Magento 2.4.7 – Uninstall Module with Database Table Schema
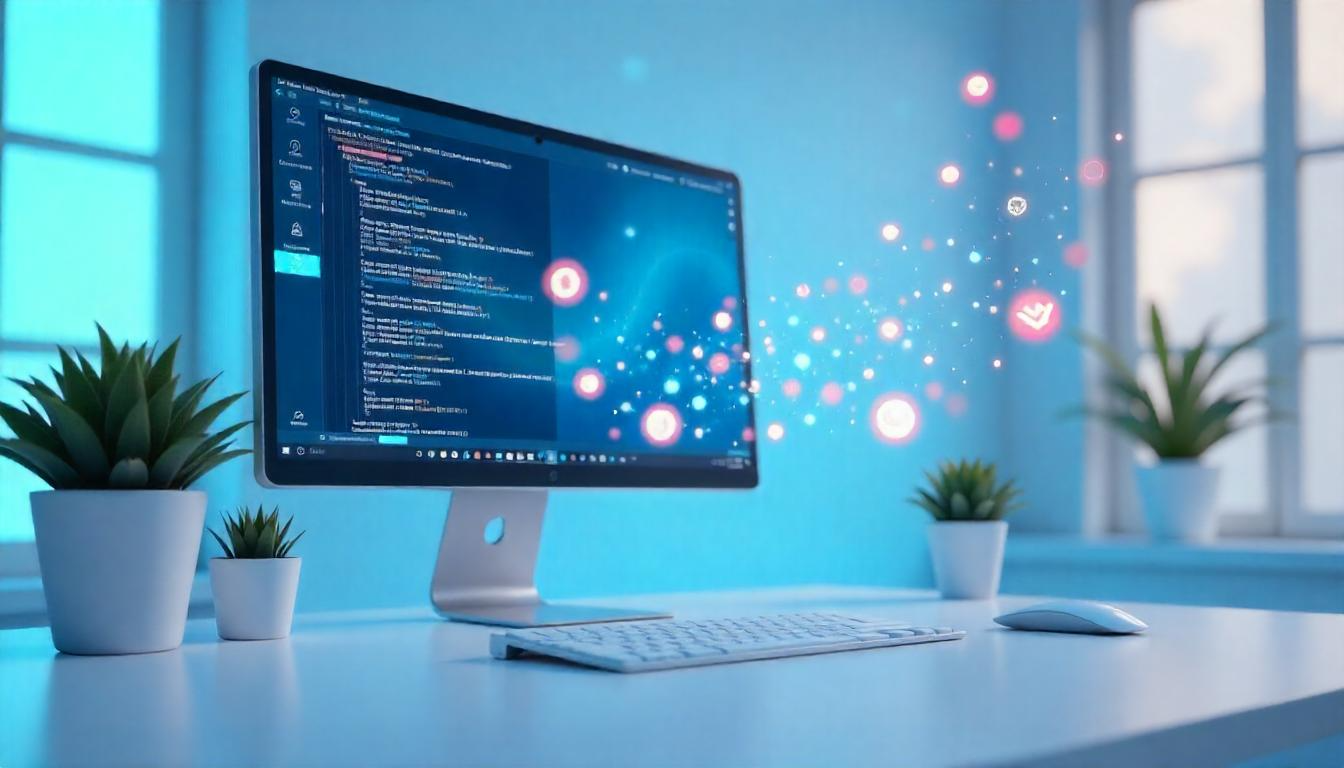
Magento 2.4.7 – Uninstall Module with Database Table Schema
Uninstalling a module in Magento 2 is more than just deleting a folder—especially when it involves cleaning up database tables, configurations, and static content. Magento 2.4.7 provides a powerful native command to handle this process, but there are rules and limitations you should know. In this guide, you'll learn how to properly uninstall a Magento 2 module along with its database schema, using best practices.
Table Of Content
Magento 2 Module Uninstallation: Readiness Checklist
Uninstalling a Magento 2 module properly requires more than just removing files. To ensure a clean and conflict-free removal—especially in production environments—follow these updated prerequisites and preparation steps.
