How to Retrieve Custom Image-Type Attribute Values from a Product in Magento 2
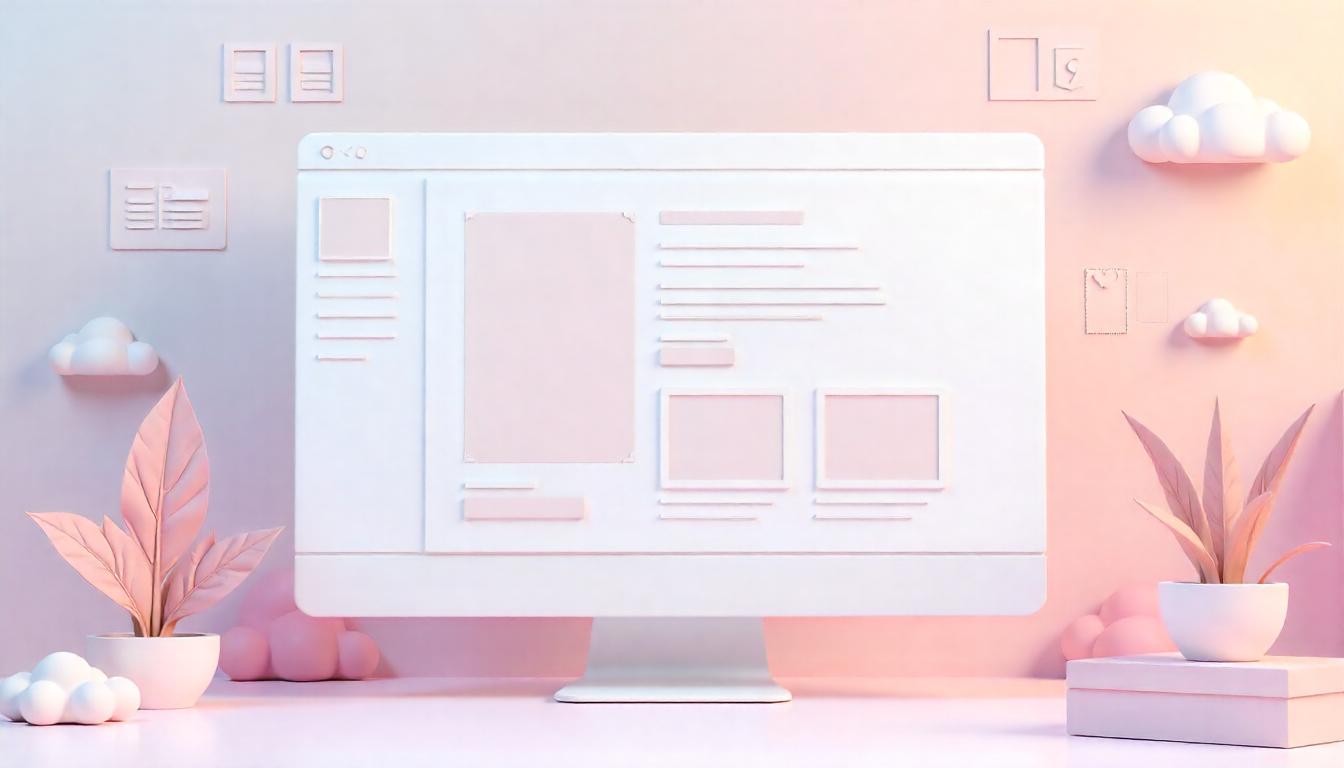
How to Retrieve Custom Image-Type Attribute Values from a Product in Magento 2
Magento 2 allows you to extend its product attributes to meet your business needs. One such extension involves custom image-type attributes, like brand_logo, which can be used for branding or additional product visuals. This guide walks you through the process of retrieving such attributes programmatically.
Table Of Content
Benefits of Custom Image-Type Attributes in Magento 2
Custom image-type attributes provide a powerful way to enhance the visual appeal of your product pages. They allow merchants to tailor the customer experience and add value beyond standard product images. Here’s why they are worth implementing:
Key Advantages
Brand Representation
- Showcase unique logos or icons to represent brands or certifications.
