How to Fetch Extension Attributes Value in Order by REST API in Magento 2.4.7 (2025)
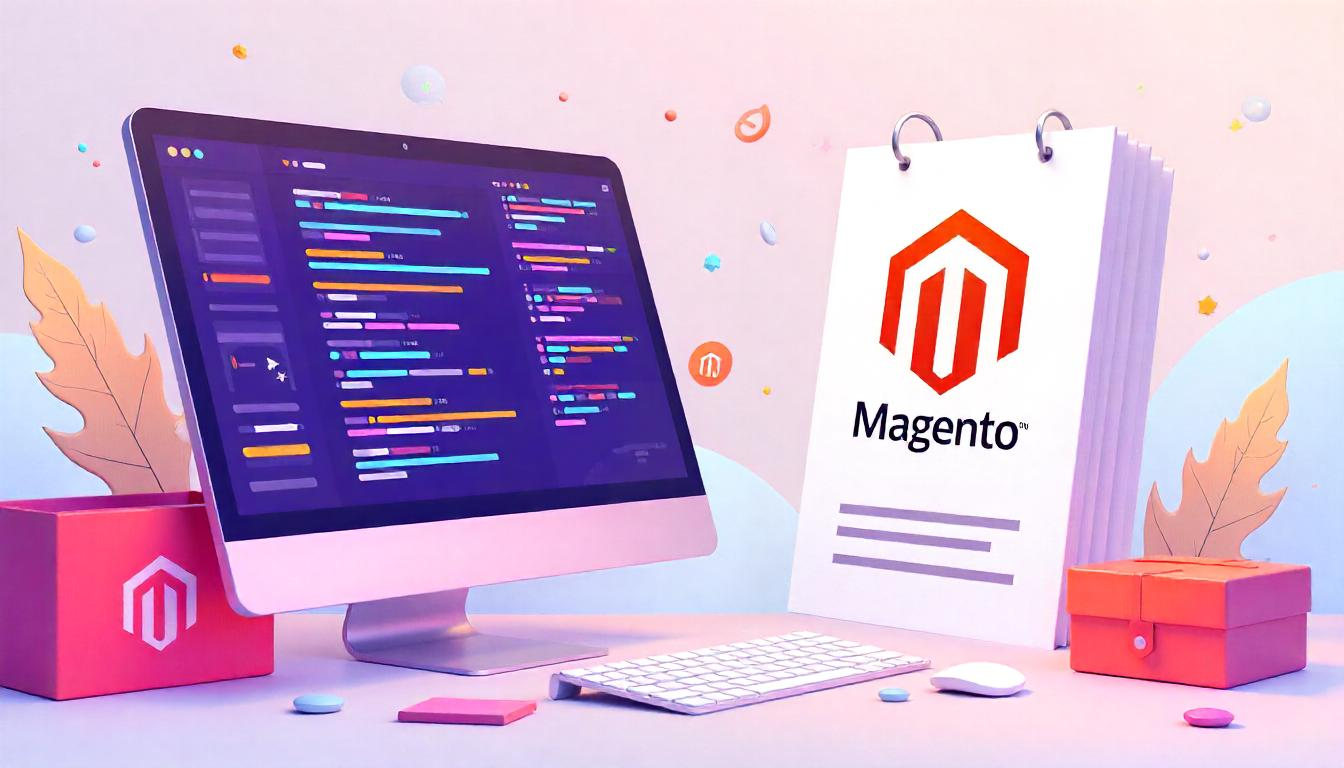
How to Fetch Extension Attributes Value in Order by REST API in Magento 2.4.7 (2025)
Magento 2's powerful Service Contracts and extensibility mechanisms allow developers to seamlessly work with custom data. When you want to fetch custom extension attribute values like an order comment in an order via REST API, you need to properly define and expose that data using Magento’s extension attribute system.
This article provides a comprehensive, updated guide (Magento 2.4.7 - 2025) for adding, fetching, and exposing custom extension attributes via API.
Table Of Content
Understanding Extension Attributes in Magento 2
In Magento 2, extension attributes are a powerful mechanism that allows developers to attach additional data to existing service contracts without modifying the core Magento classes or database schema directly. They are part of Magento's service contract design pattern and are especially useful when interacting with APIs and custom modules.
Extension attributes are ideal for cases where new data needs to be exposed or accepted via APIs, or when a module requires more flexible interaction with native entities like products, orders, customers, or quotes.
Key Characteristics of Extension Attributes
- Decoupled Architecture: Extension attributes do not require modification of core interfaces or database schema.
