Magento 2: Update Product Stock Inventory Using REST API
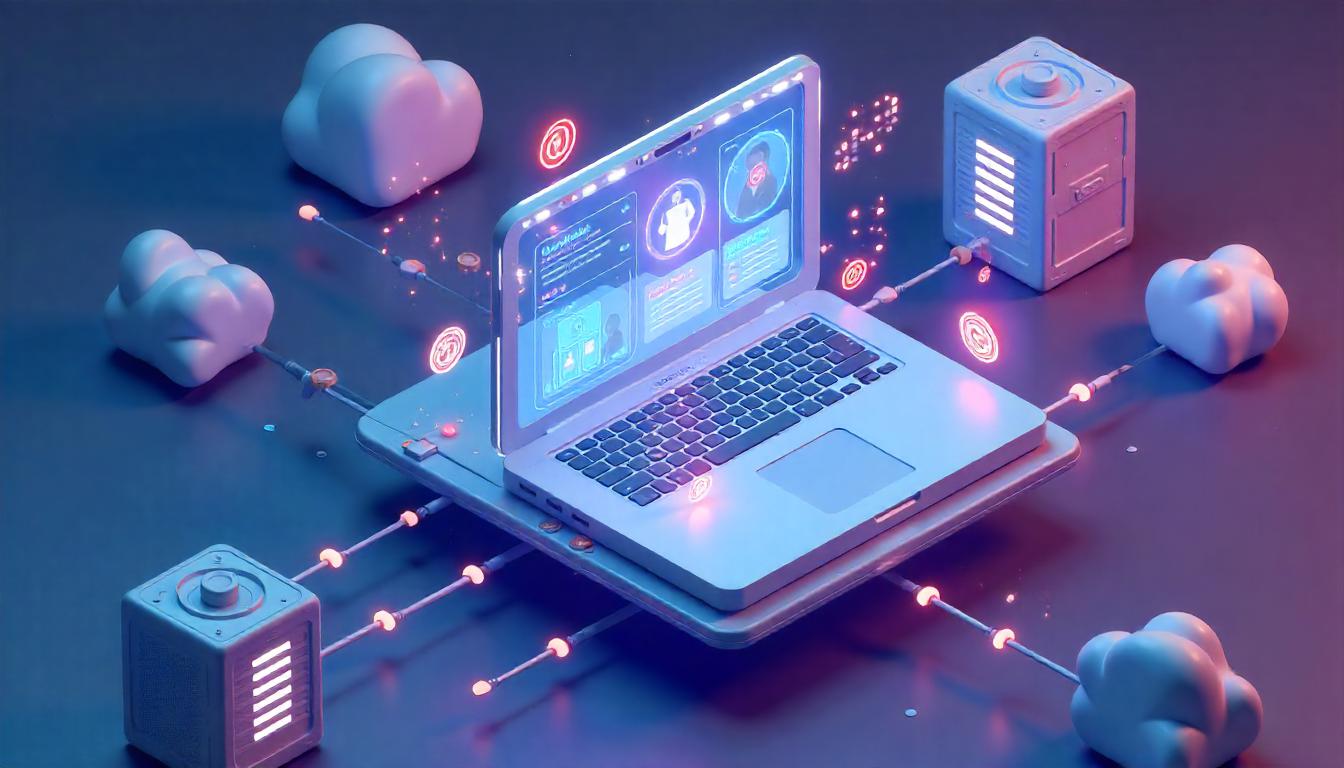
Magento 2: Update Product Stock Inventory Using REST API
Managing product stock inventory in Magento 2 is an essential part of ensuring accurate product availability in your store. This guide demonstrates how to update product stock inventory using Magento 2’s REST API, providing clear steps, payload details, and tips for efficient stock management.
Table Of Content
Overview of the REST API Endpoint
The REST API in Magento 2 provides a robust and standardized way to interact with various components of your store, including managing product stock inventory. This ensures seamless communication between external systems and your Magento store for tasks such as updating inventory data.
