked IDs only apply to guest carts.
- The cart session has expired and is no longer associated with a masked ID.
- The
quote_id_mask
table does not contain an entry for thequote_id
.
Solution:
- Confirm the cart belongs to a guest user:
- Manually fetch the masked ID:
- Regenerate a masked ID if missing:
- Ensure Magento is correctly handling guest sessions by adjusting the session timeout in:
- Stores → Configuration → Advanced → Admin → Security → Session Lifetime (Seconds)
- Check for custom overrides in
quote_id_mask
by running:
SELECT customer_id FROM quote WHERE entity_id = 1;
If customer_id
is not NULL, it’s a registered user’s cart, so a masked ID is not applicable.
SELECT masked_id FROM quote_id_mask WHERE quote_id = 1;
If masked_id is missing, you can programmatically generate it using:
use Magento\Quote\Model\QuoteIdToMaskedQuoteIdInterface;
$maskedId = $this->quoteIdToMaskedQuoteId->execute($quoteId);
SELECT * FROM quote_id_mask WHERE quote_id = 1;
3. QuoteIdToMaskedQuoteIdInterface Not Found
Cause:
- The dependency is not injected properly in the constructor.
- The di.xml file is missing a preference for
QuoteIdToMaskedQuoteIdInterface
. - The class is not declared in the correct scope (e.g., missing
use
statement).
Solution:
- Ensure proper dependency injection in your class constructor:
- Verify
di.xml
contains the correct preference: - Regenerate Magento's dependency injection cache:
- Check if another module is overriding the interface:
- Ensure the interface is properly imported in your PHP class:
use Magento\Quote\Model\QuoteIdToMaskedQuoteIdInterface;
class ExampleClass {
private $quoteIdToMaskedQuoteId;
public function __construct(QuoteIdToMaskedQuoteIdInterface $quoteIdToMaskedQuoteId) {
$this->quoteIdToMaskedQuoteId = $quoteIdToMaskedQuoteId;
}
}
File: app/code/Vendor/Module/etc/di.xml
<preference for="Magento\Quote\Model\QuoteIdToMaskedQuoteIdInterface"
type="Magento\Quote\Model\QuoteIdToMaskedQuoteId" />
php bin/magento setup:di:compile
php bin/magento cache:flush
grep -r "QuoteIdToMaskedQuoteIdInterface" app/code
use Magento\Quote\Model\QuoteIdToMaskedQuoteIdInterface;
Pro Tips
- Always validate the cart ID before querying the masked ID.
- Use dependency injection instead of object manager for better performance.
- Debug using logs by adding
$this->logger->info($maskedId);
inside your method. - For logged-in users, retrieve cart details using
\Magento\Quote\Model\QuoteRepository
instead.
Magento 2 guest cart management can be complex, but with proper debugging techniques, database queries, and API usage, you can efficiently retrieve and manage masked IDs.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Retrieving the masked ID from the cart ID in Magento 2 is a fundamental step in managing guest checkouts, improving API-based integrations, and ensuring seamless customer experiences. Since Magento 2 uses a quote_id_mask table to store masked quote IDs for guest users, leveraging the QuoteIdToMaskedQuoteIdInterface allows developers to fetch these values efficiently.
By implementing this approach, you can enhance your store’s functionality by securely identifying guest carts, resolving checkout-related issues, and integrating third-party systems that require cart data. Moreover, with Magento 2.4.7 (2025), improvements in performance, security, and stability make handling guest sessions and masked cart IDs more efficient than ever.
To maintain a high-performing eCommerce store, always follow best practices:
- Ensure proper exception handling to avoid errors when retrieving masked IDs.
- Regularly update Magento to leverage the latest improvements and security fixes.
- Use logging mechanisms to track masked quote IDs for debugging purposes.
- Optimize guest checkout workflows to minimize cart abandonment.
Mastering these techniques will allow you to streamline cart management, improve checkout performance, and enhance the overall shopping experience for customers. By staying up to date with Magento’s evolving ecosystem, your store can remain competitive and future-proof.
FAQs
What is a masked ID in Magento 2?
A masked ID is a randomly generated string stored in the quote_id_mask
table, representing the guest cart ID in Magento 2.
Why do I need to retrieve a masked ID from the cart ID?
Retrieving a masked ID allows you to manage guest carts securely, enabling seamless checkout processes and API integrations.
Which interface is used to retrieve the masked ID in Magento 2?
The Magento\Quote\Model\QuoteIdToMaskedQuoteIdInterface
is used to fetch the masked ID from the cart ID.
How can I retrieve a masked ID using PHP code?
You can use the execute($quoteId)
method of QuoteIdToMaskedQuoteIdInterface
to get the masked ID for a given quote ID.
What happens if there is no masked ID for the cart?
If no masked ID exists for the given cart, an error is thrown: "Current user does not have an active cart."
How does Magento 2 store guest cart information?
Magento 2 stores guest cart data in the quote_id_mask
table, linking a masked ID to an actual quote ID.
Can I retrieve the masked ID for a logged-in customer?
No, masked IDs are generated only for guest carts. Logged-in customers use their regular quote IDs.
Where is the masked quote ID stored in the database?
The masked quote ID is stored in the quote_id_mask
table, mapping guest cart IDs to unique masked values.
How do I retrieve the quote ID from a masked ID?
To get the original quote ID from a masked ID, use the MaskedQuoteIdToQuoteIdInterface
in Magento 2.
Is retrieving masked IDs necessary for API integrations?
Yes, masked IDs are required for API interactions with guest carts, ensuring secure access to cart data.
Can I regenerate a masked ID for an existing cart?
No, Magento 2 does not provide a way to regenerate masked IDs manually. They are created automatically when a guest cart is initiated.
What is the difference between a quote ID and a masked ID?
A quote ID is used for logged-in customers, while a masked ID is assigned to guest carts for security and API accessibility.
How can I handle exceptions when retrieving a masked ID?
Use a try-catch block to catch NoSuchEntityException
or LocalizedException
to handle missing masked IDs gracefully.
Does Magento 2.4.7 improve handling of masked IDs?
Yes, Magento 2.4.7 enhances performance and security when handling guest carts and masked quote IDs.
How can I debug issues with masked quote IDs?
You can check the quote_id_mask
table, enable logging, and use debugging tools like Xdebug to troubleshoot masked ID retrieval.