- API Compatibility: Automatically integrated into Magento’s REST and SOAP APIs.
- Auto-Generated Classes: Magento's code generation creates getter/setter methods for these attributes dynamically during compilation.
- Storage: They are stored in related tables—not in a generic
extension_attributes
table—ensuring optimized performance and consistent entity relationships.
How Extension Attributes Work
- Define in XML: Use
extension_attributes.xml
to specify which entity (e.g., product) you're extending and which custom attributes to bind. - Interface Binding: Attributes are attached to Data Interfaces (e.g.,
ProductInterface
) which are part of the service contracts. - Code Generation: Magento generates corresponding getter and setter methods for use in repositories and APIs.
- Persistence Logic: Data must be explicitly loaded and saved via plugin or repository logic; Magento does not persist these automatically unless configured.
Common Use Cases
- Adding custom product metadata for use in frontend or APIs.
- Extending customer entities with loyalty points or third-party data.
- Associating additional fields to orders such as tracking source, delivery preferences, or affiliate codes.
Extension Attributes vs Custom Attributes
Feature | Extension Attributes | Custom Attributes |
---|---|---|
Defined Via | extension_attributes.xml |
EAV or DB schema via install/upgrade scripts |
API Visibility | Automatically included in APIs | Only included if explicitly exposed |
Stored In | Custom tables or existing entity tables | EAV tables (catalog_product_entity_* ) |
Interface Dependency | Attached to Service Data Interfaces | Not dependent on interfaces |
Used For | API interaction, inter-module communication | Frontend/backend attribute values |
Steps to Implement Extension Attributes
Step | Description |
---|---|
Define XML | Create extension_attributes.xml under your module's etc directory. |
Extend Interface | Target a Magento data interface (e.g., ProductInterface ) and declare the new attribute. |
Create Data Model | Develop a PHP data model class that defines the extension attribute using getter and setter methods. |
Modify Repository | Use a plugin or override the repository class to handle reading and saving the attribute values. |
Test via API or Repository | Verify that the extension attribute appears in REST/SOAP API responses and is stored correctly. |
Best Practices
- Avoid Redundancy: Use extension attributes only when the data must be exposed via APIs or interfaces. For internal-only use, consider simple model-level customization.
- Encapsulation: Never store business logic inside extension attributes. Use service classes or plugins for processing.
- Error Handling: Always validate extension attribute data before saving to prevent exceptions during API or repository operations.
- Performance Impact: Be cautious about loading unnecessary attributes to avoid degrading performance in high-traffic APIs.
Comprehensive Guide to Using Extension Attributes in Magento 2
Extension attributes are designed to allow developers to extend core entities in a clean, decoupled, and upgrade-safe manner. They're especially useful for exposing additional data through APIs or for cross-module communication without touching core interfaces directly.
Introduction to Extension Attributes
In Magento 2, service contracts (interfaces for repositories and APIs) are designed to be immutable. Extension attributes offer a flexible mechanism to pass extra data through these contracts. These are configured via XML and automatically integrated with API data structures.
Key Advantages
- Upgrade-Safe: No core interface modification required.
- API-Ready: Included in REST/SOAP responses automatically.
- Modular: Respects Magento's design principles and dependency injection.
Step-by-Step Setup of an Extension Attribute
Let’s implement a custom order_comment attribute for Magento orders using extension attributes. This walkthrough assumes you're building a custom module.
Step 1: Define the Extension Attribute XML
Create the following file in your module directory:
Path:
app/code/Vendor/OrderComment/etc/extension_attributes.xml
Purpose
This registers order_comment
as an extension attribute for the Order entity.
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework:Api/etc/extension_attributes.xsd">
<extension_attributes for="Magento\Sales\Api\Data\OrderInterface">
<attribute code="order_comment" type="string" />
</extension_attributes>
</config>
Step 2: Register a Plugin to Load the Attribute
Create a plugin to ensure the order_comment
field is loaded with order data.
File:
app/code/Vendor/OrderComment/etc/di.xml
<type name="Magento\Sales\Api\OrderRepositoryInterface">
<plugin name="ordercomment_extension_attribute"
type="Vendor\OrderComment\Plugin\OrderRepositoryPlugin" />
</type>
Step 3: Create the Plugin Logic
This plugin loads order_comment
and attaches it to the extension attributes when orders are fetched.
