Mastering the PHP 8.3 Override Attribute in Magento 2.4.7 (2025)
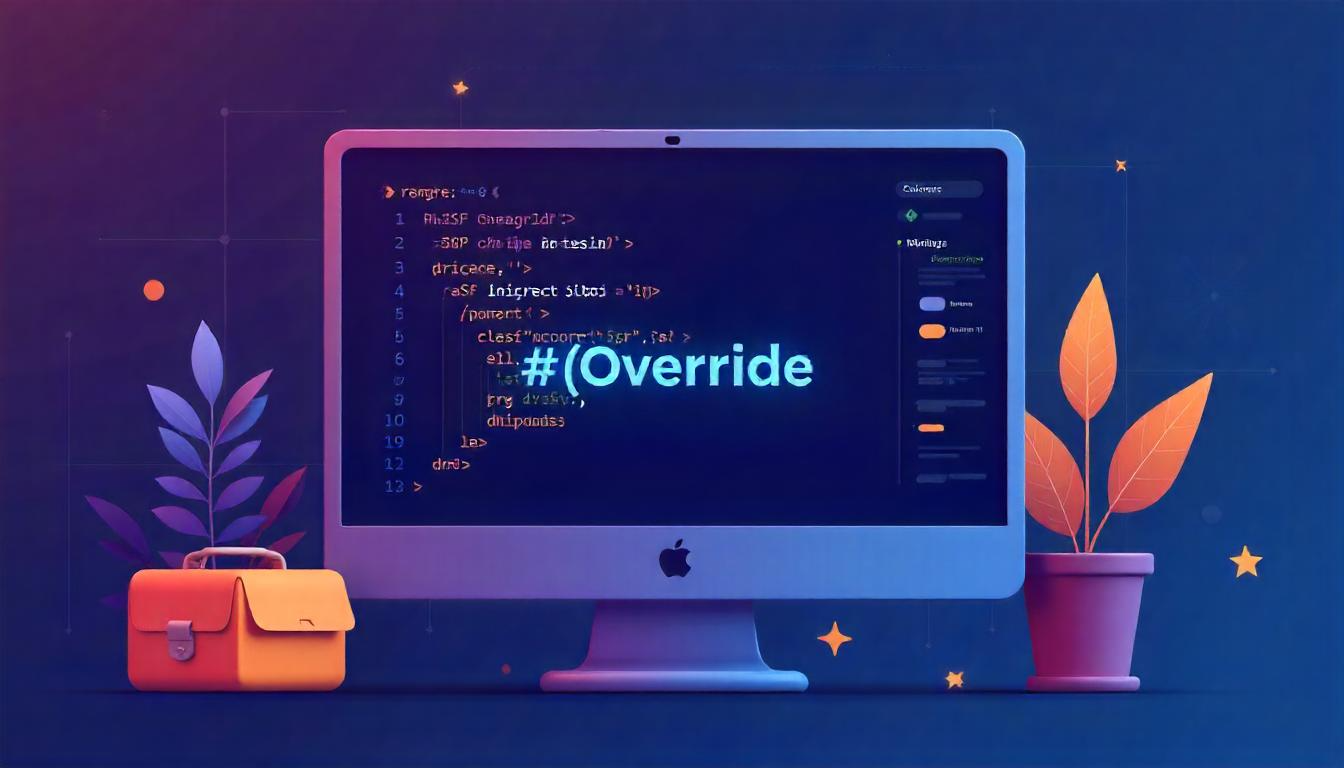
Mastering the PHP 8.3 Override Attribute in Magento 2.4.7 (2025)
Introduction: A Game-Changer for Magento Developers
As Magento development evolves with the platform's latest 2.4.7 release in 2025, we're constantly seeking tools and techniques to make our code more robust, maintainable, and future-proof. The #[\Override]
attribute introduced in PHP 8.3 represents one of the most valuable additions to our development arsenal, particularly in the complex Magento ecosystem.
This feature significantly impacts how we handle class extensions and customizations, providing guardrails that prevent silent failures when parent methods change. For Magento developers working with extensive inheritance chains and frequent upgrades, this attribute is nothing short of revolutionary.
Table Of Content
- Deep Dive into PHP Attributes in Magento 2.4.7+
- Avoiding Silent Method Override Failures in Magento with PHP Attributes
- Real-World Example of Enhanced Serialization in Magento 2.4.7
- Understanding Behavior Changes When Parent Methods Are Renamed in Magento
- Advanced Override Scenarios in Magento 2.4.7 Using PHP Attributes
- Advanced Override Patterns in Magento 2.4.7
- Advantages of Using #[\Override] in Magento 2.4.7 Development
- Integration with Magento 2.4.7's Development Tools
- Leveraging Advanced #[\Override] Usage in Magento 2.4.7+
- Conclusion
- FAQs
Deep Dive into PHP Attributes in Magento 2.4.7+
As Magento continues evolving with the latest PHP standards, PHP attributes have become a powerful and cleaner alternative to traditional annotations. They allow developers to attach structured metadata to classes, methods, properties, and parameters — all without changing runtime behavior.
What Are PHP Attributes?
PHP attributes (also called annotations or metadata tags) were introduced in PHP 8.0. They enable developers to add declarative information to code, enhancing readability, structure, and integration with frameworks or tools.
An attribute is written using #[AttributeName]
syntax and placed directly above the declaration it applies to. Unlike docblocks (which are parsed manually), attributes are first-class citizens in PHP’s syntax tree, accessible via reflection.
