Magento 2 Programmatic Reorder Implementation Guide
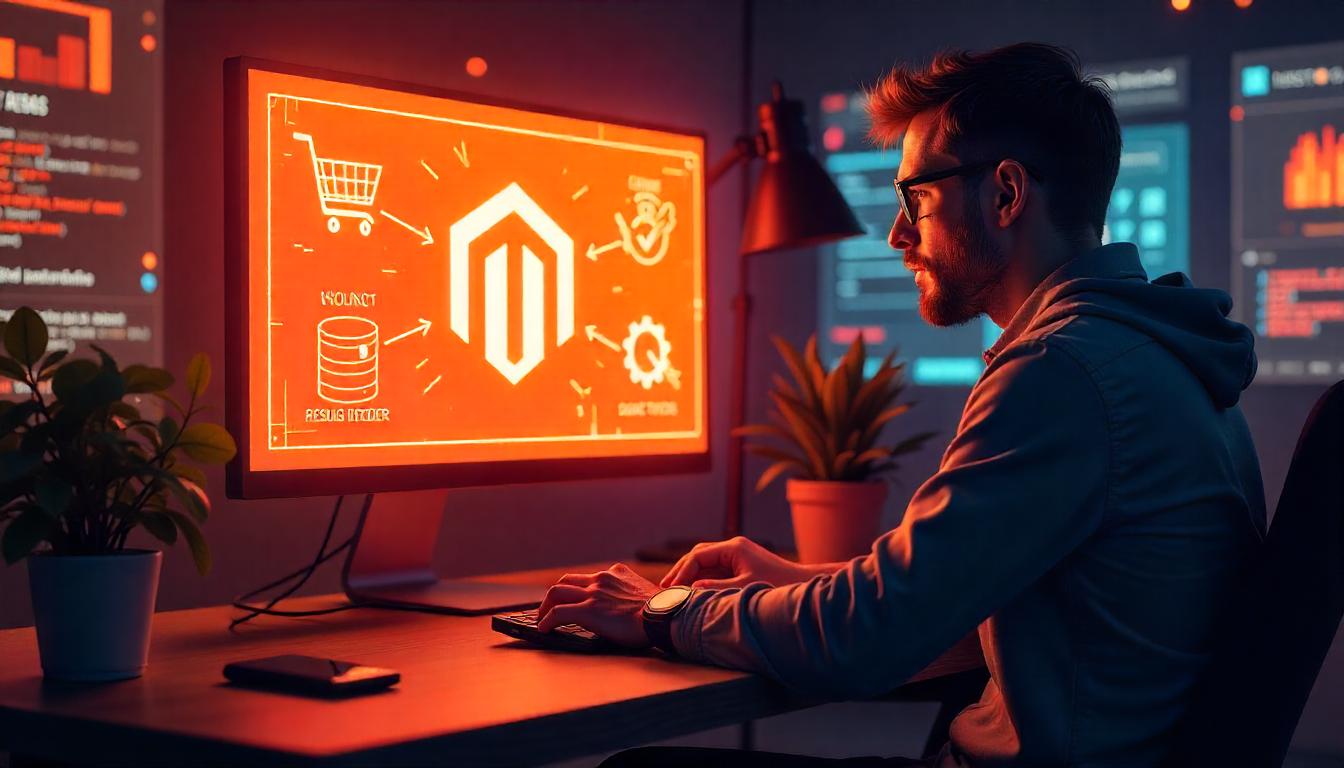
Magento 2 Programmatic Reorder Implementation Guide
Programmatic reordering in Magento 2 streamlines the checkout process by automatically duplicating previous orders. This feature recreates orders with identical products, quantities, and customer information, eliminating manual cart rebuilding.
Reorder functionality proves essential for subscription-based businesses and repeat customers. The programmatic approach bypasses standard UI workflows, enabling automated order creation through backend processes.
