Guide to Get Tracking details information by Shipment id Magento 2.4.x
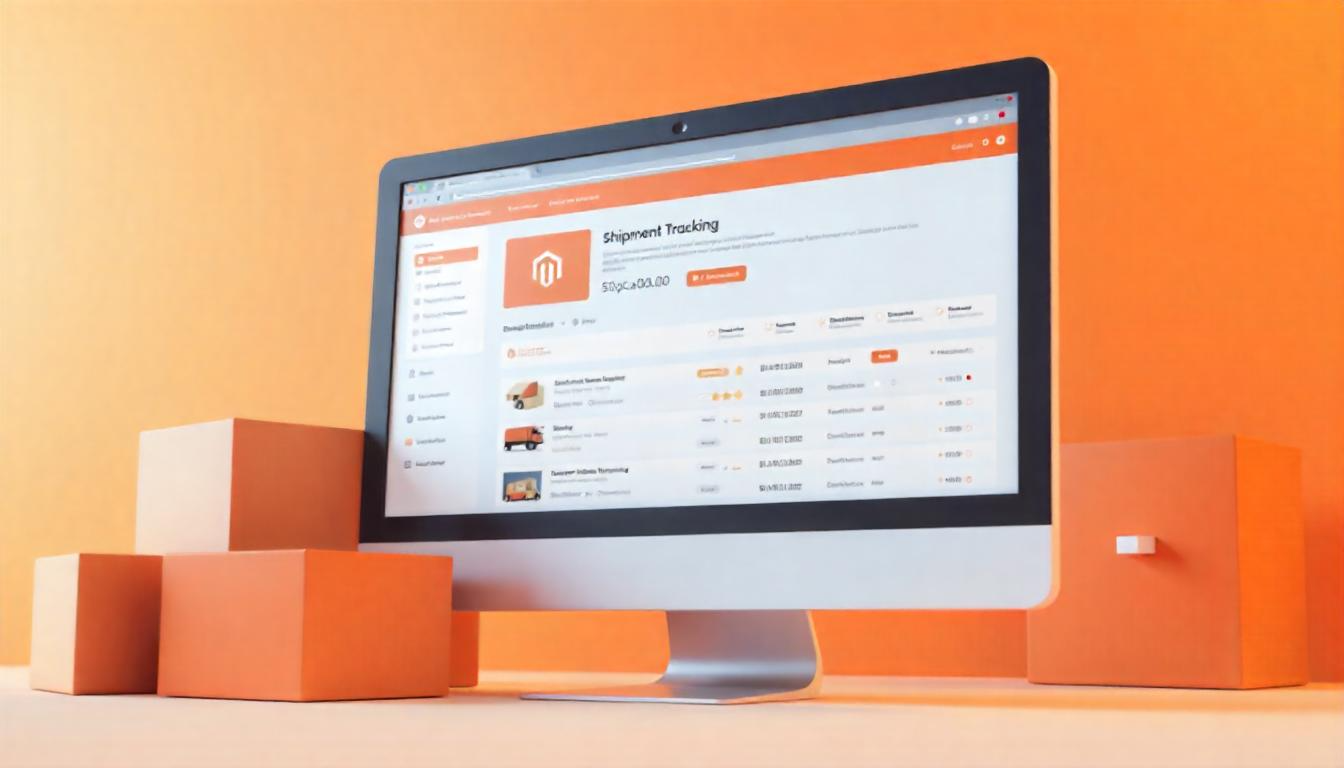
Guide to Get Tracking details information by Shipment id Magento 2.4.x
Below is a comprehensive guide for Magento 2.4.7 (2025) on fetching shipment tracking details by shipment ID using the ShipmentRepositoryInterface. It includes updated code examples, in‑depth explanations, tables, tips, and best practices.
This article covers:
- How Magento’s Sales API exposes shipments and tracks
- Retrieving a shipment object via ShipmentRepositoryInterface
- Using getTracks() and getTracksCollection() to access tracking data
- Details of the ShipmentTrackInterface methods
- Real‑world usage snippets and testing steps
- Developer tips, common pitfalls, and troubleshooting advice
By the end, you’ll have a robust, upgrade‑safe implementation to programmatically retrieve tracking numbers, carrier codes, titles, and associated order IDs—ensuring seamless integration with your custom modules or templates.
Table Of Content
Understanding Shipment and Tracking Management in Magento 2
Shipment and tracking in Magento 2 are vital parts of the order fulfillment process. Magento handles shipping data using its Sales module, allowing store owners and third-party systems to interact with shipment details programmatically.
