How to Assign a Product Attribute to an Attribute Set in Magento 2.4.7
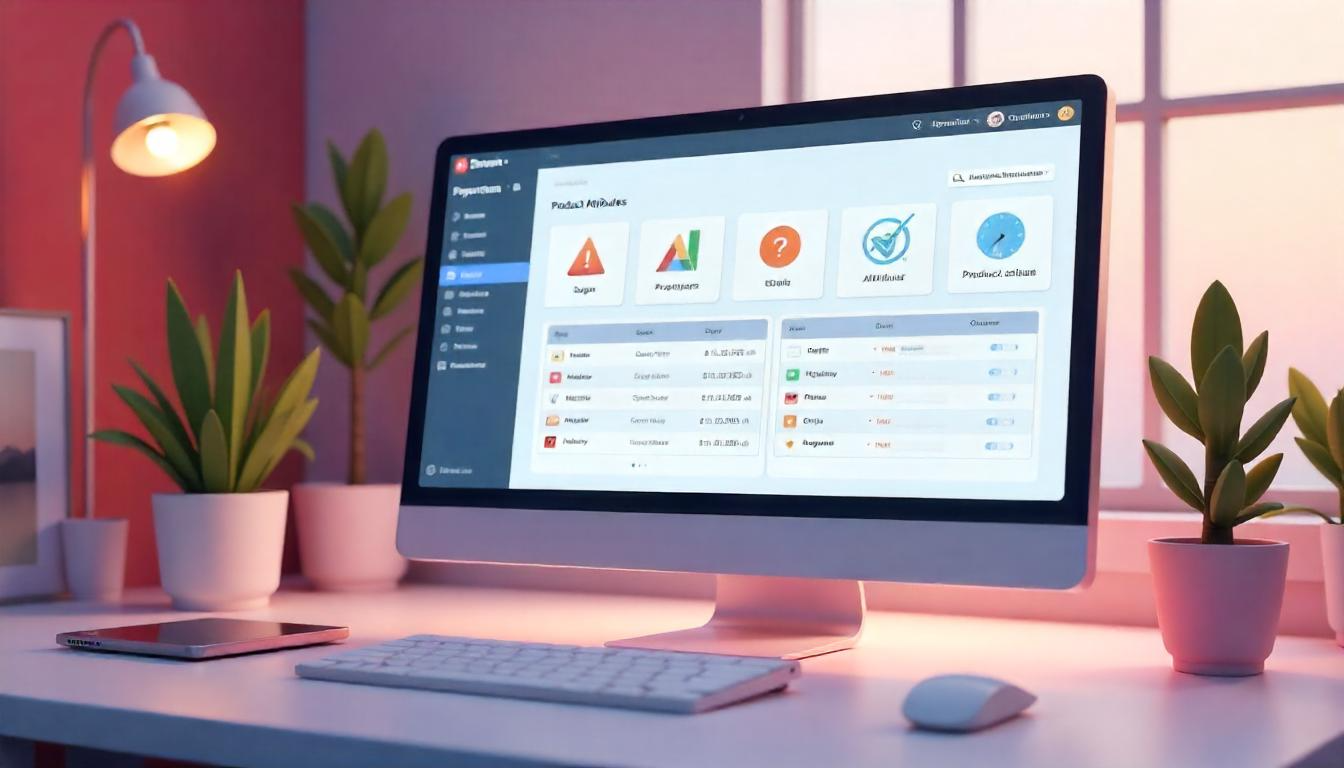
How to Assign a Product Attribute to an Attribute Set in Magento 2.4.7
Assigning attributes to attribute sets in Magento 2 ensures they appear in the product admin page under the appropriate attribute groups. This can be done manually or programmatically. This guide covers both methods and best practices for Magento 2.4.7 (2025).
Table Of Content
Assigning Attributes Manually via the Magento 2 Admin Panel
Properly assigning attributes is essential for product data management. Follow these steps to manually assign attributes to an attribute set in Magento 2.
Steps to Assign Attributes Manually
Log in to Magento Admin Panel
- Access your store's backend by navigating to your Magento Admin panel.
Navigate to Attribute Sets
- Go to Stores → Attributes → Attribute Set.
