How to Retrieve Attribute Details by Attribute Set ID in Magento 2.4.7
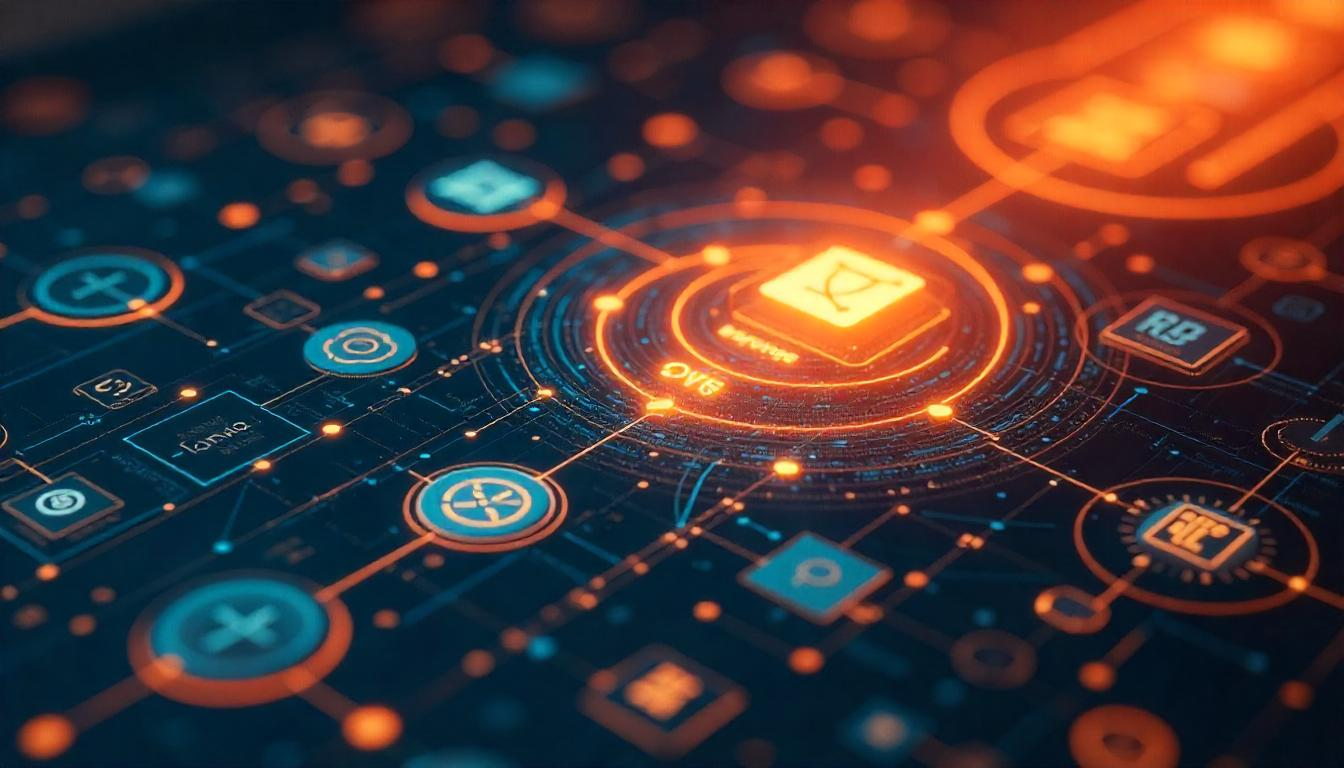
How to Retrieve Attribute Details by Attribute Set ID in Magento 2.4.7
Magento 2 provides powerful APIs to manage and retrieve product attribute information. In this guide, we demonstrate how to fetch all assigned attributes for a given attribute set using the native interface: ProductAttributeManagementInterface. This is useful for understanding the list of available attributes for any attribute set—for example, the default attribute set (ID 4).
Table Of Content
Overview
Magento’s API enables developers to programmatically retrieve all attributes assigned to a specific attribute set. This functionality is essential for custom module development, debugging attribute management, and creating dynamic forms or filtering mechanisms based on attribute data.
Key Points
- Attribute Set ID: Each attribute set in Magento has a unique identifier. For the default attribute set, the ID is typically 4.
- Interface Used: The
Magento\Catalog\Api\ProductAttributeManagementInterface
provides a method to get attributes by attribute set ID. - Error Handling: Always incorporate exception handling to catch scenarios where the attribute set might not exist or retrieval fails.