<?php
namespace Emmo\OrderComment\Plugin;
use Magento\Sales\Api\Data\OrderExtensionFactory;
use Magento\Sales\Api\Data\OrderInterface;
use Magento\Sales\Api\Data\OrderSearchResultInterface;
use Magento\Sales\Api\OrderRepositoryInterface;
class OrderRepositoryPlugin
{
public const ORDER_COMMENT_FIELD_NAME = 'order_comment';
public function __construct(
private \Magento\Sales\Api\Data\OrderExtensionFactory $orderExtensionFactory
) {}
public function afterGet(
\Magento\Sales\Api\OrderRepositoryInterface $subject,
\Magento\Sales\Api\Data\OrderInterface $order
) {
$this->addComment($order);
return $order;
}
public function afterGetList(
\Magento\Sales\Api\OrderRepositoryInterface $subject,
\Magento\Sales\Api\Data\OrderSearchResultInterface $searchResult
) {
foreach ($searchResult->getItems() as $order) {
$this->addComment($order);
}
return $searchResult;
}
private function addComment(\Magento\Sales\Api\Data\OrderInterface $order): void
{
$comment = $order->getData(self::ORDER_COMMENT_FIELD_NAME);
$extension = $order->getExtensionAttributes() ?: $this->orderExtensionFactory->create();
$extension->setOrderComment($comment);
$order->setExtensionAttributes($extension);
}
}
Key Differences – Extension vs Custom Attributes
Criteria | Extension Attributes | Custom Attributes |
---|---|---|
API Visibility | Included automatically | Requires manual addition |
Storage | Custom table or native entity table | Stored in EAV tables |
Dependency | Tied to service contracts | Used for UI/Forms |
Best Use Case | Module communication, API interaction | Backend input and display in forms |
Common Pitfalls and Their Fixes
Problem | Cause | Solution |
---|---|---|
Attribute not shown in API | Plugin not registering correctly | Verify di.xml and plugin path |
Value returns null | Plugin didn’t attach value | Check field name and plugin logic |
Compilation fails | Invalid XML or missing method signature | Validate schema and rerun di:compile |
Additional Considerations
Access and Test the Attribute
After setup, verify that your order_comment
field shows in:
- REST API calls (
/V1/orders/:id
) - Custom repository calls in other modules
- Debug logs using
print_r($order->getExtensionAttributes()->getOrderComment())
Save Operation
This example only reads the attribute. To save data to the database, you must use a beforeSave plugin or observer to write the value from the extension attribute to the order object manually.
When Should You Use Extension Attributes?
Use them when you:
- Need to pass extra data through Magento APIs
- Want to share data between modules without altering entity interfaces
- Prefer a clean separation of logic from UI layer customization
Avoid them if:
- Your data is UI-only (admin forms, customer attributes)
- You don’t need API exposure or repository integration
Accessing Custom Attributes through REST API in Magento 2
Once you have successfully implemented the extension attribute and added it to your module, the next step is to make the custom attribute accessible via the REST API. Here’s how you can achieve that:
Step 1: Verify the Custom Attribute Setup
Ensure that the extension attribute is correctly defined in the extension_attributes.xml
file and that the appropriate plugin is in place to populate the data.
Example Configuration:
- extension_attributes.xml defines the new attribute (
order_comment
) for theOrderInterface
. - The plugin logic (attached to the
OrderRepositoryInterface
) should populate the extension attribute with the relevant data.
Step 2: Fetch Data via the REST API
Once the setup is complete, you can retrieve the custom attribute via the Magento REST API. The attribute is available in the response data when you query the order details.
API Endpoint:
- GET /rest/V1/orders/{orderId}
orderId
.Step 3: Example API Response
When you call the endpoint, the custom attribute will appear in the response in the extension_attributes
section. The output might look something like this:
{
"id": 1,
"status": "pending",
"total": 100.00,
"extension_attributes": {
"order_comment": "Please deliver after 6 PM."
}
}
Step 4: API Consumption
Any third-party system or application that has access to the API can now retrieve the order_comment attribute from the order data. This ensures that additional custom information can be passed through without altering the core Magento schema.
Best Practices for Using Custom Attributes in API
- Security: Ensure that sensitive custom attributes are appropriately protected, either by restricting access or using proper authentication mechanisms.
- Performance: When dealing with large volumes of data, avoid overloading the API response by including too many custom attributes unless necessary.
- Validation: Always validate the custom attribute data before exposing it to the API to ensure the data is clean and consistent.
Troubleshooting API Access for Custom Attributes
Attribute Not Appearing in API Response:
- Check the configuration of your
extension_attributes.xml
file and ensure that the plugin correctly populates the attribute. - Ensure that the custom attribute is added to the API data interface.
Incorrect Data in the Attribute:
- Review the logic in the plugin that populates the extension attribute to confirm it is fetching and setting the correct value.