Select the Desired Attribute Set
- Locate the attribute set you want to modify and click on it.
Assign Attributes to the Attribute Set
- Drag and drop attributes from the Unassigned Attributes section to the appropriate Groups under the attribute set.
Save Changes
- Click Save to update the attribute set.
Troubleshooting Common Issues
If you encounter issues while assigning attributes, use the table below to resolve them:
Issue | Possible Cause | Solution |
---|---|---|
Attributes not appearing in Unassigned Attributes | Attributes may already be assigned or have incorrect settings | Check existing attribute groups, verify attribute scope, and ensure attribute visibility. |
Changes not saving | Cache issue or incorrect permissions | Clear cache using php bin/magento cache:flush and check file permissions. |
Attribute set not updating in frontend | Indexing issue | Run php bin/magento indexer:reindex to update indexes. |
Attributes not reflecting in product creation | Attribute settings may be incorrect | Ensure attribute settings allow visibility in forms and product types. |
Best Practices for Managing Attribute Sets
- Plan Attribute Sets Carefully: Group similar attributes together to improve usability.
- Use Clear Attribute Labels: Ensure labels are user-friendly for easy understanding.
- Minimize Required Attributes: Only mark essential attributes as required to improve flexibility.
- Enable Caching for Performance: Reduce API calls by leveraging Magento’s caching mechanisms.
- Reindex Data After Changes: Run php bin/magento indexer:reindex to reflect updates quickly.
- Test on a Staging Environment First: Avoid breaking live store functionality by testing updates in a staging environment.
Assigning Attributes Programmatically in Magento 2
Magento 2 allows you to programmatically assign attributes to an attribute set to automate attribute configurations, ensuring efficient product catalog management. This guide covers the entire process, from method implementation to performance optimization, error handling, and troubleshooting.
1. Understanding the API Method
Magento provides the following method under
Magento\Catalog\Api\ProductAttributeManagementInterface
to assign attributes:
public function assign($attributeSetId, $attributeGroupId, $attributeCode, $sortOrder);
Parameter Breakdown
Parameter | Type | Description |
---|---|---|
$attributeSetId | int | ID of the attribute set (defines which set the attribute belongs to) |
$attributeGroupId | int | ID of the attribute group within the set |
$attributeCode | string | Code of the attribute to assign |
$sortOrder | int | Position of the attribute within the group |
Example Attribute Set Data in Magento Database
To find existing attribute sets and groups, use:
SELECT * FROM eav_attribute_set;
SELECT * FROM eav_attribute_group WHERE attribute_set_id = X;
2. Code to Assign an Attribute Programmatically
Step 1: Create a Custom Model File (AssignAttributeSet.php)
app/code/Vendor/Module/Model/AssignAttributeSet.php
namespace Vendor\Module\Model;
use Magento\Framework\Exception\LocalizedException;
use Magento\Catalog\Api\ProductAttributeManagementInterface;
use Psr\Log\LoggerInterface;
class AssignAttributeSet
{
/** * @var ProductAttributeManagementInterface */
private $productAttributeManagement;
/** * @var LoggerInterface */
private $logger;
public function __construct(
ProductAttributeManagementInterface $productAttributeManagement,
LoggerInterface $logger
) {
$this->productAttributeManagement = $productAttributeManagement;
$this->logger = $logger;
}
/** * Assign Product Attribute to Attribute Set Group. * * @return int * @throws LocalizedException */
public function assignAttributeToGroup(
$attributeSetId, $attributeGroupId, $attributeCode, $sortOrder
) {
try {
$result = $this->productAttributeManagement
->assign($attributeSetId, $attributeGroupId, $attributeCode, $sortOrder);
$this->logger->info("Attribute '" . $attributeCode . "' assigned successfully to Attribute Set ID: " . $attributeSetId . ".");
return $result;
} catch (LocalizedException $exception) {
$this->logger->error("Error assigning attribute: " . $exception->getMessage());
throw new LocalizedException(__($exception->getMessage()));
}
}
}
3. Calling the Function in a Template, Controller, or Cron Job
To call this function in your module’s controller or helper:
$attributeSetId = 16;
$attributeGroupId = 91;
$attributeCode = 'size';
$sortOrder = 50;
$result = $this->assignAttributeToGroup(
$attributeSetId, $attributeGroupId, $attributeCode, $sortOrder
);
4. Expected Output & Debugging
On Success:
- The attribute is assigned, and an integer ID is returned.
