Guide for: How to get Base Url and Current Url in Magento 2.4.x
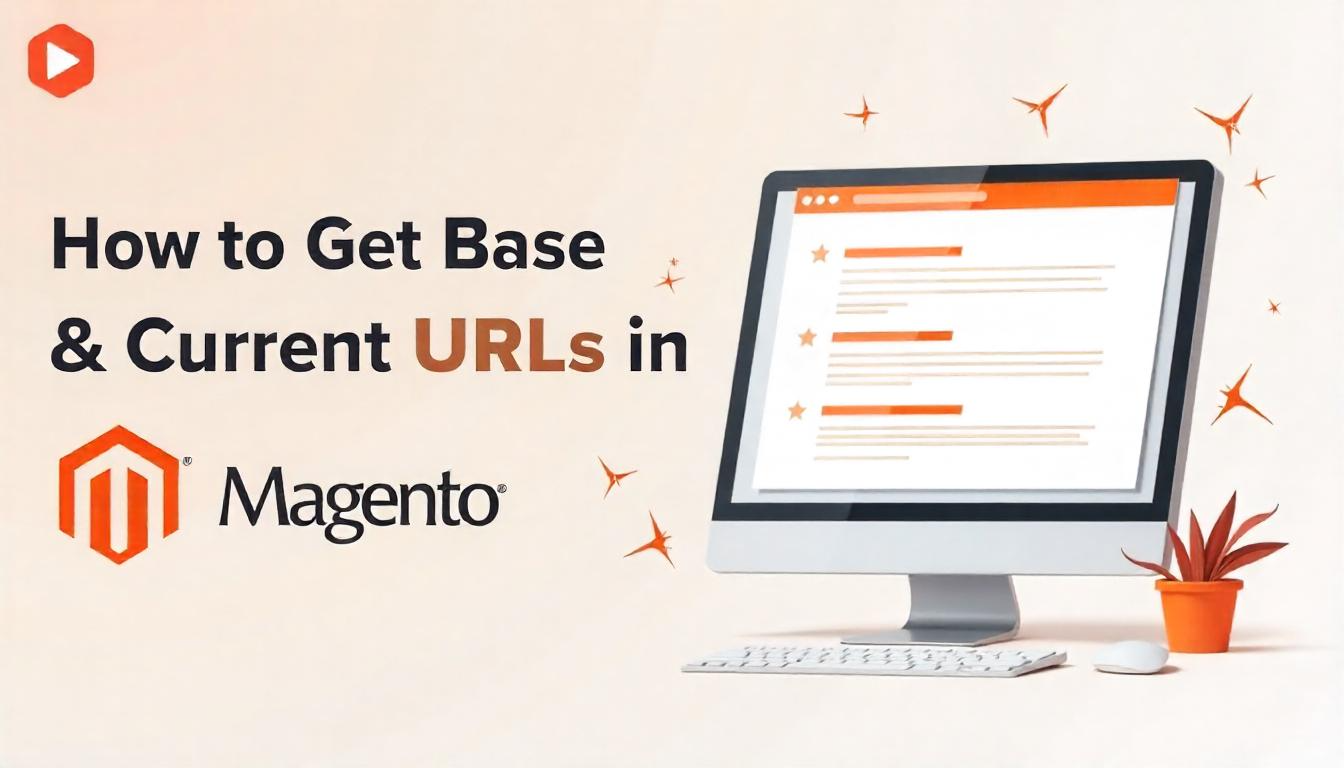
Guide for: How to get Base Url and Current Url in Magento 2.4.x
Magento 2.4.7 provides two primary, dependency-injected APIs for URL management—the Store Manager (Magento\Store\Model\StoreManagerInterface) and the URL Builder (Magento\Framework\UrlInterface). Together, they let you retrieve store-scoped base URLs (web, media, static), generate custom route URLs (secure or not), inspect the current request URL, and append query parameters—all in an upgrade-safe, testable manner. Below, you’ll find a holistic guide—beyond simply calling methods—on architecting URL retrieval, organizing your code, handling multi-store scenarios, ensuring SEO-friendly links, and avoiding common pitfalls, with best practices drawn from official Adobe Commerce documentation, community knowledge, and real-world patterns.