Table Of Content
Understanding Magento 2 Reorder Architecture (Updated 2025)
Magento 2’s reorder functionality is a powerful feature that streamlines the customer experience by allowing users to place repeat orders with minimal effort. This system is built on a modular, service-oriented architecture and involves three primary phases: order data retrieval, quote regeneration, and order conversion. Below is a detailed breakdown of how the architecture functions and the improvements made in recent versions.
1. Order Data Retrieval
The reorder process begins by accessing the customer’s historical order data stored in the sales_order table
. Magento uses the OrderRepositoryInterface
and OrderManagementInterface
to abstract database access and maintain service contracts.
Key Steps:
- The system retrieves the original order using its
increment_id
ororder_id
. - It fetches associated metadata, such as product SKUs, quantities, custom options, shipping/billing addresses, and payment information.
- If the customer is logged in, the system ensures the retrieved order belongs to their account to enforce access control.
Latest Updates (2025):
- Enhanced GraphQL support enables headless reorder workflows.
- Improved REST API endpoints allow third-party systems (e.g., CRMs, PIMs) to fetch reorder-related data securely.
2. Quote Generation (Cart Reconstruction)
Once the original order data is retrieved, Magento initiates a new quote object—a virtual shopping cart representation—populated with the order’s items and settings.
Processes Involved:
- A new quote is created via
QuoteManagementInterface
. - Each item from the original order is revalidated:
- Product existence and status (disabled or deleted products are flagged).
- Current pricing is applied to reflect catalog changes.
- Stock and inventory availability are validated using
InventorySalesApi
or MSI (Multi-Source Inventory) modules.
- Shipping and billing addresses are copied if applicable.
- Customer-specific price rules or promotional adjustments are recalculated automatically.
Support for Multi-Store & Multi-Currency:
- Magento ensures that the quote respects the current store’s currency and tax settings.
- If the original order was placed in a different store view or currency, the system uses currency converters and localization rules to align with the active storefront.
3. Order Conversion
After the quote is successfully generated and validated, Magento proceeds to convert the quote into a new order using SubmitOrderService
.
Key Actions:
- The quote is submitted using
\Magento\Quote\Model\QuoteManagement::submit()
. - A new order is created in the
sales_order
table with a new increment ID. - Order status is set according to the default workflow (e.g.,
pending
,processing
). - If the original payment method is reusable (e.g., saved credit card token or offline methods), it is reapplied. Otherwise, the system prompts for payment selection.
4. Handling Complex Reorder Scenarios
Magento 2’s reorder architecture accounts for a variety of complex business rules:
a. Product Availability & Substitution
- Discontinued or out-of-stock items are automatically skipped or replaced if rules are defined via plugins or third-party modules.
b. Dynamic Pricing & Tier Discounts
- Magento recalculates prices based on the latest tier pricing, customer group rules, catalog price rules, and cart rule configurations.
c. Custom Options & Configurables
- Reorders correctly handle configurable products, bundled items, and custom options.
- Validation ensures that required options still exist and have valid values.
d. Guest Orders Support
- As of Magento 2.4+, guest orders can be reordered if:
- The user provides a valid order ID and email address.
- The system verifies ownership using
GuestOrderRepositoryInterface
.
5. Security & Extensibility
Magento's reorder functionality prioritizes secure access and modular architecture, ensuring both protection and flexibility in custom implementations.
Key Components:
- Reorder Authorization: Magento enforces strict access control using ACL (Access Control Lists) and customer session validation to ensure only authorized users can reorder past purchases.
- Extensibility: The reorder logic is highly extensible through Magento’s architecture, including dependency injection (DI), event observers like
sales_order_reorder
, and plugins for custom logic insertion. - Logging & Auditing: Recent Magento versions include detailed logs for reorder attempts, assisting merchants in diagnosing checkout issues and inventory mismatches.
Latest Updates (2025):
- Stronger ACL rules now apply to GraphQL and REST API reorder actions.
- Enhanced audit trails provide granular tracking of reorder permissions and failures.
