How to Create a Custom GraphQL Module in Magento 2
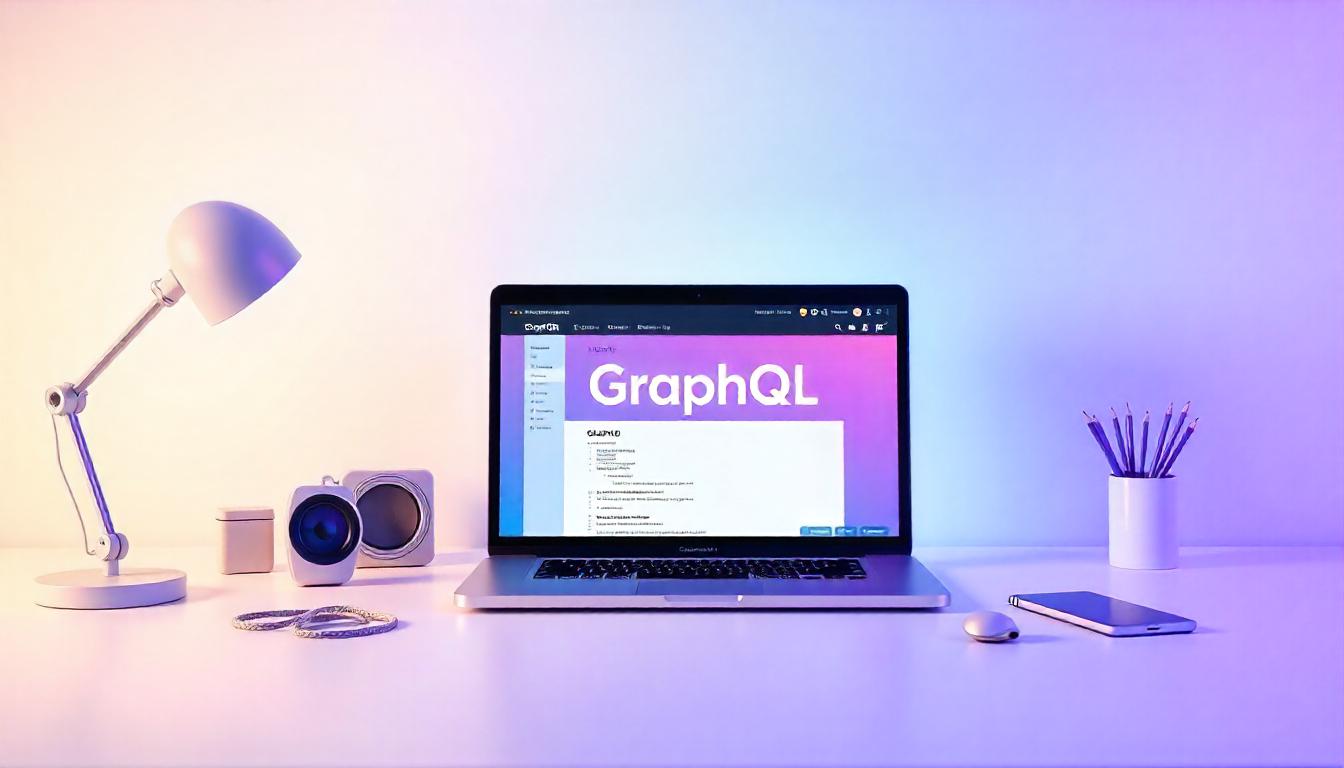
How to Create a Custom GraphQL Module in Magento 2
GraphQL is a modern query language for APIs, introduced in Magento 2.3, which allows users to fetch only the data they need, enhancing performance. Many Magento enthusiasts are eager to understand how GraphQL works within Magento 2, how to create custom modules using GraphQL, and how it interacts with Magento's underlying architecture.
In this guide, we will walk you through creating a simple custom GraphQL module in Magento 2 that fetches sales-related data. We will also discuss the core concepts of GraphQL, its importance for Magento 2, and the steps to implement it in your custom modules.
Table Of Content
- What is GraphQL?
