Understanding Polyfills: What They Are and How They Work
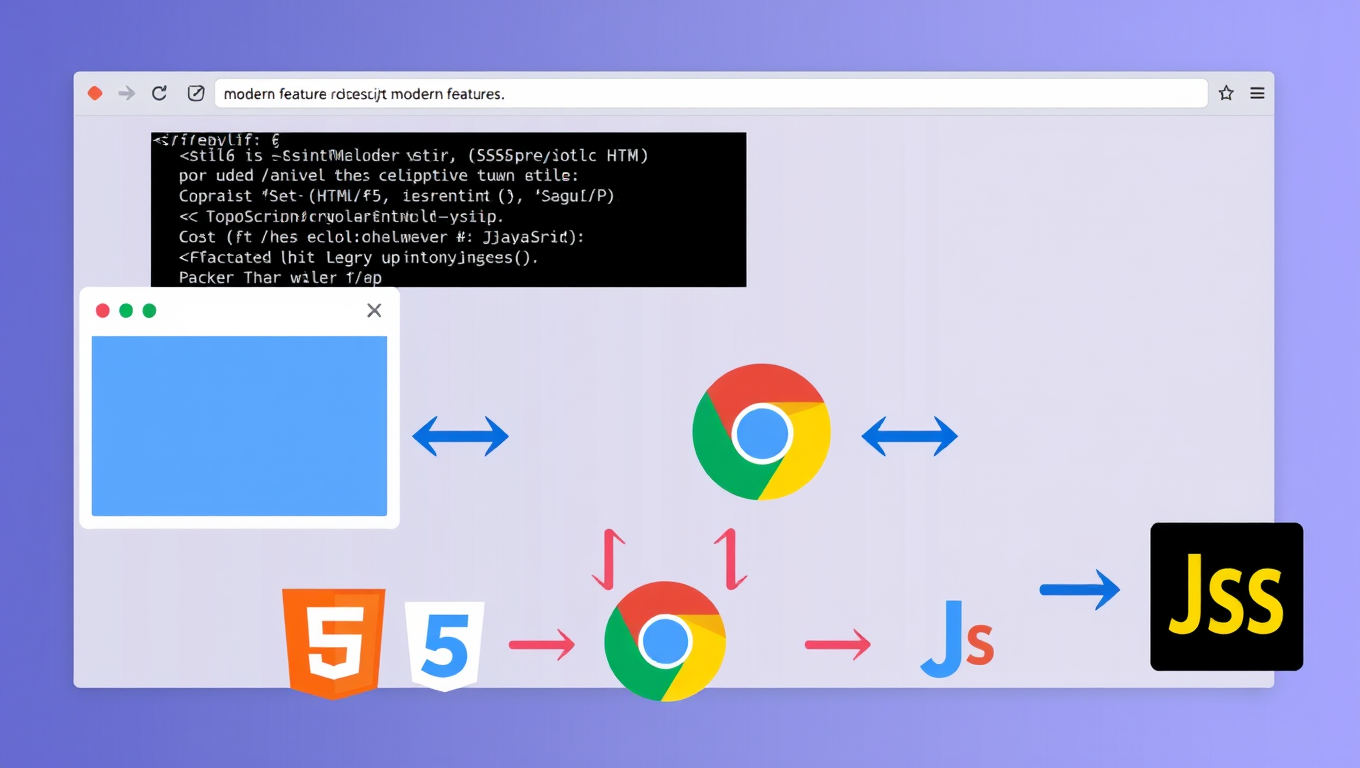
Understanding Polyfills: What They Are and How They Work
Table of Contents
What is a Polyfill?
A polyfill is a piece of code that emulates the functionality of a web feature that may not be natively supported by a browser. Polyfills are particularly important in web development because different browsers and versions support different features of modern web standards like HTML5, CSS3, or ECMAScript (JavaScript). When a new feature is introduced, older browsers might not recognize it or be able to execute it properly. Polyfills essentially "fill in the gap," allowing developers to write modern code while ensuring that it runs on older browsers.
Polyfills are typically written in JavaScript and can simulate various web features, such as new JavaScript methods, HTML5 elements, or even more complex APIs like fetch(). They ensure that users with older browsers can still access the core functionality of a website without the developers needing to rewrite the entire site using outdated practices.
Why are Polyfills Important in Web Development?