- Log:
[INFO] Attribute 'size' assigned successfully to Attribute Set ID: 16.
On Failure:
[ERROR] Error assigning attribute: Attribute Set ID does not exist.
5. Troubleshooting Common Issues
Issue | Possible Cause | Solution |
---|---|---|
No attributes returned | Incorrect or missing Attribute Set ID | Verify the ID using SELECT * FROM eav_attribute_set; and ensure attributes are assigned |
NoSuchEntityException | Attribute Set ID or Group ID doesn’t exist | Run: SELECT * FROM eav_attribute_set WHERE attribute_set_id = X; to verify |
Performance issues | Excessive API calls | Enable caching, optimize database queries, and fetch only required attributes |
Undefined method error | Incorrect dependency injection | Ensure ProductAttributeManagementInterface is correctly injected |
Empty attribute list | Attributes not assigned properly | Verify in Magento Admin (Stores → Attributes → Attribute Set) |
Slow API response | Fetching too much data at once | Implement pagination, limit the data scope, and retrieve only essential attributes |
6. Best Practices for Assigning Attributes in Magento 2
Use Dependency Injection (DI) Instead of ObjectManager
Always inject ProductAttributeManagementInterface
via the constructor rather than using ObjectManager
.
Validate Attribute Codes Before Assigning
Before assigning, check if the attribute exists:
SELECT * FROM eav_attribute WHERE attribute_code = 'size';
Use Proper Sorting for User Experience
Define sortOrder
based on importance:
Sort Order | Attribute Name |
---|---|
10 | SKU |
20 | Name |
30 | Price |
40 | Weight |
50 | Size |
Enable Magento Caching for Faster Processing
To reduce redundant queries, store frequently used attribute details in cache:
php bin/magento cache:enable
Avoid Fetching Unnecessary Attributes
Instead of retrieving all attributes, fetch only specific ones:
$attributeRepository->get('size'); // Fetch only 'size' attribute
Test in a Staging Environment Before Deployment
Never assign attributes in production without testing. Always validate:
php bin/magento setup:di:compile
php bin/magento indexer:reindex
php bin/magento cache:flush
7. Extending Functionality
Assign Multiple Attributes at Once
Modify the function to loop through multiple attributes:
$attributes = ['size', 'color', 'material'];
foreach ($attributes as $attribute) {
$this->assignAttributeToGroup(
$attributeSetId, $attributeGroupId, $attribute, 50
);
}
Remove an Attribute from an Attribute Set
To remove an assigned attribute:
public function unassignAttributeFromGroup($attributeSetId, $attributeCode)
{
return $this->productAttributeManagement->unassign($attributeSetId, $attributeCode);
}
This comprehensive guide covers every aspect of assigning attributes in Magento 2, ensuring that your implementation is efficient, error-free, and optimized.
Attribute Sort Order Example
Sort order defines the sequence in which attributes appear in the attribute set. Below is an example:
Sort Order | Attribute Name | Data Type | Is Required? | Frontend Input Type |
---|---|---|---|---|
10 | SKU | varchar | Yes | Text Field |
20 | Name | varchar | Yes | Text Field |
30 | Price | decimal | Yes | Text Field |
40 | Weight | decimal | Yes | Text Field |
50 | Size | varchar | No | Dropdown |
60 | Color | varchar | No | Dropdown |
70 | Material | varchar | No | Dropdown |
80 | Manufacturer | varchar | No | Dropdown |
Common Errors and Solutions
Here are some common issues that may arise when working with attributes and their possible solutions:
Issue | Possible Cause | Solution |
---|---|---|
No attributes returned | Incorrect or missing Attribute Set ID | Verify the ID using SELECT * FROM eav_attribute_set; and ensure attributes are assigned. |
NoSuchEntityException | Attribute Set ID or Group ID doesn’t exist | Run SELECT * FROM eav_attribute_set WHERE attribute_set_id = X; to check its existence. |
Performance issues | Excessive API calls | Enable caching, optimize database queries, and fetch only required attributes. |
Undefined method error | Incorrect dependency injection | Ensure ProductAttributeManagementInterface is correctly injected. |
Empty attribute list | Attributes not assigned properly | Verify in Magento Admin (Stores → Attributes → Attribute Set ). |
Slow API response | Fetching too much data at once | Implement pagination, limit the data scope, and retrieve only essential attributes. |
Key Takeways:
- Always use Dependency Injection to avoid direct ObjectManager usage.
