Adding Custom Fields to the Magento 2 Checkout Page: A Step-by-Step Guide
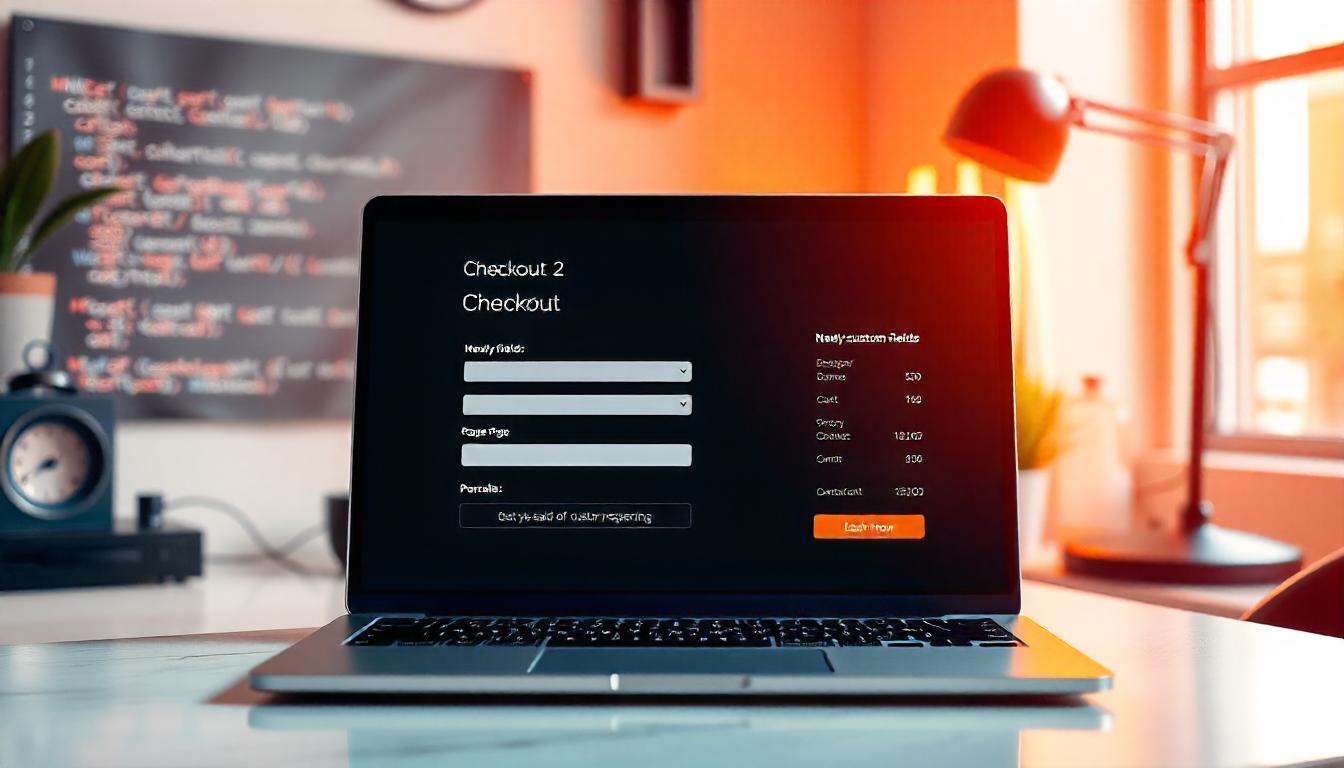
Adding Custom Fields to the Magento 2 Checkout Page: A Step-by-Step Guide
Customizing the checkout page in Magento 2 allows you to improve user experience and gather additional data. This guide will help you programmatically add custom fields to the checkout process while following best practices.
Table Of Content
Why Customize Magento 2 Checkout Fields?
Customizing checkout fields in Magento 2 goes beyond reducing cart abandonment. It helps gather valuable data, improve order fulfillment, and refine marketing strategies. Let’s break this down:
Collect Additional Customer Insights
Custom fields allow you to ask questions like "How did you hear about us?" The answers provide actionable data. If most responses point to social media, you might need to reconsider other marketing methods like SEO. Acknowledging previous missteps, leveraging this information can lead to more effective campaigns.
