How to Update Product Using REST API (/V1/products/:sku) in Magento 2.4.7 (2025)
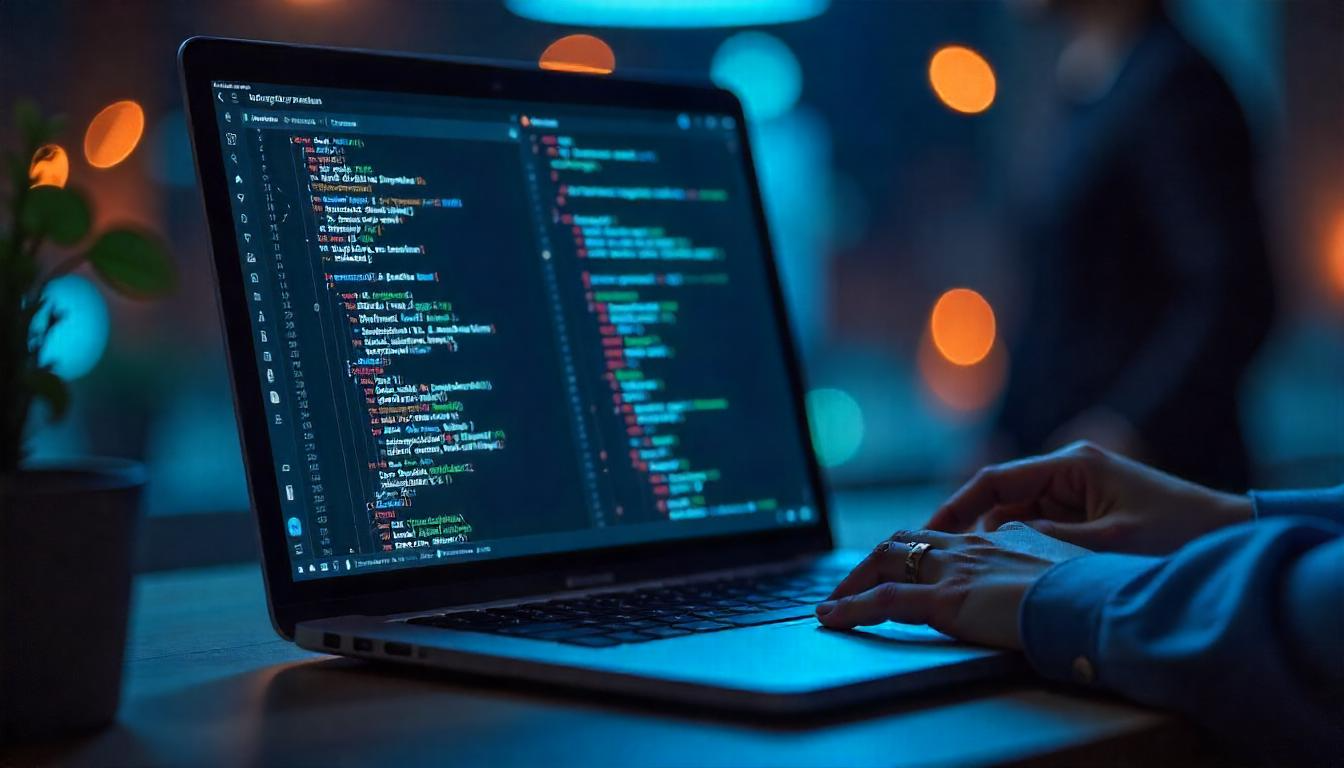
How to Update Product Using REST API (/V1/products/:sku) in Magento 2.4.7 (2025)
Updating products programmatically via REST API is a powerful and flexible method in Magento 2.4.7. It is especially useful when automating catalog updates or integrating with third-party systems like ERPs, CRMs, or mobile applications.
Table Of Content
Comprehensive Guide to Magento 2.4.7 Product Update via REST API
Magento 2.4.7 provides a robust RESTful API system for managing catalog data. One of the critical operations is updating existing product information using the PUT method. This API endpoint is essential for synchronizing catalog data from third-party systems, automating updates, and performing bulk edits programmatically.
