Magento 2.4.7 Checkout estimation.js Mixin – Complete Guide
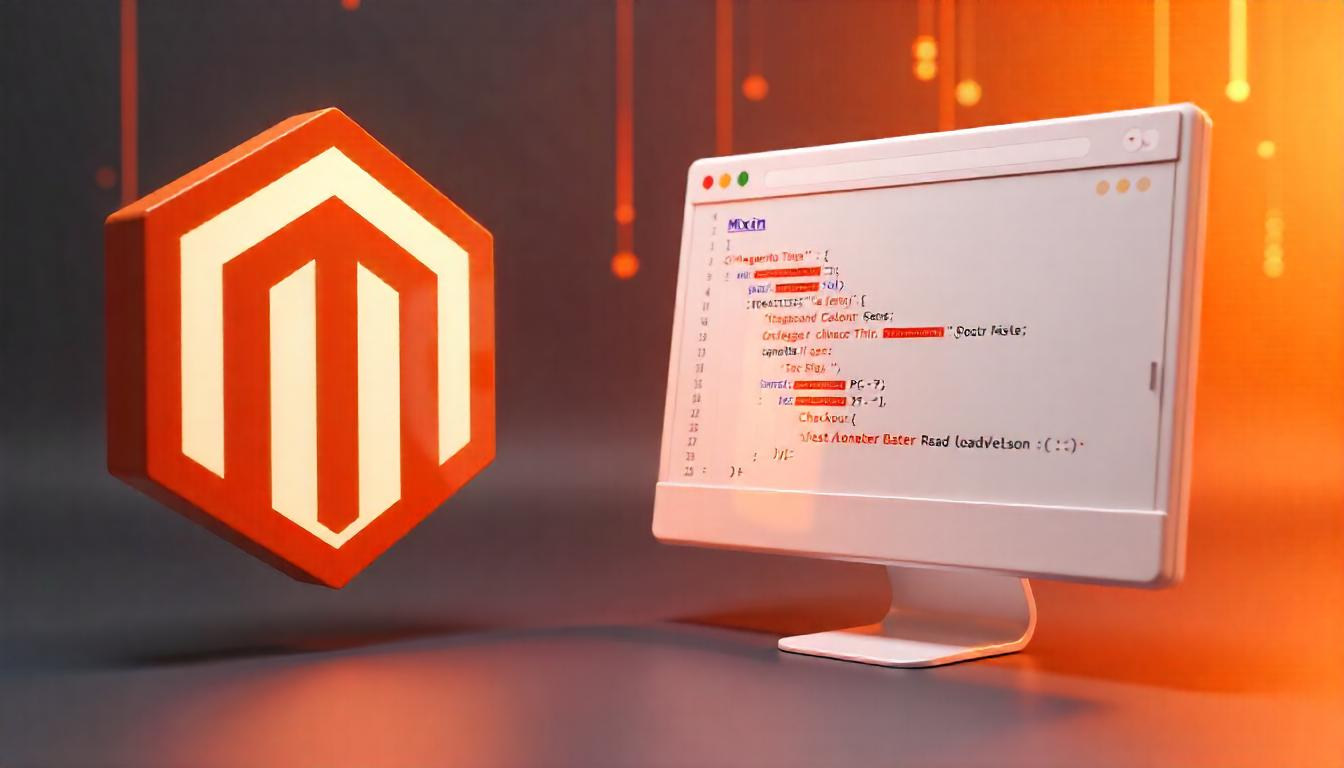
Magento 2.4.7 Checkout estimation.js Mixin – Complete Guide
Magento's checkout process heavily relies on JavaScript components like estimation.js to manage cart estimations and sidebar behavior. Instead of modifying core files (which is a bad practice), use a JavaScript mixin to safely extend or override native logic.
Table Of Content
Understanding estimation.js in Magento 2.4.7
What Is Magento_Checkout/js/view/estimation?
estimation.js
is a JavaScript component located within the Magento_Checkout module. It is responsible for managing the visibility and behavior of the shipping cost estimation sidebar, which appears during the shipping step of the checkout process. This sidebar helps users preview shipping options, prices, and estimated delivery timelines before placing an order.
In Magento 2, this component works closely with Knockout.js observables to update the checkout UI dynamically, based on changes in customer input like shipping address or cart items.
Purpose of Customizing estimation.js
Sometimes, the default estimation behavior doesn’t meet specific business needs. For example, you may want to:
- Hide the estimation panel for certain customer groups
- Show additional messages or promotions inside the sidebar
- Modify the logic that determines when the sidebar is visible
- Trigger events when estimation is calculated
Magento provides a safe and upgrade-proof way to customize this file using JavaScript mixins.