- Reinforce brand identity by adding visuals that align with your company’s tone and aesthetics.
Additional Product Visuals
- Add supplementary images, such as size charts, usage instructions, or ingredient lists.
- Use promotional banners or badges to highlight discounts or product features.
Enhanced User Experience
- Improve the layout and usability of your product pages.
- Add context or additional details to guide purchasing decisions effectively.
Best Practices for Custom Image-Type Attributes
- Maintain High-Quality Images: Ensure all uploaded images meet resolution standards for a professional appearance.
- Optimize Image Sizes: Compress images to reduce page load time without compromising quality.
- Keep It Relevant: Avoid clutter by only using images that add value to the customer’s buying experience.
- Test Across Devices: Verify that images display properly on desktop, tablet, and mobile views.
Custom Image-Type Attribute Use Cases
Use Case | Description | Example |
---|---|---|
Brand Logos | Show logos of associated brands directly on product pages to strengthen brand recognition. | Displaying a "Nike" logo for a sports shoe product. |
Certifications | Highlight certifications like organic, eco-friendly, or fair trade to build trust with customers. | Featuring an "Organic Certified" badge for a range of organic food products. |
Promotional Graphics | Include visual banners or icons for discounts and offers to attract buyers. | Adding a "50% Off" graphic to products in a clearance sale. |
Product Features | Visually showcase key features, such as durability, eco-friendliness, or waterproof capabilities. | Using an icon for "Waterproof" to highlight a feature of a travel backpack. |
Implementation Steps
Step 1: Create Custom Attributes
- Navigate to the Magento Admin Panel.
- Under Stores > Attributes > Product, create a new attribute with the type set to "Image."
- Configure settings like visibility and assign it to attribute sets.
Step 2: Upload Images
- Use the product edit page to upload custom images for the attribute.
- Ensure the images align with the size and style guidelines.
Step 3: Display Custom Images in the Frontend
- Modify the product page template in your theme to display the custom attributes.
- Utilize Magento’s block and layout system for seamless integration.
Tips for Successful Implementation
- Align with Branding: Ensure all custom images reflect your store’s branding guidelines.
- Use Descriptive File Names: Name files meaningfully to support SEO efforts.
- Test Across Browsers: Ensure compatibility with all major browsers to avoid rendering issues.
- Track Performance: Monitor engagement metrics like click-through rates and conversions after adding custom images.
By leveraging custom image-type attributes, you can not only create visually appealing product pages but also improve the overall shopping experience for your customers.
Steps to Retrieve a Custom Image-Type Attribute
Step 1: Create the Custom Image-Type Attribute
Custom attributes can be created programmatically or via the Magento Admin Panel.
For programmatic creation, use an UpgradeData script:
<?php
namespace Vendor\Module\Setup;
use Magento\Eav\Setup\EavSetup;
use Magento\Framework\Setup\UpgradeDataInterface;
use Magento\Framework\Setup\ModuleContextInterface;
use Magento\Framework\Setup\ModuleDataSetupInterface;
class UpgradeData implements UpgradeDataInterface
{
public function upgrade(ModuleDataSetupInterface $setup, ModuleContextInterface $context)
{
$eavSetup = new EavSetup($setup);
$eavSetup->addAttribute(
\Magento\Catalog\Model\Product::ENTITY,
'brand_logo',
[
'type' => 'varchar',
'label' => 'Brand Logo',
'input' => 'media_image',
'required' => false,
'sort_order' => 100,
'global' => \Magento\Eav\Model\Entity\Attribute\ScopedAttributeInterface::SCOPE_GLOBAL,
'visible' => true,
'user_defined' => true,
'group' => 'General',
]
);
}
}
Admin Panel Alternative:
Navigate to Stores > Attributes > Product and create a new attribute with:
- Input Type: Media Image
- Attribute Code:
brand_logo
- Assign it to an attribute set and save.
Step 2: Load the Product Object
To fetch product details, use ProductRepositoryInterface
:
$product = $this->productRepository->get('product-sku');
// Replace 'product-sku' with actual SKU.