6. Performance Optimizations (Magento 2.4.6+)
Magento 2.4.6+ introduces significant performance enhancements to streamline the reorder process, especially under high traffic conditions.
Key Improvements:
- Asynchronous Processing: Reorders can now be queued for background processing using message queues to prevent slowdowns during peak traffic.
- Improved MSI Handling: Multi-source inventory (MSI) now supports dynamic source selection and real-time quantity checks during reorder, ensuring accuracy and fulfillment efficiency.
7. Best Practices for Developers
Area | Best Practice |
---|---|
Custom Modules | Use plugins instead of rewriting core classes. |
Reorder Button | Validate customer/session before enabling it. |
MSI Integration | Use GetSourceItemsBySkuInterface to fetch real-time inventory. |
Logging | Add custom logs for failed item re-addition. |
API Use | Use GraphQL or REST for headless reorder UX. |
Technical Implementation Strategy: Reorder Functionality in Magento 2
Implementing a robust reorder feature in Magento 2 requires a strategic approach centered on modularity, performance, and compatibility with core Magento services. The goal is to programmatically recreate a customer’s previous order into a new quote (cart), allowing them to check out again with minimal effort.
1. Core Dependencies and Classes
To support the reorder process, the following Magento 2 classes and interfaces are critical. These dependencies enable interaction with orders, customers, quotes, and store contexts.
Class / Interface | Purpose | Required |
---|---|---|
\Magento\Sales\Model\OrderFactory | Load existing order data | Yes |
\Magento\Quote\Model\QuoteFactory | Instantiate a new quote (cart) | Yes |
\Magento\Quote\Model\QuoteManagement | Submit a quote and convert it to an order | Yes |
\Magento\Quote\Api\CartRepositoryInterface | Save and retrieve cart data | Yes |
\Magento\Customer\Model\CustomerFactory | Load customer information and assign to quote | Yes |
\Magento\Store\Model\StoreManagerInterface | Retrieve store and website context | Yes |
\Magento\Catalog\Api\ProductRepositoryInterface | Load product information from SKU or ID | Yes |
\Magento\Framework\Message\ManagerInterface | Manage system messages (optional UX enhancement) | Optional |
2. Helper Class Implementation
To encapsulate reorder logic and promote reusability, create a custom Helper class. This class will be responsible for:
- Loading the original order
- Creating a new quote for the customer
- Adding the same products (with quantity, options)
- Assigning the customer (if logged in)
- Saving and returning the new cart
File Path:
Vendor\Module\Helper\Reorder.php
Sample Implementation:
namespace Vendor\Module\Helper;
use Magento\Framework\App\Helper\AbstractHelper;
use Magento\Sales\Model\OrderFactory;
use Magento\Quote\Model\QuoteFactory;
use Magento\Quote\Model\QuoteManagement;
use Magento\Quote\Api\CartRepositoryInterface;
use Magento\Customer\Model\CustomerFactory;
use Magento\Store\Model\StoreManagerInterface;
use Magento\Catalog\Api\ProductRepositoryInterface;
class Reorder extends AbstractHelper
{
protected $orderFactory;
protected $quoteFactory;
protected $quoteManagement;
protected $cartRepository;
protected $customerFactory;
protected $storeManager;
protected $productRepository;
public function __construct(
\Magento\Framework\App\Helper\Context $context,
OrderFactory $orderFactory,
QuoteFactory $quoteFactory,
QuoteManagement $quoteManagement,
CartRepositoryInterface $cartRepository,
CustomerFactory $customerFactory,
StoreManagerInterface $storeManager,
ProductRepositoryInterface $productRepository
) {
parent::__construct($context);
$this->orderFactory = $orderFactory;
$this->quoteFactory = $quoteFactory;
$this->quoteManagement = $quoteManagement;
$this->cartRepository = $cartRepository;
$this->customerFactory = $customerFactory;
$this->storeManager = $storeManager;
$this->productRepository = $productRepository;
}
public function reorder($orderId, $customerId = null)
{
$order = $this->orderFactory->create()->load($orderId);
if (!$order->getId()) {
throw new \Magento\Framework\Exception\LocalizedException(__('Order not found.'));
}
$store = $this->storeManager->getStore();
$quote = $this->quoteFactory->create()->setStore($store);
if ($customerId) {
$customer = $this->customerFactory->create()->load($customerId);
$quote->assignCustomer($customer);
}
foreach ($order->getAllVisibleItems() as $item) {
try {
$product = $this->productRepository->getById($item->getProductId());
$quote->addProduct($product, intval($item->getQtyOrdered()));
} catch (\Exception $e) {
continue; // Product no longer available
}
}
$quote->collectTotals();
$this->cartRepository->save($quote);
return $quote;
}
}
3. Reorder Trigger Logic (Controller or UI Action)
Create a controller action to handle the reorder request. The controller can:
- Accept an order ID
- Authenticate customer (optional)
- Call the Reorder helper
- Redirect to the cart or checkout page
4. Edge Case Handling
Handle special scenarios during reorder to ensure smooth user experience and data integrity:
- Product Unavailability: Skip or notify when products are out of stock or disabled.
