How to Programmatically Create a Product Using Magento 2.4.7 REST API (2025 Guide)
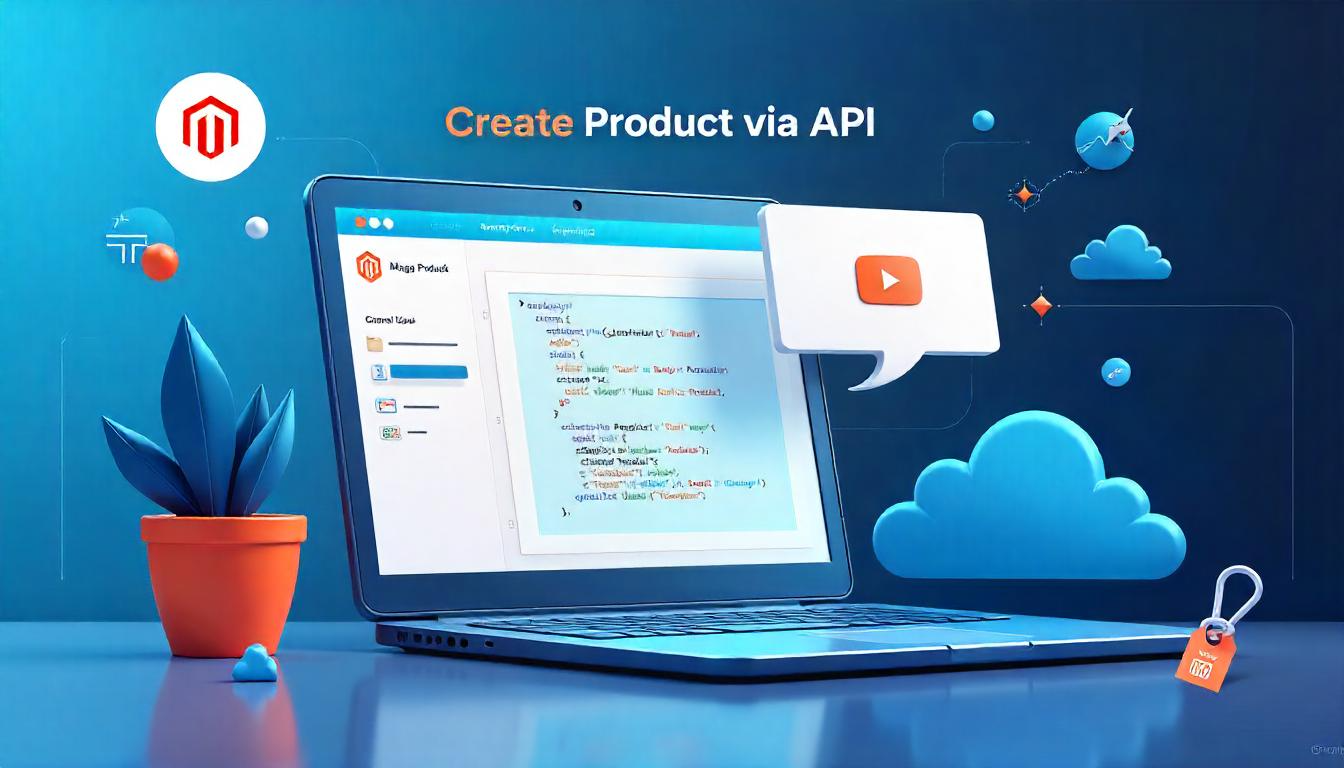
How to Programmatically Create a Product Using Magento 2.4.7 REST API (2025 Guide)
Magento 2.4.7 (2025 release) continues to support powerful integration capabilities through its REST APIs. One of the most common tasks when integrating Magento with external systems is creating products programmatically. This guide explains how to use the /V1/products
REST endpoint to create a simple product without accessing the Magento admin panel.
Table Of Content
Magento 2.4.7 Product Update API: Full Setup and Pre-requisites
Before making API calls to update or manage product information, ensure your environment meets all technical and structural requirements.
