Implementing Related Products Popup Modal in Magento 2
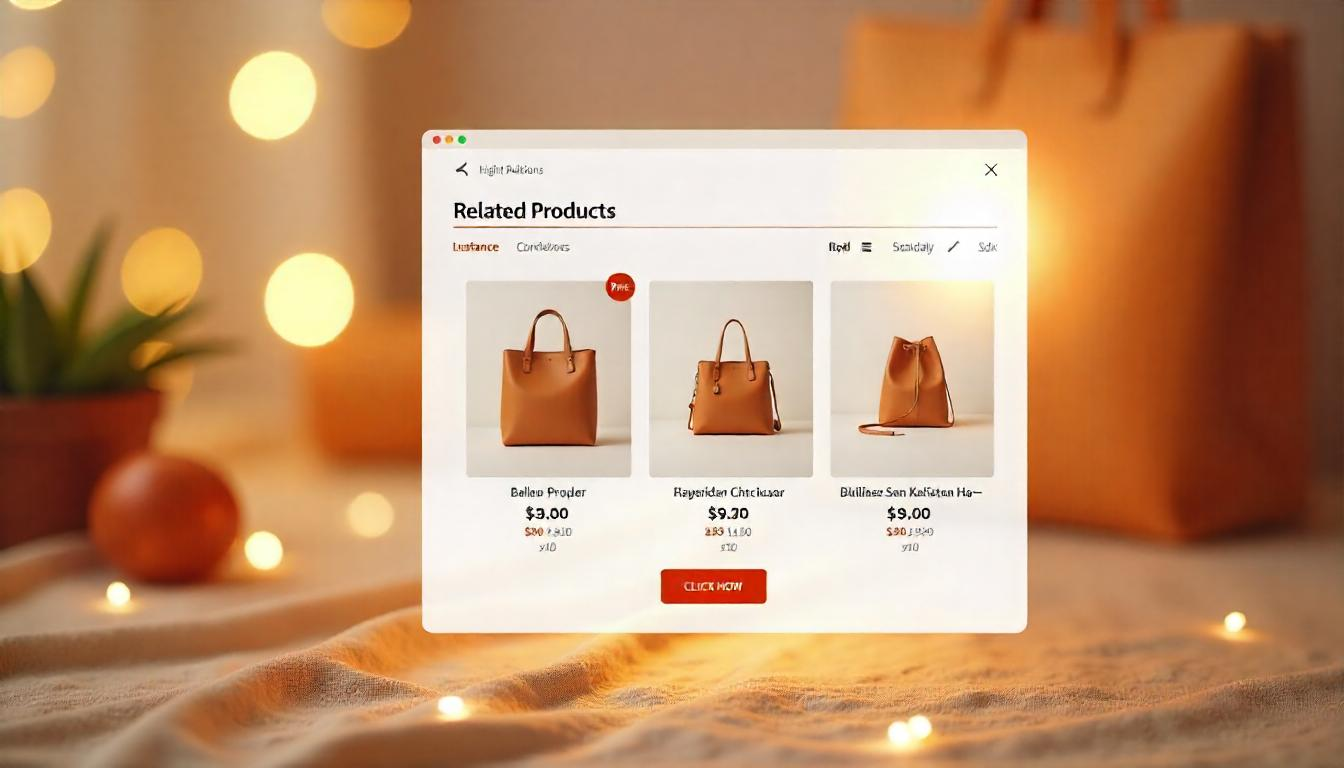
Implementing Related Products Popup Modal in Magento 2
Displaying related products in a popup modal on your Magento 2 store can enhance user engagement and potentially increase sales. This guide provides a concise, step-by-step approach to implementing this feature, ensuring clarity and adherence to best practices.
Table Of Content
Implementing Related Products Popup Modal in Magento 2
1. Create the Modal Template
Develop a new template file to define the structure of the modal.
File Path: app/code/emmo/YourModule/view/frontend/templates/modal/related_products.phtml
Sample Code:
<?php
$relatedProducts = $block->getRelatedProducts();
?>
<div id="related-products-modal" class="modal fade" tabindex="-1" role="dialog" aria-labelledby="relatedProductsModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="relatedProductsModalLabel">Related Products</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<?php if (!empty($relatedProducts)): ?>
<ul class="related-products-list">
<?php foreach ($relatedProducts as $product): ?>
<li>
<a href="<?= $product->getProductUrl() ?>">
<img src="<?= $block->getImageUrl($product) ?>" alt="<?= $product->getName() ?>" />
<span><?= $product->getName() ?></span>
</a>
</li>
<?php endforeach; ?>
</ul>
<?php else: ?>
<p>No related products found.</p>
<?php endif; ?>
</div>
</div>
</div>
</div>
2. Develop the Block Class
Create a block class to fetch related products for the current product.
