Guide to create Guest Empty Cart quote programmatically in Magento 2.4.x
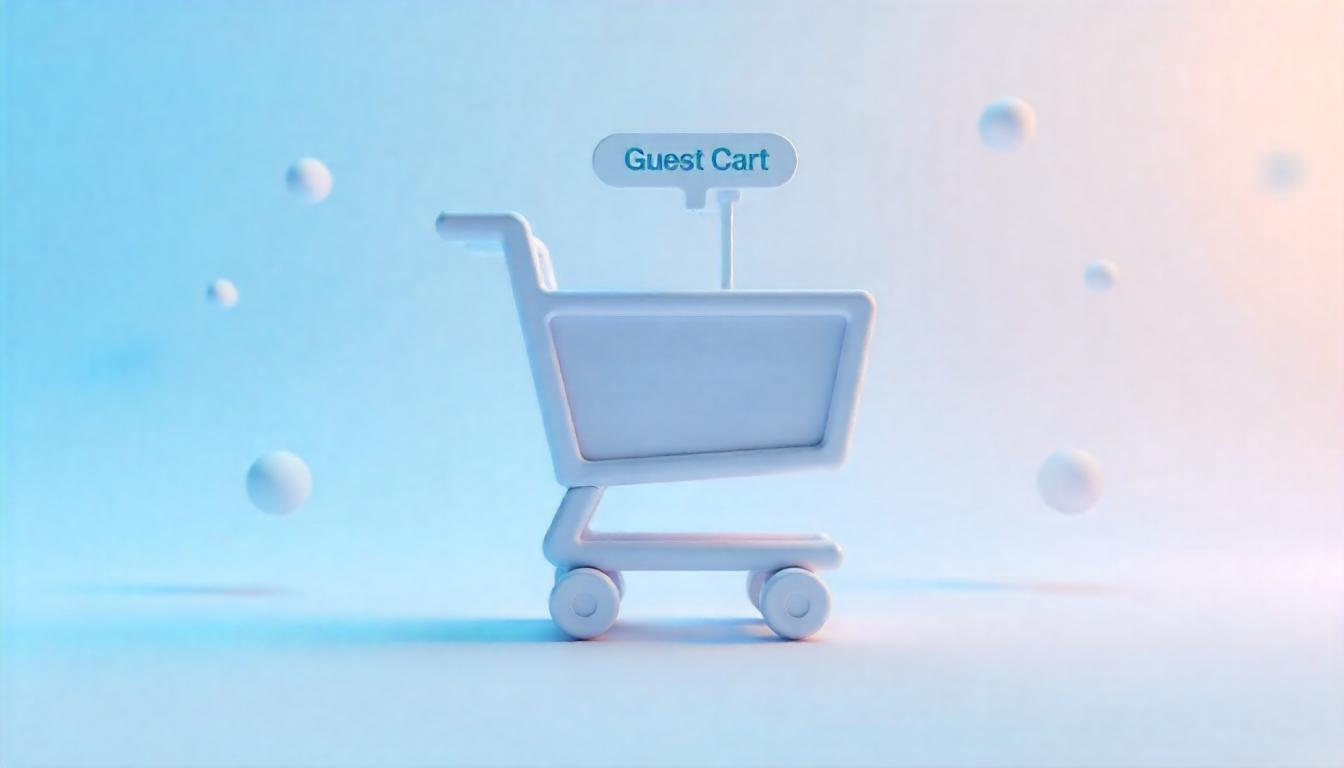
Guide to create Guest Empty Cart quote programmatically in Magento 2.4.x
Magento 2.4.7 provides a robust service contract for managing guest carts via the GuestCartManagementInterface. By calling its createEmptyCart() method, you can programmatically generate an empty quote (cart) for an anonymous user. This creates entries in the core quote tables (quote, quote_address, and quote_id_mask) and returns a masked cart ID that your front end or API clients can use to add items, configure shipping, or proceed to checkout.
Table Of Content
Guest Cart Management in Magento 2
In Magento 2, guest users—those not logged into an account—require a separate flow for cart creation. The platform addresses this through its Guest Cart Management Interface, a part of the Magento\Quote\Api\GuestCartManagementInterface
. This interface simplifies the process of generating a shopping cart (quote) for visitors who have not yet authenticated.
Rather than constructing quote objects manually or handling session-specific logic, a single call to the appropriate service will generate a new guest quote and return a masked cart ID. This unique identifier can be securely passed to frontend components or external systems.