Key REST API Endpoint for Updating Product Stock Inventory
Endpoint:
/V1/products/:productSku/stockItems/:itemId
This endpoint is specifically used to update stock inventory for a given product identified by its SKU and stock item ID.
Request Details
HTTP Method
PUT
- This method is used to update the inventory details for the specified product.
Header
To authenticate the request, include the following authorization header:
Authorization: Bearer <ADMIN_TOKEN>
Base URL Example
An example of how this endpoint can be structured with a sample base URL:
https://magento246.test/rest/V1/products/24-MB01/stockItems/90
Request Body
To update product stock inventory, send a JSON payload in the request body with the necessary details. Below is an example payload:
{
"stockItem": {
"qty": 50,
"is_in_stock": true,
"manage_stock": true,
"use_config_manage_stock": false
}
}
Explanation of Parameters:
Field | Type | Description |
---|---|---|
qty | Integer | Specifies the quantity of the product in stock. |
is_in_stock | Boolean | Indicates whether the product is in stock (true for in stock). |
manage_stock | Boolean | Specifies if inventory management is enabled for the product. |
use_config_manage_stock | Boolean | Determines if the default inventory management configuration is used. |
Example Request
Below is an example of the complete cURL request:
curl -X PUT "https://magento246.test/rest/V1/products/24-MB01/stockItems/90" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <ADMIN_TOKEN>" \
-d '{
"stockItem": {
"qty": 50,
"is_in_stock": true,
"manage_stock": true,
"use_config_manage_stock": false
}
}'
Example Response
A successful response returns a 200 HTTP status code with updated stock item data:
{
"itemId": 90,
"productId": 24,
"stockId": 1,
"qty": 50,
"is_in_stock": true,
"is_qty_decimal": false,
"manage_stock": true,
"use_config_manage_stock": false
}
Steps to Update Product Stock Inventory in Magento 2
Updating product stock inventory in Magento 2 involves several steps to ensure proper synchronization between the product and its stock values. Below is a detailed breakdown of the steps required for updating stock items through Magento's REST API.
Step 1: Generate Admin Token
Before making any API requests, you need to authenticate and authorize yourself using an admin token. This token is required for accessing protected API endpoints.
Action: Use the POST /V1/integration/admin/token
API endpoint to generate an admin token.
- HTTP Method: POST
- Endpoint:
/V1/integration/admin/token
- Request Body:
{
"username": "admin",
"password": "admin_password"
}
- Response:
"admin_token"
This admin token will be used for authentication in the subsequent API requests.
Step 2: Fetch itemId for the Product
Once the admin token is ready, the next step is to retrieve the itemId
associated with the product SKU. This ID is necessary to perform stock updates for the product.
Action: Use the GET /V1/stockItems/:SKU
API endpoint to fetch the itemId
for a given product SKU.
- HTTP Method: GET
- Endpoint:
/V1/stockItems/:SKU
- Example Request:
GET https://magento2.example.com/rest/V1/stockItems/24-MB01
- Response:
{
"itemId": 90,
"productId": 24,
"stockId": 1,
"qty": 100,
"is_in_stock": true,
"manage_stock": true
}
From this response, the itemId
(90) will be used in the next step to update the stock details.
Step 3: Prepare Payload for Stock Update
In this step, you need to craft a JSON payload that will contain the stock information for the product, including its quantity, stock status, and other related attributes.
Here is an example of a typical payload to update stock inventory:
- Request Body:
{
"stockItem": {
"qty": 50,
"is_in_stock": true,
"manage_stock": true,
"use_config_manage_stock": false
}
}
- Explanation:
qty:
Quantity of the product in stock.is_in_stock:
Boolean value indicating if the product is in stock.manage_stock:
Boolean value indicating if inventory management is enabled.use_config_manage_stock:
Boolean value to decide if the default Magento inventory configuration is used.
Step 4: Send the PUT Request
Now, you can proceed to send the PUT request to update the product stock details using the following endpoint.
Action: Use the PUT /V1/products/:productSku/stockItems/:itemId
endpoint to update the product's stock.
