Adding Custom Customer Attributes in Magento 2: A Guide
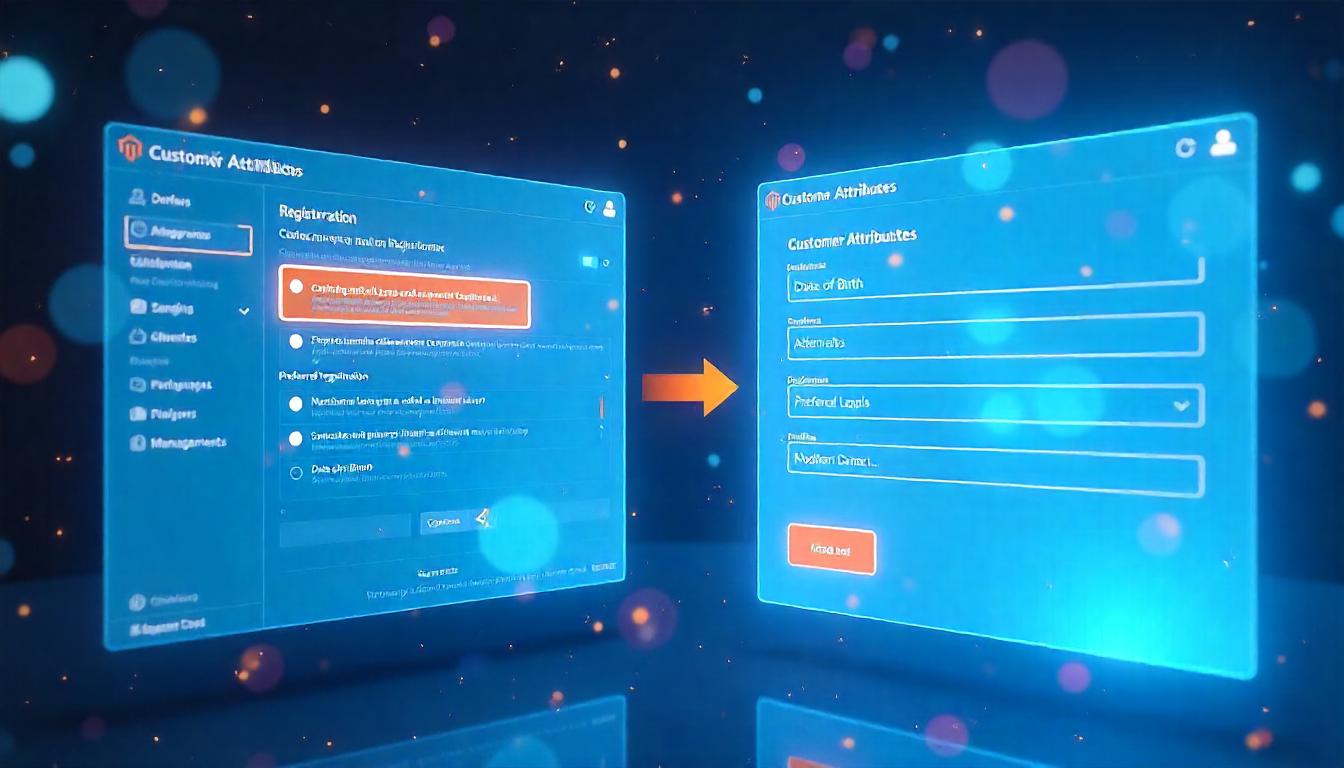
Adding Custom Customer Attributes in Magento 2: A Guide
Custom customer attributes in Magento 2 allow you to enhance customer management by collecting additional data tailored to your business needs. Here's how you can add them programmatically while adhering to Magento's coding standards.
Table Of Content
Simplify Customer Attribute Management with Magento 2 Extension
Managing customer attributes in Magento 2 doesn't have to be complicated. If creating attributes programmatically feels overwhelming, our extension offers a user-friendly alternative. This extension streamlines the process and enhances your store with customizable features, ensuring it meets your specific needs.
Key Features at a Glance
This extension supports 13 different customer attribute types, enabling you to collect and manage diverse customer data. You can easily configure fields to suit your business goals.
Feature | Description |
---|---|
Field Customization | Adjust field order, placement, and labeling to match your requirements. |
Mandatory vs. Optional | Define fields as required or optional based on your pricing or marketing needs. |
Wide Attribute Range. | Supports various field types, including dropdowns, text, dates, and more. |
Benefits for Your Store
- Improved Data Collection: Gather essential customer data to refine pricing and marketing strategies.
- Streamlined Setup: Quick and intuitive configuration without the need for programming skills.
- Enhanced User Experience: Organize and display fields in a way that suits your store’s layout.
How to Get Started
Explore all the benefits by booking a live demo. See firsthand how this extension can transform your data management process.
How to Programmatically Add Customer Attributes in Magento 2
Adding custom customer attributes in Magento 2 enhances user data collection and personalizes customer experiences. This guide outlines the steps to add attributes like text fields, dropdowns, or yes/no options using the Magento 2 framework. Here’s how you can achieve it.