Example Syntax
#[MyCustomAttribute]
class MyClass {
#[AnotherAttribute("value")]
public function doSomething() {}
}
How Magento Uses PHP Attributes
Magento started using PHP attributes more extensively in versions 2.4.6 and 2.4.7, replacing some legacy annotations. These attributes help with:
- Declaring deprecation
- Configuring test fixtures
- Improving developer tooling
- Securing sensitive data
Common PHP Attributes Used in Magento
Here is a detailed table explaining commonly used PHP attributes in Magento and their purpose:
Attribute | Scope | Description |
---|---|---|
#[ReturnTypeWillChange] |
Method overrides | Prevents compatibility issues when changing return types across inheritance. |
#[DataFixture] |
Test classes | Defines classes used to load test data into the system. |
#[Deprecated] |
Classes, methods, properties | Marks an element as deprecated to trigger IDE and static analysis warnings. |
#[SensitiveParameter] |
Method parameters | Prevents sensitive parameters from appearing in error traces or logs. |
#[AllowDynamicProperties] |
Legacy classes | Permits dynamic property creation on objects, usually for legacy compatibility. |
Attribute Spotlight: #[\Override] in PHP 8.3+
What is #[\Override]
?
Introduced in PHP 8.3, the #[Override]
attribute indicates that a method is intended to override a parent method. It helps:
- Avoid accidental method mismatches
- Improve code clarity
- Assist in refactoring and static analysis
Example Usage
class Base {
public function execute() {}
}
class Child extends Base {
#[\Override]
public function execute() {}
}
If execute()
didn’t exist in the parent, PHP would throw a compile-time error.
Potential Use in Magento
Magento hasn't yet broadly adopted #[Override]
(as of 2.4.7), but future releases may include it to:
- Enforce strict inheritance patterns
- Improve plugin and override safety
- Enhance static analysis during module development
Benefits of PHP Attributes in Magento
Benefit | Description |
---|---|
Improved IDE Support | Attributes are recognized by modern IDEs, improving autocomplete and linting. |
Stronger Typing | Replaces docblock parsing with native syntax, reducing runtime errors. |
Future Compatibility | Keeps Magento aligned with PHP core evolution (e.g., PHP 8.3+). |
Cleaner Codebase | Reduces annotation noise and maintains structural clarity. |
Reflection Compatibility | Attributes integrate with PHP’s Reflection* APIs for dynamic systems. |
Comparison: Attributes vs Docblocks
Feature | PHP Attributes | PHP Docblocks |
---|---|---|
Syntax | Native to PHP 8+ | Parsed from comments |
Performance | High (built into engine) | Slower (requires manual parsing) |
IDE Support | Strong with modern tools | Varies by plugin |
Error Detection | Compile-time (some attributes) | Manual/static analysis |
Standardization | Official PHP feature | Community-convention |
Best Practices for Using Attributes in Magento
- Use attributes when supported by the PHP version and Magento core.
- Avoid mixing docblocks and attributes unless necessary for backward compatibility.
- Rely on attributes for test configuration, overrides, and deprecated flags.
- Leverage static analysis tools that support attributes (e.g., PHPStan, Psalm).
Magento’s adoption of PHP attributes is part of its modernization with PHP 8+. Attributes like #[Deprecated], #[ReturnTypeWillChange], and the emerging #[Override] help streamline development and improve long-term maintainability. As PHP evolves, Magento developers should get familiar with these tools to stay ahead.
Avoiding Silent Method Override Failures in Magento with PHP Attributes
Introduction to the Override Risk
In Magento development, it's common to extend core classes and override methods to introduce or modify functionality. While this offers flexibility, it comes with a critical risk: silent method override failures. These bugs occur when the base class method is changed or removed during an upgrade, and your overridden method no longer matches any parent method. As a result, your logic is never called, but no error is thrown — the issue goes unnoticed until something breaks in production.
Real-World Example
Imagine this scenario in a payment customization module:
- You override a method named
getTotal()
in a custom payment method. - In a Magento upgrade, the core method is renamed to
calculateTotal()
. - Your
getTotal()
method still exists in your class, but it's no longer overriding anything.
What happens now?
- Magento’s behavior changes silently.
- Your custom total logic is never executed.
- No error, no warning — just broken functionality.
These types of issues are notoriously hard to debug because they don't surface through logs or error reports. They only appear as incorrect behavior, often after the site has gone live.
The #[Override] Attribute: A Defensive Solution
The #[Override]
attribute, introduced in PHP 8.3, directly addresses this problem. It enforces strict inheritance validation, ensuring that the method you're trying to override actually exists in a parent class or interface.
Key Benefits
- Explicit Intent: Makes your override intentions clear in code.
- Compile-Time Checking: PHP will throw a fatal error during compilation if no matching parent method exists.
- Fail-Fast Principle: Encourages safe, proactive failure during development or deployment, not in production.
- Improves Maintenance: Helps teams identify overrides quickly and ensures safer code upgrades.
How #[Override] Works
The attribute is applied directly above the method definition in a class that intends to override a parent method. If the specified method doesn’t exist in the parent class or interface, PHP throws an error.
Example Syntax:
#[Override]
public function getTotal(): float {
// Your custom implementation
}
If the parent method getTotal() no longer exists due to a rename or removal, PHP will not execute this method silently. Instead, it will trigger an error and stop execution — helping you catch the issue early in your development pipeline.
Technical Comparison: Override vs. No Override
Scenario | Without #[Override] | With #[Override] |
---|---|---|
Parent method renamed | Silent failure | Compilation error |
Method typo in override | Unused method silently created | Compilation error |
Helps during refactoring | No | Yes |
Improves upgrade safety | No | Yes |
Detectable by static analyzers | Only with advanced configuration | Always detectable by PHP engine |
Supported in PHP version | Not enforced before PHP 8.3 | Native in PHP 8.3+ |
Practical Impact on Magento Development
Magento relies heavily on inheritance and customization. Upgrading to a PHP version that supports #[Override]
can provide developers with a powerful tool to:
- Prevent upgrade-induced bugs
- Improve team collaboration by clarifying override behavior
- Enforce best practices in extension development
- Reduce time spent debugging silent functional issues
The #[Override]
attribute represents a small syntax change with a large impact. For platforms like Magento where customizations are extensive and core updates are frequent, this attribute offers a crucial safeguard. Adopting it in your codebase is a proactive step toward more stable, maintainable, and upgrade-safe Magento extensions.