- Check for any issues in the data source from where the attribute is derived (e.g., database, external systems).
API Endpoint Returns Empty Extension Attributes:
- Verify that the attribute is registered and accessible for the respective entity (in this case, the
OrderInterface
). - Ensure that the extension attribute is correctly linked and populated in the plugin.
Understanding Extension Attribute Value Flow in Magento 2
Extension attributes in Magento 2 allow developers to extend the default behavior of core entities (like orders, customers, and products) by adding custom attributes. This functionality is essential for creating tailored solutions without modifying the core schema. The extension attribute value flow represents the steps and components involved in defining, injecting, and accessing custom extension attributes in Magento 2.
Key Components of the Extension Attribute Value Flow
Before diving into the step-by-step process, let's first explore the main components that contribute to the flow of extension attribute values in Magento 2:
- extension_attributes.xml: This XML file is where you define custom extension attributes for Magento interfaces like OrderInterface, CustomerInterface, etc.
- Plugins: These are used to modify the behavior of repository methods (such as get(), getList()) to inject or modify extension attribute values when the entity is loaded.
- Service Contracts: These contracts allow extension attributes to be exposed in API responses and interact with other systems.
- API Responses: Once an order or product is loaded with the extension attributes, these values are included in the API response, making them accessible externally.
Extension Attribute Value Flow
Step | Component | Description |
---|---|---|
1 | extension_attributes.xml |
Defines the custom extension attribute for the interface. |
2 | Plugin for Repository Methods | Populates the extension attribute after the entity is loaded. |
3 | API Response | Exposes the extension attribute in the API response. |
Best Practices for Extension Attribute Value Flow
- Use Clear Naming Conventions: Ensure that the extension attribute names are clear and descriptive to avoid confusion in the future.
- Avoid Overuse of Custom Attributes: Limit the number of custom extension attributes to prevent unnecessary complexity and performance issues.
- Maintain Compatibility with Core Interfaces: When adding extension attributes, ensure that they don't break or conflict with core Magento functionality.
- Validate Data Before Insertion: Ensure that data is properly validated before being inserted into the extension attributes to prevent corrupt or invalid values.
Additional Considerations
- Performance: If you're dealing with large amounts of data, be mindful of how many custom attributes are being added to entities. Too many attributes can degrade performance, especially in API responses.
- Security: Ensure sensitive extension attribute values are protected by proper access control and are only available to authorized users or systems.
- Customization Flexibility: While extension attributes are a flexible way to extend Magento’s core functionality, always consider whether the attribute is necessary for the business logic before adding it to avoid unnecessary complexity.
By understanding and following the extension attribute value flow, developers can extend Magento in a clean, maintainable way without directly altering the core database schema or causing compatibility issues with future updates.
Best Practices for Working with Extension Attributes
Effective Strategies
Clear Cache After Attribute Creation:
Always remember to clear the generated cache and var/cache
directories after creating or updating an extension attribute. This ensures that your changes are reflected in the Magento system.
Leverage PHP 8 Constructor Promotion:
To simplify your plugin classes, use PHP 8 constructor promotion. This will reduce the boilerplate code in your classes and make your code more concise and readable.
Document Code with PHPDoc Comments:
Properly document your code using PHPDoc comments. This improves static analysis, provides clear descriptions of each method, and makes your code more maintainable, especially for team collaboration.
Ensure Dependency Injection Integrity:
When working with plugins and extension attributes, always validate that the necessary dependencies are correctly injected into your classes. This ensures smooth functionality and easier maintenance.
Common Mistakes to Avoid
Missing Extension Attributes on Entity:
A common mistake is forgetting to set the extension attributes on the entity before returning it. This can cause the attribute not to appear in the final response, leading to confusion or incomplete data.
Skipping Dependency Injection Compilation:
Neglecting to run the php bin/magento setup:di:compile
command after adding or modifying extension attributes can result in your new code not being applied properly. Always remember to recompile dependencies to reflect changes.
Incorrect or Missing XML Type Definitions:
Ensure that the proper types are defined in the extension_attributes.xml
file. Failing to do so can lead to errors or unexpected behavior, as Magento relies on these definitions for attribute handling.
Advanced Tips for Extension Attributes
Test Attributes with Real Data:
Always test your extension attributes with real data during development. This helps ensure that your attributes are correctly populated and exposed in API responses. Test them thoroughly in different environments (e.g., staging vs production).
Minimize Overloading:
When using extension attributes, minimize the need to overload core classes. Instead, focus on enhancing the functionality through plugins or observers that work in tandem with the existing Magento system.