API Configuration Overview
Parameter | Details |
---|---|
Magento Version | 2.4.7 (compatible with 2025 enhancements) |
HTTP Method | PUT |
Endpoint | /rest/V1/products/:sku |
Authentication | OAuth 1.0a or Bearer Token (preferred) |
Content-Type | application/json |
Scope | Admin REST role required |
Usage Purpose
This endpoint is used to update any product attribute such as name, price, SKU-related metadata, visibility, and custom attributes. It's highly useful in:
- Inventory management synchronization
- Custom ERP integrations
- Price and promotion adjustments
- SEO attribute updates (meta title, meta description, etc.)
Request URI and Structure
URI Format:
/rest/V1/products/{sku}
Example:
PUT /rest/V1/products/test-sku-123
Required Headers
Authorization: Bearer <access_token>
Content-Type: application/json
Payload Structure
A basic payload for updating a product might look like:
{
  "product": {
    "name": "Updated Product Name",
    "price": 149.99,
    "status": 1,
    "visibility": 4,
    "type_id": "simple",
    "custom_attributes": [
      {
        "attribute_code": "meta_title",
        "value": "New SEO Title"
      },
      {
        "attribute_code": "meta_description",
        "value": "Updated description for better visibility."
      }
    ]
  }
}
Key Product Properties for Update
Property | Description | Example Value |
---|---|---|
name | Product name shown in the catalog and product detail page | "Updated Product" |
price | Final product price (excluding tax) | 99.95 |
status | 1 for Enabled, 2 for Disabled | 1 |
visibility | 1 = Not Visible, 2 = Catalog, 3 = Search, 4 = Catalog & Search | 4 |
custom_attributes | Custom EAV attributes associated with the product | meta_title, description |
type_id | Product type (simple, configurable, etc.) | "simple" |
Response Example
Upon successful update, Magento returns a full product object with updated values.
{
  "id": 1234,
  "sku": "test-sku-123",
  "name": "Updated Product Name",
  "price": 149.99,
  ...
}
Common Issues and Resolutions
Issue | Cause | Resolution |
---|---|---|
Invalid SKU error | The SKU doesn't exist in the current catalog | Ensure the SKU is correct and available in the requested scope |
Unauthorized access | Missing or invalid bearer token | Generate a new access token using Admin API credentials |
415 Unsupported Media Type | Missing or incorrect Content-Type header | Always set Content-Type to application/json |
Attribute value not saved | Attribute not included in the allowed EAV list | Verify the attribute is set as "Used in API" in the admin backend |
Security Considerations
- Always use HTTPS endpoints to avoid transmitting access tokens in plain text.
- Limit API access via integration tokens with scoped permissions.
- Log and monitor API usage to prevent abuse or unauthorized changes.
Best Practices for API Updates
- Validate the JSON payload before sending to prevent runtime errors.
- Use admin token authentication instead of user tokens for product updates.
- Avoid frequent PUT operations in bulk; consider using the bulk API /V1/products/batch for high volume updates.
Authenticating Magento 2 REST API Requests
To securely access Magento 2's REST API and perform administrative operations (such as updating products), you must first generate an access token using admin credentials. This access token authorizes your HTTP requests to interact with the system.
1. Magento 2 API Authentication Overview
- API Type: Admin REST API
- Authentication Method: Token-based (Bearer Token)
- Magento Version: 2.4.7 and above (2025-ready)
- Token Lifetime: As configured in admin panel or default session settings
- Permissions Required: Admin role with access to relevant API resources
2. Generate Admin Access Token
Endpoint
POST /rest/V1/integration/admin/token
Request Headers
- Content-Type:
application/json
- Accept:
application/json
Request Body
{
  "username": "admin",
  "password": "admin123"
}
Use a secure method to manage credentials in production environments.
Response
"<access_token>"
- The returned value is a plain string containing your access token.
- This token must be used in the
Authorization
header of subsequent API requests.
