Efficient Ways to Load Customer by ID in Magento 2
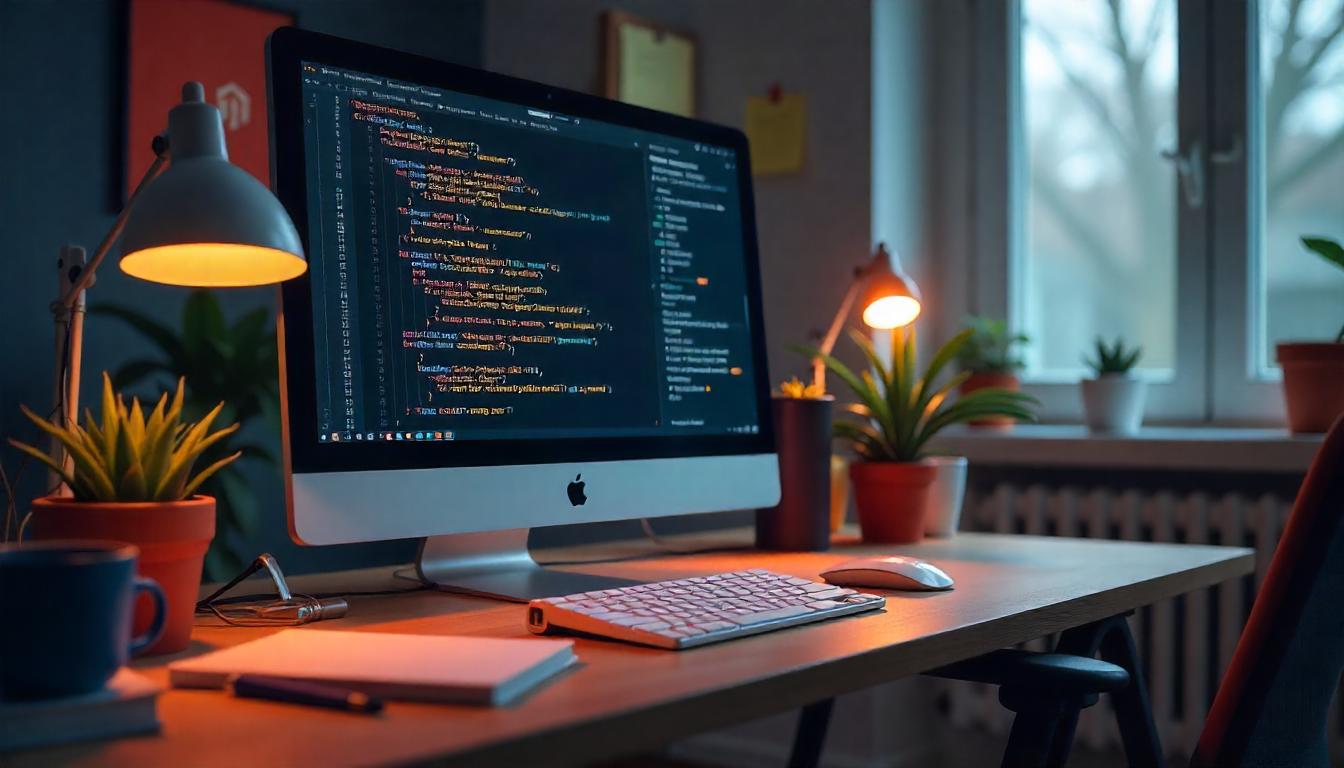
Efficient Ways to Load Customer by ID in Magento 2
Loading customer data by ID in Magento 2 is essential for managing customer information efficiently. This process is particularly useful for tasks like resolving queries, supporting data migration, or improving backend operations. Here’s a detailed look at three reliable methods:
Table Of Content
Why Efficient Customer Data Retrieval in Magento 2 Matters
Efficiently loading customer data by ID in Magento 2 is a crucial process for store owners. It streamlines operations, saves time, and ensures accuracy in handling customer information. Let’s explore its importance and how it impacts store performance.
Aspect | Why It Matters |
---|---|
Time Efficiency | Manually searching for customer data wastes time and resources. |
Better Decision-Making | Fast access to customer information improves responses and administrative tasks. |
Accurate Data Management | Ensures critical data is retrieved without errors, preventing operational issues. |
Streamlining Administrative Processes
Relying on manual methods to access customer data can bog down administrative efficiency. Loading customer data by ID allows store admins to focus on more critical tasks, like resolving customer complaints or queries swiftly. For instance, if a customer raises an issue, accessing their details quickly ensures better problem resolution, leading to improved satisfaction.