File Path
: app/code/emmo/YourModule/Block/RelatedProducts.php
Sample Code:
<?php
namespace YourVendor\YourModule\Block;
use Magento\Catalog\Block\Product\AbstractProduct;
use Magento\Catalog\Model\ProductFactory;
use Magento\Framework\Registry;
use Magento\Framework\View\Element\Template\Context;
class RelatedProducts extends AbstractProduct
{
protected $productFactory;
protected $registry;
public function __construct(
Context $context,
ProductFactory $productFactory,
Registry $registry,
array $data = []
) {
$this->productFactory = $productFactory;
$this->registry = $registry;
parent::__construct($context, $data);
}
public function getRelatedProducts()
{
$product = $this->registry->registry('current_product');
if ($product) {
return $product->getRelatedProducts();
}
return [];
}
public function getImageUrl($product)
{
$imageHelper = $this->helper(\Magento\Catalog\Helper\Image::class);
return $imageHelper->init($product, 'product_base_image')->getUrl();
}
}
3. Update the Layout XML
Integrate the modal into the product page layout.
File Path: app/code/YourVendor/YourModule/view/frontend/layout/catalog_product_view.xml
Sample Code:
<?xml version="1.0"?>
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<body>
<referenceContainer name="content">
<block class="YourVendor\YourModule\Block\RelatedProducts" name="related.products.modal" template="YourVendor_YourModule::modal/related_products.phtml" />
</referenceContainer>
</body>
</page>
4. Initialize the Modal with JavaScript
Add JavaScript to handle the modal's behavior.
File Path: app/code/emmo/YourModule/view/frontend/web/js/modal.js
Sample Code:
require([
'jquery',
'Magento_Ui/js/modal/modal'
], function($, modal) {
var options = {
type: 'popup',
responsive: true,
innerScroll: true,
title: 'Related Products'
};
var popup = modal(options, $('#related-products-modal'));
$('#show-related-products').on('click', function() {
$('#related-products-modal').modal('openModal');
});
});
5. Include JavaScript in Layout
Ensure the JavaScript file is included in the layout.
Update catalog_product_view.xml
:
<referenceContainer name="head.additional">
<block class="Magento\Framework\View\Element\Template" name="related.products.modal.js" template="Magento_Theme::js/related_products_modal.phtml" />
</referenceContainer>
into this <div class="code-block"> structure using <p class="code-line">, you need to split the XML lines into individual <p> tags. Here is the corrected version:
Create related_products_modal.phtml
:
<script type="text/javascript" src="<?= $block->getViewFileUrl('YourVendor_YourModule::js/modal.js') ?>"></script>
6. Add Trigger Button
Place a button on the product page to trigger the modal.
Update catalog_product_view.phtml
:
<button id="show-related-products" type="button" class="action primary">Show Related Products</button>
7. Deploy and Clear Cache
Run the following commands to apply changes:
php bin/magento setup:upgrade
php bin/magento setup:static-content:deploy
php bin/magento cache:flush
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Summary
By following these steps, you can effectively display related products in a popup modal on your Magento 2 store, enhancing user experience and potentially increasing conversions.
FAQs
What is a Related Products popup modal in Magento 2?
A Related Products popup modal is a user interface element that displays related or complementary products in a popup window, usually triggered when a customer adds a product to the cart or views a product detail page.
Why implement a Related Products popup modal?
It boosts upselling and cross-selling opportunities by suggesting additional products without navigating away from the current page, improving user experience and increasing average order value.
How do I create a Related Products modal in Magento 2?
You can use custom JavaScript (e.g., jQuery or RequireJS), knockout templates, and layout XML to trigger a modal window that pulls related products data via AJAX when a specific event occurs.
Where do I define the related products in Magento 2?
In the Admin Panel, go to Catalog → Products, select a product, then scroll to the Related Products, Up-Sells, and Cross-Sells section to manually assign related products.
Can the modal show dynamic product data?
Yes, using AJAX calls to Magento's backend or REST APIs, you can fetch real-time related product information and display it dynamically in the popup.
Is it possible to customize the modal design?
Absolutely. The modal template and styling can be customized using `.phtml` files, CSS/LESS, and JavaScript to match your theme’s branding and layout.
Do I need a module to implement this feature?
You can build a custom module for better maintainability and separation of concerns, or directly modify theme files if the feature is simple and doesn’t require backend logic.
How do I trigger the popup modal?
You can trigger the modal on specific events like “Add to Cart” using JavaScript event listeners tied to the button’s click event or Magento’s customer-data JS modules.
Will the modal affect site performance?
If implemented efficiently using asynchronous loading (AJAX) and lightweight templates, performance impact is minimal. Preloading or caching data can further optimize performance.
Can I include 'Add to Cart' buttons in the modal?
Yes, you can include interactive elements like 'Add to Cart' or 'View Details' within the modal for a seamless shopping experience.
Is this feature mobile-friendly?
It can be mobile-friendly if designed with responsive CSS and tested across devices. Ensure the modal scales properly and is accessible via touch interactions.
Are there extensions available for this feature?
Yes, several Magento 2 extensions offer related products in modals with configurable settings. However, custom implementation gives you more control over design and functionality.