- HTTP Method: PUT
- Endpoint:
/V1/products/:productSku/stockItems/:itemId
- Example Request:
curl -X PUT "https://magento2.example.com/rest/V1/products/24-MB01/stockItems/90" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer admin_token" \
-d '{
"stockItem": {
"qty": 50,
"is_in_stock": true,
"manage_stock": true,
"use_config_manage_stock": false
}
}'
- Response:
{
"message": "Stock item updated successfully"
}
The product's stock information is now updated in Magento 2.
Step 5: Verify Updates
Finally, it's essential to verify that the stock updates have been successfully applied. You can use the GET /V1/stockItems/:SKU
endpoint to retrieve the updated stock information for the product.
Action: Use the GET /V1/stockItems/:SKU
API endpoint to confirm the updated stock values.
- HTTP Method: GET
- Endpoint:
/V1/stockItems/:SKU
- Example Request:
GET https://magento2.example.com/rest/V1/stockItems/24-MB01
- Response:
{
"itemId": 90,
"productId": 24,
"stockId": 1,
"qty": 50,
"is_in_stock": true,
"manage_stock": true,
"use_config_manage_stock": false
}
Summary of the Steps
Step | Component | Description |
---|---|---|
1 | Generate Admin Token | Generate an authentication token to authorize API requests. |
2 | Fetch itemId for Product | Retrieve the itemId for a given SKU, which is required to update stock. |
3 | Prepare Payload for Stock Update | Create a JSON payload containing stock information to be sent in the update request. |
4 | Send the PUT Request | Use the PUT request to update the stock inventory using the product's SKU and itemId. |
5 | Verify Updates | Use the GET request to verify that the stock data was successfully updated. |
By following these steps, you can efficiently update product stock inventory in Magento 2 using the REST API.
Sample Payload Structure
The payload for updating stock should adhere to the following structure:
{
"stockItem": {
"product_id": 101,
"stock_id": 1,
"qty": 100,
"is_in_stock": true,
"is_qty_decimal": false,
"use_config_min_qty": true,
"min_qty": 0,
"use_config_min_sale_qty": true,
"min_sale_qty": 1,
"use_config_max_sale_qty": true,
"max_sale_qty": 1000,
"use_config_backorders": true,
"backorders": 0,
"use_config_notify_stock_qty": true,
"notify_stock_qty": 1,
"use_config_qty_increments": true,
"qty_increments": 0,
"use_config_enable_qty_inc": true,
"enable_qty_increments": false,
"use_config_manage_stock": true,
"manage_stock": true
}
}
Key Payload Parameters
The payload includes several fields critical for stock management. Below is a table summarizing these parameters:
Field | Description | Example Value |
---|---|---|
product_id | The ID of the product to update stock data for. | 101 |
stock_id | The stock source identifier. | 1 |
qty | The quantity of the product available in stock. | 100 |
is_in_stock | Boolean to indicate if the product is in stock. | true |
is_qty_decimal | Whether the product quantity can be fractional. | false |
use_config_min_sale_qty | Use default minimum sale quantity configuration. | true |
min_sale_qty | Minimum quantity allowed for sale. | 1 |
use_config_manage_stock | Use default stock management configuration. | true |
manage_stock | Boolean to enable or disable stock management. | true |
Sample API Call
Below is an example of a PUT
request to update the product stock:
curl -X PUT "https://magento2.example.com/rest/V1/products/24-MB01/stockItems/90" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer admin_token" \
-d '{
"stockItem": {
"qty": 50,
"is_in_stock": true,
"manage_stock": true,
"use_config_manage_stock": false
}
}'
Best Practices
- Error Handling: Always handle errors gracefully and provide meaningful messages in your integration.
- Data Validation: Validate your payload to ensure all required fields are populated correctly.
- Test Environment: Perform updates in a staging environment before applying changes to the production environment.
By following these guidelines and utilizing the provided payload structure, you can efficiently manage product stock in Magento 2 through its REST API.
Example API Requests
Here are some example API requests commonly used to manage stock and inventory in Magento 2. These requests help with tasks like updating stock quantities, marking products in or out of stock, and retrieving stock information.