Table Of Content
- Deep Dive into Magento 2 URL Generation Architecture
- Implementing URL Services Using Dependency Injection in Magento 2
- Retrieving Magento 2 Store URLs Programmatically
- Retrieving the Current URL and Performing Security Checks in Magento 2
- Generating Custom and Secure Route URLs in Magento 2
- Conclusion
- FAQs
Deep Dive into Magento 2 URL Generation Architecture
Magento 2 features a modular and layered URL generation system that separates concerns between configuration-based URLs and programmatically generated routes. This division improves flexibility, scalability, and multi-store support.
1. Base URL Management
Magento stores base URLs in the configuration at the store, website, or default level. These include:
- Base URL (Unsecure): Used for non-HTTPS access to the storefront.
- Base URL (Secure): Used for HTTPS requests, including checkout and customer areas.
- Media Base URL: Separate from static view files, this serves product images and CMS media.
- Static View Files URL: Used to serve CSS, JS, and other static assets.
These can be managed via:
- Admin Panel: Stores > Configuration > Web
- CLI:
config:set
commands - env.php or database overrides
Magento applies store scope logic so that each store view or website may serve content with distinct domain/subdomain URLs.
2. Programmatic URL Generation with URL Builder
Magento's \Magento\Framework\UrlInterface
and its concrete implementation provide dynamic route generation that includes:
- FrontName Resolution: Resolves modules and controllers using route configuration.
- Parameter Injection: Supports query parameters (_query), secure flags (_secure), and fragment anchors (_fragment).
- Custom Path Routing: Builds SEO-friendly and rewritable URLs using the URL rewrite system.
Example usage:
$url = $this->getUrl('checkout/cart', ['_secure' => true]);
This URL respects current store configuration, HTTPS context, and route rewrites.
3. Differences Between Static and Dynamic URLs
URL Type | Scope | Description |
---|---|---|
Base URLs | Store config | Defined per store/website, control domain and secure settings |
Static Asset URLs | View layer | Generated for CSS/JS/media, cache-busted for deployment versioning |
Dynamic Page URLs | URL Builder | Built via routes, respects rewrites, store codes, and HTTPS flags |
4. URL Rewrites in Routing System
Magento allows URL rewrites through both system-generated and custom entries:
- Product and Category Rewrites: Auto-generated from URL keys.
- CMS Pages: Use custom URL keys for cleaner routes.
- Custom Rewrites: Created in Admin > Marketing > URL Rewrites
These rewrites are handled in the url_rewrite table and applied in routing logic before controller execution.
5. Best Practices for URL Management
- Use \Magento\Framework\UrlInterface for dynamic routes instead of hardcoding URLs.
- Always define URL keys for categories, products, and CMS pages.
- Avoid duplicate URL keys across stores.
- Use secure URLs for checkout, login, and customer areas.
6. Common Pitfalls and How to Avoid Them
Issue | Cause | Resolution |
---|---|---|
Mixed content warnings | Using unsecure base URLs with HTTPS | Always define secure URLs in configuration |
Broken media/static URLs | Incorrect base media/static URLs | Verify configuration and static deployment |
Store code in URL unexpectedly | Enabled "Add Store Code to URLs" option | Disable it unless needed for multi-store access |
Hardcoded URLs in templates | Skipping use of URL builder methods | Refactor templates to use getUrl() or getBaseUrl() |
Magento’s URL architecture provides a highly configurable and extendable system to manage all types of URLs across store views and environments. Mastery of the URL Builder, scope-based base URLs, and rewrite engine ensures seamless navigation, SEO optimization, and environment compatibility.