Real-World Example of Enhanced Serialization in Magento 2.4.7
In this section, we will walk through a practical example of how Magento 2.4.7's new PHP attributes, such as #[Override]
, can be applied to extend and enhance core functionality. Specifically, we'll take a look at how you can improve the JSON serialization and deserialization process by leveraging better error handling and additional features.
Overview of Serialization Enhancement
Serialization and deserialization are core components of many Magento systems, particularly when dealing with data storage, APIs, and caching. In Magento 2.4.7, the introduction of enhanced serialization capabilities allows developers to build more robust and error-resistant systems. By using the #[Override]
attribute, developers can ensure that method overrides are explicit, prevent potential silent failures, and benefit from improved error handling in production environments.
In this example, we'll enhance the core JSON serializer to add better exception handling, unescaped slashes, and stronger validation checks.
Enhanced JSON Serializer with#[Override]
declare(strict_types=1);
namespace Macademy\DebugBooster\Serialize\Serializer;
use Magento\Framework\Serialize\Serializer\Json as BaseJson;
class Json extends BaseJson
{
/**
* Serialize data into string with improved error handling
*
* @param mixed $data
* @return string
* @throws \InvalidArgumentException|\JsonException
*/
#[\Override]
public function serialize($data): string
{
$result = json_encode($data, JSON_THROW_ON_ERROR | JSON_UNESCAPED_SLASHES);
if (false === $result) {
throw new \InvalidArgumentException("Unable to serialize value. Error: " . json_last_error_msg());
}
return $result;
}
/**
* Unserialize the given string with better exception handling
*
* @param string $string
* @return mixed
* @throws \InvalidArgumentException
*/
#[\Override]
public function unserialize($string): mixed
{
try {
$result = json_decode($string, null, 512, JSON_THROW_ON_ERROR);
} catch (\JsonException $e) {
throw new \InvalidArgumentException("Unable to unserialize value. Error: " . $e->getMessage());
}
return $result;
}
}
Key Enhancements
Extended Magento's Core Serializer:
- We are extending Magento's core
Json
serializer class, which is responsible for converting data to and from JSON format.
Improved Error Handling:
- By utilizing
JSON_THROW_ON_ERROR
, we ensure that any errors during JSON encoding or decoding will throw exceptions, making it easier to debug and handle issues. - If serialization fails, a detailed error message is thrown, improving the developer's ability to troubleshoot issues quickly.
Unescaped Slashes:
- The
JSON_UNESCAPED_SLASHES
option is used to ensure that slashes are not escaped in the JSON output, which is useful for better readability, especially in URLs and paths.
Explicit Overriding with#[Override]
:
- By applying
#[Override]
, we explicitly declare our intent to override methods from the parent class. This helps prevent silent failures when methods are renamed, ensuring that any changes in Magento's core system will cause immediate errors if the parent method no longer exists.
Why Use #[Override] Here?
The use of the #[Override]
attribute in this example provides several critical benefits:
- Prevents Silent Failures: If Magento updates its core methods and the method signature changes (e.g., renaming
serialize
orunserialize
), PHP will throw a compile-time error if our override no longer matches the parent method. This reduces the risk of silent failures that could lead to broken functionality in production. - Code Clarity and Intent:
#[Override]
clearly indicates that a method is overriding a parent method. This improves code readability, especially when working with complex inheritance hierarchies. - Consistency Across Codebase: As
#[Override]
becomes more common in Magento development, it will help ensure that all developers follow best practices for method overriding, leading to more maintainable and consistent code.
Additional Considerations for Enhanced Serialization
While the above example focuses on error handling and unescaped slashes, serialization can be further improved by considering the following:
- Custom Data Formats: You may want to extend the serialize and unserialize methods to handle custom data types or specific structures (e.g., handling dates or objects).
- Performance Optimization: Depending on the size and complexity of the data being serialized, consider using more efficient algorithms or formats (e.g., MessagePack, BSON) for large datasets.
- Caching: Serialization is often used in conjunction with caching systems. It’s important to ensure that your custom serialization logic is compatible with caching layers in Magento.
- Security: When unserializing data, always ensure that the input data is properly sanitized to prevent potential security vulnerabilities, such as code injection attacks.
The enhanced serialization example in Magento 2.4.7, combined with the use of the #[Override]
attribute, showcases how modern PHP features can significantly improve the stability and maintainability of your Magento extensions. By enforcing explicit method overrides and improving error handling, developers can ensure that their custom logic integrates seamlessly with the Magento core system and is more resilient to changes during upgrades.
As Magento continues to evolve, adopting PHP attributes like #[Override]
will help keep your codebase up-to-date and robust, preventing issues before they occur.
Understanding Behavior Changes When Parent Methods Are Renamed in Magento
In modern Magento development, especially from version 2.4.7 onward, developers are encouraged to extend or override core functionality to suit business requirements. However, changes in the parent classes — such as renamed or removed methods — can introduce silent and hard-to-detect bugs if not handled properly.
This section focuses on a critical scenario: what happens when the method you override in a child class is no longer present in the parent class due to changes introduced in a Magento update.
Scenario: Renamed Method in the Parent Class
Let’s explore what happens when a method is renamed in a parent class. Here's an example of a revised core class in Magento:
<?php
namespace Magento\Framework\Serialize\Serializer;
class Json implements SerializerInterface
{
public function encode($data)
{
$result = json_encode($data);
if (false === $result) {
throw new \InvalidArgumentException("Unable to encode value. Error: " . json_last_error_msg());
}
return $result;
}
}
In this update, the previously available serialize()
method has been renamed to encode()
. If you have a custom module where you've overridden the now-removed serialize()
method, your child class will continue to define serialize(), but Magento will never call it — unless you’ve used the #[Override]
attribute.
What Happens Without #[Override]?
