Adding Custom Fields to the Magento 2 Checkout Page Programmatically
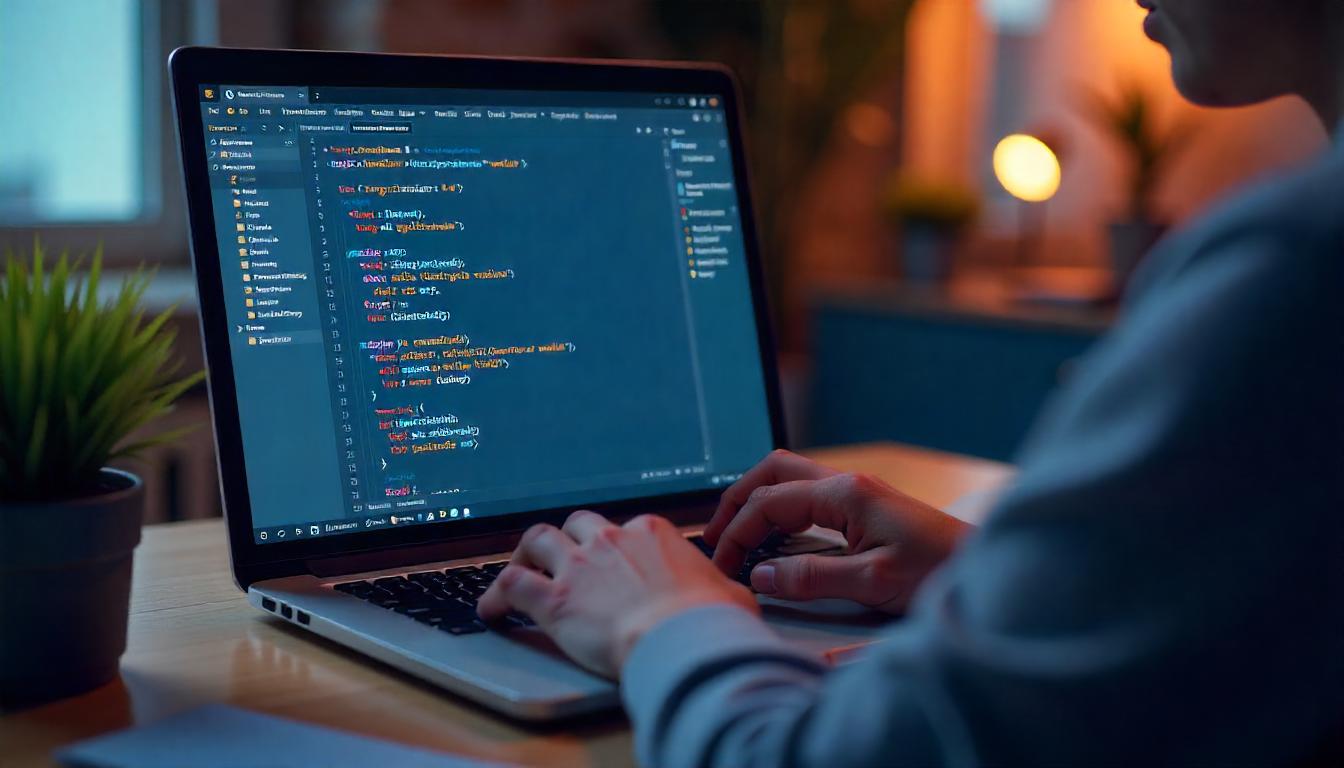
Adding Custom Fields to the Magento 2 Checkout Page Programmatically
Learn how to add custom fields to the Magento 2 checkout page programmatically with this step-by-step guide. Improve customer experience, collect valuable data, and enhance order accuracy by extending the default checkout layout using a custom module, XML layout updates, JavaScript components, and event observers—all without relying on third-party extensions.
Table Of Content
Adding Custom Fields to the Magento 2 Checkout Page Programmatically
Adding custom fields to the Magento 2 checkout page can enhance user experience and provide valuable customer insights. This guide outlines a straightforward, programmatic approach to implementing custom checkout fields in Magento 2.
Why Customize Magento 2 Checkout Fields?
A streamlined checkout process is crucial for reducing cart abandonment. By adding custom fields, you can:
- Gather additional customer information (e.g., delivery preferences, special instructions).
- Improve order fulfillment accuracy.
- Enhance marketing strategies through collected data.
Step-by-Step Guide to Adding Custom Fields Programmatically
Fully Managed Services
The platform offers a managed services infrastructure, handling routine tasks such as performance monitoring, security patches, and updates. This allows merchants to focus on core business activities without worrying about technical maintenance.
