Sending Emails Made Easy with PHPMailer
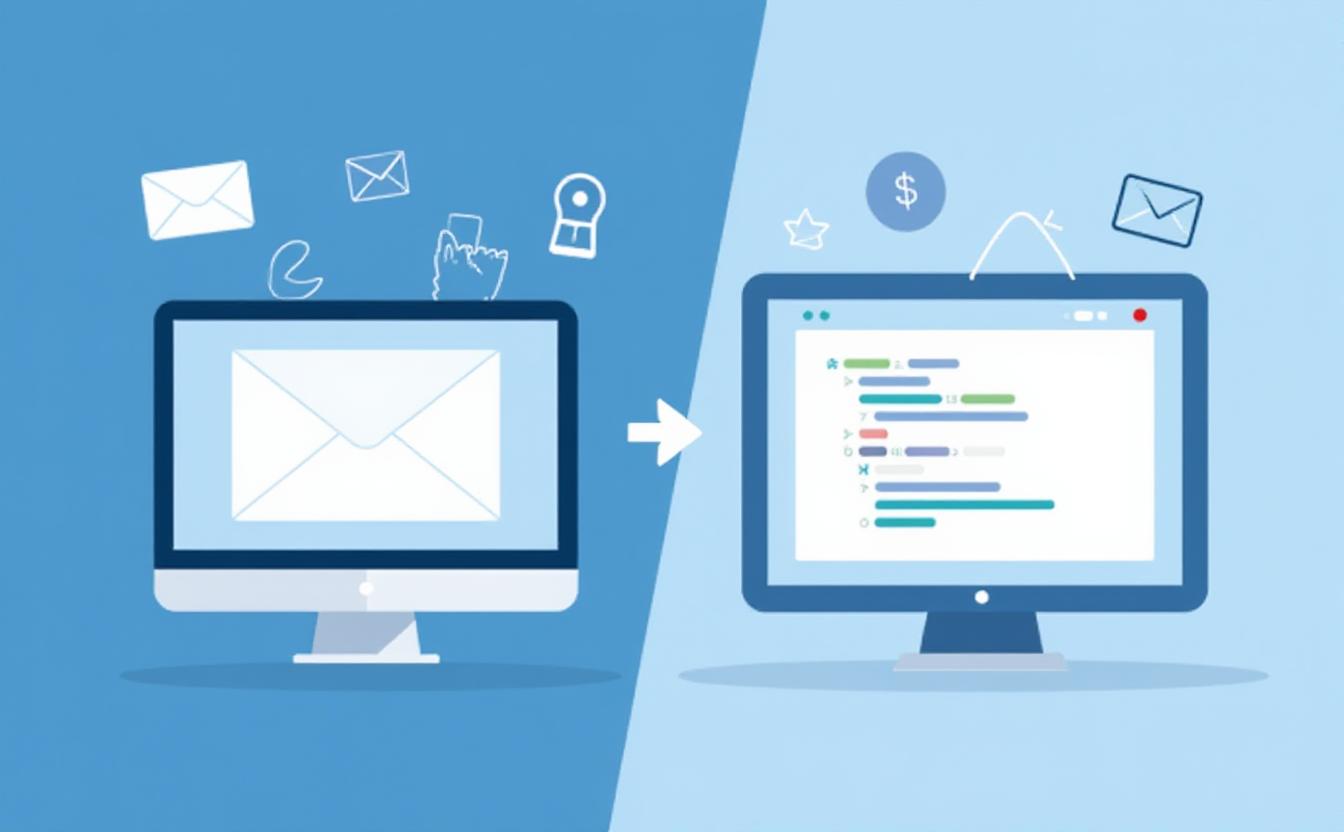
Sending Emails Made Easy with PHPMailer
Table of Contents
- Introduction to PHPMailer: What It Is and Why Use It
- Setting Up PHPMailer: Requirements and Installation
- Configuring PHPMailer for Your Email Server
- Sending Your First Email with PHPMailer: A Step-by-Step Guide
- Using SMTP with PHPMailer for Reliable Email Delivery
- Adding Attachments and HTML Content to Your Emails
- Handling Errors and Debugging in PHPMailer
- Best Practices for Securing and Optimizing PHPMailer
Introduction to PHPMailer: What It Is and Why Use It
PHPMailer is a popular open-source library in PHP that allows developers to send emails programmatically with ease. It simplifies the complexities of sending emails directly through PHP’s mail() function, offering a more reliable and flexible way to handle email deliveries. Whether you need to send basic emails or integrate more advanced features like attachments, HTML content, and SMTP servers, PHPMailer is a versatile and well-supported solution. Why Use PHPMailer? SMTP Support: PHPMailer supports Simple Mail Transfer Protocol (SMTP) for secure, reliable email delivery. HTML Emails: You can send rich HTML-based emails. Attachments: It allows for easy attachment of files. Error Handling: PHPMailer provides better error handling and debugging capabilities compared to PHP's mail() function. Security: It integrates encryption methods (SSL/TLS) for secure email sending.