- Step 1: Setting Up a Basic Magento 2 Module for GraphQL Integration
- Step 2: Define the GraphQL Schema in Magento 2
- Step 3: Implement the Resolver for GraphQL Query
- Step 4: Installing and Configuring Your Custom Module
- Step 5: Test Your GraphQL Query for Seamless Integration
- Conclusion
- FAQs
What is GraphQL?
GraphQL is a powerful and flexible alternative to traditional REST APIs that enables efficient and precise data retrieval. Unlike REST, where clients receive predefined data structures, GraphQL allows clients to request exactly the data they need, reducing over-fetching and under-fetching of information. This capability leads to faster and more efficient communication between clients and servers.
In the context of Magento 2, GraphQL plays a crucial role in enabling seamless communication between the backend and frontend applications. It allows developers to fetch and manipulate data in a structured and optimized manner, enhancing the overall performance of modern web applications like mobile apps, single-page applications (SPAs), and Progressive Web Apps (PWAs). GraphQL in Magento 2 simplifies integration with third-party services, making it a go-to choice for building robust eCommerce solutions.
Key Advantages of GraphQL in Magento 2
- Efficient Data Fetching: Request only the data you need without unnecessary queries.
- Flexible Responses: Clients can define the structure of the response, making it more tailored and useful.
- Real-time Data: GraphQL supports subscriptions, enabling real-time updates on data changes.
- Optimized for Frontend Applications: Perfect for SPAs, PWAs, and mobile apps due to its flexible and dynamic query system.
Prerequisites
Before implementing GraphQL in Magento 2, ensure that you have the following:
Prerequisite | Description |
---|---|
Magento 2.3 or later | Ensure you're using Magento 2.3 or a later version. |
Basic Knowledge of Magento 2 | Familiarity with Magento 2's module development is necessary. |
Understanding of GraphQL Concepts | A basic understanding of GraphQL syntax and its benefits. |
If you’re new to GraphQL, we recommend referring to the GraphQL Documentation to understand its core concepts and capabilities before diving into Magento-specific implementations.
Next Steps
Once you’ve ensured that you meet the prerequisites, you're ready to explore GraphQL queries and mutations within Magento 2. This will allow you to enhance your eCommerce store's performance by making data requests more efficient, and integrating seamlessly with frontend applications like SPAs and PWAs.
Step 1: Setting Up a Basic Magento 2 Module for GraphQL Integration
To implement GraphQL in Magento 2, you first need to create a basic custom module. In Magento, a module is a key unit of functionality that enables you to add features or services to your store. By following the steps below, we will create the foundation for integrating GraphQL functionality.
1.1 Create the registration.php
File
The registration.php
file is used to register the module with Magento, telling it that the module exists and where to find it.
File Path:app/code/Rbj/DemoGraphQl/registration.php
Magento 2 Module Registration Example
<?php
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Rbj_DemoGraphQl',
__DIR__
);
?>
1.2 Define Module Configuration in module.xml
The module.xml
file defines the module's basic configuration, including its dependencies on other modules. In this case, we will ensure our module depends on the Magento_Sales
and Magento_GraphQl
modules, which are required for GraphQL functionality and sales operations.
File Path:app/code/Rbj/DemoGraphQl/etc/module.xml
XML Configuration for Magento 2 Module
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Rbj_DemoGraphQl">
<sequence>
<module name="Magento_Sales"/>
<module name="Magento_GraphQl"/>
</sequence>
</module>
</config>
Overview of Module Configuration Files
Here's a summary of the key files in your module setup and their purposes:
File | Path | Description |
---|---|---|
registration.php | app/code/Rbj/DemoGraphQl </registration.php> |
Registers the module with Magento, defining the module's namespace and directory location. |
module.xml | app/code/Rbj/DemoGraphQl/ etc/module.xml |
Defines the module configuration, including dependencies on other modules like Magento_Sales and Magento_GraphQl. |
Next Steps
Once the module is registered, the next step would be to implement the GraphQL schema, which will define the data that can be queried through GraphQL. This will involve creating a schema file, resolver classes, and mapping the GraphQL queries to the module's logic.