If you're not using the #[Override]
attribute and Magento upgrades the method name or signature:
- Your overridden method becomes disconnected from the inheritance chain.
- Magento will call the new method in the parent class (
encode()
in this case), not yourserialize()
override. - No error will be thrown.
- Your custom behavior silently stops working, possibly in production.
- Debugging this failure becomes extremely difficult, especially if it only affects specific edge cases.
What Happens With #[Override]
?
If you are using the #[Override] attribute and the parent method no longer exists or its signature changes, PHP throws an immediate error at compile time:
Fatal error: Macademy\DebugBooster\Serialize\Serializer\Json::serialize() has #[\Override] attribute,
but no matching parent method exists in /var/www/html/app/code/Macademy/DebugBooster/Serialize/Serializer/Json.php on line X
This error highlights exactly where the problem lies, enabling you to fix the issue before the application is deployed.
Benefits of Using #[Override] in Evolving Codebases
Scenario | Without #[Override] | With #[Override] |
---|---|---|
Parent method renamed or removed | Silent failure | Compile-time error |
Method signature changed | Possible incorrect behavior | Compile-time error |
Visibility mismatch (public/protected) | No warning | Compile-time error |
Developer is unaware of method shift | High risk | Immediate alert |
Compatibility with upgrades | Manual QA needed | Automatically flagged |
Additional Use Cases and Considerations
1. Refactoring Safeguard
When you or your team refactor core logic, #[Override]
ensures you don’t unintentionally break inherited behavior.
2. Code Review Accuracy
Code reviewers can instantly recognize the intent of an override, making reviews more precise and less error-prone.
3. Third-party Module Conflicts
In complex Magento setups, multiple extensions may override the same method. With #[Override]
, conflicting or missing methods will be flagged early.
4. Supports Forward Compatibility
As Magento evolves to support newer PHP versions and design practices, using #[Override]
future-proofs your custom modules against breaking changes.
Magento’s reliance on inheritance and method extension makes code maintenance a complex but necessary task. The #[Override]
attribute acts as a safeguard against one of the most common and costly issues in Magento upgrades — silent override failures.
By catching these issues during development, #[Override]
helps reduce production bugs, improve developer efficiency, and maintain long-term compatibility across major upgrades.
Integrating this attribute into your development workflow is not just a PHP 8.3+ feature — it's a best practice for building stable, maintainable Magento extensions.
Advanced Override Scenarios in Magento 2.4.7 Using PHP Attributes
Magento 2.4.7 introduces more refined and layered class hierarchies, alongside an evolved use of dependency injection. In this environment, the #[Override] attribute serves as a critical tool for ensuring method-level consistency, safety, and upgrade compatibility—not only in basic class overrides but also in complex architectural patterns.
Why Complex Overrides Are Risk-Prone
When working with service contracts, plugins, or proxies in Magento, method signatures can change over time—especially during major upgrades. Without strict enforcement, these changes can go unnoticed until runtime, leading to silent logic failures, broken integrations, or business rule violations.
Overriding Service Contracts and Repositories
Magento makes extensive use of repository interfaces as part of its service layer. Overriding these repositories is common for customizing business logic (e.g., validation, caching, ACL restrictions). The #[Override]
attribute can be used to prevent mismatches when API contracts change in future versions.
Custom Repository Implementation
<?php
namespace Module\Model\Repository;
use Framework\Api\ProductRepositoryInterface;
use Framework\Api\Data\ProductInterface;
class ProductRepository implements ProductRepositoryInterface
{
private ProductRepositoryInterface $originalRepository;
public function __construct(ProductRepositoryInterface $originalRepository)
{
$this->originalRepository = $originalRepository;
}
#[\Override]
public function save(ProductInterface $product, $saveOptions = false): ProductInterface
{
if ($product->getSku() === 'RESTRICTED') {
throw new \Exception('This SKU cannot be saved.');
}
return $this->originalRepository->save($product, $saveOptions);
}
}
This ensures the overridden save() method is properly aligned with the interface signature. If save()
is renamed or removed in the base interface, a compilation error will surface immediately.
Enhancing Plugin Reliability with #[Override]
Plugins in Magento allow dynamic modification of method behavior using before
, after
, and around
methods. These are especially vulnerable to method signature drift because plugins rely on string-matched method names without compile-time enforcement.
Validating Plugin Method Signature
<?php
namespace Module\Plugin;
use Framework\Model\Cart;
class CartPlugin
{
#[\Override]
public function beforeAddProduct(Cart $subject, $productInfo, $requestInfo = null)
{
return [$productInfo, $requestInfo];
}
}
By using #[Override]
, you're telling PHP that this method must match a parent or target method exactly. Any future change in the method name or argument structure will trigger a fatal error instead of silent misbehavior.
Comparison Table: Risk Mitigation in Complex Overrides
Here’s how #[Override]
improves maintainability in complex override scenarios:
Scenario | Without #[Override] | With #[Override] |
---|---|---|
Repository method renamed or removed | Custom logic is ignored | Compilation error raised |
Plugin method signature changed | Skipped or misbehaving code | Compile-time enforcement |
Interface contract change in DI system | No warnings or alerts | Immediate visibility |
High-risk business rules in override | Silent logic failure | Fail-fast behavior |
Long-term upgrade compatibility | Manual regression testing | Static analysis & compiler checks |
In highly modular platforms like Magento 2.4.7, safe customization is essential. The #[Override]
attribute introduces a more predictable and enforceable way to work with inheritance and method-level extension. Whether you’re customizing core logic via plugins or extending repository interfaces, adopting #[Override] ensures that your intentions are clearly declared and your implementation is verifiable by the PHP engine itself.
Advanced Override Patterns in Magento 2.4.7
Magento 2.4.7 introduces deeper integration with modern PHP features, and #[\Override]
has become a critical tool for ensuring code correctness, especially when working with complex service contracts and system APIs.