Why Use a JavaScript Mixin?
Mixins allow you to extend or modify existing JavaScript components without overriding the original core files. This is especially important in Magento 2 where core files should never be edited directly to preserve upgradability.
With a mixin applied to estimation.js
, you can:
- Inject new observables or computed properties into the component
- Execute custom logic before or after existing methods
- Completely override certain methods while leaving others intact
- Add new data sources or validation layers
Real-World Use Cases for estimation.js
Mixins
Use Case | Objective |
---|---|
Show Custom Delivery Message | Display a location-based notice such as "Delivery times may vary." |
Geo-IP Based Estimation Logic | Hide estimation unless the user is from a specific region or country. |
Cart-Based Messaging | Show an alert if cart total is below a shipping threshold. |
B2B/Wholesale Customization | Apply different estimation rules for logged-in wholesale customers. |
Promotion or Discount Reminder | Inject a custom promo banner into the sidebar during estimation. |
Where the File Is Located
The JavaScript component path is:
vendor/magento/module-checkout/view/frontend/web/js/view/estimation.js
To apply a mixin, you typically create your own module and override it using requirejs-config.js
.
Pro Tips for Checkout Customization
Tip | Insight |
---|---|
Avoid editing core files | Always use mixins or layout XML to make modifications. |
Use observables wisely | Leverage Knockout.js to keep UI reactive without full page reloads. |
Isolate logic by condition | Keep your mixin logic clean and specific to the needed functionality. |
Test in guest and logged-in mode | Checkout behaves differently based on session type—test both. |
Magento 2 Mixin Folder Structure for estimation.js Customization
When customizing Magento's checkout behavior—especially the shipping estimation sidebar—it's important to use a mixin rather than modifying core files. Magento 2 supports JavaScript mixins via RequireJS and Knockout.js. Below is a detailed folder structure and explanation to help implement a safe and upgrade-friendly customization.
Project Directory Layout
Emmo/
└── Estimation/
├── etc/
│ └── module.xml
├── view/
│ └── frontend/
│ ├── requirejs-config.js
│ └── web/
│ └── js/
│ └── view/
│ └── estimation-mixin.js
└── registration.php
Folder and File Descriptions
Path | Purpose |
---|---|
Emmo/Estimation/ |
Root directory for the custom module. Follows PSR-4 naming conventions. |
etc/module.xml |
Declares the module name and version. Required for module registration. |
registration.php |
Registers the module with the Magento framework. |
view/frontend/ |
Contains all frontend-specific files, including JavaScript mixins and configuration. |
view/frontend/requirejs-config.js |
Declares the mixin and maps the core estimation.js file to the new mixin logic. |
view/frontend/web/js/view/estimation-mixin.js |
The custom JavaScript file where the mixin logic resides. Used to override or extend default Magento_Checkout/js/view/estimation . |
Additional Developer Notes:
Best Practices:
- Never edit core files. Always use mixins or plugins.
- Test changes in both guest and logged-in states. Checkout behavior may differ.
- Use
define()
andreturn function (target) {}
structure in your mixin for maximum compatibility. - Ensure
estimation-mixin.js
is only loaded when necessary to avoid performance bottlenecks.
Common Use Cases for estimation.js
Mixin:
- Custom delivery messages
- Region-based shipping logic
- B2B-specific shipping behaviors
- UI injection of promotional banners or alerts
Implementing a Custom Mixin for Shipping Estimation in Magento 2
In Magento 2, the checkout process allows you to estimate shipping costs based on the items in the cart and the user's location. Sometimes, you might need to customize the shipping estimation process to meet specific business needs. For example, you could modify the display of the shipping estimation sidebar, add custom logic for different types of customers, or include additional variables in the estimation.
To achieve this, you can use a mixin, which is a powerful feature in Magento's frontend architecture. Mixins allow you to extend or modify the behavior of existing JavaScript classes without changing the original files. In this guide, we will walk you through the steps required to create a custom mixin for the shipping estimation process during checkout.
Follow the steps below to implement the custom mixin for shipping estimation in your Magento 2 store.
Step 1: Create requirejs-config.js
Path: app/code/Emmo/Estimation/view/frontend/requirejs-config.js
In this step, you'll create a configuration file that defines the mixin. The mixin will extend the default Magento_Checkout/js/view/estimation
logic, allowing you to inject custom behavior into the checkout estimation view.
var config = {
config: {
mixins: {
'Magento_Checkout/js/view/estimation': {
'Emmo_Estimation/js/view/estimation-mixin': true
}
}
}
};
Explanation:
- This file tells Magento to use the
estimation-mixin.js
file to modify the default behavior of theMagento_Checkout/js/view/estimation
view.