This guide focuses on using the ShipmentTrackInterface
and ShipmentRepositoryInterface
to retrieve and manage shipment tracking information in Magento 2.4.7 and later.
Minimum Requirements to Work with Shipment Tracking Programmatically
Before starting development involving shipment and tracking data, ensure the following requirements are met.
System Requirements
Requirement | Description |
---|---|
Magento Version | Magento 2.4.7 or newer must be installed. Earlier versions may not fully support all tracking service contracts. |
Active Sales Module | The core Sales module must be enabled, as it manages all shipment and tracking entities. |
Correct Module Setup | You should work within a properly registered custom module that follows Magento’s module registration and DI conventions. |
Developer Prerequisites
You’ll need a working knowledge of the following to interact with shipment data:
Skills and Concepts
Concept | Why It’s Needed |
---|---|
PHP (Object-Oriented) | Core requirement to interact with Magento’s backend. |
Magento Service Contracts | Shipment and tracking rely on interfaces like ShipmentRepositoryInterface, which are part of Magento's service contracts. |
Dependency Injection (DI) | You must inject necessary services like shipment repositories or logger classes via constructor injection. |
Logger Usage | Logging errors using Psr\Log\LoggerInterface helps in debugging when shipment data is missing or malformed. |
Essential Classes for Shipment Track Retrieval
Here’s a breakdown of the key interfaces and classes used for working with shipment and tracking programmatically:
Class / Interface | Purpose |
---|---|
\Magento\Sales\Api\ShipmentRepositoryInterface | Fetches and manipulates shipment entities. |
\Magento\Sales\Api\Data\ShipmentTrackInterface | Represents the individual tracking record tied to a shipment. |
\Magento\Sales\Model\Order\Shipment\Track | Concrete class (though you should prefer the interface). |
\Psr\Log\LoggerInterface | Logs warnings or debug data during the retrieval or manipulation of shipment info. |
Example Use Cases
Here are practical examples of when you might interact with tracking data programmatically
- Build a custom admin grid that displays tracking numbers.
- Integrate Magento with a third-party shipping or logistics provider.
- Automatically send tracking updates via API or webhook.
- Log failed tracking data insertions for later review.
Best Practices
- Always rely on interfaces, not concrete classes, to keep your module compatible with Magento updates.
- Validate whether a shipment exists for the order before trying to access tracking data.
- Use Magento's native logging capabilities for debugging during development and production error handling.
- Do not assume a shipment has tracking — not all carriers or orders require it.
Understanding the Sales API Contracts
Magento's Sales API contracts provide standardized methods for interacting with shipment and tracking data. These contracts define the core structure for retrieving, managing, and manipulating shipments and tracking information. Understanding these contracts is crucial for developing custom solutions or integrations that involve shipments in Magento.
Key Interfaces and Their Purposes
Interface | Purpose |
---|---|
ShipmentRepositoryInterface | Provides methods to retrieve and manage shipment entities. Key methods include get($id) and getList(). These methods allow for fetching individual shipments or lists of shipments based on certain criteria. |
ShipmentInterface | Represents a shipment entity. It provides key data, including the method getTracks(), which returns the tracking information related to the shipment. This is essential for accessing tracking data linked to the shipment. |
ShipmentTrackInterface | Represents a single tracking record associated with a shipment. It provides essential methods such as: getTrackNumber(), getTitle(), getCarrierCode(), and getOrderId(), which allow you to retrieve detailed tracking information for each shipment. |
ShipmentTrackCollectionInterface | This is an extension of the ShipmentTrackInterface and provides a collection of tracking records. The getTracksCollection() method, typically used in models or observers, helps to gather multiple tracking entries for a given shipment, ensuring better data management. |
Using the getTracks() Method
The getTracks()
method, available on the ShipmentInterface
, is used to retrieve all tracking records associated with a particular shipment. This method returns an array of ShipmentTrackInterface
instances, which represent each individual tracking number and its associated data.
Example Flow
- Fetching Shipments: You can use the ShipmentRepositoryInterface to fetch a shipment based on its ID or retrieve a list of shipments based on filters (e.g., by date, order, or status).
- Retrieving Tracking Information: Once a shipment object is retrieved, you can call the getTracks() method on the ShipmentInterface to obtain an array of ShipmentTrackInterface instances. Each instance will hold the tracking number, carrier details, and other relevant tracking information.
- Handling Multiple Tracking Records: If a shipment has multiple tracking records, these will be returned as a collection through the getTracks() method, which can then be iterated over to gather information about each tracking number associated with the shipment.