1. Key Requirements Before Running bin/magento module:uninstall
Requirement | Description |
---|---|
Composer Installation |
The module must be installed via Composer and listed in your composer.json file under the require {} block. This ensures Magento can locate and remove all dependencies during uninstallation.
|
Defined Uninstall Script |
The module should contain a properly defined Uninstall.php class inside the Setup/ directory. This script handles database cleanup, such as dropping custom tables, removing config values, and other schema reversals.
|
Database Tables in Module |
The database schema modifications (like custom tables, columns, indexes, etc.) should have been introduced via InstallSchema , UpgradeSchema , or declarative schema XML to allow the uninstall script to reverse them properly.
|
Module is Enabled & Installed |
The module must be active (i.e., listed under bin/magento module:status ) and should not be in a disabled or corrupted state. Uninstallation won't proceed if the module is inactive or improperly registered.
|
2. Additional Best Practices Before Uninstalling
Step | Recommendation |
---|---|
Backup | Always take a full database and file backup before running the uninstall command, especially on production or staging environments. |
Data Migration | If the module stores any user data that you want to retain, export or migrate it before uninstalling. |
Dependency Check |
Check if other modules depend on the one you're trying to remove. Use composer depends or tools like modman to inspect relationships.
|
Maintenance Mode | Enable maintenance mode to prevent frontend or admin activity during uninstallation, reducing the risk of partial operations. |
Review Logs |
Monitor var/log/ and var/report/ after uninstalling to catch errors or unexpected issues.
|
3. When to Use Uninstall.php vs Declarative Schema Clean-up
Use Uninstall.php
when:
- You are working with Magento 2.4.x or older.
- You’ve implemented schema changes using
InstallSchema
orUpgradeSchema
.
Use declarative schema (in db_schema.xml
) for:
- Magento 2.3 and above.
- Automatic cleanup during module removal, especially for table and column deletions.
- More maintainable and rollback-safe schema management.
4. Command to Uninstall a Module
Run the command below only after verifying all the conditions above:
bin/magento module:uninstall Vendor_ModuleName
Use the --remove-data
flag if you want to trigger the Uninstall.php
script and fully wipe out module-related data:
bin/magento module:uninstall Vendor_ModuleName --remove-data
Advanced Magento 2 Module Uninstallation Guide
Magento 2’s CLI uninstallation command is powerful, but to truly uninstall a module cleanly and without side effects, you must understand the underlying processes and behaviors of both Composer-based and manually installed modules.
Understanding Magento 2 Uninstall Internals
When you run bin/magento module:uninstall
, Magento performs the following operations under the hood:
- Validates module status and registration
- Checks for an existing Uninstall.php script
- Calls
Uninstall.php::uninstall()
method (if exists) - Removes entries from
setup_module
table - Cleans related configuration and cache entries
- Optionally removes data and backs up resources
Extended CLI Options Reference Table
Option | Detailed Description |
---|---|
--remove-data | Executes Uninstall.php to drop schema, remove EAV attributes, or delete config values |
--backup-code | Copies the entire Magento codebase to var/backups/ before any change |
--backup-media | Backs up pub/media and related media assets to var/backups/media/ |
--backup-db | Dumps the full database before uninstallation using mysqldump or Magento's DB abstraction layer |
--clear-static-content | Removes stale frontend JS/CSS files that reference the module |
--force-remove | (Newer Magento versions) Forcibly unregister the module if inconsistencies exist |
--non-interactive | Skips confirmation prompts, suitable for scripting |
Uninstalling Declarative vs. InstallSchema Modules
Magento 2 supports two schema strategies:
Declarative Schema Modules (Magento 2.4+)
- Defined using
db_schema.xml
- Dropping schema via
Uninstall.php
is optional ifonSchemaUninstall="drop"
is declared
Install/UpgradeSchema Modules (Legacy)
- Must manually reverse changes inside Uninstall.php
Key Differences Between Composer and app/code Modules
Aspect | Composer Module | app/code Module |
---|---|---|
Installation Location | vendor/vendorname/modulename |
app/code/Vendor/Module |
Removal Support | Fully supported via CLI uninstall | Requires manual file deletion |
Dependency Detection | Uses composer.lock for dependency tracking |
No dependency awareness |
Version Management | Controlled via composer.json and tags |
Managed manually by updating the codebase |
Reinstall After Removal | Reinstallable with composer require |
Must re-copy the files manually |
Real-World Scenarios and Tips
Scenario 1: Stuck Module in Disabled State
- Symptom: Uninstall fails with "Module is not enabled"
- Solution:
bin/magento module:enable Vendor_Module
bin/magento module:uninstall Vendor_Module --remove-data
Scenario 2: Module Crashes Setup Upgrade
- Symptom:
setup:upgrade
breaks due to missing files - Solution: Manually remove entries from
setup_module
table and re-run setup after file deletion
Scenario 3: Configuration Leftovers
- Use this SQL to find leftover config:
SELECT * FROM core_config_data WHERE path LIKE '%modulename%';
- Clean up if any entries remain:
DELETE FROM core_config_data WHERE path LIKE '%modulename%';
Developer Best Practices for Future-Proof Module Removal
- Always include
Uninstall.php
for safe cleanup - Use declarative schema where possible and declare
onSchemaUninstall="drop"
- Avoid storing critical shared data inside module-specific tables
- Provide admin options to export user-generated content before uninstall
Magento 2’s uninstallation process isn't just about running a single command. It involves careful handling of data, dependencies, cache, and legacy schema logic. Understanding these moving parts ensures you don’t break your environment, lose customer data, or leave behind performance-impacting leftovers.