By following these steps, you create a solid foundation for integrating GraphQL with your custom Magento 2 module.
Step 2: Define the GraphQL Schema in Magento 2
The GraphQL schema forms the core of any GraphQL implementation. It specifies the structure of the data that can be queried and the operations that can be performed. In Magento, each GraphQL module must include a schema.graphqls
file, which outlines the data structure and available queries or mutations.
File Location:
app/code/Rbj/DemoGraphQl/etc/schema.graphqls
GraphQL Schema:
GraphQL Schema Definition
type Query {
salesOrder(
id: Int @doc(description: "ID of the Sales Order")
): SalesOrder @resolver(class: "Rbj\\DemoGraphQl\\Model\\Resolver\\SalesOrder") @doc(description: "Retrieve sales order details by ID")
}
type SalesOrder {
increment_id: String @doc(description: "Order Increment ID")
customer_name: String @doc(description: "Customer Name")
grand_total: String @doc(description: "Total amount of the order")
is_guest_customer: Boolean @doc(description: "Indicates if the order was placed by a guest")
address_array: [String] @doc(description: "Shipping address details (city, postcode, state)")
}
Schema Explanation:
Element | Description |
---|---|
Query | Defines the entry point for the GraphQL query. |
salesOrder | A query to fetch details of a specific sales order using its ID. |
SalesOrder | A custom data type representing sales order details, including customer info, total amount, and more. |
increment_id | The unique increment ID of the order. |
customer_name | The name of the customer who placed the order. |
grand_total | The total amount for the order. |
is_guest_customer | A Boolean field that indicates whether the order was placed by a guest customer. |
address_array | An array containing shipping address details such as city, postcode, and state. |
Key Benefits of Using GraphQL in Magento 2:
- Flexibility: Fetch only the data you need, minimizing unnecessary data transfers.
- Efficiency: Reduce API requests by combining multiple queries into a single call.
- Scalability: Ideal for modern applications like Progressive Web Apps (PWAs) and Single Page Applications (SPAs).
By creating and defining the schema as shown, you can build robust GraphQL queries in Magento 2 to efficiently retrieve specific sales order data.
Step 3: Implement the Resolver for GraphQL Query
The resolver in GraphQL is a critical component responsible for retrieving the required data based on the query input. Here, we will implement the SalesOrder
resolver to fetch order details dynamically.
Purpose of the Resolver
- Class Name:
SalesOrder
- Location:
app/code/Rbj/DemoGraphQl/Model/Resolver/SalesOrder.php
- Functionality:
- Validates the input (Sales Order ID).
- Retrieves the corresponding order details.
- Returns formatted data for GraphQL queries.
Code Implementation
Below is the modified PHP code for the SalesOrder
resolver:
PHP Resolver Code
<?php
declare(strict_types: true);
namespace Rbj\DemoGraphQl\Model\Resolver;
use Magento\Framework\Exception\NoSuchEntityException;
use Magento\Framework\GraphQl\Config\Element\Field;
use Magento\Framework\GraphQl\Exception\GraphQlInputException;
use Magento\Framework\GraphQl\Exception\GraphQlNoSuchEntityException;
use Magento\Framework\GraphQl\Query\ResolverInterface;
use Magento\Framework\GraphQl\Schema\Type\ResolveInfo;
/**
* Resolver for fetching sales order details
*/
class SalesOrder implements ResolverInterface
{
private $orderRepository;
public function __construct(
\Magento\Sales\Api\OrderRepositoryInterface $orderRepository
) {
$this->orderRepository = $orderRepository;
}
/**
* Fetches the sales order data.
*/
public function resolve(
Field $field,
$context,
ResolveInfo $info,
array $value = null,
array $args = null
) {
$orderId = $this->validateAndGetOrderId($args);
return $this->fetchOrderDetails($orderId);
}
/**
* Validates and extracts the Sales Order ID from arguments.