Step 3: Fetch the Custom Attribute Value
Retrieve the value of the brand_logo
attribute:
$imagePath = $product->getData('brand_logo');
Step 4: Generate the Full Image URL
Magento stores images relative to the media/catalog/product
directory. Construct the full URL as follows:
$imageUrl = $this->_storeManager->getStore()->getBaseUrl(
\Magento\Framework\UrlInterface::URL_TYPE_MEDIA
) . 'catalog/product' . $imagePath;
Use the $imageUrl
variable to render the image on the frontend.
Common Use Cases of Custom Image-Type Attributes
Use Case | Description | Example |
---|---|---|
Brand Logo | Display logos of brands associated with products. | Nike logo for sports shoes. |
Certification Badge | Showcase certifications like organic or eco-friendly. | Organic Certified badge for food items. |
Promotional Banner | Highlight discounts or offers visually. | 50% Off icon for sale items. |
Feature Icon | Illustrate unique product features like waterproofing. | Waterproof badge for bags. |
Troubleshooting Common Issues
Issue | Cause | Solution |
---|---|---|
Attribute Value is Null | The attribute is not assigned to the product. | Ensure the attribute is assigned via the admin panel or programmatically. |
Image Not Displayed | Image file is missing or path is incorrect. | Check the `media/catalog/product` directory and re-upload the image. |
Incorrect Image URL | Base media URL configuration is incorrect. | Verify the media base URL settings in the admin panel. |
Best Practices
Optimize Images
Use lightweight image formats like WebP for faster loading times.
Attribute Organization
Group attributes into logical attribute sets to streamline catalog management.
Reindexing
Regularly reindex Magento to ensure changes are reflected.
Testing
Test the custom attribute functionality in a staging environment before deploying live.
Cache Management
Clear the cache after updating attributes or templates.
Advanced Guide: Fetching Custom Attributes in GraphQL
GraphQL provides a streamlined way to fetch custom attributes in Magento 2. Using GraphQL queries ensures efficient data retrieval while adhering to modern API standards.
Benefits of Using GraphQL for Custom Attributes
Efficiency:Fetch only the required fields to optimize performance.
Flexibility: Query multiple attributes and relationships in a single request.
Modern API Design: Works seamlessly with frontend frameworks like React and Vue.js.
Real-Time Updates: Ideal for dynamic UIs and real-time data requirements.
Example Query to Fetch Custom Attributes
To retrieve a custom attribute like brand_logo
for a specific product, you can use the following GraphQL query:
{
products(filter: { sku: { eq: "product-sku" } }) {
items {
sku
name
brand_logo
}
}
}
Steps to Implement
1. Enable GraphQL in Magento
Ensure your Magento instance is GraphQL-enabled. Test your GraphQL endpoint by navigating to: https://your-magento-store.com/graphql
2. Test the Query in GraphQL Playground
Use tools like GraphiQL or Postman to execute and test the query.
3. Retrieve the Attribute
Replace product-sku
with the actual SKU of the product whose attributes you wish to fetch.
Error Handling in GraphQL Queries
Error Code | Description | Resolution |
---|---|---|
400 Bad Request | The query contains an invalid filter or syntax. | Review the query's structure and parameters for correctness. |
404 Not Found | The specified SKU does not exist in the product catalog. | Ensure the SKU is accurate and exists in the catalog. |
500 Internal Error | Server-side problem or misconfiguration of attributes. | Examine server logs to identify and fix the issue. |
Comparison of REST API vs GraphQL for Attribute Retrieval
Aspect | REST API | GraphQL |
---|---|---|
Data Fetching | Fetches predefined data sets. | Fetches specific fields as needed, minimizing over-fetching. |
Performance | May return more data than required, leading to inefficiencies. | Optimized for lightweight and precise responses. |
Ease of Use | Requires multiple endpoints to retrieve different data. | Provides a single endpoint to handle all queries efficiently. |
Use Cases | Ideal for integrating with legacy systems and simple requirements. | Highly suited for modern frontend frameworks like React or Vue.js. |
Enhancing GraphQL for Custom Attributes
- Add Custom Resolver: Create a custom GraphQL resolver to support additional logic or validations for your attributes.