1. Platform and Compatibility Overview
Requirement | Description |
---|---|
Magento Version | Magento Open Source or Adobe Commerce 2.4.7, tested for 2025 compatibility. Includes support for PHP 8.2 and Elasticsearch 8.x. |
PHP & Composer | PHP 8.2 with all required extensions. Composer 2.x should be installed for dependency management and module updates. |
Web Server | Apache 2.4 or Nginx configured to allow public access to /rest endpoints and support for .htaccess or nginx.conf rules. |
2. Authentication and Security Configuration
Requirement | Description |
---|---|
Access Credentials | Required for token generation. You can use admin login/password or an integration-based authentication key. |
Token Generation | Use the endpoint: POST /rest/V1/integration/admin/token with valid credentials to retrieve a bearer token. |
OAuth Option | OAuth 1.0a can be configured for advanced multi-app integrations, though Bearer Token is preferred for internal scripts. |
Token Expiry | By default, tokens do not expire unless manually revoked or if the session is invalidated. Keep secure. |
3. Store-Specific Parameters
Parameter | Description |
---|---|
Store Code | Default is default . For multi-store views, use the respective code (e.g., en , fr , store_uk ). Use all for global updates. |
Website & Store View Context | Ensure your updates are aligned with the right store context, especially for attributes like name , meta_title , and price . |
Attribute Set ID | Defines the group of attributes associated with the product. Use API endpoint /V1/eav/attribute-sets/list to fetch available sets. |
Category ID(s) | Products can be linked to one or multiple category IDs. Fetch these from the /V1/categories tree structure API. |
4. REST Client and Testing Tools
Tool | Purpose |
---|---|
Postman | GUI-based API client for testing endpoints and inspecting JSON responses. |
cURL | Command-line tool for quick testing and automation via shell scripts. |
PHP Script | Ideal for server-side integrations using Magento backend modules or scheduled tasks. |
API Testing Best Practice | Always test on a staging environment before executing in production. Validate each field update with the correct store view. |
5. Performance and Best Practices
Consideration | Details |
---|---|
Batch Processing | For bulk updates, use custom scripts or the bulk/V1/products endpoint. |
Field Validation | Ensure all values conform to attribute constraints (e.g., type, range, requirement). |
Error Handling | Handle common HTTP codes (400, 401, 403, 404, 415, 500) gracefully in scripts or frontend. |
API Rate Limiting | Respect server limits if using third-party clients. Magento does not enforce strict rate limiting but overuse may trigger firewalls. |
Logging & Monitoring | Log all API requests and responses. Use Magento logs or external tools to monitor API traffic. |
Magento 2.4.7 API Integration Guide: Creating a Simple Product
This guide explains how to programmatically authenticate and create a simple product in Magento 2.4.7 using REST API. It includes access token generation, product creation endpoints, and sample cURL and PHP scripts for automation.
Section 1: Authentication via Admin Token
To make authenticated API calls, you must first generate an Admin Access Token.
Endpoint
POST /rest/V1/integration/admin/token
Required Payload
{
"username": "admin",
"password": "admin123"
}
Example cURL Command
curl -X POST "http://yourdomain.com/rest/V1/integration/admin/token" \
-H "Content-Type: application/json" \
-d '{"username": "admin", "password": "admin123"}'
Response
"your-access-token"
This token must be included in all subsequent API requests in the Authorization
header as:Authorization: Bearer your-access-token
Section 2: Creating a Simple Product via REST API
Once authenticated, you can create a new product using the /V1/products endpoint.
Endpoint
POST /rest/all/V1/products
The use of /all
ensures the product is available across all websites in a multi-store setup.
Required Headers
Content-Type: application/json
Authorization: Bearer your-access-token
Example JSON Payload
{
"product": {
"sku": "test-product-1",
"name": "Test Product 1",
"attribute_set_id": 4,
"price": 99.00,
"status": 1,
"visibility": 4,
"type_id": "simple",
"weight": 1,
"extension_attributes": {
"category_links": [
{ "position": 0, "category_id": "5" },
{ "position": 1, "category_id": "7" }
],
"stock_item": {
"qty": 1000,
"is_in_stock": true
}
},
"custom_attributes": [
{ "attribute_code": "description", "value": "Long product description goes here." },
{ "attribute_code": "short_description", "value": "Short summary of the product." }
]
}
}
Section 3: Automating Product Creation with PHP (cURL)
The following PHP script performs the full workflow: authenticating and creating a product programmatically.
<?php
$url = "http://yourdomain.com/rest/all";
$token_url = "$url/V1/integration/admin/token";
$username = "admin";
$password = "admin123";
// Step 1: Generate Access Token
$ch = curl_init();
$data = json_encode(["username" => $username, "password" => $password]);
curl_setopt($ch, CURLOPT_URL, $token_url);
curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, ['Content-Type: application/json']);
$token = curl_exec($ch);
$accessToken = json_decode($token);
curl_close($ch);
// Step 2: Prepare Product Data
$apiUrl = "$url/V1/products";
$headers = [
"Content-Type: application/json",
"Authorization: Bearer $accessToken"
];
$productData = json_encode([
"product" => [
"sku" => "test-product-1",
"name" => "Test Product 1",
"attribute_set_id" => 4,
"price" => 99,
"status" => 1,
"visibility" => 2,
"type_id" => "simple",
"weight" => 1,
"extension_attributes" => [
"category_links" => [
["position" => 0, "category_id" => "5"],
["position" => 1, "category_id" => "7"]
],
"stock_item" => ["qty" => 1000, "is_in_stock" => true]
],
"custom_attributes" => [
["attribute_code" => "description", "value" => "This is a test product."],
["attribute_code" => "short_description", "value" => "Short test product."]