Detailed Explanation
Magento’s API offers robust methods to query attribute data, making it easier for developers to manage product attributes effectively. Using the getList
method from the ProductAttributeManagementInterface
, you can fetch a comprehensive list of attributes linked to a given attribute set ID. This is especially useful when:
- Listing Available Attributes: Identify all attributes for building custom modules or dynamic forms.
- Debugging: Troubleshoot issues related to attribute management by verifying the attribute set’s configuration.
- Custom Filtering: Create advanced filtering mechanisms for the storefront or admin panel based on attribute data.
Attribute Data Overview
Field | Description |
---|---|
Attribute Code | The unique identifier for the attribute used in code. |
Attribute Label | The human-readable name for the attribute. |
Data Type | The type of data the attribute holds (e.g., text, date, select). |
Is Required | Indicates whether the attribute is mandatory for product entry. |
Default Value | The default value set for the attribute, if any. |
Usage
Here is an example of how you might retrieve attributes for the default attribute set (ID 4):
$attributeManagement = $objectManager->get(\Magento\Catalog\Api\ProductAttributeManagementInterface::class);
$attributes = $attributeManagement->getList(4);
This code will return an array of attribute objects that you can use to build dynamic forms or implement custom filters.
Best Practices
- Validate API Responses: Always check that the returned collection of attributes is not empty before processing.
- Cache Attribute Data: Use caching mechanisms to store attribute data temporarily, reducing the load on your database.
- Implement Robust Error Handling: Use try-catch blocks to manage exceptions like
NoSuchEntityException
when an attribute set does not exist. - Optimize API Calls: Retrieve only the necessary attribute data to improve performance.
- Secure Your Data: Ensure that sensitive attribute data is accessible only to authorized users.
Tip:
For better performance and easier debugging, implement logging using Magento’s built-in logger \Psr\Log\LoggerInterface
. Log detailed error messages when attribute retrieval fails. This approach not only helps in diagnosing issues quickly but also ensures that your application can gracefully handle any unexpected errors.
Retrieving attribute data by attribute set ID is a powerful feature in Magento 2 that facilitates enhanced customization and dynamic functionality. By leveraging Magento’s API, employing best practices, and incorporating robust error handling and caching, developers can efficiently manage attribute data and build scalable, dynamic, and user-friendly applications.
Retrieving Attribute Details in Magento 2
Magento provides an efficient way to retrieve attribute details using the ProductAttributeManagementInterface
. This is particularly useful for dynamically managing product attributes in custom modules or debugging attribute configurations.
Step-by-Step Guide to Retrieve Attribute Details
Step 1: Inject the Required Interface
To fetch attributes, inject the ProductAttributeManagementInterface
into your class via dependency injection (DI).
namespace Vendor\Module\Model;
use Magento\Catalog\Api\ProductAttributeManagementInterface;
use Magento\Framework\Exception\NoSuchEntityException;
class AttributeRetriever
{
private $attributeManagement;
public function __construct(ProductAttributeManagementInterface $attributeManagement)
{
$this->attributeManagement = $attributeManagement;
}
}
Step 2: Fetch Attributes Using getAttributes()
Use the getAttributes()
method and pass the Attribute Set ID (e.g., 4
for default).
public function getAttributeDetails($attributeSetId)
{
try {
$attributes = $this->attributeManagement->getAttributes($attributeSetId);
return $attributes;
} catch (NoSuchEntityException $e) {
return __('Attribute Set not found.');
}
}
Step 3: Iterate Over Attributes & Display Details
Loop through the retrieved attributes to extract essential details like attribute code, label, data type, and more.
$attributeSetId = 4; // Example ID
$attributes = $this->getAttributeDetails($attributeSetId);
if (is_array($attributes)) {
foreach ($attributes as $attribute) {
echo "Code: " . $attribute->getAttributeCode() . "<br>";
echo "Label: " . $attribute->getFrontendLabel() . "<br>";
echo "Type: " . $attribute->getBackendType() . "<br>";
echo "Is Required: " . ($attribute->getIsRequired() ? "Yes" : "No") . "<br>";
echo "<hr>";
}
}
Common Issues & Fixes
Issue | Fix |
---|---|
No attributes returned | Ensure the Attribute Set ID is correct and exists in the Magento Admin panel. |
NoSuchEntityException | Verify that the requested attribute set exists. |
Slow performance | Implement caching for repeated attribute queries. |
Undefined method errors | Check if ProductAttributeManagementInterface is correctly injected. |
Tips for Optimization:
- Use Caching: Store frequently accessed attribute data in cache to reduce API calls.