- Authentication and Authorization: Secure queries by ensuring proper access controls.
- Batch Requests: Use GraphQL batching to minimize API calls when fetching attributes for multiple products.
Real-World Use Cases for Custom Attributes in GraphQL
Use Case | Description | GraphQL Query Example |
---|---|---|
Fetching Brand Logos | Retrieve brand logos for all products in a category. |
{ products(filter: { category_id: { eq: "12" } }) { items { sku brand_logo } } }
|
Getting Stock Information | Include stock status along with custom attributes. |
{ products(filter: { sku: { eq: "product-sku" } }) { items { sku stock_status } } }
|
Personalized Recommendations | Query custom attributes for displaying tailored product badges. |
{ products(filter: { sku: { eq: "sku-list" } }) { items { name badge_attribute } } }
|
By leveraging GraphQL for custom attributes in Magento 2, you can enhance performance, flexibility, and user experience on your platform.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Incorporating custom attributes into Magento 2 opens a world of possibilities for enhancing product presentation, personalizing user experiences, and streamlining backend operations. Whether you're fetching attributes like brand logos via REST API or GraphQL, creating them programmatically, or using advanced techniques for data retrieval, understanding the nuances of these processes is crucial.
GraphQL shines with its flexibility, lightweight responses, and modern capabilities, making it a preferred choice for developers seeking efficiency and precision. REST API, on the other hand, remains a reliable tool for legacy systems and broader integrations.
By leveraging these technologies effectively, businesses can deliver tailored, visually appealing, and optimized experiences to their customers. The ability to combine attributes with stock information, implement personalized recommendations, and showcase brand certifications not only elevates the shopping journey but also enhances SEO performance and brand credibility.
For developers and eCommerce managers alike, mastering these tools and strategies ensures a competitive edge in today's dynamic digital landscape. Dive into these practices, adapt them to your specific use cases, and unlock the full potential of your Magento store!
FAQs
What are custom image-type attributes in Magento 2?
Custom image-type attributes are additional product attributes used to display specific images, such as brand logos or promotional graphics, on product pages.
How do I create a custom image-type attribute programmatically?
You can create a custom image-type attribute using an UpgradeData script. The attribute is added to the product entity and configured for media image input.
How can I retrieve the value of a custom attribute?
To retrieve a custom attribute value, load the product object using the ProductRepositoryInterface and call the getData()
method with the attribute's code.
How do I display a custom attribute image on the frontend?
Generate the full image URL by appending the attribute's relative path to the base media URL using the _storeManager
.
What is the difference between REST API and GraphQL for attribute retrieval?
REST API fetches predefined data sets and may require multiple endpoints, while GraphQL allows for precise, lightweight queries using a single endpoint.
What are the benefits of using GraphQL for fetching custom attributes?
GraphQL enables fetching only the required fields, reducing response size and improving performance, especially for modern frontend frameworks.
Can I fetch stock status along with custom attributes in GraphQL?
Yes, you can include stock status in a GraphQL query by specifying the required fields in the query.
How can I fetch custom attributes for products in a specific category?
Use a GraphQL query with a category filter to fetch products and their custom attributes from a particular category.
What are some use cases for custom attributes in Magento 2?
Common use cases include displaying brand logos, product certifications, promotional graphics, and highlighting product features visually.
What are common errors when fetching attributes via GraphQL?
Common errors include invalid query syntax (400 Bad Request), non-existent SKUs (404 Not Found), and server-side issues (500 Internal Error).
How do I resolve a 400 Bad Request error in GraphQL?
Check the query structure and ensure the filters and parameters are correct.
What makes GraphQL better suited for modern frontend frameworks?
GraphQL's ability to fetch only required data using a single endpoint makes it more efficient and tailored for modern frontend applications.
Why is it important to create and use custom attributes in Magento 2?
Custom attributes allow for enhanced product presentation, improved user experience, and personalized marketing, which can boost engagement and conversions.