]
]
]);
// Step 3: Create Product via API
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $apiUrl);
curl_setopt($ch, CURLOPT_POSTFIELDS, $productData);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
$response = curl_exec($ch);
curl_close($ch);
// Step 4: Output API Response
print_r(json_decode($response));
</?php
Section 4: Reference Table – Product Visibility Options
Value | Description |
---|---|
1 | Not visible individually |
2 | Catalog only |
3 | Search only |
4 | Catalog and Search |
Product Field Explanation
This section provides a detailed explanation of the key fields used when creating or updating products in the Magento system. These fields represent product characteristics, stock information, and other key attributes.
Field | Type | Description | Example |
---|---|---|---|
sku | string | Unique product identifier that distinguishes this product from others in the catalog. | "test-product-1" |
name | string | The name of the product as displayed on the product page and catalog. | "Test Product 1" |
price | float | The price of the product excluding taxes. This is what customers will pay for the product. | 99.00 |
attribute_set_id | integer | The ID of the attribute set used for the product. The default ID is 4 for most products. | 4 |
status | integer | Defines whether the product is enabled or disabled. "1" indicates the product is enabled, "2" means disabled. | 1 |
visibility | integer | Defines where the product will appear: "1" for not visible, "2" for catalog only, "3" for search only, "4" for both catalog and search. | 4 |
type_id | string | The product type (e.g., simple, configurable). Simple products are individual items, while configurable products may have options like sizes or colors. | "simple" |
weight | float | The weight of the product, typically used for shipping calculations. | 1.0 |
category_links | array | The categories that the product belongs to. Multiple category IDs can be provided. | [5, 7] |
stock_item | array | Information about the product’s stock status, including quantity and availability. | qty: 1000, is_in_stock: true |
custom_attributes | array | Any additional attributes defined for the product, like description, short description, etc. | "description", "short_description" |
Detailed Field Descriptions
sku (Stock Keeping Unit)
- Type: string
- Purpose: This is the unique identifier for each product in the system. Every product in Magento should have a unique SKU to differentiate it from other products in the catalog.
name
- Type: string
- Purpose: The product's name is the title that will be shown on the product page and in search results on the site. It's essential for SEO and user experience.
price
- Type: float
- Purpose: The cost of the product, before taxes. This is what customers pay when purchasing the product. Pricing can be adjusted for promotions, discounts, and other sales activities.
attribute_set_id
- Type: integer
- Purpose: This ID identifies which attribute set the product belongs to. Different types of products can have different attribute sets based on their characteristics (e.g., clothing may have size and color attributes).
status
- Type: integer
- Purpose: Indicates whether the product is visible on the store. A status of "1" means the product is active, while "2" means it is disabled and not visible to customers.
visibility
- Type: integer
- Purpose: Determines where the product will appear in the catalog.
- Options:
- 1: Not visible individually
- 2: Catalog only
- 3: Search only
- 4: Both catalog and search
type_id
- Type: string
- Purpose: Defines the type of the product (e.g., "simple" for individual products, "configurable" for products with multiple options).
weight
- Type: float
- Purpose: The weight of the product, which is typically used to calculate shipping costs and determine shipping methods.
category_links
- Type: array
- Purpose: A list of category IDs that the product belongs to. Categories help organize products on the site and assist in navigation and filtering.
stock_item
- Type: array
- Purpose: Contains inventory details, including the quantity available for sale and whether the product is currently in stock.
custom_attributes
- Type: array
- Purpose: Allows you to add custom attributes to products, such as detailed descriptions, color, size, and any other product-specific information.
By understanding and correctly populating these fields, you can ensure that your product information is accurately represented in your Magento store, improving both backend management and customer experience.