Key Advantages of Magento's Guest Cart API
Benefit | Description |
---|---|
Simplified Workflow | Developers no longer need to manually instantiate quote models or manage session persistence for guests. One API call handles cart creation. |
Secure Cart Identification | Magento returns a masked (encoded) cart ID for guest sessions, protecting internal database references and preventing misuse. |
Frontend Compatibility | Works seamlessly with headless setups (e.g., PWA, React, Vue) and AJAX-based storefronts where real-time cart operations are required. |
Session Independence | Cart creation is not tied to PHP sessions, making it ideal for stateless or token-based architectures. |
REST and GraphQL Support | Both API formats support guest cart operations, making integration flexible across mobile apps and third-party services. |
Typical Use Cases for Guest Cart Initialization
1. Progressive Checkout
Allow users to begin shopping and adding items without requiring login, increasing engagement and reducing friction at early stages.
2. API-Based Frontends
Headless storefronts or SPAs that use React, Vue, or Angular often initialize the cart via API calls at the start of a session.
3. Third-Party Integrations
External platforms (e.g., voice assistants, chatbots, custom apps) can initialize a guest cart remotely using secure REST or GraphQL endpoints.
Behind the Scenes: How It Works
When createEmptyCart()
is called via the guest cart API, Magento:
- Initializes a new quote object flagged as
is_guest = true
. - Saves the quote in the database.
- Encodes the quote ID using a masking mechanism.
- Returns the masked ID, which can be used in subsequent cart updates (e.g., addItem, estimateShipping, applyCoupon).
Example API request (REST):
POST /V1/guest-carts
Response:
"6cf6b9bcb5e48f935b1d4b9c57e5e8cd"
This ID is then used in other guest cart endpoints like:
POST /V1/guest-carts/:cartId/items
GET /V1/guest-carts/:cartId/totals
Security and Best Practices
- Masked Cart ID: Never expose raw quote IDs. Use only the masked version returned by Magento.
- Token Separation: Avoid mixing guest cart operations with customer-authenticated APIs. Keep workflows isolated.
- Auto-Merge on Login: If configured, Magento can merge guest and customer carts upon login—review merge strategies to prevent data loss.
Magento 2's guest cart creation process is a robust, API-driven solution that enables smooth shopping experiences without login barriers. Whether you're building a custom checkout, integrating with mobile apps, or creating a headless frontend, this approach provides a secure and scalable foundation for guest user engagement.
Understanding Magento Guest Cart Architecture
In Magento 2, managing shopping cart functionality for guests is streamlined using specific interfaces and service classes. These components are vital for API-based checkout flows, headless storefronts, and mobile applications that need to create and handle carts without customer authentication.
Primary Interfaces and Classes for Guest Cart Management
The following are the core interfaces and classes involved in guest cart creation, management, and persistence:
Core Components
Interface / Class | Responsibility |
---|---|
GuestCartManagementInterface | Acts as the main interface for guest cart creation. It exposes the createEmptyCart() method that initializes a new quote for unauthenticated users. This service handles cart generation without requiring a customer login. |
CartManagementInterface | Used for creating and managing carts for logged-in customers. It includes methods for retrieving, merging, and modifying customer carts based on session or ID. |
QuoteRepositoryInterface | Provides persistence for quote (cart) entities. It allows developers to save, load, and delete quotes using quote ID. It is the main access point to perform CRUD operations on carts at the database level. |
QuoteIdMaskFactory | Facilitates generation of secure, masked cart IDs for guest users. These IDs prevent direct exposure of internal quote IDs in the frontend or APIs. It plays a crucial role in maintaining data integrity and avoiding cart hijacking. |
Additional Supporting Components
Component | Purpose |
---|---|
\Magento\Quote\Api\Data\CartInterface | Represents the structure of the cart (quote), allowing developers to interact with cart-level data such as items, totals, customer information, and shipping methods. |
\Magento\Quote\Model\QuoteIdMask | The model that stores the relationship between internal quote IDs and masked IDs. It is used behind the scenes to look up guest cart sessions securely. |
\Magento\Quote\Api\GuestCartRepositoryInterface | Enables programmatic access to retrieve and update guest carts using the masked ID. It complements the GuestCartManagementInterface. |
\Magento\Quote\Api\CartTotalRepositoryInterface | Allows fetching and calculating totals (subtotal, tax, discount, grand total) for guest carts, essential for real-time updates on the checkout page. |
Architectural Benefits
- Security: Masked cart IDs prevent enumeration attacks and keep internal quote IDs hidden.
- Decoupled Design: Guest and customer cart logic are separated, allowing cleaner API-driven workflows.
- Performance: Stateless operation allows frontend and backend to communicate asynchronously without PHP session dependency.
- Flexibility: These interfaces work seamlessly with REST and GraphQL APIs, ideal for mobile, PWA, or multi-channel commerce solutions.
