How to Upload Files and Images Programmatically in Magento 2
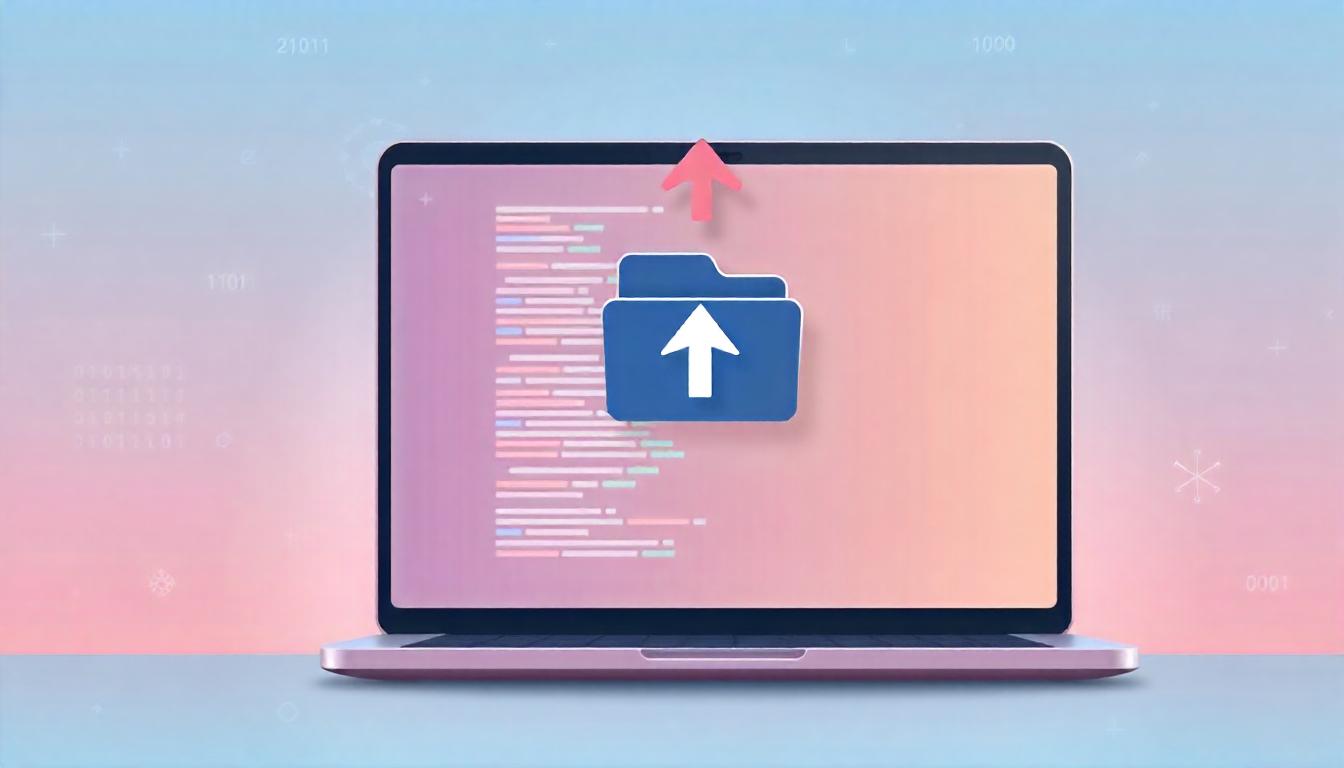
How to Upload Files and Images Programmatically in Magento 2
Uploading files such as product images is an essential task for any eCommerce store. Clear, detailed visuals help customers understand your products better and boost sales. In Magento 2, you can programmatically upload files using custom code, making the process flexible and efficient for specific business needs.
Table Of Content
Why Product Images Are Crucial
Product images significantly impact customer decision-making. For example, including multiple photos that show a product’s design, functionality, and dimensions can enhance the buyer’s confidence. These images act as digital sales tools, offering essential details in a format users trust.