- Enable Logging: Utilize \Psr\Log\LoggerInterface to log errors and debug issues.
- Batch Processing: If handling large datasets, process attributes in batches instead of fetching all at once.
- Validate Data: Ensure each attribute has a valid type and label before processing.
Retrieving attribute details programmatically is crucial for Magento 2 developers working on custom product configurations, filtering, and automation. Implementing proper error handling, caching, and batch processing can significantly improve performance and stability.
Code Explanation with Additional Data
Magento provides an efficient way to retrieve attributes from a specific attribute set using the ProductAttributeManagementInterface
. Understanding the method, process, and best practices ensures smooth implementation.
Method Signature
public function getAttributes($attributeSetId);
- $attributeSetId: The ID of the attribute set whose attributes you wish to retrieve.
- Return Type: An array of
ProductAttributeInterface
objects.
Process Overview
Step 1: Inject Required Interface
To fetch attributes programmatically, inject the ProductAttributeManagementInterface
into your class.
Step 2: Fetch Attributes
Call the getAttributes($attributeSetId)
method to retrieve an array of attributes.
Step 3: Process and Display
Loop through the result and process or display the attributes as needed.
Code Implementation
namespace Emmo\GetAttributes\Model;
use Magento\Framework\Exception\LocalizedException;
use Magento\Framework\Exception\NoSuchEntityException;
use Magento\Catalog\Api\Data\ProductAttributeInterface;
use Magento\Catalog\Api\ProductAttributeManagementInterface;
class GetAttributes {
/**
* @var ProductAttributeManagementInterface
*/
private $productAttributeManagement;
public function __construct(
ProductAttributeManagementInterface $productAttributeManagement
) {
$this->productAttributeManagement = $productAttributeManagement;
}
/**
* Get Product Attributes from the attribute set.
* @return ProductAttributeInterface[]
* @throws NoSuchEntityException
*/
public function getAttributeListBySetId() {
$attributeSetId = 4; // Default Attribute Set ID
try {
$getAttributes = $this->productAttributeManagement->getAttributes($attributeSetId);
} catch (NoSuchEntityException $exception) {
throw new NoSuchEntityException(__($exception->getMessage()));
}
return $getAttributes;
}
}
Common Issues & Fixes
Issue | Fix |
---|---|
No attributes returned | Ensure the Attribute Set ID is correct and exists in the Magento Admin panel. |
NoSuchEntityException | Verify that the requested attribute set exists. |
Slow performance | Implement caching for repeated attribute queries. |
Undefined method errors | Ensure ProductAttributeManagementInterface is correctly injected. |
Empty attribute list | Check if attributes are properly assigned to the attribute set. |
Best Practices & Optimization Tips
Tip | Description |
---|---|
Use Dependency Injection | Avoid using ObjectManager directly for better performance and maintainability. |
Cache Results | If fetching attributes multiple times, use Magento’s caching mechanisms. |
Handle Exceptions | Wrap your method calls inside try-catch blocks to prevent runtime errors. |
Validate Attribute Set ID | Always verify that the provided attributeSetId exists before making API calls. |
Optimize Queries | Fetch only necessary attributes instead of retrieving the full list. |
Log Errors | Use \Psr\Log\LoggerInterface to log issues for debugging. |
Using the Function in Your Code
To retrieve attributes assigned to a specific attribute set in Magento 2, follow these steps:
1. Call the Method and Iterate Over the Result
The function fetches attributes by attribute set ID, which you can then process.
$getAttributes = $this->getAttributeListBySetId();
foreach ($getAttributes as $attribute) {
// For debugging, print attribute details
var_dump($attribute->debug());
}
2. Expected Output
- On Success: An array of objects implementing
\Magento\Catalog\Api\Data\ProductAttributeInterface
, each containing details like attribute code, label, data type, and validation rules. - On Error: A NoSuchEntityException is thrown if the attribute set ID is invalid or does not exist.