1. Update Stock Quantity for a Product
Scenario: You need to increase the stock of a product to 500 and mark it as in stock.
Request Payload:
{
"stockItem": {
"product_id": 101,
"stock_id": 1,
"qty": 500,
"is_in_stock": true,
"manage_stock": true
}
}
2. Mark Product as Out of Stock
Scenario: You need to mark a product (SKU: 24-MB01) as out of stock.
Request Payload:
{
"stockItem": {
"product_id": 101,
"stock_id": 1,
"qty": 0,
"is_in_stock": false,
"manage_stock": true
}
}
3. Fetch Current Stock Details
Scenario: Retrieve the current stock details (quantity and status) for a product with SKU 24-MB01
.
API Request:
GET /V1/stockItems/24-MB01
Common Use Cases for Stock API
Here are some common use cases along with the API details and example scenarios:
Use Case | API Endpoint | Example |
---|---|---|
Update stock quantity | PUT /V1/products/:sku/stockItems/:itemId | Increase quantity of SKU 24-MB01 to 500. |
Mark product as out of stock | PUT /V1/products/:sku/stockItems/:itemId | Set is_in_stock to false for product SKU 24-MB01. |
Fetch current stock details | GET /V1/stockItems/:sku | Get stock quantity and status of SKU 24-MB01. |
Bulk update stock quantities | POST /V1/products/stockItems (requires custom implementation) | Update multiple SKUs in a single request. |
Each of these use cases helps you manage your stock levels in Magento 2, whether you're adjusting quantities for a single product, marking products in or out of stock, or performing bulk updates.
Explanation of Payload Fields
When you send a request to update stock information, it’s important to understand the payload structure. Below is a breakdown of each field included in the stock update payload.
Field | Description | Example Value |
---|---|---|
product_id | The ID of the product to update stock data for. | 101 |
stock_id | The stock source identifier. | 1 |
qty | The quantity of the product available in stock. | 500 |
is_in_stock | Boolean to indicate if the product is in stock. | true |
is_qty_decimal | Whether the product quantity can be fractional. | false |
manage_stock | Whether stock management is enabled for the product. | true |
use_config_min_qty | Use default minimum quantity configuration. | true |
min_qty | Minimum quantity allowed for sale. | 0 |
use_config_min_sale_qty | Use default minimum sale quantity configuration. | true |
min_sale_qty | Minimum quantity allowed for sale. | 1 |
use_config_max_sale_qty | Use default maximum sale quantity configuration. | true |
max_sale_qty | Maximum quantity allowed for sale. | 1000 |
use_config_backorders | Use default backorder configuration. | true |
backorders | Defines backorder behavior. | 0 |
use_config_notify_stock_qty | Use default notification for low stock quantity. | true |
notify_stock_qty | The threshold for when to notify low stock. | 1 |
use_config_qty_increments | Use default quantity increment configuration. | true |
qty_increments | Increment value for stock quantity (if applicable). | 0 |
use_config_enable_qty_inc | Use default configuration to enable quantity increment. | true |
enable_qty_increments | Enable or disable quantity increments. | false |
This breakdown will help you better understand how to use the stock API efficiently and make the necessary adjustments based on your product’s stock and sales strategy.
Enhancing Stock Management with Magento 2 APIs
Managing stock effectively is critical for smooth eCommerce operations. Below, you'll find practical solutions to common issues, best practices, and a comparison of stock update methods.
Troubleshooting Common Issues
Identifying and resolving issues while updating stock data is essential to maintain accuracy. Here's a quick guide:
Common Issues and Resolutions
Issue | Cause | Resolution |
---|---|---|
401 Unauthorized | Invalid or missing admin token. | Regenerate the admin token. |
404 Not Found | Incorrect SKU or itemId. | Verify the endpoint and parameters. |
400 Bad Request | Invalid payload format. | Ensure the JSON payload follows the correct structure. |
No changes visible in frontend | Cache not refreshed. | Clear Magento cache after updating stock data. |
Stock Management Best Practices
Follow these guidelines to ensure smooth and reliable stock updates:
Automate Stock Updates
Use cron jobs or scripts to periodically update stock via REST API, ensuring real-time accuracy.