API Product Creation: Best Practices and Implementation Guide
Recommended Tips for Product API Management
Tip ID | Recommendation |
---|---|
TIP 1 | Always use HTTPS for API requests to ensure secure communication and prevent data interception. |
TIP 2 | Maintain unique SKUs for each product. Duplicate SKUs cause conflicts during creation or updates. |
TIP 3 | Set visibility to 4 to ensure the product appears in both catalog listings and search results. |
TIP 4 | Validate category IDs before assigning them to prevent invalid or broken category associations. |
TIP 5 | Store access tokens in session or memory cache to reduce overhead and avoid repeated authentication calls. |
TIP 6 | Ensure that all required attributes from the assigned attribute set are included in the API payload to avoid rejection. |
Testing Tools for API Integration
To ensure successful product creation via API, testing tools can simulate real-world scenarios and catch issues early:
- REST Clients (e.g., Postman): For composing and sending REST requests with headers, body, and tokens.
- Command-Line Tools (e.g., cURL): Useful for quickly sending requests directly from the terminal.
- Automation Scripts: Use languages like PHP, Python, or JavaScript (Node.js) to script bulk operations and automate product syncing.
Implementation Summary
Creating products via the REST API in Magento 2.4.7 enables flexible, scalable catalog management. This is especially useful when integrating with external systems such as:
- Enterprise Resource Planning (ERP)
- Product Information Management (PIM)
- Custom inventory sync solutions
The API approach provides full control and bypasses manual admin work. For more advanced functionality—such as media galleries, search engine metadata, or configurable product variations—you can extend your payload by including optional or custom attribute fields.
Additional Recommendations
- Error Handling: Implement retry logic and response validation to handle failures gracefully.
- Error Handling: Implement retry logic and response validation to handle failures gracefully.
- Rate Limits: Be aware of any API rate limits and structure requests accordingly.
- Batch Processing: When creating multiple products, consider batching to optimize performance and reduce load.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Creating products programmatically in Magento 2.4.7 using the REST API empowers developers and store owners to manage catalogs more efficiently. This guide walked you through authenticating via admin tokens, constructing the correct product payload, and executing API calls using tools like Postman, cURL, and PHP scripts. These methods are crucial for businesses integrating Magento with ERP systems, CRMs, inventory databases, or external marketplaces.
By automating product creation, you reduce manual errors, save time, and ensure consistency across multiple store views and websites. Using structured JSON payloads and adhering to Magento’s field requirements—like unique SKUs, valid category IDs, and attribute sets—you maintain data integrity while scaling your product listings. Best practices, such as storing tokens securely and validating input before sending requests, further strengthen your integration’s reliability and security.
As Magento continues to evolve, REST API usage becomes an increasingly valuable skill in a developer’s toolkit. With your foundation now set, you can confidently expand into more advanced API operations—like adding media galleries, setting up configurable products, or updating inventory and pricing in bulk. Whether you're working on a one-time sync or building a fully automated backend process, the techniques covered here are a solid step toward scalable, headless Magento commerce.
FAQs
1. What is the endpoint to create a product in Magento 2.4.7?
You can use the POST endpoint /rest/all/V1/products
to create a new product programmatically.
2. Do I need admin credentials to use the product creation API?
Yes, you need valid admin credentials or an integration token with write access to use the product API.
3. What authentication method does Magento REST API use?
Magento uses token-based authentication. First, you obtain a bearer token by sending your admin credentials to the /V1/integration/admin/token
endpoint.
4. What is the default attribute_set_id in Magento?
The default attribute set ID is usually 4, but it may vary depending on your Magento setup.
5. How do I assign a product to categories using the API?
You can use the category_links
field inside extension_attributes
to assign category IDs.
6. How can I set product stock while creating it via API?
Include the stock_item
object under extension_attributes
with fields like qty
and is_in_stock
.
7. What visibility value makes the product appear in both catalog and search?
Use visibility value 4
to make products visible in both catalog and search pages.
8. What does the SKU field represent?
SKU (Stock Keeping Unit) is a unique identifier for each product and must be unique across the catalog.
9. Can I create configurable products using the same endpoint?
Yes, but you need to set type_id
to configurable
and add configuration data. This requires additional steps beyond simple product creation.
10. How do I add a product description using the API?
Use custom_attributes
to pass the description
and short_description
fields.
11. Is it possible to add product images via the REST API?
Yes, you can use the /V1/products/media
endpoint to add images after creating the product.
12. What should I do if I get a 400 or 401 error?
401 usually means invalid or missing token. 400 indicates bad request—check your JSON structure and required fields.
13. Can I update an existing product with the same endpoint?
Yes, but you must use the same SKU and a PUT request instead of POST to update an existing product.
14. How can I get the list of all attribute sets?
You can use the /V1/eav/attribute-sets/list
endpoint to retrieve all attribute sets available in your store.
15. Is there a way to bulk import products via API?
Magento Commerce supports asynchronous bulk APIs, or you can write scripts to loop through product creation requests in batches.