*/
private function validateAndGetOrderId(array $args): int
{
if (empty($args['id'])) {
throw new GraphQlInputException(__('Sales Order ID is required.'));
}
return (int) $args['id'];
}
/**
* Retrieves and formats sales order data by ID.
*/
private function fetchOrderDetails(int $orderId): array
{
try {
$order = $this->orderRepository->get($orderId);
return [
'increment_id' => $order->getIncrementId(),
'customer_name' => $order->getCustomerFirstname() . ' ' . $order->getCustomerLastname(),
'grand_total' => $order->getGrandTotal(),
'is_guest_customer' => (bool) $order->getCustomerIsGuest(),
'address_array' => [
'name' => $order->getShippingAddress()->getFirstname() . ' ' . $order->getShippingAddress()->getLastname(),
'city' => $order->getShippingAddress()->getCity(),
'postcode' => $order->getShippingAddress()->getPostcode(),
'region' => $order->getShippingAddress()->getRegion(),
],
];
} catch (NoSuchEntityException $e) {
throw new GraphQlNoSuchEntityException(__('No order found with the given ID.'));
}
}
}
Key Elements in the Resolver
Component | Description |
---|---|
resolve() |
Processes the GraphQL query and returns the data. |
validateAndGetOrderId() |
Validates the input arguments and ensures the Sales Order ID is provided. |
fetchOrderDetails() |
Retrieves and formats sales order details based on the order ID. |
orderRepository |
Uses Magento's API to fetch order details directly from the database. |
Exception Handling | Ensures proper error messages are displayed for invalid or non-existent orders. |
This ensures a comprehensive explanation for implementing the resolver effectively.
Step 4: Installing and Configuring Your Custom Module
Once you have created the necessary files for your custom Magento 2 module, it's time to install and configure it. Follow the steps below to ensure your module is properly set up and ready to use.
1. Upgrade the Magento Setup
To install your module, run the following commands in your Magento 2 root directory:
Run the Following Commands:
php bin/magento setup:upgrade
php bin/magento cache:flush
These commands perform the following tasks:
setup:upgrade
: Registers your custom module in Magento's configuration and updates the database schema, if necessary.cache:flush:
Clears all cached data to ensure the changes take effect immediately.
2. Verify the Installation
To confirm your module has been installed correctly:
- Navigate to the Magento Admin Panel.
- Go to Stores > Configuration > Advanced > Advanced.
- Locate your module in the module list and ensure its status is set to Enabled.
3. Configure Your Module
If your module has configuration options, set them up according to your needs. Configuration is typically found under Stores > Configuration, within a specific section created for your module.
Example Output After Running Commands
After running the commands, you should see output similar to the following:
Cache cleared successfully
Module 'Vendor_ModuleName' has been installed and is enabled.
Step 5: Test Your GraphQL Query for Seamless Integration
Now that your module is ready, it’s time to validate its functionality by running a GraphQL query. Testing ensures your module works as expected and provides accurate data. Utilize robust tools like ChromeiQL, Altair GraphQL, or any preferred GraphQL client for this process.
Access the GraphQL Endpoint
To begin testing, open your GraphQL client and connect to the Magento endpoint using the following URL:
http://your-magento-url/graphql
Send a Sample Query
Use this query to fetch detailed sales order information from your custom module:
{
salesOrder(id: 1) {
increment_id
grand_total
customer_name
is_guest_customer
address_array
}
}
Expected JSON Response
If everything is set up correctly, you should receive a response like this:
{
"data": {
"salesOrder": {
"increment_id": "000000001",
"grand_total": "230.00",
"customer_name": "Emmo Inc",
"is_guest_customer": false,
"address_array": [
"Emmo Inc",
"Ali Abad",
"15600",
"Gilgit"
]
}
}
}
This response confirms that the module fetches data as intended.