Step 2: Create the Mixin File
Path:app/code/Emmo/Estimation/view/frontend/web/js/view/estimation-mixin.js
Here, you will define the custom logic for modifying the shipping estimation process. This mixin file extends the base estimation behavior by adding custom methods and variables.
define([
'jquery',
'Magento_Checkout/js/model/sidebar',
'ko'
], function ($, sidebarModel, ko) {
return function (Estimation) {
return Estimation.extend({
initialize: function () {
this._super();
// Initialization logic
console.log('Estimation mixin initialized');
return this;
showSidebar: function () {
console.log('Sidebar logic modified');
// Add your own logic here
sidebarModel.show();
isEstimating: ko.observable(false),
estimateShipping: function () {
this.isEstimating(true);
setTimeout(() => {
this.isEstimating(false);
console.log('Shipping estimated');
}, 1000);
});
};
});
Explanation:
initialize
method: This is called when the mixin is initialized. The this._super() ensures that the base class functionality is preserved.showSidebar
method: Custom logic for displaying the sidebar is injected here. You can customize this to modify the sidebar behavior during checkout.isEstimating
observable: Used to track the state of the shipping estimation process.estimateShipping
method: This simulates a shipping estimation process with a delay, setting isEstimating to true during the operation and false afterward.
Step 3: Register the Module
To make your custom module recognized by Magento, you need to register it.
File:registration.php
use Magento\Framework\Component\ComponentRegistrar;
ComponentRegistrar::register(
ComponentRegistrar::MODULE,
'Emmo_Estimation',
__DIR__
);
?>
Explanation:
- The
registration.php
file registers your custom module with Magento's module registry.
File: etc/module.xml
<?xml version="1.0" encoding="UTF-8" ?
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd" >
<module name="Emmo_Estimation" setup_version="1.0.0" />
</config>
Explanation:
- The
module.xml
file defines the module's name and version, which Magento uses during module setup and upgrade processes.
Developer Tips
Tip | Details |
---|---|
Use this._super() | To extend logic from the parent class, use this._super() instead of replacing methods entirely. |
Use Chrome DevTools | Check the browser's Developer Tools console to confirm if the mixin is being loaded correctly. This helps with troubleshooting issues. |
Clear cache and static content | After making changes, clear the Magento cache and static content using: php bin/magento cache:flush && php bin/magento setup:static-content:deploy. |
Always deploy JS changes | Whenever you make changes to JavaScript files, either run the deploy command or enable Magento’s developer mode to ensure changes are applied. |
These tips will help ensure your module integrates smoothly with Magento and functions as expected during the checkout process.
Real-World Applications of Estimation Mixin in Magento 2.4.7
The estimation mixin in Magento allows for a variety of enhancements that go beyond just customizing the shipping estimation. Below are some expanded and real-world use cases, each tailored for better functionality and user experience. These use cases can help you implement robust features that will improve the checkout process and increase overall site performance.
1. Loading State Logic
Objective: Implement a loading spinner or other visual indicators to show users that the system is processing their shipping estimation. This avoids confusion and enhances user experience, especially when there are external API calls involved in the estimation process.
Details:
- When users enter the shipping information or change the cart content, they should see a loading spinner while the system fetches or processes data.
- This loading indicator can be integrated into the mixin using observables.
- Ensures that users aren’t left wondering whether the system is working, which can help reduce cart abandonment.
2. Custom API Hooks
Objective: Enhance the estimation logic by injecting custom API calls for additional functionalities, such as fetching special shipping rates, calculating taxes based on customer location, or integrating with third-party fulfillment services.
Details:
- Magento allows you to inject custom API hooks into the checkout process to enrich the estimation information.
- For example, you can integrate with third-party APIs to get real-time shipping rates or offer customized delivery options.
- This feature could allow for API calls that check stock availability, provide coupon or discount integration, or pull in geo-location data for shipping calculations.
3. Sidebar Tracking for Analytics
Objective: Track when the estimation sidebar is shown or hidden to gather insights about user behavior and checkout flow. This information can be used to make data-driven decisions regarding design changes, content placements, or call-to-action elements.
Details:
- By integrating tracking functionality in the mixin, you can capture when the sidebar is visible, how long users interact with it, and whether they engage with the shipping estimation information.
- This data can be sent to analytics platforms like Google Analytics or custom dashboards to monitor checkout behavior.
