How to Retrieve the Payment Method from an Order in Magento
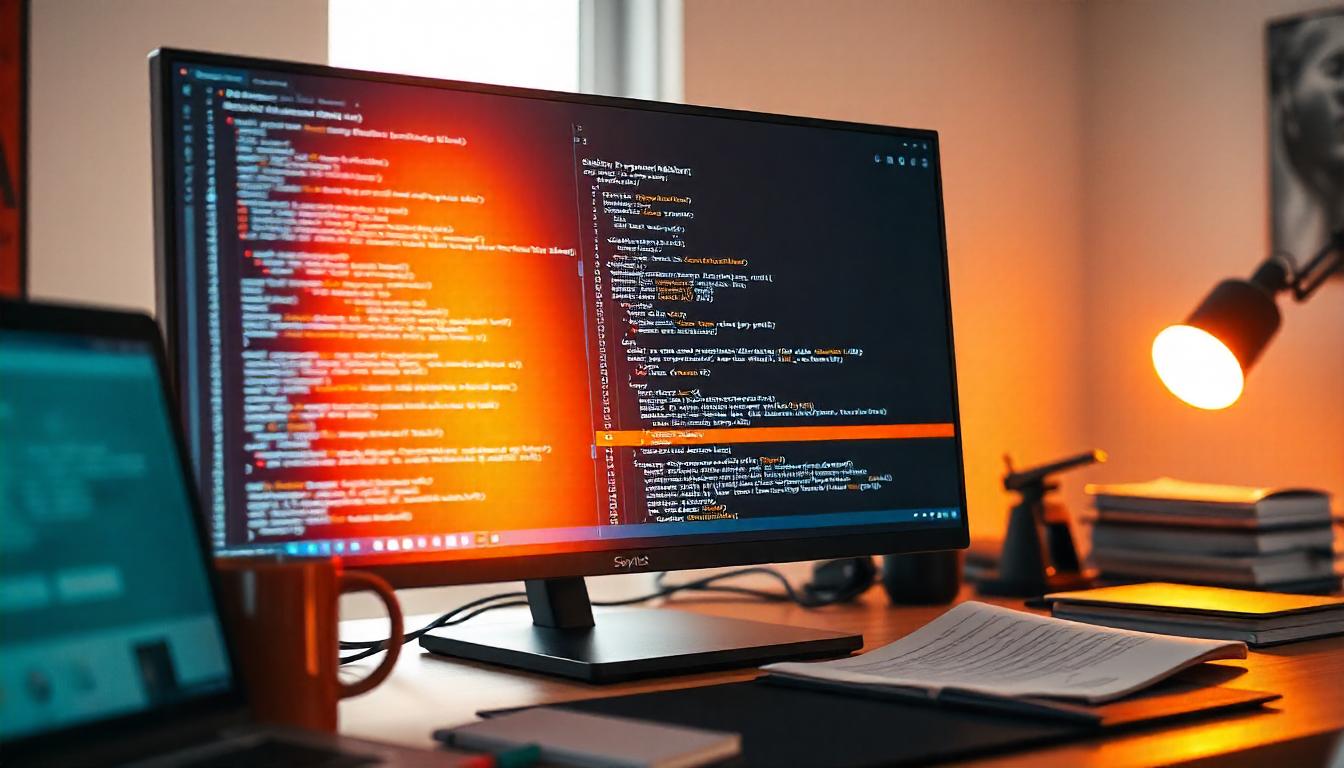
How to Retrieve the Payment Method from an Order in Magento
To retrieve the payment method from an order in Magento 2, you'll need to access the order object and use its payment data. Here’s a straightforward approach:
Table Of Content
Why You Should Display Detailed Order Information
Displaying detailed order information is a smart move for any Magento 2 store, especially when it comes to payment details. Here’s why:
- Build Customer Trust: Allowing customers to verify their payment details helps them feel more secure. When they can easily check if they entered the right information, it builds trust in your process.
- Reduce Mistakes: If there’s an error, customers can address it quickly. This means you won’t have to process incorrect orders, which can save time and prevent unnecessary hassle for both the store and the customer.
- Free Up Support Resources: By showing payment information on the order confirmation page, customers won’t need to reach out to your support team with basic payment queries. This frees up your support staff to focus on more critical issues.
- Load the Order by Increment ID: You'll need to load the order using the increment ID (e.g., 10000003).
- Get Payment Information: Once the order is loaded, access its payment information to retrieve the method used.
- The loadByIncrementId method loads the order by its unique ID.
- The $payment object is retrieved through $order->getPayment(), which contains all the payment details.
- Using $payment->getMethodInstance(), you can get the actual payment method instance, and $method->getTitle() gives you the title of the payment method used in that order.
In the end, clear order details enhance the shopping experience and make life easier for both your customers and your team. Keep your store transparent, and your customers will appreciate it.
How To Magento 2 Get Payment Method
To retrieve the payment method from an order in Magento 2, follow this simple and direct approach:
Here's the PHP code to get the payment method:
$orderIncrementId = 10000003;
$objectManager =\Magento\Framework\App\ObjectManager::getInstance();
$order = $objectManager->create('Magento\Sales\Model\Order')->loadByIncrementId($orderIncrementId);
$payment = $order->getPayment();
$method = $payment->getMethodInstance();
$methodTitle = $method->getTitle();
How It Works:
Additional Information:
If you need more than just the payment method title, you can also fetch additional details with $order->getPayment()->getAdditionalInformation().
This is helpful if your business needs more granular payment data.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What is the Purpose of Retrieving the Payment Method from an Order in Magento 2?
Retrieving the payment method allows store owners to track how payments were made, which is crucial for both customer service and reporting purposes. It helps in verifying transactions, handling refunds, and managing payment options for various orders.
How Can You Retrieve the Payment Method from an Order in Magento 2?
You can retrieve the payment method by first loading the order using its increment ID, then accessing the payment information. Here's a basic example:
$orderIncrementId = 10000003; $objectManager = \Magento\Framework\App\ObjectManager::getInstance(); $order = $objectManager->create('Magento\Sales\Model\Order')->loadByIncrementId($orderIncrementId); $payment = $order->getPayment(); $method = $payment->getMethodInstance(); $methodTitle = $method->getTitle();
What Code is Used to Retrieve the Payment Method in Magento 2?
The following code can be used to get the payment method from an order:
Where is the Payment Method Information Stored in Magento 2?
Payment method information is part of the order's payment object, which can be accessed using the $order->getPayment()
method. The method instance is retrieved by $payment->getMethodInstance()
, allowing you to access the method title and other details.
What Should Be Done After Retrieving the Payment Method?
After retrieving the payment method, you can use this data for various purposes such as displaying it on order confirmation pages, sending it in notifications, or for backend reporting. Be sure to test any changes thoroughly and clear Magento's cache if needed.
Are There Any Risks Involved in Retrieving Payment Information?
There are no inherent risks to retrieving the payment method as long as you handle the data securely. Ensure that sensitive payment information is never exposed inappropriately.
Can I Modify or Customize the Payment Method Display?
Yes, you can customize how the payment method is displayed by modifying the layout XML files or overriding templates in your theme. For example, you could show or hide payment information based on specific conditions or format it differently.
How Can I Debug Issues Related to Payment Method Retrieval?
If you're having trouble retrieving the payment method, check the order object and payment model for issues. Ensure that the increment ID is correct, and review the payment method instance to make sure it is properly loaded. Use Magento's debugging tools or log files for further troubleshooting.