Setting Up PHPMailer: Requirements and Installation
Before you can use PHPMailer, you need to install it in your PHP project. The process is straightforward, especially with Composer, PHP’s dependency manager. Requirements: PHP 5.5 or higher (PHPMailer works best with the latest versions of PHP). Composer (optional but recommended): To manage libraries efficiently. Installation: Using Composer: Open your terminal in the project directory and run: bash composer require phpmailer/phpmailer Manual Installation: Download the PHPMailer library from its GitHub repository. Include the required files in your project: php require 'path/to/PHPMailerAutoload.php';
Tip
If you offer your SEO texts in several languages or localize them for different regions, your pages may be incorrectly classified as duplicate content. However, there is a simple solution to this in the form of hreflang. Our article explains what you need to bear in mind when using hreflang.
Read more about hreflangConfiguring PHPMailer for Your Email Server
Once PHPMailer is installed, you need to configure it to connect with an email server, typically through SMTP, which is preferred for reliable and authenticated email sending.
Basic Configuration Steps:
Set the isSMTP() method to use an SMTP server.
Set the Host, Username, Password, and Port for your email server.
Example:
php
$mail = new PHPMailer();
$mail->isSMTP();
$mail->Host = 'smtp.example.com';
$mail->SMTPAuth = true;
$mail->Username = '[email protected]';
$mail->Password = 'your-email-password';
$mail->SMTPSecure = 'tls'; // Or 'ssl'
$mail->Port = 587;
Sending Your First Email with PHPMailer: A Step-by-Step Guide
Sending your first email with PHPMailer is simple once the library is configured. Follow these steps:
Create a PHPMailer instance:
php
$mail = new PHPMailer();
Set email parameters:
php
$mail->setFrom('[email protected]', 'Your Name');
$mail->addAddress('[email protected]', 'Recipient Name');
$mail->Subject = 'Test Email';
$mail->Body = 'This is a test email using PHPMailer!';
Send the email:
php
if(!$mail->send()) {
echo 'Message could not be sent. Mailer Error: ', $mail->ErrorInfo;
} else {
echo 'Message has been sent';
}
Using SMTP with PHPMailer for Reliable Email Delivery
SMTP (Simple Mail Transfer Protocol) is the standard protocol for sending emails across the internet. By configuring PHPMailer with an SMTP server, you ensure that your emails are authenticated and securely transmitted.
Steps to Use SMTP:
Enable SMTP Authentication:
php
$mail->SMTPAuth = true;
Set your SMTP credentials:
php
$mail->Host = 'smtp.example.com';
$mail->Username = '[email protected]';
$mail->Password = 'your-email-password';
Choose the correct SMTP security method:
php
$mail->SMTPSecure = 'tls'; // Or 'ssl' depending on your server.
Adding Attachments and HTML Content to Your Emails
PHPMailer allows you to send both plain text and HTML emails, as well as attach files to your email.
HTML Email Example:
This is an HTML email.
php
$mail->isHTML(true);
$mail->Body = '
Adding Attachments: You can easily attach files to your email by using the addAttachment() method:
Hello World!
php
$mail->addAttachment('/path/to/file.pdf');
Handling Errors and Debugging in PHPMailer
PHPMailer provides helpful error handling and debugging features, which can be invaluable when things go wrong.