Use Transactional Locks
Prevent simultaneous stock updates to avoid inventory mismatches.
Enable Notifications
Configure low-stock alerts to proactively address inventory shortages.
Verify SKU Accuracy
Double-check SKUs and product IDs to ensure they align with your Magento catalog.
Monitor API Logs
Regularly review logs to identify and fix failed or incomplete requests.
Stock Update Comparison Table
Here's a comparison of various stock update methods based on their use cases, advantages, and drawbacks:
Method | Use Case | Pros |
---|---|---|
REST API | Automating stock updates programmatically. | Scalable, Fast updates |
Admin Panel | Manual updates for individual products. | Easy for small catalogs |
Third-party Extensions | Advanced inventory management with additional features. | Robust and feature-rich |
Additional Tips
API Security
Ensure all API requests are secured with proper authentication tokens.
Testing Environments
Test all stock updates in a staging environment before applying them to the live site.
Regular Backups
Back up your catalog data regularly to prevent data loss during updates.
Integrate with ERP Systems
Synchronize Magento inventory with external systems for seamless stock management.
By following these practices and leveraging Magento 2 APIs effectively, you can optimize inventory management, minimize errors, and enhance overall store performance.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Managing product stock inventory is crucial for maintaining a well-functioning Magento 2 store. The REST API provides a robust and scalable solution to streamline this process, offering the flexibility to update stock levels, toggle availability, and implement automated workflows.
By following the outlined steps—fetching product details, crafting accurate payloads, and handling API requests—you can ensure your inventory is always up-to-date. Additionally, adhering to best practices like enabling notifications, monitoring logs, and automating updates can significantly enhance efficiency and reduce errors.
Whether you manage a small catalog or operate at scale, the REST API's capabilities empower you to maintain an optimized inventory system, providing a seamless shopping experience for your customers while reducing operational overhead.
FAQs
What is the purpose of the REST API in Magento 2?
The REST API in Magento 2 allows developers to interact programmatically with Magento, enabling tasks like updating inventory, fetching product details, and managing store operations seamlessly.
How can I update product stock inventory in Magento 2?
You can update product stock inventory using the REST API endpoint /V1/products/:productSku/stockItems/:itemId
with a PUT request and the appropriate payload.
What is the structure of the payload for updating stock inventory?
The payload contains fields like qty
, is_in_stock
, and manage_stock
to specify product quantity, availability status, and stock management settings.
How do I fetch the stock item ID in Magento 2?
You can fetch the stock item ID by making a GET request to the endpoint /V1/stockItems/:SKU
, which retrieves stock-related details for the given product SKU.
What HTTP method is used for updating product stock?
The HTTP method used for updating product stock inventory is PUT, which allows modifying existing resources on the server.
What is the significance of the field is_in_stock
in the payload?
The is_in_stock
field determines whether the product is available for sale. Setting it to true
makes the product in stock, while false
marks it as out of stock.
Can I manage stock increments using the REST API?
Yes, you can manage stock increments by setting the fields use_config_qty_increments
and qty_increments
in the API payload.
How can I handle API authentication in Magento 2?
API authentication is handled using an admin token in the Authorization header. For example: Authorization: Bearer <ADMIN_TOKEN>
.
What happens if the stock update API fails?
If the stock update API fails, you should check the error message in the response, verify your payload and authentication, and ensure that the endpoint URL is correct.
What is the manage_stock
field used for?
The manage_stock
field indicates whether stock management is enabled for the product. Setting it to true
enables stock tracking.
Can I automate stock updates using the Magento 2 REST API?
Yes, you can automate stock updates by integrating the Magento 2 REST API with external systems, using scripts or third-party tools to send API requests periodically.
Why is updating stock inventory important in Magento 2?
Updating stock inventory ensures accurate availability information for customers, prevents overselling, and improves operational efficiency in managing the store's catalog.