1. Create a Custom Module
Set up the module directory:
app/code/Vendor/CheckoutField
Register the module by creating registration.php:
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Vendor_CheckoutField',
__DIR__
);
Define the module in module.xml
:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Vendor_CheckoutField" setup_version="1.0.0"/>
</config>
2. Enable the Module
Run the following commands:
php bin/magento setup:upgrade
php bin/magento cache:flush
3. Add the Custom Field to the Checkout Layout
Create checkout_index_index.xml
in view/frontend/layout/
:
<?xml version="1.0"?>
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<body>
<referenceContainer name="checkout.root">
<block name="checkout.field" before="-" template="Vendor_CheckoutField::checkout/field.phtml" />
</referenceContainer>
</body>
</page>
4. Create the Custom Field Template
In view/frontend/templates/checkout/field.phtml
:
<div class="field custom-field">
<label for="custom_field">Custom Field</label>
<input type="text" name="custom_field" id="custom_field" data-bind="value: customField" />
</div>
5. Add JavaScript Component
Create custom_field.js
in view/frontend/web/js/view/
:
define([
'uiComponent',
'ko'
], function (Component, ko) {
'use strict';
return Component.extend({
defaults: {
template: 'Vendor_CheckoutField/checkout/field'
},
customField: ko.observable(''),
initialize: function () {
this._super();
}
});
});
6. Configure RequireJS
In view/frontend/requirejs-config.js
:
var config = {
config: {
mixins: {
'Magento_Checkout/js/view/shipping': {
'Vendor_CheckoutField/js/view/custom_field': true
}
}
}
};
7. Save Custom Field Value to Order Data
Create events.xml
. in etc/frontend/
:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework/Event/etc/events.xsd">
<event name="checkout_submit_all_after">
<observer name="vendor_checkoutfield_save_custom_field" instance="Vendor\CheckoutField\Observer\SaveCustomFieldObserver"/>
</event>
</config>
Then, create the observer SaveCustomFieldObserver.php
in Observer/
:
<?php
namespace Vendor\CheckoutField\Observer;
use Magento\Framework\Event\ObserverInterface;
use Magento\Framework\Event\Observer;
class SaveCustomFieldObserver implements ObserverInterface
{
public function execute(Observer $observer)
{
$order = $observer->getEvent()->getOrder();
$customFieldValue = $observer->getEvent()->getRequest()->getParam('custom_field');
if ($customFieldValue) {
$order->setData('custom_field', $customFieldValue);
$order->save();
}
}
}
8. Add Custom Field to Database Schema
Create InstallData.php
in Setup/:
<?php
namespace Vendor\CheckoutField\Setup;
use Magento\Framework\Setup\InstallDataInterface;
use Magento\Framework\Setup\ModuleContextInterface;
use Magento\Framework\Setup\SchemaSetupInterface;
use Magento\Framework\DB\Ddl\Table;
class InstallData implements InstallDataInterface
{
public function install(SchemaSetupInterface $setup, ModuleContextInterface $context)
{
$setup->startSetup();
$setup->getConnection()->addColumn(
$setup->getTable('sales_order'),
'custom_field',
[
'type' => Table::TYPE_TEXT,
'length' => 255,
'nullable' => true,
'comment' => 'Custom Field'
]
);
$setup->endSetup();
}
}
9. Finalize Setup
php bin/magento setup:upgrade
Test the module to ensure the custom field appears on the checkout page and the data is saved correctly.
Summary Table
Step | Description |
---|---|
1 | Create module directory and files |
2 | Enable the module |
3 | Add custom field to checkout layout |
4 | Create custom field template |
5 | Add JavaScript |
Adding custom fields to the Magento 2 checkout page can enhance user experience and provide valuable customer insights. This guide outlines a straightforward, programmatic approach to implementing custom checkout fields in Magento 2.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What does it mean to add custom fields to the Magento 2 checkout page programmatically?
It means modifying the checkout process using code to include additional input fields, like date pickers or text boxes, without relying on third-party modules or manual admin configuration.
Why would I need to add custom fields to checkout?
Custom fields can collect extra information from customers, such as delivery instructions, gift messages, or custom notes—helping businesses tailor the order process to their needs.
Which files are typically modified to add custom checkout fields?
You usually need to modify or extend files like `checkout_index_index.xml`, UI components (like `checkout_cart_index.xml`), `LayoutProcessor.php`, and custom JavaScript for Knockout bindings.
Can I save custom checkout field data to the order?
Yes. You can capture the custom field values and save them to the order database tables using custom plugins or observers during the checkout process.
How can I display the custom field data in the admin panel?
By extending the admin order view layout files and adding custom code, you can display the custom data on the order detail page within the Magento admin panel.
Is JavaScript needed when adding custom fields?
Yes. Magento 2 uses Knockout.js for its checkout UI, so you'll need to create or modify JavaScript files to handle the frontend behavior of custom fields properly.
What are the common mistakes when adding custom fields?
Common issues include forgetting to update the LayoutProcessor, failing to save the data to the database, or not validating input properly on both frontend and backend.
Do I need a custom module to implement this?
Yes. Creating a custom module is the best practice, as it ensures your custom checkout fields are upgrade-safe and encapsulated within reusable code.
Can I make the custom fields conditionally visible?
Yes. You can use Knockout.js bindings to control the visibility of fields based on customer selections or cart values during checkout.
How do I validate custom field input on checkout?
You can use Magento's built-in form validation rules in your UI component and JavaScript. Backend validation should also be implemented in your observer or plugin logic.
Will this work on both shipping and billing steps?
Yes. You can programmatically inject fields into either the shipping or billing step, depending on where they are relevant to your business logic.
Is it possible to show custom fields only for specific products?
Yes. You can use logic based on the cart contents to dynamically render custom fields for specific SKUs or product types.
Do I need to recompile after adding custom fields?
Yes. After adding or modifying custom checkout components, it’s recommended to clear cache, recompile DI, and deploy static content to reflect changes properly.
Is this approach upgrade-safe?
If implemented inside a custom module following Magento’s coding standards and using dependency injection and layout XML properly, then yes—it is upgrade-safe.