Best Practices & Optimization Tips
Tip | Description |
---|---|
Use Dependency Injection | Always inject ProductAttributeManagementInterface instead of using ObjectManager. |
Validate Attribute Set ID | Ensure the ID exists before making API calls to prevent unnecessary errors. |
Error Handling | Wrap method calls inside try-catch blocks to catch NoSuchEntityException . |
Optimize Query Performance | Fetch only the required attributes instead of retrieving the full list. |
Cache Results | Use Magento’s caching mechanisms to avoid repeated database queries. |
Enable Logging | Utilize \Psr\Log\LoggerInterface to log unexpected issues for debugging. |
Test in a Staging Environment | Always test your implementation on a development server before deploying to production. |
Common Issues & Fixes
Issue | Fix |
---|---|
No attributes returned | Ensure the Attribute Set ID exists in the Magento Admin Panel. |
NoSuchEntityException | Verify the requested attribute set ID is valid and exists. |
Slow performance | Implement caching to reduce repeated queries. |
Undefined method errors | Ensure ProductAttributeManagementInterface is correctly injected into your class. |
Empty attribute list | Confirm that attributes are properly assigned to the attribute set in Magento Admin. |
Attributes Table with Additional Details
Below is an expanded table representing attribute details retrieved from an attribute set. This includes more metadata, such as default values, validation rules, and whether the attribute is visible on the frontend.
Attribute Code | Label | Data Type | Is Required? | Frontend Input Type | Default Value | Validation Rules | Visible on Frontend? |
---|---|---|---|---|---|---|---|
color | Color | varchar | Yes | Dropdown | None | Not Empty | Yes |
size | Size | varchar | No | Text Field | None | Alphanumeric | Yes |
material | Material | varchar | No | Dropdown | None | Not Empty | Yes |
weight | Weight | decimal | Yes | Text Field | 0.0 | Numeric | No |
manufacturer | Manufacturer | varchar | No | Dropdown | None | Not Empty | Yes |
sku | SKU | varchar | Yes | Text Field | Auto-Generated | Alphanumeric, Unique | No |
price | Price | decimal | Yes | Text Field | 0.00 | Numeric, Positive | No |
special_price | Special Price | decimal | No | Text Field | None | Numeric, Positive | No |
status | Status | int | Yes | Dropdown | Enabled | 1 (Enabled) / 2 (Disabled) | Yes |
visibility | Visibility | int | Yes | Dropdown | Catalog, Search | 1-4 Options | No |
Note: The actual attributes depend on your Magento installation and the selected attribute set. You can also customize or add more attributes based on your store's requirements.
Best Practices for Attribute Management
To ensure efficient use of attributes in Magento, follow these best practices:
Tip | Description |
---|---|
Use Dependency Injection | Always inject ProductAttributeManagementInterface instead of using ObjectManager . |
Validate Attribute Set ID | Ensure the ID exists before making API calls to prevent errors. |
Optimize Query Performance | Retrieve only the required attributes instead of fetching everything. |
Use Magento’s Caching Mechanisms | Implement caching to reduce redundant API calls and database queries. |
Enable Logging | Use \Psr\Log\LoggerInterface to log unexpected errors for debugging. |
Set Proper Validation Rules | Define validation rules to maintain data integrity. |
Keep Required Attributes Minimal | Only set essential attributes as required to improve product management flexibility. |
Use Indexed Attributes for Performance | Ensure frequently used attributes are indexed for faster retrieval. |
Test in a Staging Environment | Always test attribute configurations and API calls in a staging setup before applying changes to the live store. |
By following these guidelines, you can efficiently manage attributes in Magento, optimize store performance, and enhance user experience.
Best Practices and Tips
Always validate the attribute set ID before making API calls to prevent runtime errors. If necessary, check the ID directly in the database.
Use Dependency Injection (DI) by injecting ProductAttributeManagementInterface
in the constructor rather than using ObjectManager
. This improves maintainability and follows Magento’s best coding practices.
Implement exception handling with try-catch
blocks to manage errors like NoSuchEntityException
and InputException
. This ensures smooth execution even when the requested attribute set is missing.
Enable caching for attribute data if it’s accessed frequently. Magento’s built-in caching mechanisms can significantly reduce redundant database queries and improve performance.
Optimize attribute queries by fetching only necessary attributes> instead of retrieving the entire set. Indexed attributes further improve performance and query speed.
Use debugging techniques such as var_dump()
, print_r()
, or Magento’s logging system \Psr\Log\LoggerInterface
to analyze attribute data and identify potential issues during development.