- Custom Options: Clone custom options where possible.
- Customer Groups: Ensure pricing and tax rules are respected per group.
- Coupons & Discounts: Reapply or log discount codes separately.
- Guest Orders: Either block or create a temporary account session.
5. Future Enhancements (Optional)
- Admin Panel Reorder: Enable admins to reorder on behalf of customers directly from the backend.
- GraphQL API Support: Allow reorder functionality to be accessed via headless setups using GraphQL.
- Reorder Suggestions: Automatically display eligible past orders on the customer dashboard for quick reordering.
- Partial Reorder: Let customers select specific items from a previous order to reorder instead of the entire order.
6. Magento Version Compatibility
This implementation is compatible with Magento 2.3.x through Magento 2.4.x. However, test thoroughly in:
- Magento Open Source vs Adobe Commerce (some features like B2B may interfere)
- Multi-store and multi-currency setups
- Headless / PWA storefronts (API extension required)
Advanced Reorder Features
Reordering functionality is critical for businesses that rely on repeat purchases, such as subscription services, wholesale suppliers, and B2B companies. Modern customers expect seamless, fast, and flexible reorder experiences that reduce friction and increase retention. Advanced reorder features enable companies to maximize customer lifetime value, streamline operations, and improve satisfaction by anticipating needs and simplifying repurchasing.
Key Advanced Reorder Features
- One-Click Reordering: Instantly reorder previous purchases with a single click, skipping the need to search or customize again.
- Personalized Reorder Suggestions: Use AI-driven recommendations based on purchase history and preferences.
- Scheduled and Automated Reordering: Allow recurring reorders (weekly, monthly) with automatic order creation and notifications.
- Order History Insights: Show past orders, delivery timelines, and reorder frequency to guide decisions.
- Multi-Item Bulk Reordering: Let customers select and reorder multiple products in one action.
- Flexible Order Modification: Enable quantity, variant, or add-on adjustments during reorder without starting over.
- Mobile-Optimized Reorder: Ensure the entire reorder flow works seamlessly on mobile devices.
- Inventory & Availability Alerts: Notify users if a product is out of stock or on sale, and offer alternatives or waitlist options.
- Integration with Loyalty Programs: Tie reorders to reward points or incentives to boost repeat purchases.
Latest Trends and Data
- Subscription Economy Growth: The global subscription e-commerce market is projected to exceed $500 billion by 2028, driven by consumer demand for convenience and personalization.
- AI & Machine Learning: AI-powered reorder prediction models can boost reorder rates by up to 25%, reducing churn and improving the overall customer experience.
- Customer Expectations: A 2024 survey revealed that 78% of customers consider easy reordering a key factor in brand loyalty—highlighting the importance of seamless reorder tools.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Bulk Reorder Processing
Bulk reorder processing is an essential capability for subscription services, wholesale distributors, and businesses managing large volume or recurring orders. It enables efficient handling of multiple products and orders simultaneously, improving operational efficiency and customer satisfaction.
