How to Apply Sort Order in Search Criteria Repositories in Magento 2
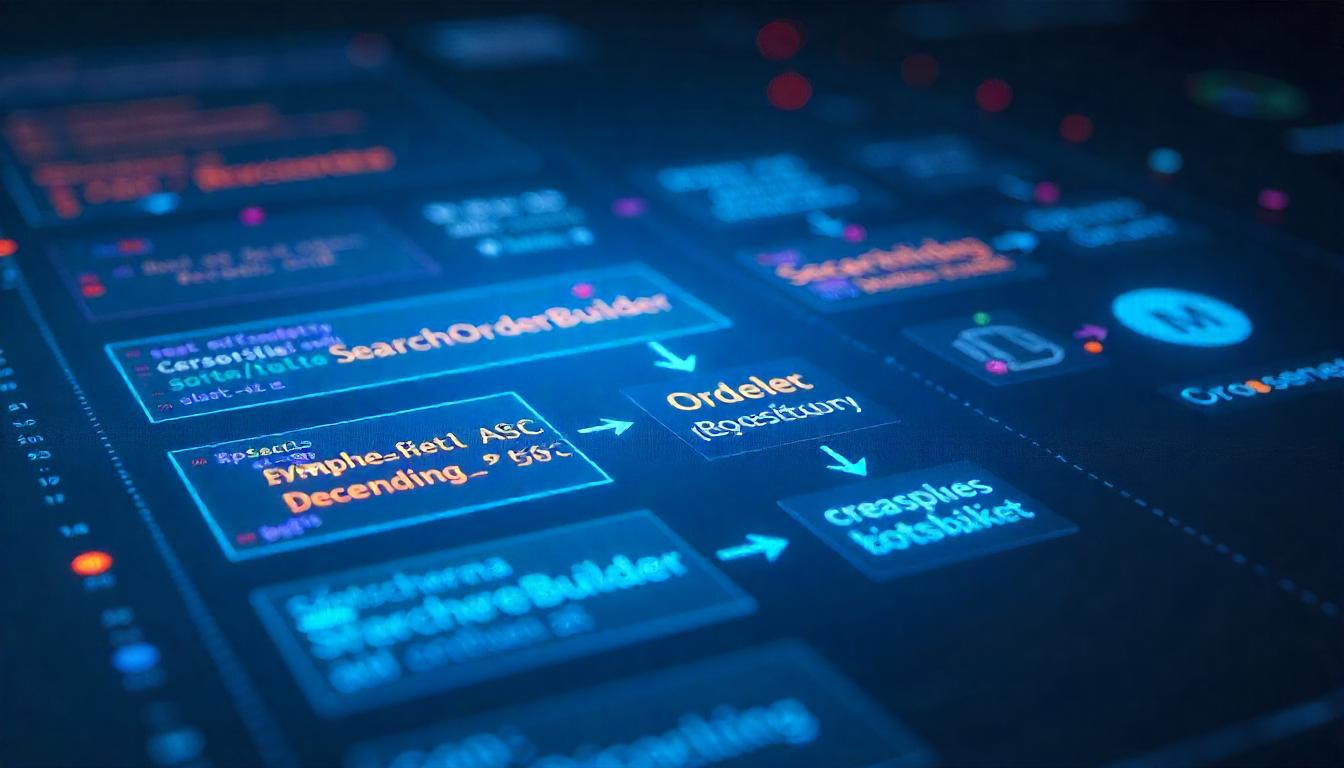
How to Apply Sort Order in Search Criteria Repositories in Magento 2
In Magento 2, Search Criteria is a key component used for querying data from repositories. It helps filter and sort data when retrieving lists of entities, such as products, categories, customers, etc., from the database. The ability to apply sort orders to search results is essential when you need to order data based on specific fields, such as price, name, or any custom attribute.
Table Of Content
How to Apply Sort Order in Search Criteria Repositories in Magento 2
In Magento 2, you can easily apply a sort order to your search criteria repositories using the Magento\Framework\Api\SortOrderBuilder class.
This allows you to sort results in either ascending (ASC) or descending (DESC) order by any field.
Here’s an example of how to use the SortOrderBuilder to sort orders by creation date in descending order:
$sortOrder = $this->sortOrderBuilder
->setField('created_at') // Field to sort by
->setDirection(SortOrder::SORT_DESC) // Sorting direction
->create();
This code snippet creates a sort order based on the created_at field in descending order, ensuring that the most recent orders are fetched first.