Polyfills play a crucial role in modern web development because they ensure compatibility across different browsers. Every web browser has its own implementation of web standards, and some lag behind others when it comes to supporting the latest features. This fragmentation in browser support makes it challenging to build web applications that work consistently for all users.
Without polyfills, developers would be forced to either avoid using new features until all browsers catch up, or risk breaking functionality for users with outdated browsers. Polyfills offer a middle ground, allowing developers to take advantage of the latest web technologies while maintaining backward compatibility. This improves user experience, as it reduces the likelihood of encountering errors or missing functionality when accessing a website from an older browser.
Moreover, polyfills help future-proof web applications. As browsers continue to evolve, developers can rely on polyfills to ensure that their applications remain functional and performant across different browser versions, even as the web standards landscape changes.
Tip
If you offer your SEO texts in several languages or localize them for different regions, your pages may be incorrectly classified as duplicate content. However, there is a simple solution to this in the form of hreflang. Our article explains what you need to bear in mind when using hreflang.
Read more about hreflangHow Do Polyfills Work?
Polyfills work by detecting whether a specific feature is supported by the user's browser. If the feature is missing, the polyfill provides an alternative implementation that mimics the behavior of that feature. This is usually done by checking the existence of a function or method in JavaScript and then providing a fallback if necessary.
Here’s an example: Let's say a developer uses the fetch() API to make network requests. If the user’s browser does not support fetch(), a polyfill can step in to provide the same functionality using older methods like XMLHttpRequest. The polyfill would look something like this:
javascript
if (!window.fetch) {
// Define fetch polyfill using XMLHttpRequest or another alternative
window.fetch = function() {
// Implementation of fetch using older methods
};
}
In this case, the polyfill checks if window.fetch is available. If not, it defines the fetch() method manually to provide similar functionality. The key is that polyfills are usually lightweight, and they only run when necessary, ensuring they do not slow down modern browsers that already support the feature.
Common Use Cases for Polyfills
Polyfills are used in a variety of situations where older browsers lack support for modern web technologies. Some of the most common use cases include:
CSS Features: Layout systems such as Flexbox and CSS Grid are not fully supported by some older browsers. Polyfills can ensure that these layouts work consistently, even when the browser doesn’t support them natively. ES6+ JavaScript Features: Modern JavaScript (ECMAScript 6 and above) introduces many useful features, such as promises, arrow functions, and destructuring. However, older browsers may not support these. Libraries like Babel, combined with polyfills like core-js, help developers use these features without worrying about compatibility. APIs and DOM Methods: Many modern APIs, such as fetch(), Promise, IntersectionObserver, and requestAnimationFrame, are commonly polyfilled to support older browsers. These APIs offer improved performance and functionality, but polyfills are needed to extend them to browsers that don’t yet have native support.
Difference Between Polyfills and Shims
The terms "polyfill" and "shim" are often used interchangeably, but they serve slightly different purposes:
Polyfill: A polyfill adds a feature that doesn’t exist in the browser at all. It fills in a missing piece of functionality and mimics the behavior of a feature as closely as possible. Polyfills are backward-compatible solutions designed to replicate the behavior of modern APIs. Shim: A shim typically refers to code that modifies or extends existing functionality in a browser. It doesn't necessarily aim to replicate missing features but rather adjusts or enhances the behavior of existing ones. For instance, a shim may override a native method with a custom implementation to fix bugs or offer more consistent behavior across browsers.
Implementing Polyfills in Your Projects
To implement polyfills, you have several options. The most common ways to add polyfills to your projects include:
Manual Inclusion: You can manually add specific polyfill scripts into your project. For example, if you know that certain browsers lack support for a feature, you can download and include the necessary polyfill in your project files or through a CDN. Babel and Core-js: Tools like Babel allow you to write modern JavaScript and automatically include polyfills for features that are not supported in older browsers. By using the @babel/preset-env and core-js together, Babel can determine which polyfills are needed based on the browsers you're targeting. Polyfill.io: Polyfill.io is a service that serves only the polyfills required by the user’s browser. Instead of loading all polyfills, it conditionally loads only what’s necessary, making it more efficient.
By using these methods, you can easily ensure that your web application supports a wide range of browsers while still taking advantage of modern web standards.
