How to Generate Coupon Codes Programmatically in Magento 2
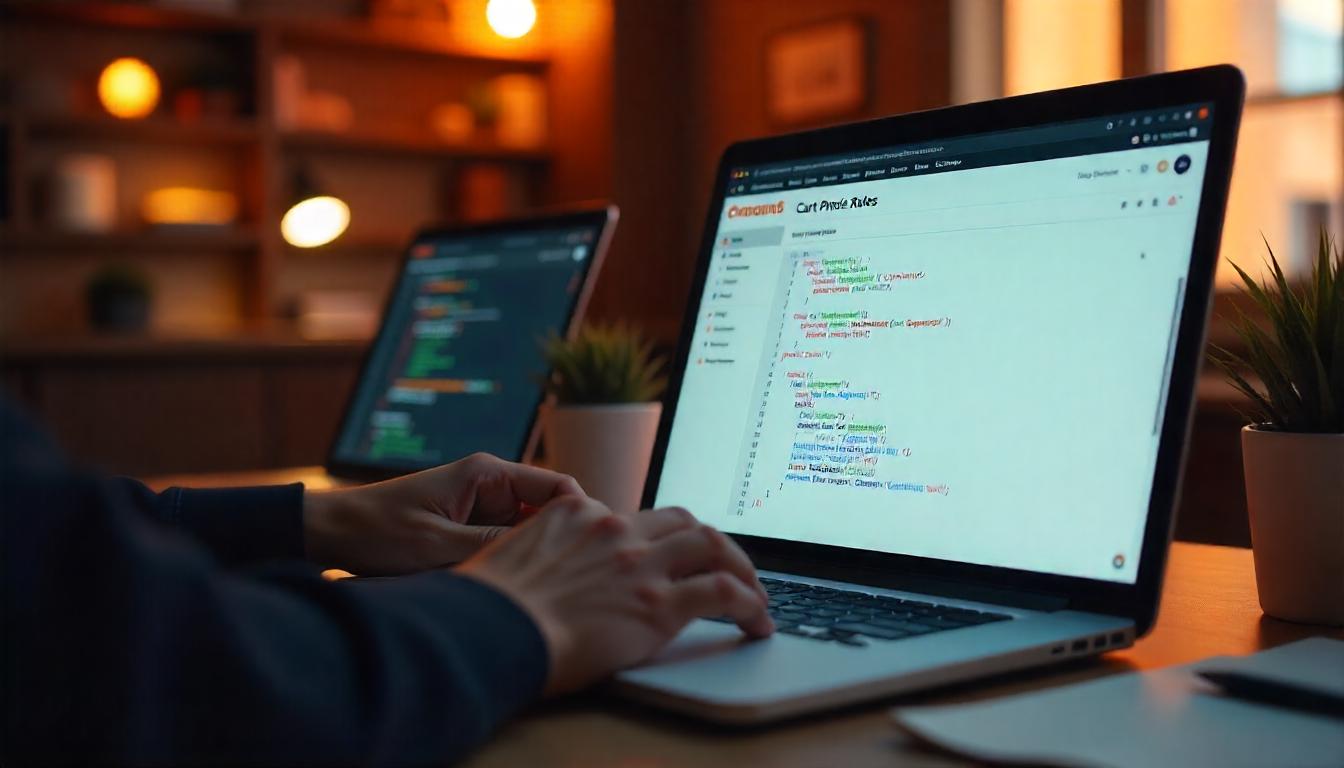
How to Generate Coupon Codes Programmatically in Magento 2
Generating coupon codes programmatically in Magento 2 allows you to automate discounts, streamline promotions, and enhance customer engagement. By creating sales rules and assigning coupon codes through code, you can efficiently manage discounts without manual setup.
Table Of Content
How to Generate Coupon Codes Programmatically in Magento 2
Creating coupon codes programmatically in Magento 2 involves setting up a sales rule and generating a corresponding coupon code. This approach allows for dynamic discount offerings without manual intervention.
Steps to Create a Coupon Code Programmatically in Magento 2:
- Initialize the Environment: Begin by setting up the Magento framework environment to access necessary classes and methods.