Example: Fetch Orders by Created Date with Sort Order
The following example demonstrates how to apply the sort order when retrieving orders. In this case, we sort the orders by their creation date.
namespace YourNamespace\Sales\Model;
use Magento\Framework\Api\SortOrder;
use Magento\Framework\Api\SortOrderBuilder;
use Magento\Framework\Api\SearchCriteriaBuilderFactory;
use Magento\Sales\Api\Data\OrderSearchResultInterface;
use Magento\Sales\Api\OrderRepositoryInterface;
class SortBySalesOrder
{
private SortOrderBuilder $sortOrderBuilder;
private OrderRepositoryInterface $orderRepository;
private SearchCriteriaBuilderFactory $searchCriteriaBuilderFactory;
public function __construct(
SortOrderBuilder $sortOrderBuilder,
OrderRepositoryInterface $orderRepository,
SearchCriteriaBuilderFactory $searchCriteriaBuilderFactory
) {
$this->sortOrderBuilder = $sortOrderBuilder;
$this->orderRepository = $orderRepository;
$this->searchCriteriaBuilderFactory = $searchCriteriaBuilderFactory;
}
public function getOrdersByCreatedDate(): OrderSearchResultInterface
{
$searchCriteriaBuilder = $this->searchCriteriaBuilderFactory->create();
$searchCriteriaBuilder->addFilter('state', ['new', 'pending_payment'], 'in'); // Filter orders by state
$sortOrder = $this->sortOrderBuilder
->setField('created_at') // Sorting by creation date
->setDirection(SortOrder::SORT_DESC) // Descending order
->create();
$searchCriteriaBuilder->addSortOrder($sortOrder);
$searchCriteria = $searchCriteriaBuilder->create();
return $this->orderRepository->getList($searchCriteria); // Fetch the orders
}
}
In this example, we filter orders by their state (new or pending_payment) and then apply the sort order on the created_at field to get the latest orders.
Key Takeaways:
- Sorting:Sort orders or any data by any field using the SortOrderBuilder.
- Sorting Direction: You can choose between ascending (ASC) or descending (DESC) order.
- Search Criteria: Use
SearchCriteriaBuilder
to apply filters and sort orders together.
By following this approach, you can control the sorting of any data retrieved via the search criteria interface in Magento 2. It ensures you get the results that matter most, in the correct order.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What is Sort Order in Magento 2?
Sort order in Magento 2 allows you to control how the results of a search query are ordered, either in ascending or descending order, based on a specified field. It helps organize data in a way that makes it easier to manage and view.
How Do You Apply Sort Order in Magento 2 Search Criteria Repositories?
To apply sort order in Magento 2, use the SortOrderBuilder class. You can specify a field to sort by, such as `created_at`, and set the direction to either ASC or DESC.
What Fields Can You Use for Sort Order in Magento 2?
You can use any field available in your database for sorting. Common fields include created_at
, updated_at
, or custom fields depending on your requirements.
How Do You Sort Orders by Created Date in Magento 2?
To sort orders by their creation date, use the SortOrderBuilder with the field set to `created_at` and the direction set to SortOrder::SORT_DESC for descending order, ensuring the most recent orders are returned first.
How Can You Combine Filters and Sort Order in Magento 2?
You can combine filters and sort orders by using the SearchCriteriaBuilder to add filters (e.g., filtering orders by their state) and then applying the sort order to the search criteria.
Can You Apply Multiple Sort Orders in Magento 2?
Yes, you can apply multiple sort orders in Magento 2. Simply create multiple SortOrder objects and add them to the search criteria. This is useful for sorting by more than one field, such as by `created_at` and `updated_at`.
How Does Sort Order Affect Search Results in Magento 2?
Sort order determines the order in which results are displayed. For example, applying DESC
to the `created_at` field will display the most recent results first, improving data accessibility and relevance.
What is the Default Sort Order in Magento 2?
The default sort order is typically set to ASC
(ascending) for most fields, but this can be customized based on specific use cases and requirements within your Magento setup.
Can You Sort Data Dynamically in Magento 2?
Yes, Magento 2 allows dynamic sorting based on different criteria by using custom logic and adding sort orders programmatically. You can adjust the sorting order based on user inputs or other conditions in your application.
What Are the Benefits of Using Sort Order in Magento 2?
Using sort order improves data organization, helps deliver results in a user-friendly manner, and ensures that data is fetched in the desired sequence. It enhances the overall functionality of search and reporting systems in Magento 2.