3. Accessing Secure Endpoints
Once you have the token, include it in all REST API calls using the Authorization
header:
Authorization: Bearer <access_token>
4. Example: Update Product by SKU
Use the following format to update a product:
Endpoint Structure
PUT /rest/V1/products/{sku}
Full Example
PUT http://yourdomain.com/rest/V1/products/test-sku
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
Sample Request Body
{
  "product": {
    "name": "Updated Product Name",
    "price": 129.99,
    "status": 1,
    "visibility": 4,
    "type_id": "simple",
    "custom_attributes": [
      {
        "attribute_code": "meta_title",
        "value": "Updated Meta Title"
      }
    ]
  }
}
5. Common Issues and Resolutions
Issue | Cause | Recommended Solution |
---|---|---|
Invalid credentials | Wrong username or password | Confirm admin account credentials |
401 Unauthorized | Missing or invalid bearer token | Include a valid token in the Authorization header |
403 Forbidden | User lacks API permissions | Assign proper API access rights in the admin panel |
415 Unsupported Media Type | Incorrect Content-Type header | Always set Content-Type to application/json |
Invalid SKU | The product SKU does not exist | Verify SKU before making the PUT request |
Magento 2: Update Product Programmatically via PHP cURL Script
Updating product data using a REST API in Magento 2 is a powerful tool for automation and integration with external systems. Below is a fully documented example of how to perform a product update securely and correctly using PHP and cURL.
Sample PHP cURL Script to Update a Product
This script updates a product’s visibility, price, and description using its SKU.
<?php
$url = "http://yourdomain.com/rest";
$accessToken = "YOUR_ACCESS_TOKEN";
$headers = [
    'Content-Type:application/json',
    'Authorization:Bearer ' . $accessToken
];
$sku = "test-sku";
$apiUrl = $url . "/V1/products/" . rawurlencode($sku);
// Define data payload
$data = [
    "product" => [
        "visibility" => 2, // 2 = Catalog
        "price" => 250.00,
        "custom_attributes" => [
            [
                "attribute_code" => "description",
                "value" => "Updated product description for API demonstration."
            ]
        ]
    ]
];
// Initialize cURL session
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $apiUrl);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "PUT");
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false); // Disable only in development
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
// Execute and fetch response
$response = curl_exec($ch);
curl_close($ch);
// Output the response
echo "<pre>"; print_r(json_decode($response, true));
Notes on Script Behavior
- The Authorization header must be set with a valid token.
- The rawurlencode function ensures special characters in SKUs are properly encoded.
- The custom_attributes array is used to update additional product attributes.
CURLOPT_SSL_VERIFYPEER
should be set totrue
in production for security.
Common Updatable Product Fields
Field | Description | Example Value |
---|---|---|
name | Product name visible in catalog and product page | "New Product Title" |
price | Product price excluding tax | 249.99 |
status | Product status (1 = Enabled, 2 = Disabled) | 1 |
visibility | Visibility scope (1 = Not Visible, 2 = Catalog, 3 = Search, 4 = Both) | 2 |
type_id | Product type identifier (simple, configurable, etc.) | "simple" |
sku | Product Stock Keeping Unit, unique per product | "test-sku" |
custom_attributes | Used to modify or add EAV attributes to the product | description, meta_title |
Troubleshooting & Best Practices
Issue | Likely Cause | Recommended Fix |
---|---|---|
Invalid SKU | SKU not found in catalog | Verify that the SKU exists and is properly indexed |
Unauthorized (401) | Missing or expired token | Generate a new admin token and use it in the header |
Invalid Content-Type (415) | Wrong or missing Content-Type header | Always set Content-Type: application/json |
Attribute value not saving | Attribute is not API-exposed or not configured properly | Enable "Used in API" for the attribute in admin |
HTTP 403 Forbidden | Insufficient permissions for the API user | Check role permissions for assigned Admin REST role |
Extended API Examples for Product Attribute Updates
When interacting with Magento 2's REST API (version 2.4.7 and above), it’s essential to understand how to structure specific payloads to update individual attributes like product status, SEO metadata, and media labels. Below are practical and detailed JSON examples to illustrate common update scenarios.