Benefits of Understanding the Sales API Contracts
- Consistency in Code: By using the standard interfaces, your code adheres to Magento's best practices and is less likely to break with future updates.
- Extendability: Magento's API contracts allow you to easily extend or modify the behavior of shipment and tracking management, enabling you to integrate custom shipment carriers or additional tracking features.
- Clear Separation of Concerns: With interfaces like
ShipmentTrackInterface
andShipmentRepositoryInterface
, Magento provides a clear distinction between the data model (shipment and tracking data) and the logic for retrieving and manipulating it, allowing for easier testing and maintenance.
Additional Considerations
- Custom Implementations: If you need to extend the functionality of these contracts, you can create custom implementations of the
ShipmentTrackInterface
orShipmentRepositoryInterface
. This allows for more specialized logic, such as integrating third-party tracking services or adding custom tracking fields. - Observers and Plugins: You can use observers and plugins to add custom functionality when shipments or tracking records are updated. For example, you might trigger email notifications or update external systems whenever tracking information is updated.
Building a Shipment Tracking Retriever in Magento 2.4.7
Magento 2.4.7 uses a strong service contract structure for managing shipment and tracking entities. To retrieve shipment tracking details programmatically, you can create a typed class using ShipmentRepositoryInterface
and log any exceptions using LoggerInterface
. This modular design ensures your code remains decoupled and testable.
Prerequisites Before Implementation
Make sure the following are in place before building this class:
- Magento 2.4.7 is installed and running.
- Your module is properly registered under
app/code/Vendor/Module
. - The Sales module is active and functional.
- You understand basic PHP OOP and dependency injection.
Creating a Custom Service Class
This class provides a clean way to fetch tracking entries for a specific shipment using its ID.
namespace Vendor\Module\Model\Shipment;
use Magento\Sales\Api\ShipmentRepositoryInterface;
use Psr\Log\LoggerInterface;
use Magento\Sales\Api\Data\ShipmentTrackInterface;
class TrackingFetcher
{
private ShipmentRepositoryInterface $shipmentRepository;
private LoggerInterface $logger;
public function __construct(
ShipmentRepositoryInterface $shipmentRepository,
LoggerInterface $logger
) {
$this->shipmentRepository = $shipmentRepository;
$this->logger = $logger;
}
/** * Retrieve tracking entries for a given shipment ID. * * @param int $shipmentId * @return ShipmentTrackInterface[]|null */
public function getTrackingByShipmentId(int $shipmentId): ?array
{
try {
$shipment = $this->shipmentRepository->get($shipmentId);
return $shipment->getTracks();
} catch (\Magento\Framework\Exception\NoSuchEntityException $e) {
$this->logger->warning("Shipment ID $shipmentId not found.");
} catch (\Exception $e) {
$this->logger->error("Failed to load shipment $shipmentId: " . $e->getMessage());
}
return null;
}
}
Example Usage in a Block or Template
Here’s how you might use this class to get shipment tracking information in a controller or template file:
$shipmentId = 100001;
$tracks = $trackingFetcher->getTrackingByShipmentId($shipmentId);
if (!empty($tracks)) {
foreach ($tracks as $track) {
echo 'Tracking Number: ' . $track->getTrackNumber();
echo 'Carrier Title: ' . $track->getTitle();
echo 'Carrier Code: ' . $track->getCarrierCode();
echo 'Order ID: ' . $track->getOrderId();
}
} else {
echo 'No tracking information found for Shipment ID ' . $shipmentId;
}
Why This Approach Matters
- Service Contract Alignment: It uses Magento's ShipmentRepositoryInterface, keeping your module decoupled and upgrade-safe.
- Error Resilience: Logging catches missing shipments or general failures without halting application flow.
- Scalability: Easy to extend, allowing support for more complex logic (e.g., filtering carriers, formatting tracking data).
Key Interfaces Involved
Interface | Purpose |
---|---|
ShipmentRepositoryInterface |
Fetches shipment entities using get() and getList() methods. |
ShipmentTrackInterface |
Represents individual tracking records linked to a shipment. |
LoggerInterface |
Captures exceptions and debugging info without disrupting frontend or backend output. |
Shipment Tracking Methods and Their Responsibilities
In Magento 2.4.7 and above, the shipment tracking system is built upon a layered architecture using service contracts. These interfaces allow developers to fetch, manage, and analyze shipping data tied to customer orders in a structured and standardized way.