Step 1: Set Up the InstallData File
The first step involves creating the InstallData.php file to define custom attributes. Here’s an example:
File Path: app/code/Company/Mymodule/Setup/InstallData.php
<?php
namespace Company\Mymodule\Setup;
use Magento\Eav\Model\Config;
use Magento\Eav\Setup\EavSetup;
use Magento\Eav\Setup\EavSetupFactory;
use Magento\Framework\Setup\InstallDataInterface;
use Magento\Framework\Setup\ModuleContextInterface;
use Magento\Framework\Setup\ModuleDataSetupInterface;
class InstallData implements InstallDataInterface
{
private $eavSetupFactory;
private $eavConfig;
public function __construct(EavSetupFactory $eavSetupFactory, Config $eavConfig)
{
$this->eavSetupFactory = $eavSetupFactory;
$this->eavConfig = $eavConfig;
}
public function install(ModuleDataSetupInterface $setup, ModuleContextInterface $context)
{
$eavSetup = $this->eavSetupFactory->create(['setup' => $setup]);
// Add custom text field
$eavSetup->addAttribute(
\Magento\Customer\Model\Customer::ENTITY,
'custom_text_field',
[
'label' => 'Middle Name',
'type' => 'varchar',
'input' => 'text',
'visible' => true,
'system' => 0,
'user_defined' => true,
'used_in_forms' => ['adminhtml_customer', 'customer_account_create', 'customer_account_edit'],
]
);
// Add dropdown field
$eavSetup->addAttribute(
\Magento\Customer\Model\Customer::ENTITY,
'custom_dropdown',
[
'label' => 'How did you hear about us?',
'type' => 'int',
'input' => 'select',
'source' => 'Company\Mymodule\Model\Source\Customdropdown',
'visible' => true,
'system' => 0,
'user_defined' => true,
'used_in_forms' => ['adminhtml_customer', 'customer_account_create', 'customer_account_edit'],
]
);
}
}
Step 2: Create a Custom Dropdown Source File
For dropdown attributes, define the selectable options in a custom source file.
File Path: app/code/Company/Mymodule/Model/Source/Customdropdown.php
<?php
namespace Company\Mymodule\Model\Source;
class Customdropdown extends AbstractSource
{
protected $_options;
public function getAllOptions()
{
if ($this->_options === null) {
$this->_options = [
['value' => '', 'label' => __('Please Select')],
['value' => '1', 'label' => __('Google')],
['value' => '2', 'label' => __('Friend')],
['value' => '3', 'label' => __('Email')],
['value' => '4', 'label' => __('Other')],
];
}
return $this->_options;
}
}
Step 3: Run Upgrade Scripts
After completing the setup, run the following commands to apply changes:
php bin/magento setup:upgrade
php bin/magento setup:static-content:deploy -f
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Are Custom Customer Attributes in Magento 2?
Custom customer attributes in Magento 2 allow you to collect and store additional information about your customers beyond the default fields. These attributes can be used for personalization, reporting, or custom business logic.
Why Would You Add Custom Customer Attributes?
Custom attributes are useful for capturing data specific to your business needs, such as preferences, demographics, or consent for marketing. They enhance customer segmentation and improve overall user experience.
How Do You Create Custom Customer Attributes Programmatically?
To create custom customer attributes programmatically, you need to define them in a custom module. This involves creating an `InstallData.php` script that uses Magento's `EavSetup` class to add attributes.
namespace Vendor\Module\Setup; use Magento\Eav\Setup\EavSetup; use Magento\Eav\Setup\EavSetupFactory; use Magento\Framework\Setup\InstallDataInterface; use Magento\Framework\Setup\ModuleContextInterface; use Magento\Framework\Setup\ModuleDataSetupInterface; class InstallData implements InstallDataInterface { private $eavSetupFactory; public function __construct(EavSetupFactory $eavSetupFactory) { $this->eavSetupFactory = $eavSetupFactory; } public function install(ModuleDataSetupInterface $setup, ModuleContextInterface $context) { $setup->startSetup(); $eavSetup = $this->eavSetupFactory->create(['setup' => $setup]); $eavSetup->addAttribute( \Magento\Customer\Model\Customer::ENTITY, 'custom_attribute_code', [ 'type' => 'varchar', 'label' => 'Custom Attribute', 'input' => 'text', 'required' => false, 'visible' => true, 'user_defined' => true, 'system' => false, ] ); $setup->endSetup(); } }
Can Custom Attributes Be Added Using the Admin Panel?
Yes, Magento 2 Enterprise Edition includes a feature for adding custom attributes through the Admin Panel. However, in the Community Edition, this feature is not available, and attributes must be added programmatically.
How Can You Display Custom Attributes in the Registration Form?
To display custom attributes on the registration form, you need to customize the layout and template files. Extend the customer registration template and include the custom attribute fields.
What Are the Risks of Adding Custom Attributes?
Improper implementation of custom attributes can lead to performance issues or conflicts with third-party extensions. Always test changes in a staging environment before applying them to production.
How Can You Debug Issues with Custom Attributes?
To debug issues, review your module code for errors, check log files, and ensure the attribute configuration matches Magento's requirements. Use the Developer Mode in Magento for more detailed error messages.
Can You Remove or Modify Custom Attributes Later?
Yes, you can remove or modify custom attributes by updating your module's `UpgradeData.php` script. Ensure that any dependent functionality is updated to reflect these changes.