Refine Targeted Marketing
Custom fields can also reveal customer preferences, allowing you to tailor campaigns to specific needs. This leads to higher engagement and improved conversion rates. For instance, knowing customer interests can help you promote relevant products or services more effectively.
Enhance Order Fulfillment
Missed deliveries are a common issue. Adding a field to request a preferred delivery time minimizes these risks. This not only cuts down on costs but also boosts customer satisfaction.
Adding Custom Fields to Magento 2 Checkout: A Guide
Enhancing your Magento 2 checkout with custom fields, like a delivery date or special instructions, improves customer experience and supports unique business needs. However, Magento 2 lacks native support for checkout field customization. Here are two primary methods to achieve this:
1. Create a Custom Module (Programmatically)
This approach offers full control and flexibility. Key steps include:
- Extend the Layout: Modify the checkout_index_index.xml file to include your custom field in the checkout layout.
- UI Component Integration: Use Knockout.js to bind the custom field's data with the backend.
- Save Data: Add logic in a custom controller to store the new field's value in the quote or order table.
- Validate Input: Ensure customer input is validated before saving.
This method is ideal for developers familiar with Magento 2's architecture and coding practices. It requires creating or modifying files such as requirejs-config.js, custom JavaScript, and backend controllers for saving data.
2. Use an Extension
Magento extensions simplify the process without requiring custom coding. These pre-built solutions often provide drag-and-drop interfaces for adding fields, configuring their placement, and handling backend storage. While they save time, ensure compatibility with your theme and other extensions.
5. Range Filtering
Filter products with IDs greater than 5:
$collection->addFieldToFilter('entity_id', ['gt' => 5]);
Key Considerations
- Backup First: Before making changes, backup your store to prevent data loss.
- Testing: Thoroughly test the checkout process to ensure the custom field integrates smoothly.
- Error Handling: Use robust error-handling practices to prevent disruptions during checkout.
Both methods have their benefits, depending on your technical expertise and budget. For a more hands-on approach, custom modules are ideal. Extensions, on the other hand, are perfect for rapid deployment without technical complexity.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
How to Add a Custom Field to Magento 2 Checkout Programmatically
Adding a custom field to the checkout page in Magento 2 involves creating a custom module. Follow these straightforward steps:
1. Set Up the Custom Module
Create a folder structure under app/code as follows:
app/code/Vendor/CheckoutField
Register the Module:
Add registration.php inside app/code/Vendor/CheckoutField/:
?php,
\Magento\Framework\Component\ComponentRegistrar::register(,
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Vendor_CheckoutField',
__DIR__,
);,
Define module.xml:
Place module.xml in app/code/Vendor/CheckoutField/etc/:
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Vendor_CheckoutField" setup_version="1.0.0"/>
</config>
2. Activate the Module
Run these commands:
php bin/magento setup:upgrade ,
php bin/magento cache:flush ,
3. Customize the Layout
Create checkout_index_index.xml in app/code/Vendor/CheckoutField/view/frontend/layout/:
<?xml version="1.0"?>
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<body>
<referenceContainer name="checkout.root">
<block name="checkout.field" before="-" template="Vendor_CheckoutField::checkout/field.phtml" />
</referenceContainer>
</body>
</page>
4. Add the Custom Field Template
Create field.phtml in app/code/Vendor/CheckoutField/view/frontend/templates/checkout/:
<div class="field custom-field">
<label>('Custom Field')</label >
<input type="text" name="custom_field" id="custom_field" data-bind="value: customField"/>
</div">
5. Integrate JavaScript
Create custom_field.js in app/code/Vendor/CheckoutField/view/frontend/web/js/view/:
define([,
uiComponent,
'ko',
], function (Component, ko) {,
'use strict';,
return Component.extend({,
defaults: {,
template: 'Vendor_CheckoutField/checkout/field',
},
customField: ko.observable(''),,
initialize: function () {,
this._super();,
},
});,
});,
Configure requirejs-config.js in app/code/Vendor/CheckoutField/view/frontend/:
var config = {,
config: {,
mixins: {
'Magento_Checkout/js/view/shipping': {,
'Vendor_CheckoutField/js/view/custom_field': true,