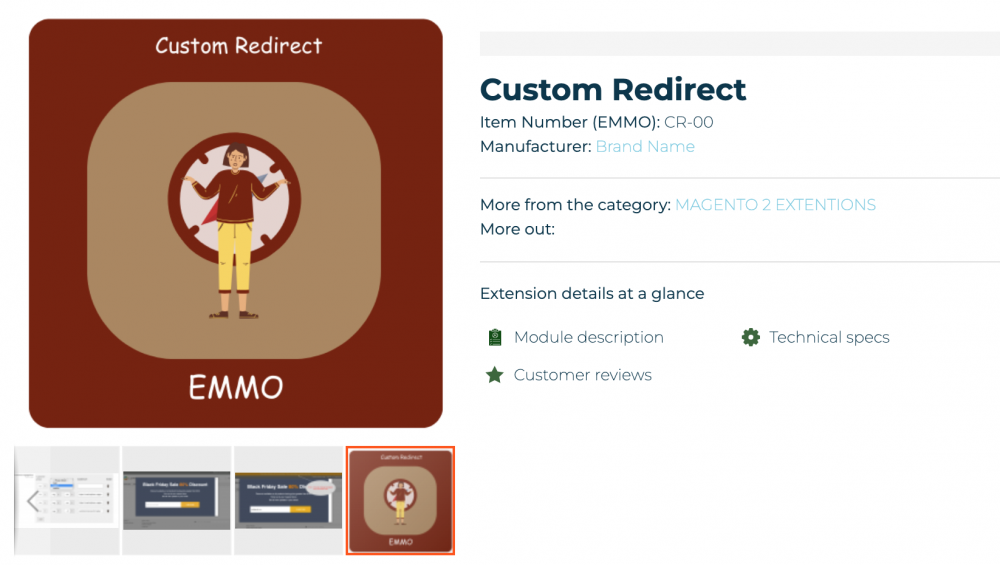
Programmatically Uploading Files in Magento 2
To upload files programmatically in Magento 2, follow these steps:
1. Set Up the Form
Create an HTML form with a file input field. Use enctype="multipart/form-data"
in the form tag to allow file uploads. For example:
HTML File Upload Form
<form method="post" enctype="multipart/form-data" action="{form-action-url}">
<input type="file" name="file" />
<button type="submit">Upload</button>
</form>
2. Create the Controller
Develop a controller to handle the file upload logic. This controller processes the incoming file, validates it, and stores it in a desired directory.
Here’s a basic structure for the controller:
Magento File Upload Controller
<?php
namespace Vendor\Module\Controller\Adminhtml\File;
use Magento\Backend\App\Action;
use Magento\Framework\App\Filesystem\DirectoryList;
use Magento\MediaStorage\Model\File\UploaderFactory;
class Upload extends Action
{
protected $uploaderFactory;
protected $fileSystem;
public function __construct(
Action\Context $context,
UploaderFactory $uploaderFactory,
Filesystem $fileSystem
)
{
parent::__construct($context);
$this->uploaderFactory = $uploaderFactory;
$this->fileSystem = $fileSystem;
}
public function execute()
{
try {
$uploader = $this->uploaderFactory->create(['fileId' => 'file']);
$uploader->setAllowedExtensions(['jpg', 'png', 'pdf']);
$uploader->setAllowCreateFolders(true);
$path = $this->fileSystem
->getDirectoryWrite(DirectoryList::MEDIA)
->getAbsolutePath('custom_files/');
$uploader->save($path);
$this->messageManager->addSuccessMessage('File uploaded successfully.');
} catch (\Exception $e) {
$this->messageManager->addErrorMessage($e->getMessage());
}
}
}
3. Destination Path
Set the upload directory using the DirectoryList
class. For example, save files to pub/media/custom_files/
.
4. Custom Validation (Optional)
Add validation callbacks to ensure file integrity and type safety. Use addValidateCallback
in the uploader.