Below is a breakdown of important methods provided by key shipment-related interfaces and what they return.
Method | Returns | Description |
---|---|---|
ShipmentRepositoryInterface::get($id) | ShipmentInterface | Retrieves a complete shipment entity using the provided shipment ID. This is the main entry point to access shipment-related data including tracking, comments, and shipping address. |
ShipmentInterface::getTracks() | ShipmentTrackInterface[] | Returns an array of tracking records associated with the shipment. Each item in the array contains granular details about a tracking entry. |
ShipmentTrackInterface::getTrackNumber() | string | Provides the actual tracking number assigned by the shipping carrier. This can be used to check shipment status on external tracking platforms. |
ShipmentTrackInterface::getTitle() | string | Returns a human-readable name of the carrier, such as "FedEx" or "UPS." Useful for displaying user-friendly carrier names in the admin or frontend. |
ShipmentTrackInterface::getCarrierCode() | string | Returns a system-friendly string representing the carrier. Common values include fedex, dhl, or custom. This is useful for integrations or condition-based shipping logic. |
ShipmentTrackInterface::getOrderId() | int | Identifies the order to which the shipment (and track) belongs. Helpful when reverse-mapping shipment data from tracking systems. |
Additional Tips for Working with Tracking Data
- Always validate the presence of tracking records using count($shipment->getTracks()) > 0 before looping.
- Use dependency injection to retrieve instances of ShipmentRepositoryInterface rather than creating objects manually.
- Log failures gracefully using a logger implementation, especially when dealing with non-existent shipment IDs or corrupted data.
- Avoid hardcoding carrier codes, especially if working across regions or with custom shipping methods.
Testing & Verification of Shipment Tracking Logic
Thorough testing ensures that your custom shipment tracking logic is functioning as intended within your Magento 2.4.7 environment. Below is a step-by-step breakdown of testing strategies, including developer mode verification, unit testing, database validation, and API testing.
Developer Environment Setup
Before initiating tests, set your Magento environment to Developer Mode for better error visibility and log output.
bin/magento deploy:mode:set developer
This mode provides:
- Detailed error messages
- Exception stack traces
- Real-time compilation
- Log verbosity for debugging
Template Output Validation
After implementing your tracking retrieval logic in a block or template:
- Load the frontend or admin UI where the block/template is rendered.
- Inspect console logs (if you're outputting using JavaScript or UI components).
- Check
var/log/system.log
andvar/log/exception.log
for any PHP errors or warnings. - Validate that tracking details such as carrier name, tracking number, and order ID are displayed as expected.
Unit Testing Strategy
Testing should be done in isolation to validate your logic without dependencies on real database data.
Recommended Unit Testing Steps:
Component | Mocked Class | Purpose |
---|---|---|
Shipment Repository | Magento\Sales\Api\ShipmentRepositoryInterface |
Simulate return of a shipment object |
Shipment Entity | Magento\Sales\Api\Data\ShipmentInterface |
Mock getTracks() to return dummy data |
Track Record | Magento\Sales\Api\Data\ShipmentTrackInterface |
Provide fake tracking info (number, title, code, order ID) |
Logger | Psr\Log\LoggerInterface |
Ensure error logs are captured if shipment load fails |
These mocks allow simulation of various conditions: no tracks, multiple tracks, exceptions, etc.
Database Verification
You can directly validate tracking data in the database using the following table:
sales_shipment_track
Column | Description |
---|---|
entity_id | Unique ID of the tracking entry |
parent_id | Links to the shipment entity ID |
order_id | Related order |
track_number | The carrier’s tracking code |
title | Display name of the carrier |
carrier_code | System-friendly carrier code |
Use a SQL query to verify entries:
SELECT * FROM sales_shipment_track WHERE parent_id = <shipment_id>;
This ensures that the shipment you queried in code has corresponding data in the database.
Custom API Endpoint Verification (if applicable)
If you've exposed your tracking logic through a custom REST API endpoint, ensure:
- The endpoint returns valid JSON containing tracking entries.
- Proper HTTP status codes: 200 for success, 404 for not found, 500 for internal error.
- Data structure matches your defined schema or response contract.
- Use tools like Postman, cURL, or Magento Swagger UI for manual verification.