<?php
use Magento\Framework\App\Bootstrap;
require __DIR__ . '/app/bootstrap.php';
$params = $_SERVER;
$bootstrap = Bootstrap::create(BP, $params);
$obj = $bootstrap->getObjectManager();
$state = $obj->get('Magento\Framework\App\State');
$state->setAreaCode('adminhtml');
- Define Coupon Details: Specify the properties of the coupon, such as its name, description, discount type, and amount.
$couponData = [
'name' => 'Special Discount',
'description' => '10% off on all products',
'start_date' => date('Y-m-d'),
'end_date' => '', // Leave empty for no expiration
'max_redemptions' => 1,
'discount_type' => 'by_percent', // Options: 'by_percent', 'by_fixed', etc.
'discount_amount' => 10, // Represents 10% discount
'free_shipping' => false,
'usage_limit' => 1,
'coupon_code' => 'SAVE10' // Customize your coupon code
];
- Create the Sales Rule: Utilize Magento's SalesRule model to create a new rule with the defined properties.
$rule = $obj->create('Magento\SalesRule\Model\Rule');
$rule->setName($couponData['name'])
->setDescription($couponData['description'])
->setFromDate($couponData['start_date'])
->setToDate($couponData['end_date'])
->setUsesPerCustomer($couponData['max_redemptions'])
->setCustomerGroupIds([0, 1, 2, 3]) // Adjust based on your customer groups
->setIsActive(1)
->setSimpleAction($couponData['discount_type'])
->setDiscountAmount($couponData['discount_amount'])
->setApplyToShipping($couponData['free_shipping'])
->setTimesUsed($couponData['usage_limit'])
->setWebsiteIds([1]) // Adjust based on your website IDs
->setCouponType(2) // 2 denotes a specific coupon
->setCouponCode($couponData['coupon_code'])
->save();
After executing this script, the new coupon code will be active and available for use in your Magento 2 store. You can verify its creation by navigating to Marketing > Promotions > Cart Price Rules in the Magento admin panel.
Important Considerations
- Customization: Adjust the
$couponData
array to fit your promotional requirements, such as setting specific start and end dates or defining different discount types. - Validation: Ensure that the coupon code does not conflict with existing codes to prevent errors.
- Testing: Before deploying to a live environment, test the script in a staging setup to confirm its functionality.
By following these steps, you can efficiently create and manage coupon codes programmatically in Magento 2, enhancing your store's promotional capabilities.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Create a Coupon Code Programmatically in Magento 2?
You can generate a coupon code by first creating a sales rule using Magento\SalesRule\Api\Data\RuleInterfaceFactory
and then applying the rule ID to generate a coupon using Magento\SalesRule\Api\Data\CouponInterface
.
What PHP Code Can I Use to Create a Coupon?
Use the following PHP snippet to create a coupon:
$rule = $this->ruleFactory->create();
$rule->setName('20$ Discount')
->setDiscountAmount(20)
->setCouponType(RuleInterface::COUPON_TYPE_SPECIFIC_COUPON);
$this->ruleRepository->save($rule);
$coupon = $this->couponFactory->create();
$coupon->setCode('20FIXED')->setRuleId($rule->getRuleId());
$this->couponRepository->save($coupon);
What Parameters Are Required to Create a Coupon?
To create a coupon, you need:
$ruleId
- The ID of the sales rule.$couponCode
- The unique code customers will use.$discountAmount
- The value of the discount applied.
How Can I Handle Errors When Creating a Coupon?
Use a try-catch block to handle errors while saving the coupon:
try {
$this->couponRepository->save($coupon);
} catch (Exception $e) {
$this->logger->error($e->getMessage());
}
Where Are Coupon Codes Stored in Magento 2?
Coupons and their rules are stored in the salesrule
and salesrule_coupon
tables in the Magento database.
What Happens If the Rule ID Is Invalid?
If the rule ID does not exist, the coupon creation process will fail, and an exception will be thrown. Always verify the rule ID before assigning it to a coupon.