- This tracking can help determine if the sidebar is being utilized effectively or if users are skipping over important information.
4. UX Enhancements During Checkout Estimation
Objective: Improve user experience during the checkout estimation phase, where users often make critical decisions related to shipping options. This can be done by simplifying the flow, offering better feedback, or making the interface more intuitive.
Details
- One potential enhancement is to dynamically update the available shipping methods based on the user’s selected region or cart contents.
- Another approach might involve improving the design by incorporating features like tooltips, more prominent buttons, or collapsible sections to declutter the UI and make it easier to navigate.
- Making the checkout process more intuitive can lead to higher conversion rates by removing friction points during the estimation step.
5. Customizing Shipping Options Based on Cart Content
Objective: Modify available shipping methods dynamically based on the contents of the cart or user preferences. This could involve hiding specific shipping methods for certain products or offering special delivery options for high-value items.
Details:
- For example, you could restrict certain shipping methods for oversized products or fragile items, offering more expensive, secure shipping instead.
- This feature would enhance UX by only presenting the most relevant and available shipping methods to the customer, reducing decision fatigue and optimizing the checkout flow.
6. Real-Time Shipping Rates Based on Customer Location
Objective: Provide customers with real-time shipping rates based on their entered address or geo-location. This could dynamically update the available shipping methods without requiring the customer to refresh the page.
Details:
- By leveraging the estimation mixin, you can integrate third-party shipping APIs to pull in real-time rates and shipping times.
- This improves the customer experience by offering more accurate and timely shipping estimates, ensuring that customers are aware of their costs and delivery options before they proceed with payment.
7. Mobile-Optimized Estimation Interface
Objective: Create a mobile-friendly estimation interface that dynamically adjusts based on screen size, making the estimation process as smooth and responsive as possible on mobile devices.
Details:
- Responsive design ensures that customers shopping from mobile devices can easily view, interact with, and use the shipping estimation sidebar.
- You might consider incorporating touch gestures, larger buttons, or swipe functionality for smoother navigation.
8. Integration with Loyalty Programs
Objective: Integrate shipping estimation with loyalty programs to show users potential discounts based on their membership status or points balance.
Details:
- Customers can be notified of discounts or free shipping eligibility directly in the shipping estimation process.
- This creates an incentive for customers to engage with loyalty programs and can help increase customer retention rates.
Summary Table of Use Cases
Use Case | Objective | Benefit |
---|---|---|
Loading State Logic | Display a loading spinner while fetching shipping estimates or processing API calls. | Reduces user uncertainty and improves experience during checkout. |
Custom API Hooks | Inject custom API calls to fetch external shipping data or calculate custom delivery options. | Extends Magento’s default functionality with external services. |
Sidebar Tracking | Track when the estimation sidebar is shown or hidden for analytics purposes. | Provides insights to improve user engagement and the checkout process. |
UX Enhancements | Improve user experience during the shipping estimation process. | Increases conversion rates and simplifies user decision-making. |
Customizing Shipping Options | Dynamically adjust shipping methods based on cart contents or user preferences. | Reduces clutter and presents relevant options to the user. |
Real-Time Shipping Rates | Fetch live shipping rates based on user location and cart contents. | Provides accurate and transparent shipping costs. |
Mobile-Optimized Interface | Optimize the estimation interface for mobile devices. | Enhances accessibility and ease of use on smaller screens. |
Integration with Loyalty Programs | Display shipping discounts based on loyalty program status. | Encourages loyalty program participation and enhances retention. |
By leveraging these real-world use cases, you can enhance the checkout experience in Magento 2.4.7 and provide more value to both the business and the customer. Each customization not only improves the functionality of the checkout process but also adds flexibility to adapt to different business needs and customer expectations.
Verifying Your Estimation Mixin Integration
Once you’ve implemented your custom mixin for Magento_Checkout/js/view/estimation
, it's crucial to verify that it functions as intended. Proper validation ensures smooth checkout UX, fewer errors, and effective integration of your custom logic.
Step-by-Step Verification Process
1. Enable Developer Mode
Before testing, make sure Magento is in Developer Mode. This allows real-time JS updates and detailed error reporting
Run the following command if needed:
php bin/magento deploy:mode:set developer
2. Open Your Browser Developer Tools
Launch your preferred browser (e.g., Chrome, Firefox).
Open the Developer Console (F12 or Ctrl+Shift+I)
and go to the Console tab.
3. Reload the Checkout Page
Navigate to your checkout page where estimation.js
is used.
Refresh the page with the console open to see initialization logs and console messages.