Summary Checklist
Task | Status |
---|---|
Developer mode enabled for verbose debugging | Completed |
Console and log output inspected for errors | Completed |
Unit tests created with mocks for each dependency | Completed |
Database table sales_shipment_track verified | Completed |
Custom REST endpoint behavior tested (if implemented) | Completed |
This structured and layered testing approach helps catch edge cases, ensure integration consistency, and build confidence before deploying to production.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
In conclusion, retrieving shipment tracking details by shipment ID in Magento 2.4.7 is a vital capability for enhancing customer service and operational efficiency. By utilizing the ShipmentRepositoryInterface and accessing the getTracks() method from the ShipmentInterface, developers can seamlessly fetch essential tracking information such as tracking numbers, carrier codes, and order IDs. The implementation outlined in this guide not only adheres to Magento’s best practices but also ensures that the solution is upgrade-safe and scalable.
In conclusion, retrieving shipment tracking details by shipment ID in Magento 2.4.7 is a vital capability for enhancing customer service and operational efficiency. By utilizing the ShipmentRepositoryInterface and accessing the getTracks() method from the ShipmentInterface, developers can seamlessly fetch essential tracking information such as tracking numbers, carrier codes, and order IDs. The implementation outlined in this guide not only adheres to Magento’s best practices but also ensures that the solution is upgrade-safe and scalable.
In conclusion, retrieving shipment tracking details by shipment ID in Magento 2.4.7 is a vital capability for enhancing customer service and operational efficiency. By utilizing the ShipmentRepositoryInterface and accessing the getTracks() method from the ShipmentInterface, developers can seamlessly fetch essential tracking information such as tracking numbers, carrier codes, and order IDs. The implementation outlined in this guide not only adheres to Magento’s best practices but also ensures that the solution is upgrade-safe and scalable.
FAQs
1. How can I retrieve shipment tracking information by shipment ID in Magento 2?
To retrieve shipment tracking details, you can use the `ShipmentRepositoryInterface` to get the shipment object and then use the `getTracks()` method to fetch tracking data like tracking numbers, carrier codes, titles, and order IDs.
2. What method is used to fetch shipment tracking information in Magento 2?
The `getTracks()` method of the `ShipmentInterface` is used to retrieve shipment tracking details in Magento 2.
3. How do I handle errors when fetching shipment details in Magento 2?
You can handle errors using try-catch blocks and log the exception messages using the `LoggerInterface` to ensure smooth error handling and debugging.
4. Where do I find the shipment tracking data in the shipment object?
The shipment tracking data is accessible through the `getTracks()` method of the shipment object in Magento 2.
5. How do I retrieve the carrier code for a shipment in Magento 2?
You can retrieve the carrier code of a shipment by calling the `getCarrierCode()` method on each track in the `getTracks()` collection.
6. How do I retrieve the tracking number for a shipment in Magento 2?
You can retrieve the tracking number using the `getTrackingNumber()` method on each track in the `getTracks()` collection.
7. What is the purpose of the `ShipmentRepositoryInterface` in Magento 2?
The `ShipmentRepositoryInterface` is used to retrieve shipment objects by shipment ID, enabling you to fetch the associated tracking data and other details.
8. Can I get shipment tracking details by order ID in Magento 2?
In Magento 2, shipment tracking details are accessed via the shipment ID, not directly by order ID. However, you can find the related shipment by using the order ID first and then fetch the tracking details from the shipment.
9. What information can I retrieve using shipment tracking in Magento 2?
You can retrieve the tracking number, carrier code, carrier title, and associated order ID from the shipment's tracking data in Magento 2.
10. How do I use the shipment tracking data in my custom Magento 2 module?
You can use the tracking data by calling the `getTracks()` method in your custom module’s code, and iterating over the returned collection to extract tracking numbers, carrier codes, and other details.
11. Is it necessary to use dependency injection for shipment tracking in Magento 2?
Yes, to retrieve shipment tracking details, you should inject the `ShipmentRepositoryInterface` into your class via dependency injection for better code flexibility and testability.
12. Can I add custom fields to the shipment tracking information in Magento 2?
Yes, you can extend the shipment tracking functionality by creating a custom module and modifying the tracking information using plugins or observers.
13. What is the role of the `ShipmentTrackInterface` in Magento 2?
The `ShipmentTrackInterface` provides the basic functions and methods needed to handle shipment tracking information, including methods to get the tracking number, carrier code, and title.
14. How can I debug shipment tracking issues in Magento 2?
You can debug shipment tracking issues by logging errors, verifying that the shipment ID exists, and checking if the tracking data is correctly linked to the shipment object.
15. How do I display shipment tracking information on the frontend in Magento 2?
To display shipment tracking information on the frontend, you can create a custom block and use the `getTracks()` method to retrieve and display the tracking number and other details on your template files.