This section explores commonly overridden components and shows how the #[\Override]
attribute provides enhanced reliability and protection against silent failures during upgrades.
Why #[\Override] Matters in Magento
In Magento’s architecture, developers frequently override core logic across modules for customization. However, changes in method signatures or missing contract definitions can result in serious bugs if these overrides are not correctly enforced. PHP 8.3+ introduces native override checking, which Magento 2.4.7 can now leverage.
Common Override Targets in Magento 2.4.7
Here are key patterns where #[\Override]
is especially valuable in Magento 2.4.7 development:
1. Overriding GraphQL Resolvers
With GraphQL APIs becoming more robust in Magento 2.4.7, many modules implement or extend resolvers for custom fields. Ensuring the correct override of resolver interfaces is vital for query stability.
Example: Custom GraphQL Product Resolver
<?php
namespace Vendor\Module\Model\Resolver;
use Magento\Framework\GraphQl\Config\Element\Field;
use Magento\Framework\GraphQl\Query\ResolverInterface;
use Magento\Framework\GraphQl\Schema\Type\ResolveInfo;
class CustomProductResolver implements ResolverInterface
{
private ResolverInterface $originalResolver;
public function __construct(ResolverInterface $originalResolver)
{
$this->originalResolver = $originalResolver;
}
#[\Override]
public function resolve(Field $field, $context, ResolveInfo $info, array $value = null, array $args = null)
{
// Pre-resolution logic
$result = $this->originalResolver->resolve($field, $context, $info, $value, $args);
// Post-resolution logic
return $result;
}
}
Benefit: If the core resolver interface changes, your method throws a compile-time error instead of silently breaking.
2. Extending API Extension Attributes
Magento allows modules to define extension attributes on entities (e.g., Product, Customer). These attributes often require interface implementation and must be correctly overridden to function.
Example: Custom Product Extension Attribute
<?php
namespace Vendor\Module\Model;
use Magento\Catalog\Api\Data\ProductExtensionInterface;
class ProductExtension implements ProductExtensionInterface
{
#[\Override]
public function getCustomAttribute()
{
// Return custom data
}
}
Why Use #[\Override]
: Ensures that if the ProductExtensionInterface
is modified, or method signatures change, your implementation does not quietly drift into incompatibility.
3. Common Override Risks and Attribute Impact
Override Pattern | Without #[\Override] | With #[\Override] |
---|---|---|
GraphQL resolver refactoring | May fail silently | Compile-time enforcement |
Extension attribute method renamed | Logic ignored | Immediate error on deployment |
Incorrect return types | Runtime type errors | Early detection during compilation |
Invalid method visibility | Inconsistent behavior | Compile-time alert |
API schema changes | Requires manual QA | Flags override inconsistency |
Additional Advantages of Using #[\Override]
- Better Maintenance: Developers unfamiliar with a module can immediately see what’s intentionally overridden.
- Safe Upgrades: Automated tools or IDEs will catch problems earlier when Magento core methods change.
- Cleaner Integration: Promotes consistency across plugin, preference, and interface-based customizations.
When to Always Use #[\Override]
- Implementing service contracts (e.g., repositories, interfaces)
- Creating plugins (before, after, around methods)
- Rewriting framework or model classes using preference
- Extending abstract or virtual types defined by DI
Advantages of Using #[\Override] in Magento 2.4.7 Development
The #[\Override] attribute, introduced in PHP 8.3, is a major step forward in helping Magento developers write safer and more maintainable code—especially when dealing with Magento 2.4.7’s complex and layered architecture.
Why #[\Override] Matters in Magento Development
Magento heavily relies on object-oriented principles like inheritance, plugins, preferences, and service contracts. A minor change in a parent class or a refactored method signature can silently break overridden functionality. The #[\Override] attribute prevents such failures by introducing compile-time validation, improving developer confidence and long-term stability.
Benefits of #[\Override] in Magento 2.4.7
Benefit Category | Description | Magento 2.4.7 Impact |
---|---|---|
Error Detection | Immediately fails if no matching method exists in the parent | Crucial in layered service and repository inheritance |
Intent Clarity | Explicitly indicates which method is meant to override a parent method | Enhances readability and reduces misinterpretation in team workflows |
Refactoring Support | Warns if the parent method is renamed, moved, or removed | Simplifies Magento upgrade paths and reduces QA effort |
Plugin Method Safety | Prevents signature mismatches in before/after/around plugin methods | Enforces strict compatibility in plugin architecture |
Upgrade Safety | Breaks early if upstream code changes impact downstream overrides | Acts as a guardrail during minor and major Magento updates |
Maintainability | Easier to trace override relationships in large class hierarchies | Valuable for debugging and long-term code ownership |
Static Analysis Support | Allows IDEs and analyzers to catch errors before runtime | Boosts development efficiency |
Forward Compatibility | Silently ignored in PHP versions below 8.3 | Safe to introduce in modules targeting mixed PHP environments |
Best Practices: When and Where to Use #[\Override] in Magento Projects
Understanding the correct use cases ensures you maximize the benefit of the attribute while avoiding misuse.
Recommended Use Scenarios
Scenario Type | Use #[\Override]? | Reasoning |
---|---|---|
Extending a Magento class | Yes | Confirms your intention to override an existing method |
Implementing an interface | No | You are not overriding but fulfilling a contract |
Overriding a trait method | Yes | Traits may change in future Magento releases |
Plugin method interception | Yes | Ensures alignment with the core method signature |
Extending a virtual type | Yes | Virtual types often override real implementations |
Declaring preferences in di.xml | Yes | Reinforces override intention for auto-wired services |
Overriding static methods | Yes | Static method behavior follows the same inheritance rules |
Additional Guidelines for Magento Teams
- Team Policy Integration: Introduce #[\Override] as part of Magento module development guidelines.
- CI/CD Integration: Use PHP 8.3 in CI pipelines to detect override issues before deployment.
- Code Reviews: Flag missing #[\Override] in PRs where method overriding is expected.