Implementing URL Services Using Dependency Injection in Magento 2
Dependency Injection (DI) is a core design principle in Magento 2. It promotes clean, modular, and testable code. When working with URL generation, developers should inject services like UrlInterface
and StoreManagerInterface
into their classes instead of using the Object Manager directly.
Why Use Constructor-Based Dependency Injection?
Constructor injection is preferred in Magento 2 for the following reasons:
- Improves Testability: Services can be easily mocked during unit testing.
- Follows Magento's Best Practices: Avoids tight coupling and direct use of the Object Manager.
- Ensures Clear Dependencies: Makes dependencies explicit in the constructor signature.
- Enhances Maintainability: Code becomes easier to manage, refactor, and extend.
Relevant Services for URL Handling
Service Interface | Purpose |
---|---|
Magento\Framework\UrlInterface | Generates dynamic URLs (routes, rewrites, query strings, etc.) |
Magento\Store\Model\StoreManagerInterface | Provides access to store-specific data, including base URLs |
Magento\Framework\View\Element\Template\Context | Provides access to context objects, including the URL builder |
Sample Block Class with Proper Injection
<?php
namespace Vendor\Module\Block;
use Magento\Framework\View\Element\Template;
use Magento\Store\Model\StoreManagerInterface;
use Magento\Framework\UrlInterface;
class UrlDemo extends Template
{
private StoreManagerInterface $storeManager;
private UrlInterface $urlBuilder;
public function __construct(
Template\Context $context,
StoreManagerInterface $storeManager,
UrlInterface $urlBuilder,
array $data = []
) {
parent::__construct($context, $data);
$this->storeManager = $storeManager;
$this->urlBuilder = $urlBuilder;
}
public function getCustomUrl(): string
{
return $this->urlBuilder->getUrl('custom/route', ['_secure' => true]);
}
public function getMediaBaseUrl(): string
{
return $this->storeManager->getStore()->getBaseUrl(\Magento\Framework\UrlInterface::URL_TYPE_MEDIA);
}
}
Key Implementation Notes
getUrl('custom/route'):
Generates a route-based URL that respects store settings and rewrites.['_secure' => true]:
Ensures the URL uses HTTPS when required.getBaseUrl(URL_TYPE_MEDIA):
Returns the store-specific media base path, useful for images or uploads.
Best Practices for URL Injection
- Never call the Object Manager directly inside methods.
- Use the
Context
object when extending template-based classes—it already provides access to common services. - Isolate URL logic inside helper classes or blocks, especially when rendering dynamic URLs in templates.
Additional Resources for Developers
- Use
<preference>
indi.xml
only when overriding existing implementations. - Use
getUrl()
in templates sparingly and only when$block
provides it via proper injection.
Retrieving Magento 2 Store URLs Programmatically
Magento 2 provides multiple ways to retrieve various types of URLs dynamically. The Store object, accessible through the StoreManagerInterface
, allows developers to fetch base, media, and static resource URLs for use in templates, scripts, and custom modules.
This method ensures that URLs are consistent with the store's configuration, respecting store scopes, security settings (HTTP/HTTPS), and versioned asset paths.
1. Understanding Base URL Types in Magento 2
Magento categorizes base URLs into specific types for better separation of concerns. The getBaseUrl()
method on the store object accepts one of several predefined constants from the UrlInterface
.
Usage Pattern:
<?php
$store = $this->storeManager->getStore();
echo $store->getBaseUrl(\Magento\Framework\UrlInterface::URL_TYPE_WEB);
echo $store->getBaseUrl(\Magento\Framework\UrlInterface::URL_TYPE_MEDIA);
echo $store->getBaseUrl(\Magento\Framework\UrlInterface::URL_TYPE_STATIC);
2. Supported Base URL Types
The table below outlines each URL type constant, what it returns, and typical use cases.