1. Updating Product Status
Use this payload to enable or disable a product in the catalog.
{
  "product": {
    "status": 2
  }
}
Details:
Value | Meaning |
---|---|
1 | Enabled |
2 | Disabled |
The status
field controls product visibility and availability on the storefront.
2. Updating SEO Meta Title
To improve on-page SEO, you can directly update the meta title for a product using custom_attributes
.
{
  "product": {
    "custom_attributes": [
      {
        "attribute_code": "meta_title",
        "value": "New Optimized SEO Title"
      }
    ]
  }
}
Note: The meta title affects how your product appears in search engine results and browser tabs.
3. Updating Product Image Label
Set or modify the image label that appears on product images (e.g., "Front View", "Main Display").
{
  "product": {
    "custom_attributes": [
      {
        "attribute_code": "image_label",
        "value": "Main Front View"
      }
    ]
  }
}
Use Case: This is often used in themes that display image labels on thumbnails or product detail views for accessibility and presentation.
Additional Attribute Update Examples
Here are more custom_attributes
you can update via the API:
Attribute Code | Description | Example Value |
---|---|---|
meta_description | Controls SEO description snippet | "Buy the best product..." |
short_description | Shown on listing and summary pages | "Lightweight and durable" |
tax_class_id | Assigns tax class to the product | 2 |
manufacturer | Sets the brand or manufacturer name | "Generic Brand" |
color | Updates product color if defined as EAV | "Red" |
Best Practices
- Always wrap attribute updates within the "
product
" object. - Use
attribute_code
exactly as defined in your product EAV configuration. - Ensure any custom attributes are marked as "Used in API" in the admin backend to make them accessible via REST.
- Confirm values match allowed options or data types configured in Magento (e.g., dropdowns or integers).
Use Cases for Product API in Magento 2.4.x
1. Sync Products with ERP System
By leveraging the Magento API, businesses can sync their product catalog with external ERP (Enterprise Resource Planning) systems. This allows for real-time updates of product data such as stock levels, pricing, and other critical information, ensuring consistency across all platforms.
Key Benefits:
- Seamless integration with ERP systems to avoid manual data entry errors.
- Real-time synchronization of product information, reducing the risk of discrepancies between the website and backend systems.
- Streamlined inventory management and order processing.
2. Regularly Update Product Pricing
Managing pricing across a large product catalog can be time-consuming. With the use of the product API, businesses can automate the updating of product prices based on various factors such as discounts, seasonal changes, or dynamic pricing strategies.
Key Benefits:
- Automation of pricing updates across all sales channels, ensuring consistent pricing strategies.
- Real-time pricing adjustments based on market conditions or promotions.
- Reduces the need for manual price updates, saving time and reducing human error.
3. Push SEO Data Updates for Meta Tags
The Magento API can be utilized to automate updates to SEO-related fields like meta titles and descriptions. This is especially useful when refreshing SEO strategies or ensuring that all products are correctly optimized for search engines.
Key Benefits:
- Automatically update meta tags for large product catalogs, saving SEO specialists time.
- Ensure that SEO data remains consistent across all product pages, enhancing search engine visibility.
- Support for various custom SEO fields like meta keywords and descriptions, improving organic search performance.
4. Automate Catalog Changes from CSV/JSON
Bulk product uploads or modifications can be done seamlessly by automating catalog changes through CSV or JSON files. By integrating the Magento product API with external systems or internal tools, businesses can easily update product details, categories, or attributes without manual intervention.
Key Benefits:
- Import/export products in bulk from CSV or JSON files, minimizing manual work.
- Ensure data accuracy by automating the process and reducing human errors.
- Speed up catalog updates and changes, making it easier to launch new products or modify existing ones.