- IDE Plugins: Encourage developers to use IDEs that support PHP 8.3 annotations for real-time feedback.
Integration with Magento 2.4.7's Development Tools
Magento 2.4.7 introduces several new and enhanced development tools that integrate seamlessly with the #[\Override]
attribute. These tools provide better validation, support for modern IDEs, and make the process of development smoother and more error-proof.
Compatibility with Magento Static Testing Framework
Magento 2.4.7's static testing framework now includes support for the #[\Override]
attribute. This ensures that your custom code adheres to Magento's standards and allows for immediate feedback during development.
<!-- In your ruleset.xml -->
<rule ref="Magento2.Classes.MethodOverrideCheck">
<properties>
<property name="requireOverrideAttribute" value="true"/>
</properties>
</rule>
This rule enforces the requirement of the #[\Override]
attribute for any method that is intended to override a parent method. This strengthens the codebase by ensuring consistent and predictable behavior across all custom modules.
IDE Integration for Magento Development
Modern integrated development environments (IDEs), like PhpStorm, offer strong support for the #[\Override]
attribute, making the development process more efficient and accurate.
- Automatic Validation: The IDE automatically validates the correctness of method overrides.
- Quick-Fix Options: Missing override attributes are highlighted, and you are provided with options to quickly add them.
- Method Navigation: Easily navigate between parent and child methods, reducing time spent searching for related code.
These IDE features significantly improve developer productivity and reduce the chances of human error during development.
Performance Considerations in Magento 2.4.7
While the use of the #[\Override]
attribute adds some overhead during compilation and development, it has no impact at runtime, which is crucial for Magento’s performance in production environments.
- Compile-time: Slight increase in compile time (negligible with OPcache enabled)
- Runtime: Zero impact, as attributes are not evaluated during execution
- Development: Positive impact with faster error detection, which leads to less time spent debugging
- Production: Zero overhead, with no performance penalty in live environments
Magento production environments typically use OPcache, meaning that the #[\Override] attribute’s impact is effectively zero in live stores.
Future-Proofing Your Magento Modules
With Magento’s rapid development cycle and frequent minor releases, using the #[\Override]
attribute is key to future-proofing your custom modules.
- Upgrades: When upgrading from Magento 2.4.6 to 2.4.7, the #[\Override] attribute helps identify compatibility issues early.
- Upcoming Releases: For future releases like 2.5.x, overridden methods will be automatically validated, making it easier to keep your codebase compatible with the latest Magento features.
- PHP Evolution: As PHP continues to evolve, your use of #[\Override] ensures your code is following the best practices of modern PHP development.
Preparing for Adobe Commerce Cloud Compatibility
Adobe Commerce Cloud environments also benefit from the use of the #[\Override] attribute, which helps ensure that custom modules work seamlessly within the cloud infrastructure.
<?php
namespace Vendor\Module\Model\Cloud;
use Magento\CloudStorage\Model\StorageInterface;
class CustomStorage implements StorageInterface
{
private StorageInterface $originalStorage;
public function __construct(StorageInterface $originalStorage)
{
$this->originalStorage = $originalStorage;
}
#[\Override]
public function storeFile(string $path, $contents): bool
{
// Add custom logging before storage
$this->logger->info("Storing file at: " . $path);
return $this->originalStorage->storeFile($path, $contents);
}
}
This example demonstrates how you can override methods in a cloud-based environment to introduce custom functionality (e.g., logging) while maintaining compatibility with the core Magento code.
Common Pitfalls and How to Avoid Them
While the #[\Override]
attribute provides significant benefits, there are some common pitfalls developers should avoid. These include:
Pitfall | Description | Solution |
---|---|---|
Forgetting Interfaces | Using #[\Override] with interface implementations | Only use #[\Override] with extended classes |
Incorrect Signatures | Method signature differs from parent method | Ensure parameter types and return types match the parent method |
Missing in Preferences | Not using #[\Override] in classes declared as preferences in di.xml | Always use the attribute to catch API changes when preferences are declared |
Plugin Method Confusion | Unsure about plugin method signatures | Use #[\Override] for before, after, or around methods to ensure signature compatibility |
Unintended New Methods | Typos in method names create new methods | Use #[\Override] to catch these mistakes at compile-time |
By following these best practices and avoiding common mistakes, developers can fully leverage the benefits of the #[\Override]
attribute in Magento 2.4.7. This ensures better code quality, reduces errors, and prepares your modules for future upgrades and cloud compatibility.
Leveraging Advanced #[\Override] Usage in Magento 2.4.7+
Overview of Parameterized Attributes in Magento
Magento 2.4.7 starts to align with modern PHP attribute capabilities, including support for future-proofing via parameterized attributes. Although #[\Override(silent: true)]
is not yet part of mainstream PHP behavior, it is a placeholder for evolving behavior in newer versions (post PHP 8.3+). This positions Magento at the forefront of best-practice adoption.
Example: Experimental Use of #[\Override(silent: true)]
<?php
namespace Vendor\Module\Controller;
use Magento\Framework\App\ActionInterface;
class CustomController implements ActionInterface
{
#[\Override(silent: true)]
public function execute()
{
// Custom execution logic
}
}
The silent:
true parameter (not currently functional) indicates how attributes could be used in the future to suppress certain behaviors or warnings. While not processed at runtime today, including such syntax signals intent and forward compatibility.
Transitioning Legacy Magento Modules to #[\Override]
If you're managing older Magento 2 codebases that were built before the attribute system was introduced, migrating safely and systematically to #[\Override]
improves maintainability, upgrade readiness, and debugging efficiency.
Migration Steps for Legacy Codebases
1. Scan for Class Inheritance
Identify all custom classes extending Magento core classes or other custom modules:
find app/code -name "*.php" -exec grep -l "extends" {} \;
2. Locate Overridden Method
Use static analysis tools (like PHPStan with Magento extensions) to identify which child classes override parent methods.