URL Type Constant | Description | Example Output |
---|---|---|
URL_TYPE_WEB | Returns the storefront base URL | http://yourdomain.com/ |
URL_TYPE_LINK | Similar to WEB, but may exclude store code in some cases | http://yourdomain.com/ |
URL_TYPE_DIRECT_LINK | Generates direct URL links, store code agnostic | http://yourdomain.com/ |
URL_TYPE_MEDIA | Returns the base URL for media assets | http://yourdomain.com/pub/media/ |
URL_TYPE_STATIC | URL to static view files (CSS, JS) with cache busting | http://yourdomain.com/pub/static/version12345/ |
3. Best Practices When Working with Store URLs
- Always use
StoreManagerInterface
for retrieving URLs instead of hardcoding paths. - Use
getBaseUrl()
with the appropriate constant to retrieve the correct type of URL. - For static and media files, Magento may append version strings for cache busting—do not rely on hardcoded paths.
- Respect HTTPS settings by using
_secure => true
when generating dynamic URLs if needed. - Do not concatenate paths manually—use Magento’s built-in URL service methods.
4. Dynamic Usage in Templates and Blocks
For example, to load an image from the media directory:
$imageUrl = $this->storeManager->getStore()->getBaseUrl(UrlInterface::URL_TYPE_MEDIA) . 'catalog/product/sample.jpg';
Or, to reference a JavaScript file from the static directory:
$scriptUrl = $this->storeManager->getStore()->getBaseUrl(UrlInterface::URL_TYPE_STATIC) . 'frontend/Theme_Name/en_US/js/custom.js';
Magento’s structured base URL system allows you to reliably access different types of resources, maintain multi-store compatibility, and avoid issues with incorrect or outdated paths. Using the correct base URL constants ensures your code is upgrade-safe, cache-aware, and aligned with best practices.
Retrieving the Current URL and Performing Security Checks in Magento 2
Magento provides different ways to fetch the current URL depending on your needs and how much information you require from the request. The URL retrieval can be done with or without query parameters, and security checks can also be implemented to verify if the request is using HTTPS.
1. Retrieving the Current URL
- StoreManager Method (
getCurrentUrl
):
ThegetCurrentUrl
method, available through the Store Manager, allows you to retrieve the URL of the current page. By default, this will return the URL without query parameters, but you can pass a boolean argument to include or exclude the query string. - Syntax:
$this->storeManager->getStore()->getCurrentUrl($includeQuery = false
- Example:
- Without query string:
- With query string:
- UrlInterface Method (
getCurrentUrl
):
If you need the full URL of the current request, including all query parameters, you can use the
UrlInterface
method. This always returns the full URL, including the protocol (HTTP/HTTPS), domain, path, and query parameters if present. - Syntax:
$this->urlBuilder->getCurrentUrl()
- Example:
echo $store->getCurrentUrl(false);
echo $store->getCurrentUrl(true);
echo $this->urlBuilder->getCurrentUrl();
2. Checking for HTTPS (Secure URL)
Magento offers an easy way to verify whether the current URL is secure (i.e., uses HTTPS). The isCurrentlySecure()
method of the StoreManagerInterface
can be used to check if the current store's URL is secure.
- StoreManager Method (
isCurrentlySecure
):
This method returns a boolean value, indicating whether the current URL is secure (HTTPS) or not (HTTP). - Syntax
$this->storeManager->getStore()->isCurrentlySecure()
- Example:
$isSecure = $this->storeManager->getStore()->isCurrentlySecure();
This will return true
if the current request uses HTTPS, and false
if it uses HTTP.
3. Table: URL Retrieval and Security Check Methods
Method | Description | Example Output |
---|---|---|
getCurrentUrl($includeQuery = false) | Retrieves the current URL, optionally including query parameters. Default excludes them. | http://example.com/product?id=123 |
getCurrentUrl(true) | Retrieves the current URL, including query parameters. | http://example.com/product?id=123&ref=home |
getCurrentUrl() (using UrlInterface) | Retrieves the full URL of the current request, including all parameters and protocol. | https://example.com/product?id=123&ref=home |
isCurrentlySecure() | Checks if the current URL uses HTTPS (secure). | true (if HTTPS), false (if HTTP) |
4. Best Practices for URL Handling
- Avoid Hardcoding URLs: Always use Magento's URL Builder and Store Manager to fetch URLs dynamically to ensure that your site is adaptable to different environments and configurations.
