Implementing Pagination in Magento 2 Custom Collections
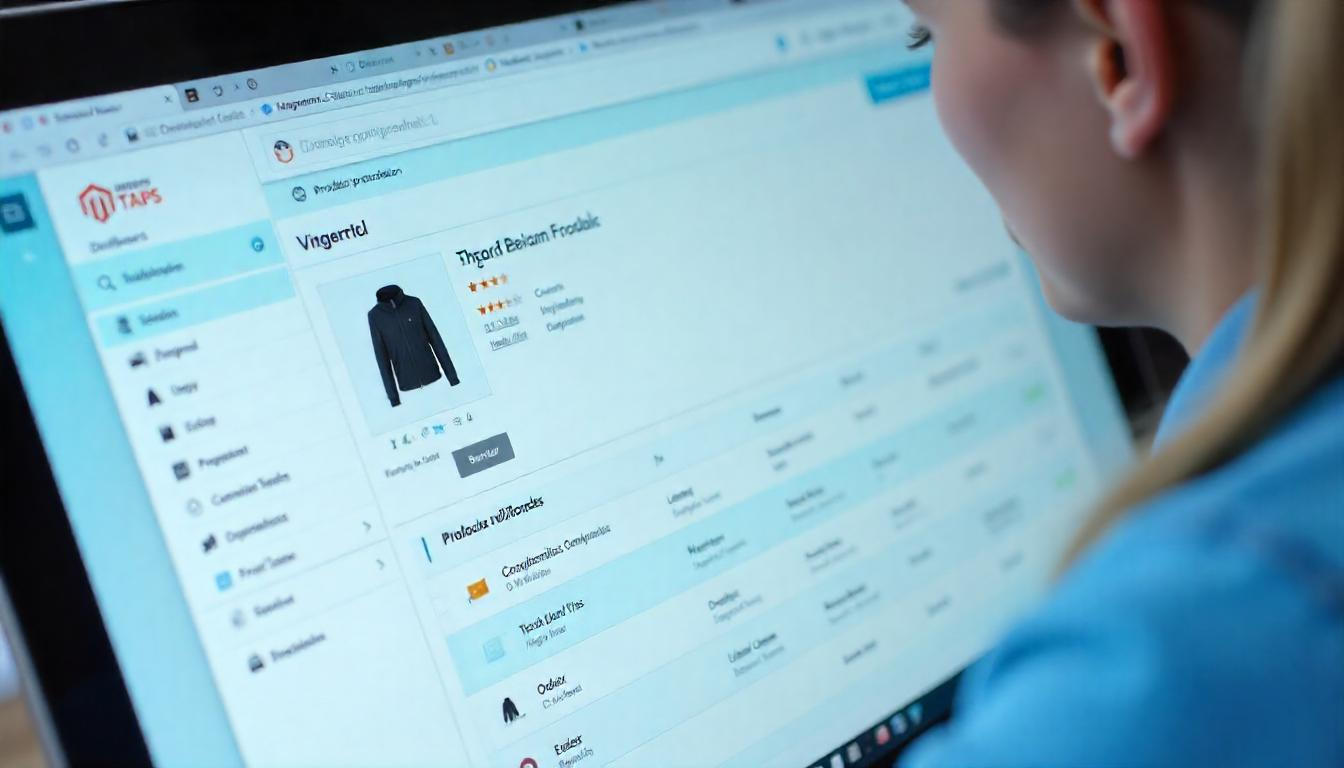
Implementing Pagination in Magento 2 Custom Collections
Learn how to implement pagination in Magento 2 custom collections to improve user experience and site performance. This guide walks you through creating a paginated collection using clear, concise code and best practices. Ideal for custom modules like blogs, image galleries, and product lists where Magento's default pagination isn't available.
Table Of Content
Implementing Pagination in Magento 2 Custom Collections
Magento 2's default collections come with built-in pagination. However, for custom collections—like blogs, image galleries, or custom modules—you need to manually add pagination. Here's a concise guide to achieve this:
Step 1: Create the Custom Collection
Define a method to retrieve your custom collection with pagination parameters:
protected $customCollectionFactory;
public function __construct(
\Vendor\Module\Model\ResourceModel\Custom\CollectionFactory $customCollectionFactory
) {
$this->customCollectionFactory = $customCollectionFactory;
}
public function getCustomCollection()
{
$page = ($this->getRequest()->getParam('p')) ?: 1;
$pageSize = ($this->getRequest()->getParam('limit')) ?: 10;
$collection = $this->customCollectionFactory->create();
$collection->setPageSize($pageSize);
$collection->setCurPage($page);
return $collection;
}
Step 2: Add Pagination to the Layout
In your block class, integrate the pager into the layout:
protected function _prepareLayout()
{
parent::_prepareLayout();
$this->pageConfig->getTitle()->set(__('Custom Collection'));
if ($this->getCustomCollection()) {
$pager = $this->getLayout()->createBlock(
\Magento\Theme\Block\Html\Pager::class,
'custom.collection.pager'
)->setAvailableLimit([5 => 5, 10 => 10, 15 => 15])
->setShowPerPage(true)
->setCollection($this->getCustomCollection());
$this->setChild('pager', $pager);
$this->getCustomCollection()->load();
}
return $this;
}
Step 3: Retrieve Pager HTML in Template
Create a method to fetch the pager HTML:
public function getPagerHtml()
{
return $this->getChildHtml('pager');
}
Step 4: Display Pagination in Template File
In your .phtml file, render the pagination controls:
<?php if ($block->getPagerHtml()): ?>
<div class="pagination-container">
<?= $block->getPagerHtml(); ?>
</div>
<?php endif; ?>
Best Practices for Pagination Limits
Choosing the right number of items per page is crucial:
Content Type | Recommended Items per Page |
---|---|
Images/Galleries | 5–10 |
Text Lists | 10–15 |
Product Listings | 12–20 |
Adjust these values based on your content's nature and user preferences to ensure optimal performance and usability.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What is pagination in Magento 2 custom collections?
Pagination in custom collections allows you to split large data sets into multiple pages, making it easier for users to browse content without long loading times.
Why should I implement pagination in a custom collection?
Implementing pagination improves user experience and performance by preventing large datasets from loading all at once and making navigation more manageable.
How do I set up pagination in a custom block class?
Use the setPageSize()
and setCurPage()
methods on your collection within your block class to control how many items appear per page and which page is displayed.
How do I add pagination controls in the layout?
Create a pager block using \Magento\Theme\Block\Html\Pager
in the _prepareLayout()
method and set it as a child block.
How can I render the pager in the template?
Use $block->getPagerHtml()
in your .phtml
template to render the pagination links for navigation.
Can I control how many items appear per page?
Yes, you can define available limits such as 5, 10, or 15 items per page when creating the pager block using setAvailableLimit()
.
What’s the best page size for custom collections?
It depends on content type. For example, galleries perform well with 5–10 items, while product listings typically show 12–20 items per page.
Do I need to load the collection manually?
Yes, after setting pagination parameters, you should call load()
on the collection before rendering it to ensure data is retrieved correctly.
Does pagination affect performance?
Yes, it improves performance by loading only a subset of items at a time, which reduces memory usage and speeds up page rendering.
Where can I learn more about Magento 2 custom pagination?
You can refer to Magento DevDocs, community forums, or open-source modules that demonstrate custom collection pagination implementations.