3. Incremental Attribute Adoption
Focus first on:
- Services
- Controllers
- Repositories
- Plugins with before/after/around methods
Add #[\Override]
gradually to these high-impact areas.
4. Test Thoroughly
Adding #[\Override]
can expose hidden problems such as:
- Typo-based unintended method creation
- Mismatched method signatures
- Outdated method names in inherited classes
Use integration and unit tests to validate behavior.
Migration Checklist for Legacy Codebases
Task | Description | Recommended Tools or Strategy |
---|---|---|
Identify Class Extensions | Find where core/custom classes are extended | grep, find, or IDE tools |
Detect Override Candidates | Locate overridden methods using static analysis | PHPStan with Magento plugins |
Add #[\Override] | Start with repositories, services, plugins, and critical controllers | Incremental manual refactoring |
Validate with Static Tools | Catch errors at compile time before runtime failures | PHPStan, Psalm, IDE inspections |
Perform Regression Testing | Ensure nothing breaks due to strict signature enforcement | Magento Integration Tests, PHPUnit |
Enforce via Coding Standards | Ensure team members continue to use #[\Override] | Add to ruleset.xml for Magento2 coding standards |
Developer Benefits of Migrating Legacy Code
- Improved Upgrade Safety: Future Magento updates that change parent methods will now generate compile-time failures instead of silent runtime issues.
- Better Code Readability: Maintainers can quickly identify overridden logic.
- Reduced Debugging Time: Unexpected inheritance behavior is immediately visible.
- Team Onboarding: New developers can follow inheritance chains with clarity.
- IDE Optimization: PhpStorm and other IDEs can provide better navigation, suggestions, and quick fixes.
Adopt #[\Override]
not just for compliance with Magento 2.4.7, but as part of a broader initiative toward robust, self-documenting code. Begin with high-risk overrides and gradually apply throughout your codebase. Integrate validation into your CI pipeline to make it part of your development lifecycle.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
The #[\Override]
attribute represents a significant improvement in how we maintain and extend Magento code. By explicitly marking methods that override parent class methods, we gain:
- Improved code clarity and self-documentation
- Early detection of inheritance issues
- Enhanced maintainability during Magento upgrades
- Better alignment with modern PHP development practices
As Magento continues to evolve in 2025 and beyond, adopting language features like #[\Override] positions your codebase for long-term stability and easier maintenance. The minimal overhead of adding these attributes is far outweighed by the benefits they provide in preventing subtle bugs and making your code intentions explicit.
By incorporating this attribute into your Magento development practices, particularly with version 2.4.7's complex class hierarchies, you'll create more reliable e-commerce solutions, reduce debugging time, and deliver better experiences for your clients and their customers.
FAQs
What is the #[\Override] attribute in PHP 8.3 and why is it important for Magento developers?
The #[\Override] attribute is a PHP 8.3 feature that explicitly marks methods intended to override parent class methods. It's particularly important for Magento developers because it helps prevent silent failures when parent methods change during upgrades. When a method marked with #[\Override] doesn't actually override any parent method (perhaps due to a rename in the parent class), PHP will throw an error rather than allowing the code to silently malfunction. This is crucial in Magento's complex inheritance hierarchies where method overrides are common practice and upgrades frequently involve API changes.
Does using the #[\Override] attribute affect my Magento store's performance?
The performance impact of using the #[\Override] attribute in Magento 2.4.7 is negligible. The attribute is checked at compile-time rather than runtime, so it won't slow down your live Magento store's operations. There might be a very slight increase in the time it takes for PHP to compile your code initially, but this is typically unnoticeable, especially when using OPcache in production environments (which you should be). In fact, by helping prevent bugs that could impact performance, using #[\Override] might actually improve your store's overall stability and performance in the long run.
Should I use #[\Override] when implementing interface methods in Magento?
No, you should not use the #[\Override] attribute when implementing interface methods in Magento. The #[\Override] attribute is specifically designed for methods that override parent class methods, not for methods implementing an interface. Interface implementations are not considered "overrides" in PHP's terminology—they're implementations of a contract. Using #[\Override] on interface implementations will cause PHP to throw an error since there's no parent method being overridden. Reserve #[\Override] for true parent-child class inheritance scenarios.
What happens if I use #[\Override] on a method and then a Magento update changes the parent method name?
If you use the #[\Override] attribute on a method and a subsequent Magento update changes the parent method name, PHP will throw a fatal error when trying to load your class. The error will clearly indicate that your method has the #[\Override] attribute but no matching parent method exists. This is exactly the protection the attribute provides—instead of your override silently becoming a new method that never gets called (which would happen without the attribute), you get an immediate, obvious error that alerts you to the need to update your code to match the new parent method name.
How should I integrate #[\Override] with Magento's plugin (interceptor) system?
When working with Magento's plugin system, the #[\Override] attribute can be valuable for plugin methods (before/around/after) to ensure they match the expected signatures. While plugin methods technically don't override the original methods, using #[\Override] can help catch signature mismatches early. For example, if you have a beforeAddProduct() plugin method for Magento\Checkout\Model\Cart::addProduct(), using #[\Override] ensures your plugin method signature matches what Magento expects. This can prevent subtle bugs where your plugin might not be called or might receive unexpected parameters due to signature changes in core methods during upgrades.
Is #[\Override] compatible with older PHP versions used in some Magento installations?
The #[\Override] attribute is a PHP 8.3 feature, so it's only fully functional in PHP 8.3 and later. However, if your Magento installation runs on an older PHP version, you can still include the attribute in your code as it will simply be ignored by the older PHP version rather than causing errors. This approach allows you to write forward-compatible code that will gain the benefits of #[\Override] validation once you upgrade to PHP 8.3+. Just be aware that you won't get the error-checking benefits of the attribute until you're running on PHP 8.3 or newer. Many Magento 2.4.7 installations should be running on compatible PHP versions, as Adobe has been encouraging the use of modern PHP versions.