Popular Polyfill Libraries for Modern Browsers
Several libraries and services offer polyfills for modern browsers, enabling developers to implement modern features without sacrificing compatibility. Some of the most widely used polyfill libraries include:
core-js: One of the most comprehensive polyfill libraries, core-js provides polyfills for ECMAScript (ES6+), as well as features related to web APIs and global objects. It is often used with Babel to ensure full JavaScript compatibility. html5shiv: This polyfill allows older browsers, particularly Internet Explorer 6-8, to recognize and render HTML5 elements, enabling them to behave more like modern browsers. Polyfill.io: This service conditionally serves polyfills based on the requesting browser's capabilities, optimizing load times by only providing the necessary code for unsupported features. ES5-shim: ES5-shim provides compatibility for ECMAScript 5 features in older browsers, offering a smooth upgrade path for developers using older ECMAScript syntax.
Best Practices for Using Polyfills Efficiently
While polyfills are essential for ensuring compatibility across browsers, it’s important to implement them efficiently to avoid unnecessary performance overhead. Here are some best practices:
Selective Polyfilling: Only include the polyfills that are needed for the browsers you're targeting. Using tools like @babel/preset-env or services like Polyfill.io, you can ensure that only the required polyfills are loaded, reducing file size and improving page load times. Use Modernizr: Modernizr is a JavaScript library that detects which features a browser supports and loads polyfills dynamically as needed. This allows you to include polyfills conditionally, minimizing the amount of unnecessary code served to modern browsers. Optimize Performance: Be mindful of the performance impact of loading too many polyfills, especially for mobile users or those on slow networks. Always test your application’s performance in older browsers to ensure polyfills don’t negatively affect the user experience. Monitor Browser Support: Regularly review the browser support landscape using tools like caniuse.com. As older browsers become less common and new features gain wider support, you can remove unnecessary polyfills from your project to reduce bloat.
Understanding Polyfills: What They Are and How They Work | |
---|---|
1. What is a Polyfill? | A polyfill is a code solution that mimics web features not supported by a browser, ensuring compatibility with modern web standards. |
2. Why are Polyfills Important in Web Development? | Polyfills enable websites to function properly across different browsers, especially older ones, ensuring a consistent user experience. |
3. How Do Polyfills Work? | Polyfills detect unsupported features in a browser and provide fallback implementations, allowing websites to use modern functionality seamlessly. |
4. Common Use Cases for Polyfills | Polyfills are commonly used to support HTML5 elements, CSS features, modern JavaScript functions, and web APIs in older browsers. |
5. Difference Between Polyfills and Shims | Polyfills add missing features, while shims modify existing functionality to fix inconsistencies across browsers. |
6. Implementing Polyfills in Your Projects | Polyfills can be implemented manually, through libraries like core-js, or by using services like Polyfill.io to load only necessary features. |
7. Popular Polyfill Libraries for Modern Browsers | Core-js, html5shiv, Polyfill.io, and ES5-shim are some of the most popular libraries for implementing polyfills in web development projects. |
8. Best Practices for Using Polyfills Efficiently | Use selective polyfilling, tools like Modernizr, and monitor browser support to ensure efficient polyfill usage without negatively impacting performance. |
FAQs
How Does Tailgating Impact Corporate Security?
Tailgating occurs when an unauthorized individual gains access to a restricted area by closely following someone who is authorized, often bypassing security measures without detection. It is a significant physical security risk for businesses, especially in high-traffic areas.
What Situations Commonly Lead to Tailgating?
Tailgating is most common at office entry points, parking garages, and during large deliveries when multiple people enter at once. It frequently occurs when employees hold doors open for others, especially during rush hours or in poorly monitored areas.
What Security Risks Does Tailgating Pose to Companies?
Tailgating can lead to serious security risks such as data breaches, theft of assets, and damage to infrastructure. Unauthorized individuals can access sensitive areas, putting the company’s reputation, legal compliance, and financial stability at risk.
Why Is Physical Security Vulnerable to Tailgating?
By bypassing security measures, tailgating compromises the physical safety of a business. Intruders can tamper with equipment, steal valuable assets, or even pose direct threats to employees, potentially leading to costly consequences.
What Are Effective Ways to Prevent Tailgating?
Effective measures include access control systems (biometric scanners, two-factor authentication), physical barriers (turnstiles, mantraps), visitor management systems, surveillance cameras, and alarms to detect unauthorized access.
How Can Employees Help Prevent Tailgating?
Employee training is essential in preventing tailgating. Staff should be taught to follow access control policies and challenge unknown individuals who attempt to enter restricted areas, as well as report suspicious activity to security teams.