- Always Check for HTTPS: If handling sensitive data like passwords or payment details, ensure the request is made over a secure (HTTPS) connection.
- Dynamic Query Handling: When dealing with query parameters, always verify if you need them. In some cases, they might expose sensitive data or need to be sanitized.
Magento's robust URL handling capabilities allow for flexible and secure retrieval of URLs. By utilizing the StoreManager
and UrlInterface
services, developers can fetch URLs dynamically while also implementing secure URL checks for enhanced security. These features are especially important in multi-store setups or when dealing with different environments (development, staging, production).
Generating Custom and Secure Route URLs in Magento 2
In Magento 2, URL generation is a core part of creating dynamic and customizable routes. The URL builder helps generate URLs for both custom and secure routes, which can include query parameters and specific conditions, such as whether the URL should be HTTP or HTTPS. This flexibility is essential for dynamic eCommerce sites, ensuring the proper generation of URLs for customer login, product pages, and other critical functionalities.
1. Basic Route URL Generation
To generate URLs for specific routes within the Magento 2 application, you can use the getUrl()
method provided by the UrlInterface
. This method builds a URL for a given route and any parameters passed to it.
For example, generating a URL for customer login can be done as follows:
// Customer login (HTTP)
echo $this->urlBuilder->getUrl('customer/account/login');
To create a secure link using HTTPS, the _secure
parameter can be set to true
:
// Secure login link (HTTPS)
echo $this->urlBuilder->getUrl('customer/account/login', ['_secure' => true]);
This ensures that the generated URL respects the current store's security settings, including whether the store operates over HTTPS.
2. Adding Query Parameters
In addition to basic route URLs, Magento allows appending query parameters to URLs. This is useful for adding additional data to the URL, such as filters or search parameters. You can chain multiple setQueryParam()
calls before calling getUrl()
to add query parameters.
Here’s an example of how to append query parameters to a search result URL:
$url = $this->urlBuilder->setQueryParam('foo', 'bar')
->setQueryParam('baz', 'qux')
->getUrl('catalogsearch/result/index');
echo $url; // Output: /catalogsearch/result/index?foo=bar&baz=qux
This allows for dynamic URL generation based on specific needs, such as filtering or sorting products within the catalog.
3. Securing Route URLs
When generating URLs for sensitive areas such as customer accounts, checkout, or login, it’s critical to ensure that the URLs are secure. This can be achieved by setting the _secure parameter to true. For instance, when generating a checkout URL:
// Secure checkout URL
echo $this->urlBuilder->getUrl('checkout/cart', ['_secure' => true]);
Ensuring that URLs are secure helps protect sensitive customer data and enhances the overall security of your Magento store.
4. Advanced URL Generation Techniques
Generating Store-Specific URLs
Magento’s URL generation system can be extended to generate store-specific URLs, especially when managing multiple store views. The base URL for each store view can differ, and Magento provides mechanisms to adjust the URL accordingly. For example, to generate a URL for a product in a specific store:
// Generate URL for a product in a specific store
$store = $this->storeManager->getStore('store_code');
echo $this->urlBuilder->getUrl('catalog/product/view', ['_store' => $store->getId()]);
This approach ensures that URLs respect the store view and allow for proper localization or multi-store setups.
Using URL Rewrites
Magento 2 supports URL rewrites to customize URLs for better SEO. When generating URLs for products, categories, or CMS pages, you can leverage the built-in URL rewrite functionality to ensure clean, SEO-friendly URLs are generated.
