How to Truncate Strings in Magento 2: Best Practices
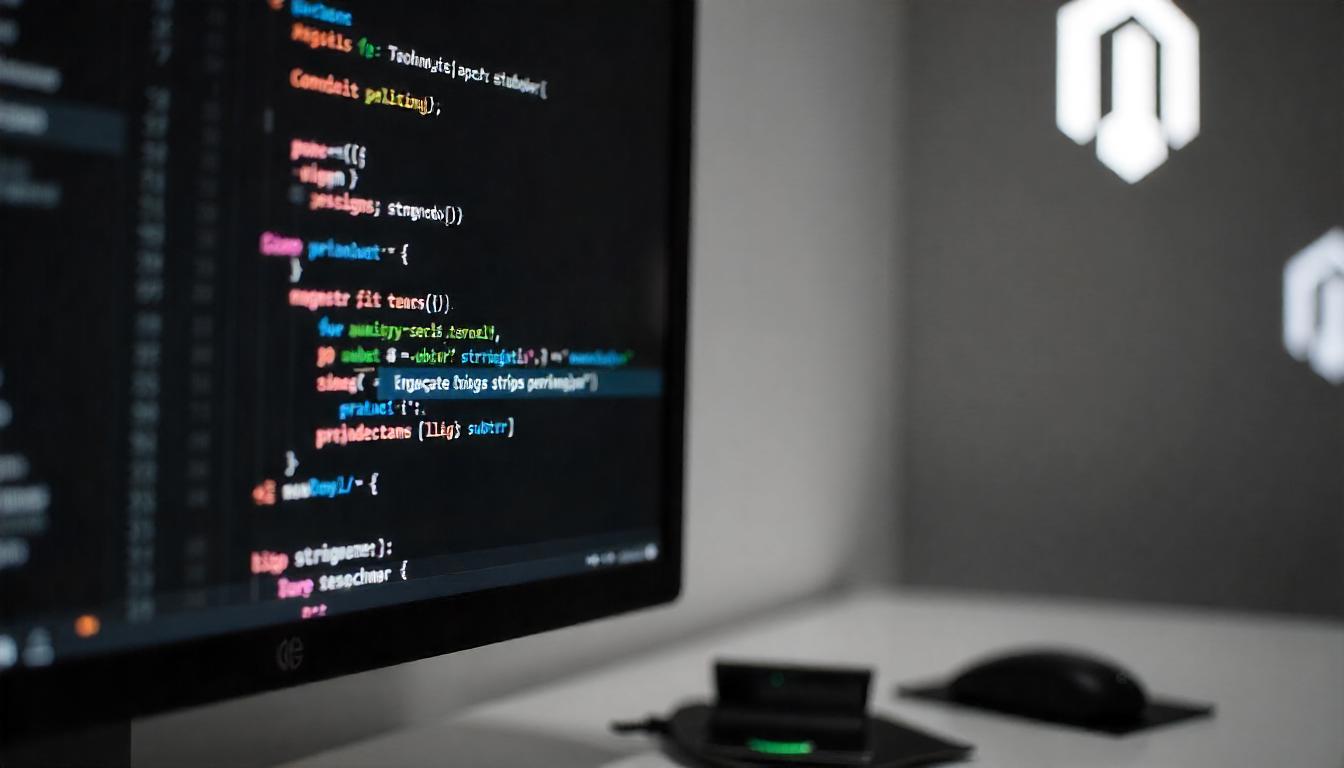
How to Truncate Strings in Magento 2: Best Practices
Learn the best way to truncate strings in Magento 2 using Magento\Framework\Stdlib\StringUtils. This guide covers safe, efficient methods that follow Magento coding standards—ideal for handling multibyte strings and avoiding common pitfalls. Includes examples and practical use cases for both PHP classes and template files.
Table Of Content
How to Truncate Strings in Magento 2: Best Practices
If you're working with Magento 2 and need to truncate strings—like product descriptions or titles—it's best to use the built-in StringUtils
class. This method ensures your code remains clean, maintainable, and aligned with Magento's standards.
Why Use StringUtils
for Truncating Strings?<
Magento provides the StringUtils
class for string manipulation tasks. Using this class is recommended over PHP's native substr()
function because:
- It integrates seamlessly with Magento's architecture.
- It handles multibyte character encodings properly.
- It maintains consistency across your codebase.
Step-by-Step Guide to Truncate a String
Inject the StringUtils
Class
In your class constructor, inject the StringUtils class:
public function __construct(
\Magento\Framework\Stdlib\StringUtils $stringUtils
) {
$this->stringUtils = $stringUtils;
}
Create a Method to Truncate the String
Define a method that uses StringUtils to truncate your string:
public function truncateString($string, $length = 50)
{
return $this->stringUtils->substr($string, 0, $length);
}
Use the Method
Call your method to get the truncated string:
$truncated = $this->truncateString("This is a long product description.", 30);
echo $truncated; // Outputs: This is a long product
Alternative: Using FilterManager
for Truncation
Magento's FilterManager
also offers a truncation method. To use it:
Inject FilterManager
public function __construct(
\Magento\Framework\Filter\FilterManager $filterManager
) {
$this->filterManager = $filterManager;
}
Truncate the String
$truncated = $this->filterManager->truncate("This is a long product description.", ['length' => 30]);
echo $truncated; // Outputs: This is a long product
When to Use Which Method
Use Case | Recommended Method |
---|---|
General string truncation | StringUtils |
Truncation with HTML handling | FilterManager |
Custom truncation logic | Custom implementation |
For most scenarios, especially when working with plain text, the StringUtils class is sufficient and aligns with Magento's best practices. Reserve FilterManager for cases where you need to handle HTML content or require additional filtering.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What is the recommended way to truncate strings in Magento 2?
Use Magento’s Magento\Framework\Stdlib\StringUtils
class. It handles multibyte characters properly and integrates with Magento’s architecture.
Can I still use PHP's substr() function?
Yes, but it’s not recommended. substr()
doesn’t support multibyte characters well. StringUtils
is safer for Magento projects.
How do I use StringUtils in my module?
Inject Magento\Framework\Stdlib\StringUtils
in your constructor and use its substr()
method to truncate strings.
What’s an example of truncating a string with StringUtils?
$this->stringUtils->substr("This is a long string", 0, 10);
returns This is a
.
Do I need to write a helper method for truncation?
It’s a good idea. Wrap the logic in a reusable method to keep your code clean and consistent across modules.
Is there another way to truncate strings in Magento?
Yes. You can also use Magento\Framework\Filter\FilterManager
and its truncate()
method, especially for HTML strings.
Which method should I use for plain text?
Use StringUtils
for plain text. It’s efficient and recommended for most truncation tasks.
When should I use FilterManager for truncation?
If you're working with HTML or need additional filtering, use FilterManager
for better control.
Does StringUtils support UTF-8 characters?
Yes. That’s one of its key advantages over plain PHP substr functions.
Why isn't my truncation working as expected?
Check if your string contains multibyte characters or if you're using PHP’s substr() directly. Switch to StringUtils
for better results.