Complete Guide to Removing Module-Specific Database Tables in Magento 2
Removing database tables safely and completely during a module uninstall is essential for maintaining data hygiene and avoiding residual clutter in your Magento 2 database.
Why Table Cleanup Is Important
When uninstalling a module, especially one that adds custom tables or modifies the schema, it's important to clean up all associated data to:
- Prevent leftover data from affecting performance
- Avoid conflicts during future module reinstalls
- Maintain a clean and optimized Magento database
- Ensure security by removing potentially sensitive data
Step 1: Confirm the Module Is Installed via Composer
Only Composer-installed modules support automated cleanup through the module:uninstall
command with the --remove-data
flag. Verify the module in your root composer.json
:
"require": {
"vendor/module-name": "^1.0"
}
This ensures Magento will properly register and execute the module’s Uninstall.php
script.
Step 2: Create an Uninstall Script to Drop Tables
To handle table removal, you need to implement the UninstallInterface
inside the module.
File Path:app/code/Vendor/ModuleName/Setup/Uninstall.php
Sample Implementation:
<?php declare(strict_types=1);
namespace Vendor\ModuleName\Setup;
use Magento\Framework\Setup\UninstallInterface;
use Magento\Framework\Setup\SchemaSetupInterface;
use Magento\Framework\Setup\ModuleContextInterface;
class Uninstall implements UninstallInterface
{
public function uninstall(SchemaSetupInterface $setup, ModuleContextInterface $context): void
{
$setup->startSetup();
// Drop custom tables
$setup->getConnection()->dropTable($setup->getTable('vendor_custom_table'));
$setup->getConnection()->dropTable($setup->getTable('vendor_logs'));
$setup->getConnection()->dropTable($setup->getTable('vendor_reporting'));
$setup->endSetup();
}
}
Step 3: Use --remove-data Option During Uninstall
To invoke the uninstall script that drops the tables, use:
bin/magento module:uninstall Vendor_ModuleName --remove-data
Sample Table Structure (for Reference)
Table Name | Description |
---|---|
vendor_custom_table | Stores custom entity records for the module |
vendor_logs | Stores system logs, event records, or audit entries |
vendor_reporting | Stores data for analytics or export reports |
Best Practices and Tips
Replace Placeholder Tables
Make sure to replace vendor_custom_table
, vendor_logs
, etc., with real table names used in your module. You can find these in:
InstallSchema.php
UpgradeSchema.php
db_schema.xml
Drop Conditional Tables
If certain tables are created conditionally (e.g., based on config), check if the table exists before dropping:
if ($setup->getConnection()->isTableExists($setup->getTable('vendor_custom_table'))) {
$setup->getConnection()->dropTable($setup->getTable('vendor_custom_table'));
}
Avoid Data Loss in Shared Tables
Do not drop core Magento tables or shared third-party tables unless you are sure no other module depends on them.
Additional Cleanup Steps (Optional)
Besides dropping tables, you can also clean:
- Custom configuration values (
core_config_data
) - EAV attributes
- URL rewrites
- Custom indexes or foreign keys
This helps you revert the database to a pre-installation state.
Magento 2 Uninstall Process Reference (with Core Module Example)
Magento core modules such as Temando_Shipping
serve as practical examples of proper uninstallation implementation. Specifically, the core module defines a well-structured Uninstall.php
script located at:
vendor/magento/module-temando-shipping/Setup/Uninstall.php
This class implements UninstallInterface
and handles removal of database tables, configuration values, and EAV attributes. It provides a reliable model for custom module developers to follow.
Consequences of Skipping --remove-data During Uninstallation
If you uninstall a module without using the --remove-data
flag, the module will be unregistered from Magento, but several remnants will remain in your system. This can cause data inconsistencies, configuration pollution, and performance issues later on.