How can I retrofit #[\Override] to existing Magento modules with many class extensions?
Retrofitting the #[\Override] attribute to existing Magento modules requires a systematic approach: First, identify all class extensions in your codebase using tools like grep or your IDE's search functionality. Next, locate all methods that override parent methods. You can use static analysis tools like PHPStan with Magento-specific extensions to help identify these. Then, add the #[\Override] attribute incrementally, starting with the most critical components like payment processors, checkout flows, and core service classes. Be sure to test thoroughly after each batch of changes, as adding the attribute might reveal unexpected inheritance issues that were previously silent. Consider automating some of this process with scripts if you have a large codebase.
Can #[\Override] help with Adobe Commerce Cloud deployments of Magento 2.4.7?
Yes, #[\Override] can be particularly valuable for Adobe Commerce Cloud deployments of Magento 2.4.7. Cloud environments often receive platform updates on a regular schedule, which can introduce changes to parent class methods that might affect your overrides. By using the #[\Override] attribute in your custom modules and extensions, you'll get immediate feedback if a cloud platform update includes API changes that break your overrides. This prevents deployment of code that would silently fail in production. Additionally, the attribute helps maintain consistency across different cloud environments (development, staging, production) by ensuring that your overridden methods always correspond to existing parent methods across all environments.
Should I use #[\Override] with Magento's preference system declared in di.xml?
Yes, using #[\Override] with Magento's preference system is highly recommended and perhaps one of the most important use cases. When you declare a class as a preference for another class or interface in di.xml, you're essentially telling Magento to use your class instead of the original one. Any methods in your preference class that override methods from the original should be marked with #[\Override]. This ensures that if the original class changes its method signatures during a Magento upgrade, you'll be alerted immediately rather than having your preference silently break. Since preferences are a core part of Magento's dependency injection system and often involve critical functionality, protecting them with #[\Override] can prevent serious issues.
What are the common mistakes when using #[\Override] in Magento development?
Common mistakes when using #[\Override] in Magento development include: Using it with interface implementations (which is incorrect, as interfaces are implemented, not overridden); forgetting to update method signatures to match parent methods exactly (parameter types, return types, and nullability must match); misspelling method names (which creates new methods instead of overrides); omitting it in preference classes (where it's particularly important); assuming trait methods always need it (depends on how the trait is used); and inconsistent application across the codebase (leading to some overrides being protected while others remain vulnerable). Another mistake is failing to update the attribute usage after refactoring code, such as when changing inheritance hierarchies or moving methods.
How does #[\Override] interact with Magento's GraphQL resolvers in version 2.4.7?
In Magento 2.4.7, GraphQL capabilities have been significantly expanded, and resolver overriding is a common customization pattern. When extending or replacing GraphQL resolvers, the #[\Override] attribute ensures that your custom resolver methods correctly match the expected signatures of the resolver interface or parent class. This is particularly important because GraphQL resolvers have specific parameter requirements (Field, context, ResolveInfo, etc.), and any mismatch can cause subtle errors in your GraphQL API. By marking your resolver methods with #[\Override], you ensure compatibility with Magento's GraphQL schema and prevent breaking changes during upgrades. This is especially valuable as Magento continues to evolve its GraphQL implementation with each release.
Can static analysis tools like PHPStan replace the need for #[\Override] in Magento projects?
While static analysis tools like PHPStan with Magento-specific rules can detect some of the same issues that #[\Override] catches, they don't completely replace the need for the attribute. Both approaches are complementary and provide different benefits. Static analysis runs during development or CI/CD processes but doesn't prevent problematic code from being deployed if analysis is skipped. The #[\Override] attribute, on the other hand, is enforced by PHP itself at runtime, providing a final safety net even in production. Additionally, #[\Override] serves as self-documenting code that clearly indicates developer intent. For optimal protection, use both: static analysis during development and the #[\Override] attribute in your code to catch any issues that might slip through analysis.
How should I handle method signature differences between Magento versions when using #[\Override]?
Handling method signature differences between Magento versions when using #[\Override] requires careful approach to backward compatibility. If you're maintaining modules that need to work across multiple Magento versions with different method signatures, consider using conditional code based on the Magento version. For example, you might need different class implementations for different Magento versions, selected at runtime via factory patterns or preference configurations specific to each version. Alternatively, you can sometimes create compatible signatures that work across versions by using union types (|) in PHP 8, nullable parameters, or default parameter values. Just remember that #[\Override] will enforce exact signature matching with the parent method in the currently loaded codebase, so your method signatures must match the specific Magento version where the code runs.
Does #[\Override] work with virtual types in Magento's dependency injection system?
The #[\Override] attribute works with classes that are used as virtual types in Magento's dependency injection system, but there are some nuances to understand. When you create a virtual type in di.xml, you're essentially creating a variant configuration of an existing class. The underlying class should use #[\Override] for any methods it overrides from its parent. However, the virtual type itself is just a configuration construct, not a separate PHP class, so the attribute isn't applied directly to virtual types. What's important is ensuring that the class being used as a virtual type properly implements all required methods with the correct signatures. Using #[\Override] in that class helps ensure it remains compatible with its parent even as Magento evolves.
What IDE support is available for working with #[\Override] in Magento 2.4.7 development?
Modern IDEs like PhpStorm (version 2023.1 and later) offer excellent support for the #[\Override] attribute in Magento 2.4.7 development. Features include: automatic validation that verifies if methods marked with #[\Override] actually override parent methods; quick-fix options to add missing #[\Override] attributes where appropriate; enhanced navigation between parent and child methods through gutter icons; inspections that detect signature mismatches between overriding and parent methods; and code generation tools that automatically add the attribute when generating overriding methods. Additionally, IDEs can be configured with Magento-specific coding standards that enforce the use of #[\Override] through inspections or formatting settings. These IDE features significantly streamline the process of working with overridden methods in Magento's complex class hierarchy.