Limit the number of required attributes to improve flexibility in product management. Setting too many required fields can make product imports and updates more difficult.
Leverage Magento’s API (REST or GraphQL) to retrieve and manage attributes dynamically, making integration with external systems more efficient.
Monitor database performance to ensure attribute retrieval is fast. Indexing attributes and optimizing queries can prevent slow responses.
Always test in a staging environment before applying changes to the live store. This helps catch potential errors without disrupting the customer experience.
Troubleshooting Common Issues
Issue | Possible Cause | Solution |
---|---|---|
No attributes returned | Incorrect or missing Attribute Set ID | Verify the ID and ensure attributes are assigned. Run php bin/magento indexer:reindex to refresh data. |
NoSuchEntityException | Attribute Set ID does not exist | Run the query: SELECT * FROM eav_attribute_set WHERE attribute_set_id = X; to check its existence. |
Performance issues | Excessive API calls or unoptimized queries | Enable caching, optimize database queries, and fetch only required attributes. |
Undefined method error | Incorrect dependency injection | Ensure ProductAttributeManagementInterface is correctly injected. Run php bin/magento setup:upgrade and php bin/magento setup:di:compile . |
Empty attribute list | Attributes not properly assigned | Verify attribute assignment in Magento Admin and reassign if needed. |
Slow API response | Fetching too much data at once | Implement pagination, limit the data scope, and retrieve only essential attributes. |
Tip:
To avoid performance issues, always cache frequently used attribute data and use indexed attributes for faster retrieval. Additionally, log API errors using \Psr\Log\LoggerInterface
to track and debug unexpected issues efficiently.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Retrieving attribute details by attribute set ID in Magento 2.4.7 (2025) is an essential skill for developers working with product data and custom module development. By leveraging the ProductAttributeManagementInterface, you can efficiently fetch, analyze, and utilize attribute data to enhance store functionality.
This guide covered everything from understanding attribute sets to implementing a working solution with robust exception handling, best practices, and optimization techniques. With proper error handling, caching strategies, and debugging methods, you can ensure a seamless and efficient attribute management process.
By following this structured approach, Magento developers can create dynamic, scalable, and well-optimized eCommerce solutions while maintaining flexibility in product attribute management. Implementing these techniques will help you build better-performing Magento stores with improved customization capabilities.
FAQs
What is an attribute set in Magento 2?
An attribute set in Magento 2 is a predefined collection of attributes assigned to products, allowing structured product information.
How do I retrieve attributes by attribute set ID in Magento 2?
You can use the Magento\Catalog\Api\ProductAttributeManagementInterface
and call the getAttributes($attributeSetId)
method to fetch assigned attributes.
What is the default attribute set ID in Magento 2?
By default, Magento assigns an attribute set ID of 4 to the "Default" attribute set.
Which Magento interface is used to get attributes by attribute set ID?
The Magento\Catalog\Api\ProductAttributeManagementInterface
is used to retrieve attributes for a given attribute set.
How do I handle errors when fetching attributes?
Use a try-catch block to handle exceptions like NoSuchEntityException
to prevent errors when an invalid attribute set ID is provided.
What is the output format when fetching attributes?
The method returns an array of objects implementing \Magento\Catalog\Api\Data\ProductAttributeInterface
.
Can I fetch attributes for a custom attribute set?
Yes, you can retrieve attributes for any attribute set by passing the correct attribute set ID.
How do I loop through attributes after retrieving them?
Use a foreach loop like foreach ($getAttributes as $attribute) { var_dump($attribute->debug()); }
to iterate over the attributes.
What are common attributes included in an attribute set?
Common attributes include color
, size
, material
, and weight
, among others.
Why is no attribute data returned?
Ensure the attribute set ID exists and has assigned attributes. Also, check if caching is affecting the results.
How can I improve performance when retrieving attributes?
Cache the attribute data and avoid frequent API calls to optimize performance.
Can I retrieve attributes programmatically in a custom module?
Yes, implement ProductAttributeManagementInterface
in a custom module to fetch attributes dynamically.
What happens if an invalid attribute set ID is provided?
A NoSuchEntityException
is thrown, indicating that the attribute set does not exist.
Where can I find attribute set IDs in Magento?
Attribute set IDs can be found in the Magento admin under Stores → Attributes → Attribute Set or by querying the eav_attribute_set
database table.