},
},
},
};,
6. Save the Custom Field Value
Add events.xml in app/code/Vendor/CheckoutField/etc/frontend/:
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework/Event/etc/events.xsd">
<event name="checkout_submit_all_after">
<observer name="vendor_checkoutfield_save_custom_field" instance="Vendor\CheckoutField\Observer\SaveCustomFieldObserver"/>
</event>
</config>
Create the observer SaveCustomFieldObserver.php in app/code/Vendor/CheckoutField/Observer/:
<?php
namespace Vendor\CheckoutField\Observer;
use Magento\Framework\Event\Observer;
use Magento\Framework\Event\ObserverInterface;
class SaveCustomFieldObserver implements ObserverInterface {
public function execute(Observer $observer) {
$order = $observer->getEvent()->getOrder();
$customFieldValue = $observer->getEvent()->getRequest()->getParam('custom_field');
if ($customFieldValue) {
$order->setData('custom_field', $customFieldValue);
} }
}
7. Modify the Database Schema
Create InstallData.php in app/code/Vendor/CheckoutField/Setup/:
<?php
namespace Vendor\CheckoutField\Setup;
use Magento\Framework\Setup\InstallSchemaInterface;
use Magento\Framework\Setup\ModuleContextInterface;
use Magento\Framework\Setup\SchemaSetupInterface;
use Magento\Framework\DB\Ddl\Table;
class InstallSchema implements InstallSchemaInterface
{
public function install(SchemaSetupInterface $setup, ModuleContextInterface $context)
{
$setup->startSetup()
$setup->getConnection()->addColumn(
$setup->getTable('sales_order'),
'custom_field',
[
'type' => Table::TYPE_TEXT,
'length' => 255,
'nullable' => true,
'comment' => 'Custom Field'
]
);
$setup->endSetup();
}
}
Summary Table
Step | Action |
---|---|
Module Creation | Folder, registration.php, and module.xml |
Activation | Run setup:upgrade and cache:flush commands |
Layout File | checkout_index_index.xml setup |
Field Template | Create field.phtml template |
JavaScript Integration | Add custom_field.js and configure requirejs-config.js |
Save Field Value | Add events.xml and SaveCustomFieldObserver.php |
Database Modification | Create InstallData.php to save field to sales_order |
This method ensures seamless integration of a custom field in the checkout process, improving order customization while adhering to Magento's best practices.
FAQs
How Do I Establish a Database Connection in Magento 2?
To execute custom queries in Magento 2, you first need to establish a database connection. Use the following code:
$this->_resources = \Magento\Framework\App\ObjectManager::getInstance() ->get('Magento\Framework\App\ResourceConnection'); $connection = $this->_resources->getConnection();
This creates a connection instance to interact with the database.
How Can I Insert Data Using a Custom Query?
To insert data into a table, use the insert()
method. Here’s an example:
$array = ['column1' => 'value1', 'column2' => 'value2']; $connection->insert($this->_resources->getTableName('test_table'), $array);
This inserts the specified values into the test_table
.
How Do I Retrieve Data Using a Select Query?
Use the select()
method to fetch data from a table:
$select = $connection->select()->from(['o' => $this->_resources->getTableName('test_table')]); $result = $connection->fetchAll($select); foreach ($result as $data) { echo $data['id']; }
This retrieves all records from the table and iterates through them.
How Can I Update Data Using a Custom Query?
To update table data, you can use an SQL query:
$id = 1; $sql = "UPDATE " . $this->_resources->getTableName('test_table') . " SET `title` = 'new title' WHERE `id` = " . $id; $connection->query($sql);
This updates the record with ID 1
in the test_table
.
How Do I Delete Records Using a Custom Query?
To delete records from a table, use the delete()
method with a condition:
$id = 1; $condition = $connection->quoteInto('id = ?', $id); $connection->delete($this->_resources->getTableName('test_table'), $condition);
This deletes the record with the specified ID.
How Can I Join Tables in a Custom Query?
To join tables, use the joinLeft()
method:
$select = $connection->select() ->from(['o' => $this->_resources->getTableName('test_table')]) ->joinLeft( ['t1' => $this->_resources->getTableName('test_table1')], 'o.id = t1.id', ['column1', 'column2'] );
This performs a LEFT JOIN between the specified tables.
What Are the Best Practices for Using Custom Queries?
Follow these best practices to avoid issues when working with custom queries:
- Validate product or table IDs before executing queries.
- Optimize performance by minimizing product loading in loops.
- Leverage Magento's caching mechanisms for frequently accessed data.