Summary Table of Use Cases
Use Case | Description | Key Benefits |
---|---|---|
Sync products with ERP system | Real-time synchronization of product catalog data with external ERP systems. | Reduced errors, real-time data synchronization, efficient inventory management. |
Regularly update product pricing | Automate the updating of product prices based on various business factors. | Consistent pricing, automated updates, reduced manual effort. |
Push SEO data updates for meta tags | Automate the update of SEO-related data such as meta titles and descriptions. | Improved SEO, consistent meta tags, time-saving automation. |
Automate catalog changes from CSV/JSON | Bulk upload and update product details from external data files. | Bulk product updates, minimal manual work, faster catalog management. |
By leveraging the power of the Magento Product API for these use cases, businesses can significantly improve their operational efficiency and streamline their eCommerce workflows.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
In conclusion, Magento 2 provides powerful and flexible tools for managing product data, including the ability to update products via REST APIs. By using the PUT
method with the /V1/products/:sku
endpoint, you can seamlessly update product attributes such as price, visibility, descriptions, and custom attributes, all while leveraging the security of authentication tokens for API access.
This approach is particularly useful for managing product data at scale and integrating external systems that need to interact with your Magento store. Whether you are performing bulk updates or adjusting individual product details, Magento's robust API system ensures smooth and secure communication between your platform and external tools.
By following the best practices outlined in this guide, including correct API configurations, using headers like Content-Type<: application/json
and Authorization: Bearer
, you can streamline your product management workflow and ensure your store remains up-to-date with minimal manual intervention.
Magento 2 continues to evolve, and staying updated with the latest features and methodologies is essential to maintaining an efficient and secure eCommerce experience for both you and your customers.
FAQs
How do I update a product using the REST API in Magento 2?
To update a product using REST API in Magento 2, you need to send a PUT request to the URL /rest/V1/products/:sku, passing the updated data in JSON format. Include the proper headers, such as Content-Type: application/json and Authorization: Bearer
What is the purpose of using the PUT method for updating products?
The PUT method is used in the REST API to update the existing product data. It allows you to modify various product attributes such as price, visibility, and custom attributes.
How do I obtain the access token for using the Magento 2 API?
You can obtain the access token in Magento 2 by navigating to the Admin Panel, then to System > Extensions > Integration, and creating a new integration. After saving, you will be provided with an access token.
Can I update custom attributes for a product using the REST API?
Yes, you can update custom attributes for a product by including them in the "custom_attributes" section of your API request. Make sure to specify the attribute code and the new value.
What is the correct endpoint for updating a product in Magento 2?
The correct endpoint for updating a product in Magento 2 is /rest/V1/products/:sku, where :sku is the SKU of the product you want to update.
What headers should I use when updating a product through the Magento 2 API?
When updating a product, you should use the following headers: 'Content-Type: application/json' and 'Authorization: Bearer
How do I send a PUT request to update product data in Magento 2?
You can send a PUT request using cURL in PHP or any HTTP client by specifying the request method as PUT, setting the target URL, and passing the updated product data as JSON in the request body.
Can I use the Magento 2 REST API for bulk product updates?
The Magento 2 REST API is designed for individual product updates. For bulk updates, consider using batch processing or other methods such as data import tools or custom scripts.
What is the format of the data when updating a product through the API?
The data format for updating a product through the Magento 2 REST API is JSON. The request body should include product information, such as visibility, price, and custom attributes, in the correct structure.
What happens if I send invalid data when updating a product?
If invalid data is sent, the API will return an error message indicating the issue. Common errors include missing required fields or incorrect data types for product attributes.
How do I check the response after updating a product?
You can check the response by printing the response data using PHP’s print_r() function or by checking the status code. A successful update will typically return the updated product data or a success message.
Is it possible to update only one attribute of a product via the Magento 2 API?
Yes, you can update a single attribute of a product by including only the attribute you wish to modify in the request body, while leaving other attributes unchanged.
How can I update the product price using the Magento 2 API?
You can update the product price by including the "price" field in the product data when sending the PUT request to the API. Set the desired value for the price attribute.
Can I update the product visibility status using the Magento 2 API?
Yes, you can update the product visibility status by modifying the "visibility" attribute. The visibility field can have values like 1 (Not Visible Individually), 2 (Catalog), 3 (Search), or 4 (Catalog, Search).
What is the URL format for updating a product through the Magento 2 API?
The URL format for updating a product through the Magento 2 API is