Implementing Bulk Reorder Capabilities for Subscription Services
- Unified Cart for Bulk Orders: Allow customers to add multiple previously purchased or subscription items to their cart in one action to speed up checkout.
- Batch Editing Tools: Let users update quantities, delivery schedules, and shipping addresses for multiple items at once.
- Automated Bulk Invoicing & Billing: Use billing systems that can handle multiple reorders at once to reduce errors and save admin time.
- API Integration: Support bulk reorder via API to sync with ERP, WMS, or 3PL systems for real-time inventory and fulfillment updates.
- Order Templates & Favorites: Let customers save templates for recurring orders to speed up repeat purchases and reduce errors.
- Scalable Backend Processing: Use cloud infrastructure and queue systems to handle high-volume reorders without affecting performance.
- Multi-Channel Support: Ensure customers can reorder in bulk through web, mobile, or phone support with a consistent experience.
Benefits of Bulk Reorder Processing
- Increased Efficiency: Customers save time placing repeat orders, boosting satisfaction and loyalty.
- Lower Operational Costs: Automation cuts down manual work and reduces errors in fulfillment and billing.
- Improved Inventory Management: Bulk orders help forecast demand more accurately, reducing out-of-stock issues.
- Enhanced Customer Retention: Convenient reorder processes keep customers coming back.
Industry Data & Best Practices
- Performance Impact: Companies using bulk reorder features report up to 40% faster processing and a 15% boost in reorder frequency.
- Cloud Scalability: Cloud-native systems can scale to handle millions of orders per month with zero downtime.
- Security & Compliance: Make sure all bulk reorder tools meet PCI-DSS and GDPR standards to keep customer data safe.
Summary
Advanced reorder and bulk reorder features are not just conveniences—they are strategic capabilities that drive customer retention, operational efficiency, and revenue growth. By investing in AI personalization, automation, scalable infrastructure, and seamless multi-channel experiences, businesses in subscription and bulk ordering industries can meet modern customer expectations and stay competitive.
FAQs
What is programmatic reorder in Magento 2?
Programmatic reorder in Magento 2 allows you to recreate a past order using code — typically by triggering a controller or helper that copies the original order’s items into the current cart for fast checkout.
Why implement bulk reorder capabilities for subscriptions?
Bulk reorder improves customer experience by allowing them to repurchase multiple items at once, especially useful for subscriptions or frequently ordered products, streamlining the checkout process.
What features improve bulk reorder functionality?
Key features include unified cart actions, batch editing tools, order templates, and API support for syncing with ERP, WMS, or 3PL systems for real-time order and inventory management.
How does API integration help with reorders?
API integration enables automated syncing with backend systems like ERP or warehouse management, making bulk reorders scalable, reliable, and accurate in real-time.
What are the performance benefits of programmatic reorder?
Businesses report up to 40% faster order processing and a 15% increase in reorder frequency, thanks to streamlined workflows and less manual input for returning customers.
How can I scale reorder functionality during peak periods?
Use cloud infrastructure with queue-based background processing to handle spikes in reorder volume, ensuring the site remains responsive and stable.
Is multi-channel support possible with bulk reorders?
Yes. You can offer consistent bulk reorder options across web, mobile apps, and call centers, ensuring seamless experiences no matter how customers place their orders.
How does programmatic reorder support customer retention?
Convenient, fast reorder options encourage repeat purchases and increase customer satisfaction, especially for B2B clients or recurring consumer needs.
What are best practices for building a reorder feature?
Include logic in controllers or UI buttons that accepts the original order ID, authenticates the customer, and programmatically adds those items to the cart using helper classes.
How can I ensure compliance and security in reorder systems?
Ensure all reorder functionality complies with PCI-DSS and GDPR by securing customer data, encrypting sensitive information, and validating all API or form inputs.