Here’s what typically happens when you skip --remove-data
:
Effect | Explanation |
---|---|
Database Tables Remain | All custom tables created by the module will persist in the database. |
Orphaned Configuration | Configuration settings in core_config_data may continue to exist. |
Admin UI Artifacts | Custom admin sections or fields may still appear unless manually removed. |
EAV Attributes Persist | Any custom EAV attributes (product, customer, etc.) may still exist. |
Indexing or Caching Issues | Stale data might affect indexers and result in caching anomalies. |
Best Practice: Implement a Full Uninstall.php Script
To avoid leftover data or broken admin UIs, always define and register a proper Uninstall.php
script. This script should:
- Drop all custom tables
- Remove configuration keys
- Delete any custom EAV attributes
- Unregister cron jobs or observers
- Clean up URL rewrites or custom logs
Final Uninstallation Readiness Checklist
Before running the uninstall command, confirm the following steps are completed to ensure a clean and safe uninstallation process:
Task | Status |
---|---|
Module is defined in composer.json | Yes |
Uninstall.php exists in the module’s Setup directory | Yes |
All custom tables and configurations are included in Uninstall.php | Yes |
You plan to run the uninstall command with --remove-data | Yes |
A full backup of code and database exists | Recommended |
The module is not a dependency for other active modules | Recommended |
Maintenance mode is enabled during uninstall (on production) | Recommended |
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Uninstalling a Magento 2 module with its database schema and associated data is a crucial part of module management, especially when you're working with Composer-based installations. By leveraging the powerful Magento CLI command module:uninstall along with a well-defined Uninstall.php script, you ensure that your module and its associated data are removed cleanly from the system.
The process not only clears up the codebase but also handles any database tables, schemas, and configurations tied to the module. While the module:uninstall command works seamlessly for Composer-managed modules, manual intervention may be necessary for modules installed directly through app/code.
By following the right steps, including creating a detailed uninstall script, verifying table names, and using the --remove-data option, you can safely and efficiently uninstall Magento modules without leaving unnecessary data or configurations behind. Always back up your data and configurations to prevent any accidental loss during this process.
This approach ensures your Magento store remains optimized and free from unused or obsolete modules, contributing to smoother performance and easier maintenance. Whether you're managing a large eCommerce store or just cleaning up after a testing environment, this method provides a solid foundation for module uninstallation in Magento 2.4.7.
With these steps, you'll be able to uninstall modules effectively, remove all traces from the database, and maintain a clean and efficient Magento environment.
FAQs
What is the Magento 2 uninstall command?
The `bin/magento module:uninstall` command is used to remove a module from the Magento system, including its database schema and data, if specified with the `--remove-data` option.
How does the `module:uninstall` command work in Magento 2?
The `module:uninstall` command removes the module's code, tables, and data from the database. It works only for modules installed using Composer and with the `--remove-data` flag enabled, it will also remove the associated database schema.
What happens if I uninstall a module that is not installed via Composer?
If a module is installed manually (not via Composer), the `module:uninstall` command will not remove it. You will need to delete the module folder manually from `app/code` and handle database cleanup yourself.
What is an Uninstall.php file in Magento 2?
The `Uninstall.php` file is a custom file you need to create in your module's `Setup` folder. It implements the `UninstallInterface` and defines how to remove any database tables, data, or configurations during the uninstallation process.
How do I remove database tables when uninstalling a module?
To remove database tables during module uninstallation, define the tables you want to drop in the `uninstall()` method of the `Uninstall.php` class, using the `$installer->getConnection()->dropTable()` method.
Do I need to manually clear static content during uninstallation?
If you want to remove static content during uninstallation, use the `--clear-static-content` option with the `module:uninstall` command. This will clean up static assets like CSS, JavaScript, and images.
What are the different ways to uninstall a Magento module?
You can uninstall a module via CLI using the `bin/magento module:uninstall` command, manually remove the module folder, or run the uninstall class for a Composer-based module with the necessary flags to remove code and data.
Can I remove multiple tables when uninstalling a module?
Yes, you can remove multiple tables by adding more `dropTable()` calls in the `uninstall()` method of the `Uninstall.php` class, one for each table you want to remove.
What is the `--remove-data` option in the `module:uninstall` command?
The `--remove-data` option ensures that all database-related data and schema associated with the module are removed during uninstallation, not just the module code.
Can I reinstall a module after uninstalling it in Magento 2?
Yes, after uninstalling a module, you can reinstall it using the `composer require` command (if it was installed via Composer) or by copying the module code back to the `app/code` directory and running the necessary setup commands.
What should I do if I need to keep module data while uninstalling it?
If you want to keep the module's data but remove the module itself, you can run the `module:uninstall` command without the `--remove-data` option, which will only remove the code, not the database entries.
What happens if I don't create an Uninstall.php class for my module?
If you don't create an `Uninstall.php` class for your module, Magento won't be able to clean up database tables, data, or other configurations during uninstallation, and you will need to remove them manually.
How can I check if a module was installed via Composer?
You can check the `composer.json` file in the root directory of your Magento project. If the module is listed under the `require` section, it was installed via Composer.
Is there a way to back up the module data before uninstalling?
Yes, you can use the `--backup-db` option with the `module:uninstall` command to create a backup of the module's database before removing it. You can also back up the module files manually.