5. File Handling in System Configuration
To upload files in the Magento configuration area, define a field in the system.xml
file with the type="file"
attribute. Configure the upload_dir
parameter to specify the file's storage path.
This approach is effective for uploading and processing files programmatically in Magento 2, allowing flexibility in handling files through forms or system configurations. For more details, you can explore examples from sources like emmo
Why Product Images and Files Matter in eCommerce
Product images and associated files significantly impact customer trust and purchase decisions. For example:
Image Type | Purpose |
---|---|
Close-up images | Showcase product details like texture or material. |
Multi-angle views | Provide a complete understanding of the product's look. |
User manuals (PDFs) | Help customers use the product correctly. |
Demo videos | Demonstrate functionality or use cases. |
Having a variety of visuals and documents ensures customers have all the information they need.
Benefits of Programmatic File Uploads
- Full control over the upload logic and destination.
- Ability to integrate additional validations or preprocessing steps.
- No reliance on external extensions, reducing dependencies.
Use Cases
- Adding custom attachments to products, such as warranty documents or installation guides.
- Uploading promotional banners or media files for marketing campaigns.
- Custom user-upload workflows in multi-vendor setups.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Uploading files programmatically in Magento 2 is an essential skill for developers looking to enhance their eCommerce stores with custom functionalities. Whether you're attaching detailed product manuals, uploading personalized customer files, or automating bulk uploads, programmatic solutions provide unparalleled flexibility and control.
This approach is particularly beneficial for complex scenarios, such as integrating file uploads into workflows, associating files with customer or product data, or adding advanced validations and access restrictions. By leveraging Magento’s robust file management architecture and adhering to best practices, developers can create efficient, scalable, and secure solutions.
For a seamless experience, always ensure proper permissions, implement validation mechanisms, and consider leveraging Magento extensions for tasks requiring less development effort. With the right balance of customization and optimization, programmatic file uploads can significantly enhance your Magento 2 store’s functionality and user experience.
FAQs
How do I upload a file programmatically in Magento 2?
To upload a file programmatically, use Magento's \Magento\Framework\File\UploaderFactory to handle file uploads and \Magento\Framework\Filesystem to manage directories. These components help validate, save, and store the file securely in your desired folder.
Where are uploaded files stored in Magento 2?
Uploaded files are typically stored in the pub/media
directory by default. You can customize the storage location programmatically using Magento's filesystem functions.
What file types can I upload in Magento 2?
By default, Magento 2 supports standard file types like JPG, PNG, PDF, and DOC. To upload other file types, update the allowed extensions in the configuration or validate them programmatically.
How do I validate file uploads in Magento 2?
Validation can be handled using Magento's uploader component by specifying allowed extensions, file size limits, and custom validation callbacks to ensure secure file uploads.
Can I restrict uploaded files to specific customer groups?
Yes, you can restrict uploaded files by associating them with customer group IDs. Use Magento's customer session to determine the user's group and control file access accordingly.
How do I display uploaded files on the frontend?
To display uploaded files, retrieve the file path from the database or product attribute, and use media_url
helpers to generate the URL for embedding or linking the file on the frontend.
What are common issues with file uploads in Magento 2?
Common issues include incorrect directory permissions, invalid file types, and large file sizes. These can be resolved by adjusting permissions, extending file validations, or increasing server upload limits.
Can I automate file uploads in Magento 2?
Yes, file uploads can be automated using cron jobs or custom scripts. This is useful for bulk uploading files like product catalogs, images, or price sheets on a regular schedule.
What permissions should I set for the upload directory?
Set the directory permissions to 775
for secure write access. Ensure ownership is correctly assigned to the web server user to avoid permission errors.
Is there an extension for easier file uploads in Magento 2?
Yes, you can use extensions like Magento 2 Product Attachments. These extensions provide user-friendly interfaces to upload and manage files without requiring custom coding.