Enhancing Back-End Data Operations
Accurate data retrieval ensures seamless back-end operations, from order processing to system migrations. Errors in accessing or transferring customer data can disrupt workflows and damage customer trust. By loading customer data by ID, you avoid delays and maintain operational integrity.
Common Mistakes and How to Avoid Them
If admins previously relied on slow or error-prone methods, it's essential to switch to ID-based retrieval for reliability. Ensure the system is configured to handle customer IDs effectively, and double-check database integrations during migrations.
Effective Ways to Retrieve Customer Data by ID in Magento 2
1. Using the Constructor Method (Best Practice)
The constructor method is the recommended approach. It ensures code is clean, modular, and adheres to Magento 2 standards. Here's how to implement it:
API Repository to Use:\Magento\Customer\Api\CustomerRepositoryInterface
Code Example:
protected $_customerRepositoryInterface;
public function __construct(
\Magento\Customer\Api\CustomerRepositoryInterface $customerRepositoryInterface
) {
$this->_customerRepositoryInterface = $customerRepositoryInterface;
}
public function getCustomerById($customerId) {
return $this->_customerRepositoryInterface->getById($customerId);
}
How to Call the Function:
$customerId = 1;
$customer = $this->getCustomerById($customerId);
2. Using the Factory Method
This method is useful for loading customers in module-specific contexts. It is slightly less efficient but provides flexibility.
Code Example:
namespace Company\Module\Block;
class CustomerLoader extends \Magento\Framework\View\Element\Template
{
protected $customer;
public function __construct(
\Magento\Customer\Model\Customer $customer
) {
$this->customer = $customer;
}
public function loadCustomerById($customerId) {
return $this->customer->create()->load($customerId);
}
}
How to Use It:
How to Call the Function:
$customerId = 1;
$customer = $this->loadCustomerById($customerId);
3. Using Object Manager (Not Recommended)
The Object Manager is a quick solution but violates dependency injection principles. Avoid this unless there’s no alternative.
Code Example:
$objectManager = \Magento\Framework\App\ObjectManager::getInstance();
$customerData = $objectManager->create('Magento\Customer\Model\Customer')->load($customerId);
Key Points to Remember
- Constructor Method is the most reliable and preferred way. It supports clean and maintainable code.
- Factory Method works well for specific, encapsulated operations.
- Object Manager should only be a last resort. Magento discourages its use for standard practices.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Are the Best Ways to Load a Customer by ID in Magento 2?
The best way to load a customer by ID in Magento 2 is by using the Constructor method. It follows Magento’s dependency injection principles and ensures maintainable, clean code.
How Can I Use the Constructor Method to Load a Customer by ID?
To use the Constructor method, include the `\Magento\Customer\Api\CustomerRepositoryInterface` in your constructor and call the `getById()` function with the customer ID. This is the recommended approach for reliability and maintainability.
Can I Use the Factory Method to Load a Customer by ID?
Yes, the Factory method allows you to load a customer by ID. Use the `\Magento\Customer\Model\Customer` class, call the `create()` method, and then load the customer using the ID.
Why Is the Object Manager Method Not Recommended?
The Object Manager method bypasses dependency injection and violates Magento’s coding standards. It should only be used as a last resort or for quick, non-production testing.
What Are the Key Advantages of Using the Constructor Method?
The Constructor method ensures that your code adheres to Magento standards. It promotes better maintainability, cleaner architecture, and less coupling between modules.
How Do I Set Up the Factory Method for Customer Loading?
To use the Factory method, initialize the `\Magento\Customer\Model\Customer` class in your constructor. Use the `create()` method and call the `load()` function with the customer ID to retrieve the data.
What Are the Risks of Using the Object Manager?
Using the Object Manager can make your code harder to test and maintain. It also increases the risk of breaking changes during Magento updates. Always prefer dependency injection methods.
Can I Use the Constructor and Factory Methods Together?
While possible, combining these methods is not recommended. Stick to the Constructor method for primary operations and use the Factory method in specific, encapsulated cases.
How Do I Debug Issues When Loading Customers by ID?
Check if the customer ID exists in your database and ensure the correct repository or model is being called. Enable Magento’s developer mode to trace any errors in the logs.
Are There Extensions That Simplify Customer Data Management?
Yes, several extensions enhance customer management by providing GUI tools for filtering, editing, and exporting customer data. They can complement the native methods for loading customer information.