Enable Debugging: To enable detailed error messages, use:
php
$mail->SMTPDebug = 2;
This will output the full SMTP conversation, making it easier to identify issues with email delivery.
Error Handling: After sending an email, you can check for errors by inspecting the $mail->ErrorInfo property:
php
if(!$mail->send()) {
echo 'Mailer Error: ' . $mail->ErrorInfo;
} else {
echo 'Message sent!';
}
Best Practices for Securing and Optimizing PHPMailer
To ensure the best performance and security when using PHPMailer, follow these best practices:
Use TLS or SSL: Always use encrypted connections to prevent sensitive information from being intercepted.
php
$mail->SMTPSecure = 'tls';
Limit Email Size: Keep attachments and content to a minimum to avoid deliverability issues.
Validate Email Addresses: Ensure email addresses are properly validated before sending to reduce bounce rates.
Use a Reputable SMTP Provider: Choose reliable SMTP services (e.g., Gmail, SendGrid) to improve email delivery success.
These best practices will help optimize the performance of PHPMailer and ensure your emails are sent securely and efficiently.
Sending Emails Made Easy with PHPMailer | |
---|---|
1. Introduction to PHPMailer | PHPMailer is a popular open-source library that simplifies the process of sending emails using PHP. It supports various protocols like SMTP and integrates easily with modern PHP applications. |
2. Why Use PHPMailer Over mail() Function? | PHPMailer offers advanced features like attachments, HTML content, and secure email delivery using SMTP, which the basic mail() function lacks. |
3. Setting Up PHPMailer | Setting up PHPMailer involves downloading the library via Composer, configuring SMTP settings, and writing the email sending logic in your PHP application. |
4. Sending HTML Emails | PHPMailer makes it easy to send rich HTML emails with inline images and attachments, which are essential for newsletters and marketing emails. |
5. Adding Attachments to Emails | You can easily add file attachments to emails using the `addAttachment` method in PHPMailer, supporting a variety of file formats. |
6. SMTP Authentication and Security | PHPMailer supports secure SMTP protocols like TLS and SSL, ensuring your emails are sent securely and authenticated with your email provider. |
7. Handling Email Errors | PHPMailer includes error handling mechanisms that allow you to track and manage issues like failed emails or server connection problems. |
8. Integrating PHPMailer with SMTP Providers | PHPMailer can integrate with popular SMTP providers like Gmail, SendGrid, and Amazon SES, offering flexible and reliable email delivery options. |
9. Best Practices for Sending Emails | Following best practices such as using proper authentication, including unsubscribe links, and sending emails at optimal times can improve your email delivery rates. |
10. Advanced Features of PHPMailer | PHPMailer offers advanced features such as DKIM signing, custom headers, and support for multiple recipients, making it versatile for complex email campaigns. |
FAQs
What is PHPMailer?
PHPMailer is a PHP library designed for sending emails efficiently. It offers advanced features like SMTP support, attachments, and HTML formatting, making it a more robust solution than the built-in PHP mail() function.
How do you install PHPMailer?
PHPMailer can be installed using Composer, a dependency manager for PHP. Simply run the command composer require phpmailer/phpmailer
in your project directory, and you’ll be ready to integrate it into your application.
What are the benefits of using PHPMailer over mail()?
PHPMailer provides secure email transmission, supports SMTP authentication, and enables sending attachments and HTML content. It also helps manage email errors and is widely supported with good documentation.
How do you send an email with attachments using PHPMailer?
You can send attachments using the addAttachment()
method in PHPMailer. This method allows you to attach various file types to your email by specifying the file path.
Can PHPMailer send HTML formatted emails?
Yes, PHPMailer allows you to send HTML formatted emails easily. You can set the isHTML(true)
method to send HTML emails, which is useful for newsletters and marketing purposes.
What security features does PHPMailer offer?
PHPMailer supports secure SMTP connections using SSL or TLS encryption, ensuring that your email transmission is protected from eavesdropping or tampering.
How do you troubleshoot PHPMailer errors?
PHPMailer provides detailed error messages when issues arise. By enabling error reporting using $mail->SMTPDebug = 2;
, you can view detailed debugging information to help troubleshoot issues with email delivery.