Handle Errors Gracefully
Errors are a natural part of development. Magento provides built-in exceptions for GraphQL operations to help you identify issues quickly:
Error Type | Description |
---|---|
GraphQlInputException | Triggered when mandatory input data is missing or invalid. |
NoSuchEntityException | Thrown when the requested entity, such as a sales order, cannot be located in the database. |
For example, if you send an invalid id
, you might receive an error message similar to this:
{
"errors": [
{
"message": "The sales order does not exist.",
"category": "graphql",
"locations": [{ "line": 2, "column": 3 }],
"path": ["salesOrder"]
}
]
}
Advanced Features and Optimizations
To make your module more dynamic and scalable, consider implementing the following enhancements:
Complex Queries and Mutations
Add support for related operations like fetching multiple orders or updating order statuses.
Caching Mechanisms
Use Magento’s built-in caching system to store frequently queried data, improving performance.
Access Control
Validate user roles and permissions to restrict access to sensitive order data, ensuring security.
Enhanced Validation
Incorporate advanced checks for input values and user actions to reduce errors during GraphQL interactions.
Custom Error Messages
Provide user-friendly error messages to help developers debug issues more efficiently.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Creating and testing a custom GraphQL module in Magento 2 opens up a world of possibilities for streamlined data access and enhanced user experiences. By following the step-by-step process outlined—starting with module creation, defining resolvers, testing GraphQL queries, and handling errors—you can build robust, scalable integrations that meet your eCommerce needs.
Through practical examples like retrieving sales order details, you’ve learned how to structure queries, manage exceptions, and optimize performance. Additionally, advanced considerations such as caching and security underscore the importance of a well-rounded approach to development.
GraphQL’s flexibility and Magento’s extensibility combine to create powerful solutions that elevate your store's functionality, improve developer efficiency, and ultimately enhance customer satisfaction. By implementing these techniques and best practices, you’ll ensure your Magento store stays competitive and future-ready.
FAQs
What is GraphQL in Magento 2?
GraphQL is a query language for APIs that enables clients to request exactly the data they need, making it more efficient than traditional REST APIs. Magento 2 supports GraphQL for enhanced data fetching and flexibility.
How do I create a custom module for GraphQL in Magento 2?
To create a custom module in Magento 2, define the necessary files such as the module declaration, configuration, and resolver classes. Then, implement the required logic to handle GraphQL queries.
What is a resolver in GraphQL?
A resolver in GraphQL is a PHP class that contains the logic to fetch and return data based on the specified GraphQL query. It acts as a handler for the requested query.
How do I test a GraphQL query in Magento 2?
You can test a GraphQL query using tools like ChromeiQL or Altair GraphQL. Simply send your query to the Magento GraphQL endpoint and verify the response.
What GraphQL tools can I use for testing in Magento 2?
Tools like ChromeiQL, Altair GraphQL, and Postman are ideal for testing GraphQL queries and inspecting responses in Magento 2.
What is the expected response for a sales order query?
The expected response for a sales order query typically includes details like order increment ID, customer name, grand total, guest status, and address array.
How do I handle errors in GraphQL queries?
Errors in GraphQL queries are handled by throwing exceptions such as GraphQlInputException for invalid input and NoSuchEntityException for missing entities like a sales order.
What are the common exceptions thrown in GraphQL queries?
Common exceptions include GraphQlInputException, which occurs when the input is invalid, and NoSuchEntityException, which happens when an entity is not found.
What should I do if I encounter an error like "The sales order does not exist"?
If this error occurs, verify that the provided sales order ID is correct and exists in the database. This error typically happens when querying a non-existent order.
How do I set up GraphQL queries for Magento 2?
Set up GraphQL queries by creating a schema for the query, defining resolvers for the logic, and ensuring the query structure matches Magento's GraphQL setup.
What are the best practices for optimizing GraphQL queries in Magento 2?
Best practices include using caching mechanisms for frequent queries, minimizing the amount of data returned, and validating user permissions for secure access to sensitive data.
Can I extend GraphQL functionality in Magento 2?
Yes, you can extend GraphQL functionality by adding more complex queries, mutations, and integrating additional features like user permissions and caching mechanisms.
What are the main benefits of using GraphQL in Magento 2?
GraphQL offers efficient data fetching by allowing clients to request only the necessary data, reducing over-fetching, and providing a more flexible and optimized solution for complex APIs.