Building a Magento 2 Guest Cart Creation Module
Creating a module for guest cart creation enables seamless shopping experiences for non-logged-in users. This guide covers everything you need — from module setup to advanced concepts — ensuring full compliance with Magento 2 architecture.
Directory Structure Overview
Organize your files i n Magento’s PSR-4 compliant structure:
app/code/Vendor/GuestCart/
├── etc/
│ └── module.xml
├── registration.php
└── Block/
└── Cart/
└── Demo.php
Detailed Explanation:
Folder/File | Responsibility |
---|---|
etc/module.xml |
Defines module metadata, such as the module name and setup version. |
registration.php |
Registers the module with Magento's autoloading mechanism. |
Block/Cart/Demo.php |
Implements the business logic to create a guest cart via dependency injection. |
Step-by-Step Module Setup
1. registration.php
Registers your module with Magento during runtime.
<?php
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Vendor_GuestCart',
__DIR__
);
Important:
This ensures that Magento can find and activate your module during setup:upgrade
.
2. module.xml
Declares the module to the system.
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Vendor_GuestCart" setup_version="1.0.0"/>
</config>
Important:
The setup_version
is crucial. It defines when Magento needs to run new upgrade scripts (if added later).
Creating the Guest Cart Block
Build a block class to generate a guest cart using Magento's native interfaces.
3. Block/Cart/Demo.php
<?php
namespace Vendor\GuestCart\Block\Cart;
use Magento\Framework\View\Element\Template;
use Magento\Quote\Api\GuestCartManagementInterface;
class Demo extends Template
{
private GuestCartManagementInterface $guestCartManagement;
public function __construct(
Template\Context $context,
GuestCartManagementInterface $guestCartManagement,
array $data = []
)
{
parent::__construct($context, $data);
$this->guestCartManagement = $guestCartManagement;
}
/**
* Create and return a new guest cart ID.
*
* @return string
*/
public function createEmptyCart(): string
{
return $this->guestCartManagement->createEmptyCart();
}
}
Understanding GuestCartManagementInterface
Element | Description |
---|---|
createEmptyCart() | Initializes a new quote (cart) for a guest user and returns a masked cart ID. |
Stateless Operation | The guest cart is not dependent on a server-side session. Perfect for headless commerce. |
Security | Internal quote IDs are hidden to prevent misuse or data leakage. |
Behind the Scenes: What Happens During createEmptyCart()?
New Quote Entity
A new quote
record is created in the database without a customer ID.
Quote Masking
Magento generates a masked ID to replace the real quote ID for frontend/API use.
Return Masked ID
The masked ID is returned to the calling function, which is used for further cart actions like adding products or setting shipping.
Stateless Checkout
No need for PHP sessions — allows easy integration with mobile apps, PWAs, SPAs (React, Vue), or external systems.
Related Key Magento 2 Components
Component | Purpose |
---|---|
\Magento\Quote\Api\Data\CartInterface | Represents the data structure of a cart, including items, billing, shipping, and totals. |
\Magento\Quote\Model\QuoteIdMask | Stores mappings between internal quote IDs and secure masked IDs for guest operations. |
\Magento\Quote\Api\GuestCartRepositoryInterface | Enables access to retrieve and update guest carts using the masked cart ID. |
\Magento\Quote\Api\CartTotalRepositoryInterface | Provides access to calculate cart totals for guest users. |
Advanced Tips: Improving Your Guest Cart Module
1. Caching Strategies
For performance optimization, cache masked cart IDs associated with the guest session/token to avoid repeated creation requests.
2. API Customization
Build custom REST or GraphQL endpoints around GuestCartManagementInterface
to provide:
- Instant cart creation
- Add products directly to the newly created guest cart
- Set shipping and billing addresses
3. Error Handling
Implement robust error-handling patterns.
- Check if cart creation fails due to database lock or resource issue.
- Validate masked ID before performing cart operations.
Use Magento's \Magento\Framework\Exception\LocalizedException to return user-friendly error messages.
4. Logging Guest Cart Creation
Inject \Psr\Log\LoggerInterface
to log cart creation activities for debugging and monitoring:
$this->logger->info('Guest cart created with ID: ' . $maskedCartId);
Best Practices Checklist
Practice | Reason |
---|---|
Always use masked IDs | Avoid security risks like direct database enumeration. |
Validate cart existence before creating a new one | Prevent duplicate carts for the same guest. |
Secure APIs properly | Authenticate and validate masked IDs in custom endpoints. |
Cleanup abandoned carts regularly | Reduce database clutter and improve performance. |
Full Lifecycle: From Cart Creation to Checkout
- Create an empty cart → Receive masked cart ID.