// Generating SEO-friendly URL for a product
$product = $this->productRepository->getById($productId);
echo $this->urlBuilder->getUrl('catalog/product/view', ['_secure' => true, '_query' => ['product' => $product->getUrlKey()]]);
By using URL rewrites and custom URL keys, you can optimize your URLs for search engines, improving their ranking and visibility.
5. Common Issues and Resolutions
Issue | Cause | Resolution |
---|---|---|
URL generated with incorrect scheme | The _secure parameter is not set for secure areas |
Always ensure _secure is set to true for sensitive routes |
Query parameters not appended | Incorrect usage of setQueryParam() chaining |
Ensure proper chaining of setQueryParam() calls before getUrl() |
URL doesn't respect store code | Store code not specified or misconfigured | Use the _store parameter to ensure the correct store URL is generated |
Magento 2’s flexible URL generation system provides a robust mechanism to create secure, dynamic, and SEO-friendly URLs. By leveraging the UrlInterface and incorporating best practices such as securing sensitive URLs and using URL rewrites, you can ensure that your store’s URLs are both functional and optimized for customer experience and search engine visibility.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
In Magento 2.4.7, managing and retrieving URLs is a core part of dynamic content rendering, secure navigation, and multi-store support. By using StoreManagerInterface and UrlInterface, developers can efficiently fetch base URLs, media/static resource paths, current URLs, and custom route links—all while maintaining Magento’s best practices for performance and security.
Whether you're customizing templates, building plugins, or integrating frontend components, knowing how to correctly retrieve and structure URLs empowers you to deliver reliable and scalable Magento solutions. With the latest improvements in Magento 2.4.7 and the examples shared here, you're now equipped to handle URL generation with precision and flexibility—essential for any serious Magento development in 2025 and beyond.
FAQs
How do I get the base URL in Magento 2 using StoreManagerInterface?
You can use $this->storeManager->getStore()->getBaseUrl()
to get the base URL.
What is the difference between URL_TYPE_WEB and URL_TYPE_MEDIA?
URL_TYPE_WEB returns the main website base URL, while URL_TYPE_MEDIA returns the media URL (e.g., pub/media).
How can I retrieve the current page URL in Magento 2?
You can call $this->storeManager->getStore()->getCurrentUrl()
to get the current page URL.
How do I create a secure custom URL?
Use $this->storeManager->getUrl('route/path', ['_secure' => true])
to generate an HTTPS URL.
How can I get the login page URL?
Use $this->storeManager->getUrl('customer/account/login')
or $this->urlBuilder->getUrl('customer/account/login')
.
How do I check if the current URL is secure in Magento 2?
You can use $this->storeManager->isUrlSecure()
to determine if the current page is served over HTTPS.
What’s the use of UrlInterface in Magento 2?
UrlInterface is used for building and retrieving URLs programmatically, especially in plugins and observers.
How can I set query parameters in a URL using UrlInterface?
You can use $this->urlBuilder->setQueryParam('key', 'value')
to set a single query parameter.
Can I add multiple query parameters in Magento 2 URLs?
Yes, call setQueryParam()
multiple times and then use getUrl()
to return the final URL.
How do I retrieve media URLs for product images or uploads?
Use getBaseUrl(\Magento\Framework\UrlInterface::URL_TYPE_MEDIA)
to get the media base path.
What URL does URL_TYPE_STATIC return?
It returns the static assets path (like CSS/JS), typically something like /pub/static/version...
.
Where can I use these URL helpers in Magento 2?
You can use them in blocks, templates, controllers, plugins, and observers depending on your implementation needs.
What is the output of getBaseUrl() without parameters?
It returns the default base URL with the store code (e.g., http://example.com/default/
).
Can I override Magento URLs in my module?
Yes, using dependency injection and URL rewrite techniques, you can override or customize Magento URLs.
How can I use these URL methods in PHTML template files?
You can call injected methods from your block class using $block->getMethod()
inside the template.