Troubleshooting Extension Attributes
Missing Attributes in API Response:
If your extension attributes are not showing in API responses, double-check the plugin logic to ensure it correctly populates the extension attribute before returning the entity.
Incorrect Data Format:
If the data for the extension attribute appears but is incorrectly formatted or inconsistent, ensure the data model's getter and setter methods are properly defined to handle the data type correctly.
Extension Attribute and Performance Considerations
Keep Attributes Simple:
While extension attributes are powerful, avoid making them too complex or heavily interdependent. Keep attributes as simple as possible to maintain performance, especially when dealing with large datasets or high traffic.
Use Indexed Data Efficiently:
If your extension attributes are used frequently in queries or API responses, ensure that the data is indexed properly. Use the bin/magento indexer:reindex
command regularly to keep your indexes up to date.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
In conclusion, integrating extension attributes into Magento 2, particularly when working with REST APIs, provides a flexible and efficient method for extending core functionalities. By utilizing service contracts and defining custom extension attributes, such as adding a new "order_comment" field to the Order entity, you can tailor your Magento 2 store to meet unique business requirements. The use of plugins for enhancing order data access ensures that these extensions are seamlessly integrated into both API responses and internal data structures.
With the steps outlined, including creating the necessary extension_attributes.xml file, configuring the plugin for the OrderRepositoryInterface, and managing the custom data, Magento developers can now confidently handle extension attributes in their projects. The approach ensures data integrity, scalability, and smoother interaction with third-party applications.
By leveraging these advanced techniques, you can further enrich your Magento 2 store's API capabilities and provide enhanced customer experiences, especially in complex systems that require customized order management workflows.
FAQs
What are extension attributes in Magento 2?
Extension attributes are custom fields added to Magento entities, such as orders or products, which are not stored in the database but can be used for API extensions and custom functionalities.
How do I add a custom field to an Order entity in Magento 2?
To add a custom field to an Order entity, you need to create an `extension_attributes.xml` file in your module, and then add the custom attribute to the `OrderInterface`.
Where do I define my extension attribute in Magento 2?
Extension attributes are defined in the `extension_attributes.xml` file, located in your module's `etc` folder. You specify the entity and the custom attribute you want to add.
How can I use a custom field like "order_comment" in Magento 2?
You can create a custom `order_comment` extension attribute and access it through the `OrderRepositoryInterface` using plugins, allowing you to add the field's value to the order data.
What is the purpose of a plugin in Magento 2?
Plugins in Magento 2 are used to modify or extend the behavior of existing methods in classes without altering the core files. They allow for additional functionality to be injected into existing code.
How do I fetch extension attribute values in Magento 2?
To fetch extension attribute values, you can use the `afterGet` and `afterGetList` plugin methods, which are triggered after the order data is fetched from the repository, adding the extension attributes to the order entity.
How do I create a plugin for Magento 2?
To create a plugin in Magento 2, you define it in the `di.xml` file under the `type` node, specifying the class you want to extend and the plugin class that modifies the functionality.
What is the role of `OrderRepositoryInterface` in Magento 2?
The `OrderRepositoryInterface` in Magento 2 is responsible for managing order entities, including fetching single or lists of orders. It's often used in combination with plugins to modify or add new data to orders.
Can I add custom data to order APIs in Magento 2?
Yes, you can add custom data, such as a `order_comment`, to the order entity in the API response by creating extension attributes and using plugins to inject the data into the order API responses.
What is a `Service Contract` in Magento 2?
A service contract in Magento 2 is a set of PHP interfaces used to define the business logic for services. These contracts consist of service and data interfaces that allow clean separation of logic and ensure backward compatibility.
How do I define a new extension attribute for an entity in Magento 2?
To define a new extension attribute, you create an `extension_attributes.xml` file in your module and specify the entity (e.g., `Magento\Sales\Api\Data\OrderInterface`) and the new attribute details, such as `order_comment`.
How do plugins work with the `OrderRepositoryInterface`?
Plugins modify the behavior of the `OrderRepositoryInterface` by hooking into methods like `get` and `getList`. You can add custom logic or data to orders using the `afterGet` and `afterGetList` plugin methods.
How do I manage custom order data in Magento 2?
Custom order data can be managed by creating extension attributes and using plugins to inject the custom data into order entities. This method allows seamless integration with Magento's API and order management systems.
Can I access custom fields like `order_comment` through a third-party API?
Yes, once you add an extension attribute like `order_comment` to an order entity, it becomes accessible via any API request that fetches order data, allowing third-party systems to retrieve the custom fields.