- Add products using the masked cart ID.
- Set shipping address and method.
- Apply discounts or promotions.
- Set billing address.
- Submit cart for order placement.
Each step must operate based on the masked ID, ensuring that guest sessions stay isolated and secure.
Database Changes When Creating a Guest Cart
Creating a guest cart in Magento impacts multiple database tables, ensuring secure cart handling for non-logged-in users. Magento assigns a unique masked cart ID, preventing direct exposure of internal quote IDs.
Main Tables Affected
Table Name | Purpose |
---|---|
quote | Stores cart details; guest carts have customer_id = NULL . |
quote_address | Stores billing/shipping addresses linked to the cart. |
quote_id_mask | Maps masked cart IDs to internal quote_id for security. |
What Happens Internally?
When calling createEmptyCart()
, Magento performs the following actions:
- Inserts a new row into the
quote
table withis_active = 1
andcustomer_id = NULL
. - Adds corresponding entries into
quote_address
for shipping and billing details. - Adds corresponding entries into
quote_address
for shipping and billing details. - Generates and stores a masked cart ID in
quote_id_mask
, making it safe for frontend exposure.
Practical Use Cases for Guest Cart Creation
Guest carts allow flexibility in eCommerce operations, particularly in headless commerce and customer experience optimization.
Scenario | Benefit |
---|---|
Headless Storefronts | Frontend SPAs (Single Page Applications) can create carts without requiring user authentication. |
Progressive Checkout Flows | Initialize a cart early and allow users to add products, addresses, or other details later. |
API-First Integrations | Mobile apps can create carts via REST/GraphQL before login, enabling seamless shopping experiences. |
A/B Testing on Cart Experience | Test multiple checkout and cart workflows for anonymous visitors to optimize conversion rates. |
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
In Magento 2.4.7 (2025), creating a Guest Empty Cart programmatically is easier, more secure, and fully aligned with modern service contracts using the GuestCartManagementInterface. With a simple method call to createEmptyCart(), you can instantly generate a new quote for anonymous customers, safely masked with a unique cart ID.
This process automatically updates the core quote, quote_address, and quote_id_mask tables in the database without exposing sensitive information like the internal quote_id. It's the preferred approach for headless storefronts, progressive checkout flows, mobile apps, and any anonymous customer journeys.
By following best practices — such as using dependency injection, securing masked IDs, caching guest carts where necessary, and preparing for customer assignment — developers can build reliable, scalable, and future-proof guest cart systems.
Mastering guest cart creation not only improves user experience but also strengthens your Magento store’s ability to support modern eCommerce strategies.
FAQs
What is a Guest Empty Cart in Magento 2?
A Guest Empty Cart is a shopping cart created for an anonymous (non-logged-in) user without any products initially added.
Which Magento 2 interface is used to create a Guest Cart?
The GuestCartManagementInterface is used to create a guest empty cart programmatically.
How do you create a Guest Empty Cart in Magento 2.4.7?
Inject the GuestCartManagementInterface into your class and call the createEmptyCart() method.
What happens after calling createEmptyCart()?
A new record is automatically inserted into the quote, quote_address, and quote_id_mask tables in the database.
Is it secure to create Guest Carts programmatically?
Yes, Magento automatically masks the quote ID using quote_id_mask to ensure security for guest users.
Where is the GuestCartManagementInterface located?
It is located in Magento\Quote\Api\GuestCartManagementInterface namespace.
Can you create multiple Guest Carts programmatically?
Yes, you can create multiple guest carts by repeatedly calling the createEmptyCart() method.
Is a quote created with createEmptyCart() linked to a customer account?
No, it is linked to an anonymous session until the customer logs in or checks out.
Do we need a module to implement Guest Empty Cart creation?
It's recommended to implement it within a custom module to maintain Magento's coding standards and scalability.
What database tables are updated when a Guest Cart is created?
The quote, quote_address, and quote_id_mask tables are updated automatically.
Is dependency injection mandatory for Guest Cart creation?
Yes, injecting GuestCartManagementInterface into your class constructor is the recommended and cleanest approach.
Can the Guest Cart be converted to a customer cart later?
Yes, Magento can associate the cart with a customer once the guest logs in during checkout.
Is this method recommended for headless Magento setups?
Absolutely, it is highly recommended for headless storefronts, PWA, and mobile apps to manage anonymous cart sessions efficiently.
Does Magento 2.4.7 improve Guest Cart performance?
Yes, Magento 2.4.7 optimizes guest cart creation with better database indexing and API efficiency compared to older versions.
What are the best practices when handling Guest Carts programmatically?
Always use service contracts, secure masked IDs, validate sessions properly, and clean up abandoned guest carts periodically.