4. Look for Custom Initialization Message
Check if the following message is visible in the console:
Estimation mixin initialized
This indicates that your mixin has successfully overridden or extended the original estimation module.
5. Test Modified Methods and UI Behavior
Try interacting with the shipping estimation section (e.g., selecting a shipping address, changing delivery method).
Check if custom methods like showSidebar
or estimateShipping()
execute as expected.
You can also add console logs within each function to trace execution.
6. Validate Observables and States
If you've introduced custom observables like isEstimating
, check their state transitions.
Example:
- Add
console.log(this.isEstimating())
inside your custom logic. - Confirm that it reflects the correct boolean status during the estimation process.
Advanced Validation Tips
Technique | Description |
---|---|
Use Chrome DevTools Breakpoints | Pause JS execution in real-time and inspect the flow of execution. |
Use console.table() |
Output structured arrays/objects like shipping rates in a readable format. |
Simulate Multiple Scenarios | Test as both guest and logged-in user with different shipping methods. |
Monitor Network Requests | Check XHR or Fetch calls to ensure APIs respond correctly. |
Test with Real and Mock Data | Simulate API failures, timeouts, and empty results for robust coverage. |
Validating the estimation mixin is not just about checking console logs. It’s about ensuring complete integration with Magento’s checkout process, accurate display of estimation data, and consistency across user roles, devices, and browsers. By following a structured verification checklist, you can confidently ship a robust and scalable checkout customization.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Customizing the checkout experience in Magento 2.4.7 is essential for improving performance, user experience, and aligning with unique business needs. Using JavaScript mixins—especially for core components like Magento_Checkout/js/view/estimation—is the most efficient and upgrade-safe method to extend default behavior without modifying core files.
In this guide, we explored how to:
- Properly register and structure a Magento 2 module for checkout customization
- Set up a RequireJS mixin targeting
estimation.js
- Add or override methods like
showSidebar
and introduce new observables - Apply real-world enhancements and debugging tips
- Keep your changes modular and aligned with Magento’s best practices
By following this approach, you ensure that your checkout enhancements are flexible, maintainable, and fully compatible with future Magento updates. Whether you're adding loaders, analytics, or custom API triggers—mixins give you full control over the checkout frontend logic.
FAQs
What is a JavaScript mixin in Magento 2?
A JavaScript mixin in Magento 2 is a way to extend or override core JS components without modifying the original file, ensuring upgrade-safe customizations.
Which component does the estimation mixin target?
It targets the Magento_Checkout/js/view/estimation
component, which is responsible for controlling the shipping estimation sidebar in the checkout.
Where should I place the requirejs-config.js file for a mixin?
You should place it under view/frontend/requirejs-config.js
inside your custom module directory.
What is the purpose of using mixins for estimation.js?
Mixins allow you to add or override logic such as showing/hiding the sidebar, triggering custom events, or interacting with observables during the checkout estimation process.
Can I override multiple methods in estimation.js using a mixin?
Yes, you can override or extend as many methods as needed within the mixin, as long as you return the extended component correctly.
Is it safe to use mixins in production?
Yes, mixins are Magento’s recommended way to customize frontend components safely and efficiently, especially in production environments.
How do I confirm if my mixin is working?
Use browser developer tools to check for console logs or method overrides. You should see custom messages like "Estimation mixin initialized" if set correctly.
Do I need to use this._super()
in mixins?
Yes, it is a best practice when extending existing methods to ensure original behavior is preserved along with your custom logic.
What is sidebarModel
used for in estimation-mixin.js?
sidebarModel
is a Magento model that controls the visibility and data of the mini cart sidebar, which can be used in your mixin to manipulate checkout behavior.
Do I need to deploy static content after creating a mixin?
Yes, after creating or updating frontend JavaScript files, run php bin/magento setup:static-content:deploy
to reflect the changes on the frontend.
Can I use Knockout.js observables in my mixin?
Absolutely. You can use Knockout observables like ko.observable()
to track dynamic values and bind them to the UI.
Is it possible to use mixins for other checkout components?
Yes, you can use mixins to customize any JS component in Magento, including payment, shipping, totals, and form validators.
What mode should I use during development for JS mixins?
It's best to enable Developer Mode, which allows immediate reflection of JS changes and makes debugging easier.
How do I avoid conflicts when using mixins?
Make sure your mixin does not directly override essential logic unless intended, and test thoroughly. Also, namespace your mixin functions clearly.
Why should I avoid editing core Magento JS files?
Editing core files breaks Magento’s upgrade path and can cause issues during version updates. Mixins provide a safe alternative to modify behavior.