- Validate attribute IDs before making assignments.
- Use caching to reduce redundant attribute fetch operations.
- Optimize queries by retrieving only necessary attributes.
- Handle exceptions properly to prevent system crashes.
- Log all attribute assignment errors for better debugging.
- Index frequently used attributes to improve performance.
- Test all attribute modifications in a staging environment before deploying to production.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Assigning product attributes to attribute sets in Magento 2.4.7 (2025) is crucial for maintaining a structured and efficient product catalog. Whether done manually through the admin panel or programmatically using ProductAttributeManagementInterface, this process enables better organization, streamlined workflows, and improved product management.
By leveraging Magento’s API, developers can automate attribute assignments, reducing manual effort and minimizing errors. This is especially useful for stores with large product catalogs, as it ensures consistency across all products. Implementing best practices such as validating attribute IDs, using dependency injection, and handling exceptions properly can further enhance performance and stability.
Additionally, a well-structured attribute set improves data retrieval speed, enhances product filtering, and supports better SEO optimization. With the right approach, businesses can create a more seamless experience for store administrators and customers alike. By keeping your attribute assignments optimized, you ensure a scalable, flexible, and well-maintained Magento store that meets evolving business needs.
FAQs
What is an attribute set in Magento 2?
An attribute set in Magento 2 is a predefined collection of attributes assigned to products, allowing structured product information.
How can I assign a product attribute to an attribute set manually?
You can manually assign attributes by navigating to Stores → Attributes → Attribute Set, then dragging and dropping the attribute from the Unassigned Attributes section to a group.
Can I assign an attribute to an attribute set programmatically?
Yes, you can use the Magento\Catalog\Api\ProductAttributeManagementInterface to assign an attribute to an attribute set programmatically.
What method is used to assign attributes programmatically in Magento 2?
The method assign($attributeSetId, $attributeGroupId, $attributeCode, $sortOrder)
is used to assign attributes programmatically.
What parameters are required to assign an attribute programmatically?
You need to pass $attributeSetId
(attribute set ID), $attributeGroupId
(attribute group ID), $attributeCode
(attribute code), and $sortOrder
(position in the group).
What is the default method for retrieving attribute details from an attribute set?
The method getAttributes($attributeSetId)
from Magento\Catalog\Api\ProductAttributeManagementInterface
retrieves all attributes assigned to an attribute set.
How do I get all assigned attributes from an attribute set?
You can retrieve assigned attributes by calling getAttributes($attributeSetId)
and iterating over the returned list.
What happens if an invalid attribute set ID is provided?
Magento will throw a "No such entity with id" exception if an invalid attribute set ID is provided.
Can I change the sort order of an attribute in an attribute set?
Yes, the $sortOrder
parameter in the assign
method determines the attribute's position in the group.
Why should I assign attributes to attribute sets?
Assigning attributes to attribute sets ensures better organization, improves product filtering, and enhances catalog management.
What is the benefit of assigning attributes programmatically?
Assigning attributes programmatically allows bulk updates, automation, and reduces manual errors in large catalogs.
How do I check if an attribute is successfully assigned?
The assign method returns an integer representing the assigned attribute ID. You can also verify the assignment in the Magento Admin Panel.
What are the best practices for managing attribute sets in Magento 2?
Best practices include using meaningful attribute names, keeping attributes grouped logically, avoiding redundant attributes, and validating IDs before assignment.
Does assigning attributes to attribute sets affect SEO?
Yes, properly assigned attributes improve product filtering, structured data, and searchability, enhancing SEO for eCommerce stores.